Intro
Master Excel VBA with cell address techniques. Learn 5 ways to use cell addresses in VBA, including referencing cells, looping through ranges, and manipulating data. Discover how to use Range, Cells, and Offset properties to optimize your VBA code. Improve your Excel automation skills with these practical tips and examples.
Cell addresses are the foundation of working with data in Excel, and when combined with VBA, they become a powerful tool for automating tasks and manipulating data. In this article, we'll explore five ways to use cell addresses in VBA, from the basics to more advanced techniques.
Understanding Cell Addresses in VBA
Before we dive into the five ways to use cell addresses in VBA, let's cover the basics. In VBA, a cell address is used to refer to a specific cell or range of cells in a worksheet. Cell addresses can be expressed in two ways: using the Range object or using the Cells property.
The Range object is used to refer to a specific range of cells, such as "A1:B2" or "Sheet1!A1:B2". The Cells property, on the other hand, is used to refer to a specific cell or range of cells using its row and column numbers, such as "Cells(1, 1)" or "Cells(1, 1).Resize(2, 2)".
1. Using Cell Addresses to Select a Range of Cells
One of the most common ways to use cell addresses in VBA is to select a range of cells. This can be done using the Range object or the Cells property. Here's an example of how to select a range of cells using the Range object:
Range("A1:B2").Select
This code selects the range of cells from A1 to B2. You can also use the Cells property to select a range of cells:
Cells(1, 1).Resize(2, 2).Select
This code selects the same range of cells as the previous example.
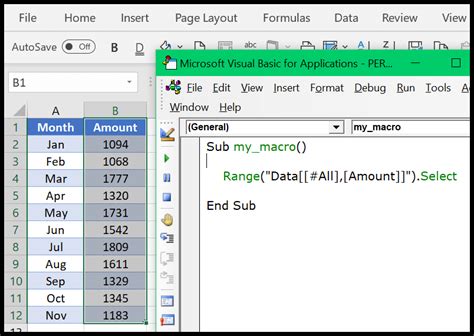
2. Using Cell Addresses to Write Data to a Range of Cells
Another way to use cell addresses in VBA is to write data to a range of cells. This can be done using the Range object or the Cells property. Here's an example of how to write data to a range of cells using the Range object:
Range("A1:B2").Value = "Hello World"
This code writes the text "Hello World" to the range of cells from A1 to B2. You can also use the Cells property to write data to a range of cells:
Cells(1, 1).Resize(2, 2).Value = "Hello World"
This code writes the same data to the same range of cells as the previous example.
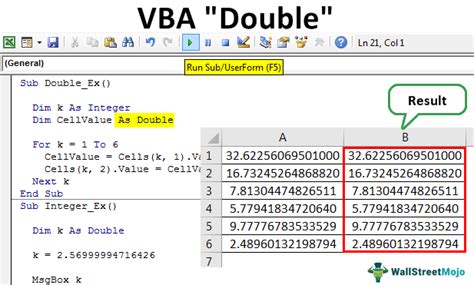
3. Using Cell Addresses to Loop Through a Range of Cells
Looping through a range of cells is a common task in VBA, and cell addresses are essential for this task. Here's an example of how to loop through a range of cells using the Range object:
For Each cell In Range("A1:B2")
cell.Value = "Hello World"
Next cell
This code loops through the range of cells from A1 to B2 and writes the text "Hello World" to each cell. You can also use the Cells property to loop through a range of cells:
For i = 1 To 2
For j = 1 To 2
Cells(i, j).Value = "Hello World"
Next j
Next i
This code loops through the same range of cells as the previous example and writes the same data to each cell.
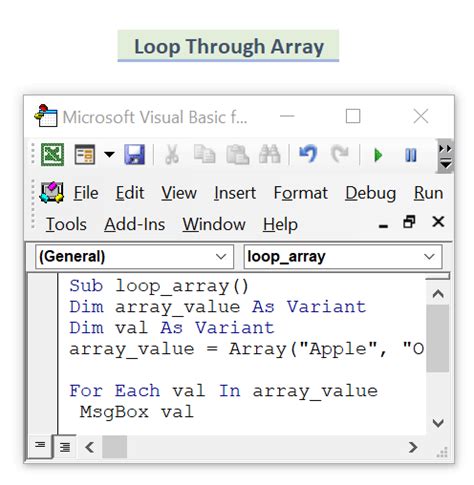
4. Using Cell Addresses to Refer to a Cell on Another Worksheet
Sometimes you need to refer to a cell on another worksheet in your VBA code. This can be done using the Range object or the Cells property. Here's an example of how to refer to a cell on another worksheet using the Range object:
Range("Sheet2!A1").Value = "Hello World"
This code writes the text "Hello World" to cell A1 on worksheet Sheet2. You can also use the Cells property to refer to a cell on another worksheet:
Worksheets("Sheet2").Cells(1, 1).Value = "Hello World"
This code writes the same data to the same cell as the previous example.
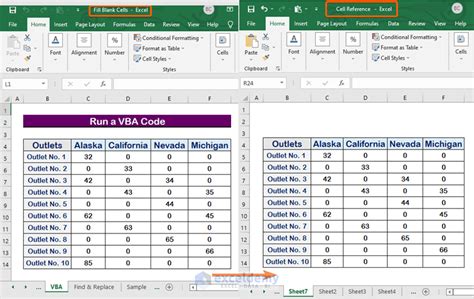
5. Using Cell Addresses to Create a Chart
Finally, cell addresses can be used to create a chart in VBA. Here's an example of how to create a chart using the Range object:
Range("A1:B2").CreateChart
This code creates a chart using the data in the range of cells from A1 to B2. You can also use the Cells property to create a chart:
Cells(1, 1).Resize(2, 2).CreateChart
This code creates the same chart as the previous example.
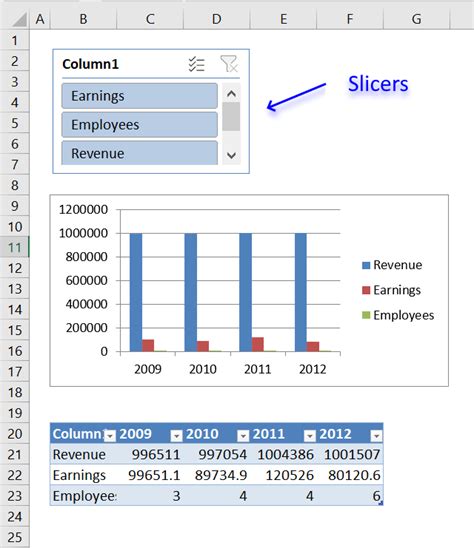
Gallery of VBA Cell Address Examples
VBA Cell Address Examples
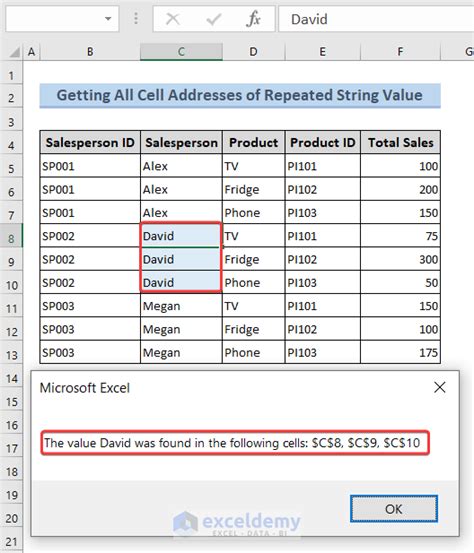
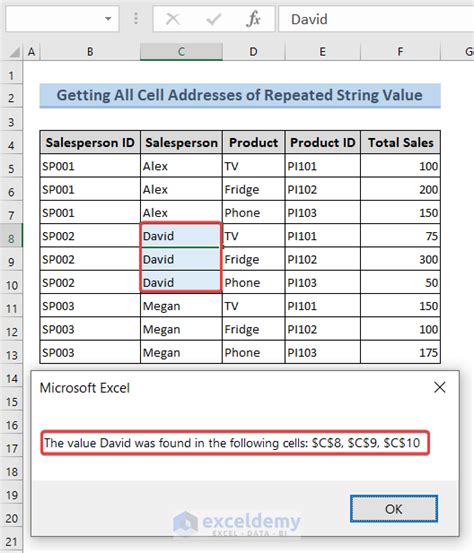
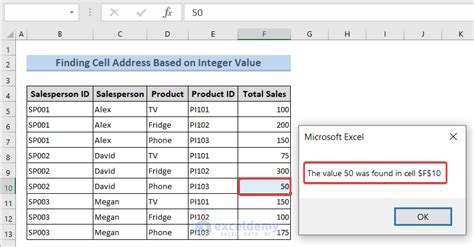
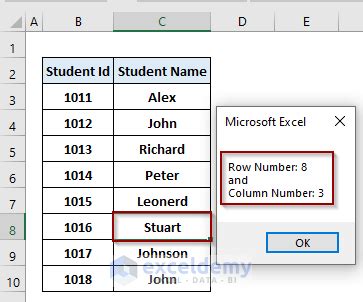
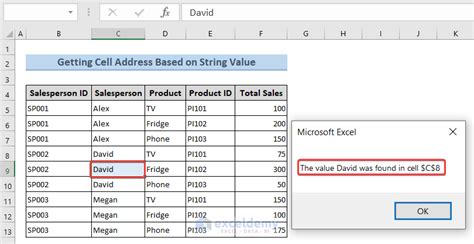
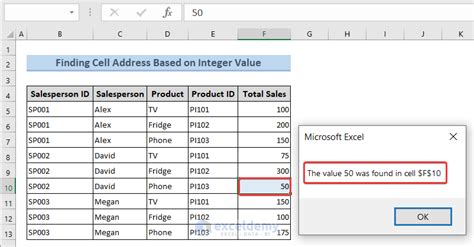
We hope this article has provided you with a comprehensive understanding of how to use cell addresses in VBA. Whether you're a beginner or an advanced user, cell addresses are an essential part of working with data in Excel and VBA. By mastering the five ways to use cell addresses in VBA, you'll be able to automate tasks, manipulate data, and create powerful Excel applications.