Intro
Effortlessly duplicate data with our expert guide on how to copy a row to another column in Google Sheets using a script. Master Google Apps Script and automate tasks with ease. Learn how to transfer rows, duplicate data, and manipulate columns like a pro. Boost productivity and simplify your workflow with this step-by-step tutorial.
Managing data in Google Sheets often requires manipulating and organizing information across different columns. One common task is copying data from one column to another. While Google Sheets provides a variety of functions and formulas to handle data, using Google Apps Script can offer more flexibility and automation for such tasks. Below is a guide on how to copy a row to another column in Google Sheets using Google Apps Script.
Why Use Google Apps Script?
Before diving into the script, it's worth understanding why you might want to use Google Apps Script for this task. While you can manually copy and paste or use formulas like =A1
to copy data, Google Apps Script allows you to automate the process, especially useful when dealing with large datasets or repetitive tasks. It can also be triggered by specific events, such as when a new row is added or edited.
Basic Script to Copy a Row to Another Column
To get started, you'll need to access the Google Apps Script editor in your Google Sheet. Here's how you can do it:
- Open your Google Sheet.
- Click on
Extensions
>Apps Script
. - This will open the Google Apps Script editor.
Here's a basic script to copy data from one column to another. This example assumes you want to copy data from Column A to Column B when a new row is added.
function onEdit(e) {
var sheet = e.source.getActiveSheet();
var range = e.range;
// Check if the edit occurred in Column A and if the edit was in the first row of the range
if (range.getColumn() === 1 && range.getRow() === e.value.getRow()) {
var targetCell = sheet.getRange(range.getRow(), 2); // Define the target cell in Column B
targetCell.setValue(e.value); // Copy the value to Column B
}
}
This script uses the onEdit(e)
trigger, which runs automatically when the sheet is edited. However, this trigger will run for every edit, which might not be efficient if you only want to copy data under specific conditions or from a specific column.
Copying Data on Demand
If you prefer to copy data on demand rather than automatically with each edit, you can create a custom function that you can trigger manually. Here's how you can do it:
function copyRowToAnotherColumn() {
var sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet();
var sourceRange = sheet.getRange("A1:A"); // Define the source range
var targetRange = sheet.getRange("B1:B"); // Define the target range
// Loop through each cell in the source range and copy the value to the target range
var sourceValues = sourceRange.getValues();
for (var i = 0; i < sourceValues.length; i++) {
targetRange.offset(i, 0).setValue(sourceValues[i][0]);
}
}
To run this function manually, you can add a button to your sheet and assign this script to it:
- Go to your Google Sheet.
- Click on
Insert
>Drawing
. - Draw a button.
- Right-click on the button >
Assign script
. - Enter
copyRowToAnotherColumn
and clickOK
.
Now, when you click the button, the script will copy the data from Column A to Column B.
Tips for Customization
- Specify Ranges: Make sure to adjust the ranges in the scripts according to your sheet's structure. For example, if you want to copy from Column E to Column F, you would use
getRange("E1:E")
andgetRange("F1:F")
. - Trigger Options: Google Apps Script offers various trigger options. For tasks that should run automatically, consider using triggers based on specific events or schedules.
- Error Handling: For more robust scripts, especially those dealing with large datasets or critical operations, consider implementing error handling mechanisms.
By following these steps and customizing the scripts to your needs, you can effectively manage and automate the task of copying rows to another column in Google Sheets using Google Apps Script.
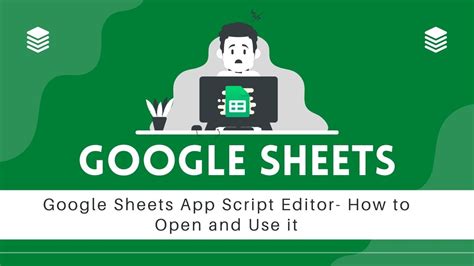
Common Issues and Solutions
Issue 1: Script Not Running Automatically
- Solution: Ensure that the trigger is set correctly. Go to Triggers in the Apps Script editor, and verify that the
onEdit
function has a trigger set.
Issue 2: Incorrect Data Being Copied
- Solution: Double-check the source and target ranges defined in the script. Ensure that the ranges match the columns you intend to copy from and to.
Issue 3: Script Running Too Slowly
- Solution: Optimize your script by minimizing unnecessary operations and using batch operations. For example, instead of writing values one by one, use
setValues
once with an array of values.
Gallery of Google Sheets Script Examples
Google Sheets Script Gallery
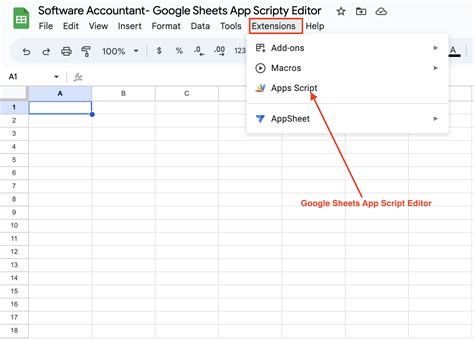
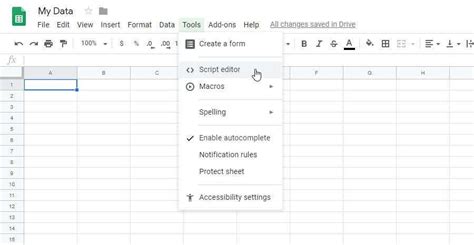
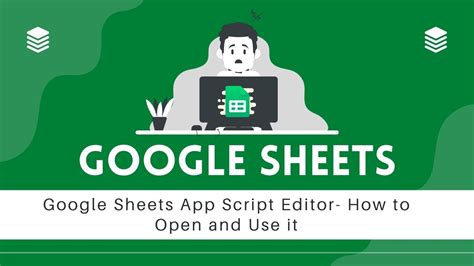
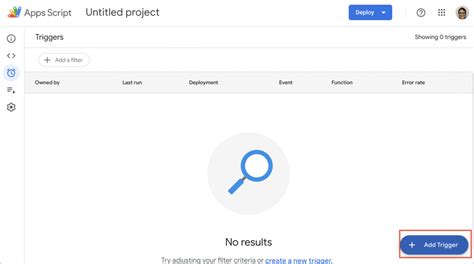
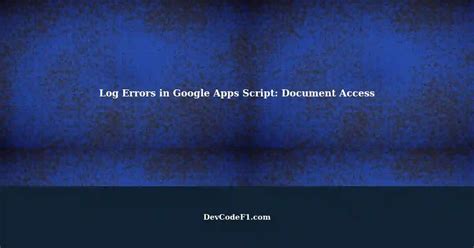
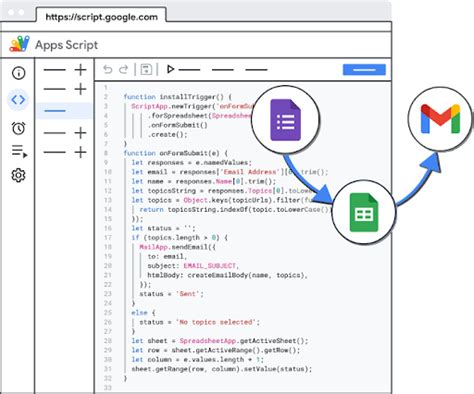
Do you have any specific questions about using Google Apps Script for copying rows to another column in Google Sheets? Feel free to ask, and we'll be happy to help you with more tailored advice or script adjustments!