Intro
Master VBA array creation with our step-by-step guide. Learn how to create arrays in VBA, including dynamic and multi-dimensional arrays, using VBA syntax and array functions. Improve your Excel automation skills with expert tips on declaring, initializing, and manipulating arrays in Visual Basic for Applications (VBA).
Working with arrays in VBA is an essential skill for any Excel developer. Arrays allow you to store and manipulate large datasets efficiently, making them a fundamental data structure in programming. In this article, we will delve into the world of arrays in VBA, exploring what they are, why you need them, and how to create and use them effectively.
What is an Array in VBA?
An array in VBA is a collection of values of the same data type stored in a single variable. You can think of it as a container that holds multiple elements, each identified by an index or key. Arrays can be one-dimensional (like a list) or multi-dimensional (like a table or matrix). They are useful when you need to work with a large amount of data that has a consistent structure.
Why Do You Need Arrays in VBA?
Arrays are a powerful tool in VBA programming, offering several benefits:
- Efficient data storage: Arrays allow you to store a large amount of data in a single variable, reducing memory usage and improving performance.
- Simplified data manipulation: Arrays make it easy to perform operations on multiple data elements at once, such as sorting, searching, and aggregating.
- Flexibility: Arrays can be used to represent various data structures, including lists, tables, and matrices.
Creating an Array in VBA
Now that we've covered the basics, let's dive into the step-by-step process of creating an array in VBA.
Step 1: Declare the Array
To create an array, you need to declare it first. You can do this using the Dim
statement, followed by the array name, data type, and dimensions.
Dim myArray() As Variant
In this example, myArray
is the name of the array, and Variant
is the data type. The empty parentheses ()
indicate that the array has no dimensions yet.
Step 2: Set the Array Dimensions
To set the dimensions of the array, you can use the ReDim
statement or the Dim
statement with the array size specified.
ReDim myArray(1 To 10) As Variant
This sets the array myArray
to have 10 elements, indexed from 1 to 10.
Step 3: Initialize the Array
Once the array is declared and dimensioned, you can initialize it with values. You can do this using a loop or by assigning values directly to the array elements.
For i = 1 To 10
myArray(i) = i * 2
Next i
This code initializes the array myArray
with values from 2 to 20, using a loop.
Example Use Case: Creating an Array from a Range
Suppose you have a range of cells in Excel that you want to convert to an array in VBA. You can do this using the Range
object and the Value
property.
Dim myArray() As Variant
myArray = Range("A1:A10").Value
This code creates an array myArray
from the values in the range A1:A10
.
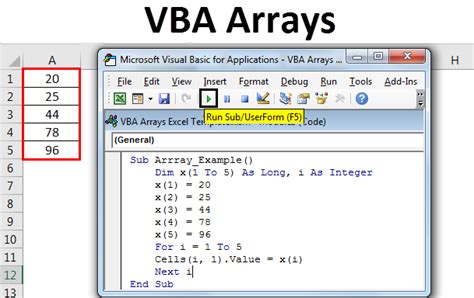
Working with Arrays in VBA
Now that you've created an array, you can perform various operations on it, such as:
- Accessing array elements: Use the array index to access individual elements, e.g.,
myArray(1)
. - Modifying array elements: Use the array index to modify individual elements, e.g.,
myArray(1) = 10
. - Looping through arrays: Use a loop to iterate through the array elements, e.g.,
For i = 1 To 10:... Next i
. - Sorting and searching arrays: Use built-in VBA functions, such as
Sort
andFind
, to manipulate the array.
Best Practices for Working with Arrays in VBA
When working with arrays in VBA, keep the following best practices in mind:
- Use meaningful variable names: Choose descriptive names for your arrays to make your code easier to understand.
- Dimension arrays carefully: Make sure to dimension your arrays correctly to avoid errors and improve performance.
- Use loops efficiently: Use loops to iterate through arrays, but avoid using unnecessary loops or nesting loops excessively.
- Test and debug your code: Verify that your array-based code works correctly and debug any issues promptly.
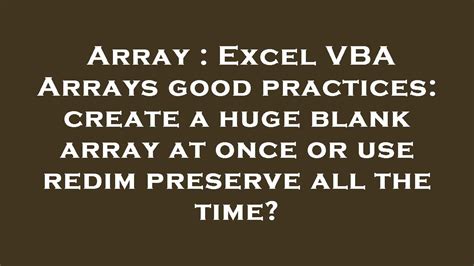
Gallery of VBA Array Examples
Here are some additional examples of working with arrays in VBA:
VBA Array Examples
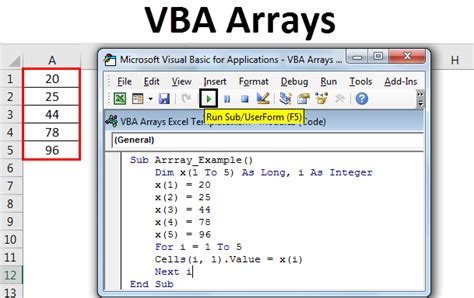
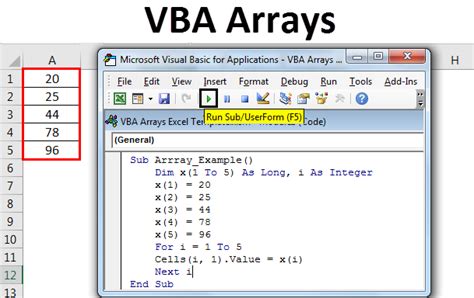
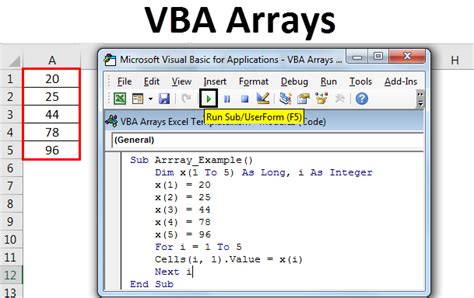
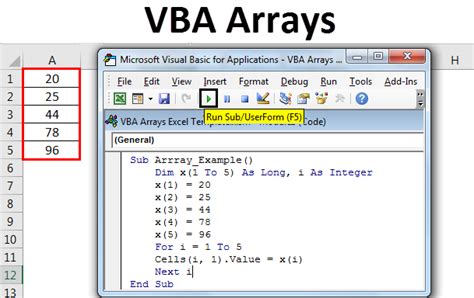
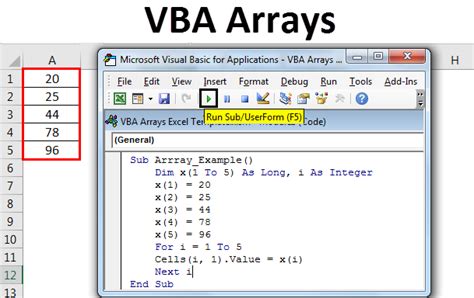
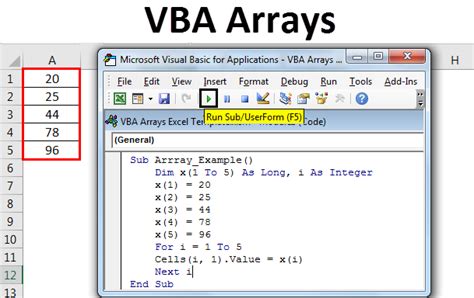
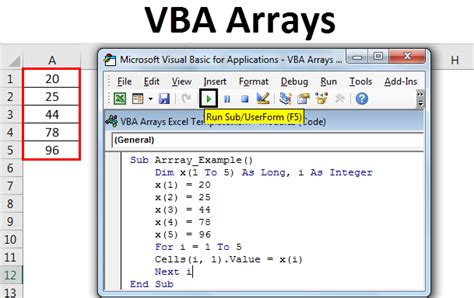
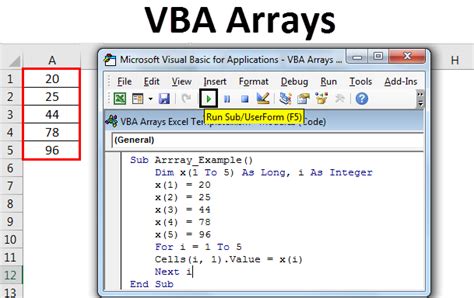
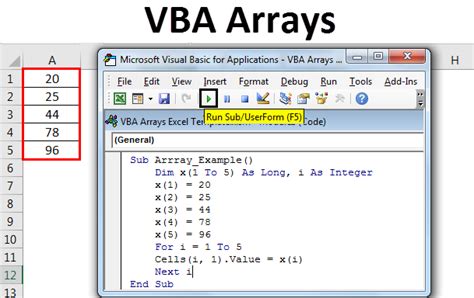
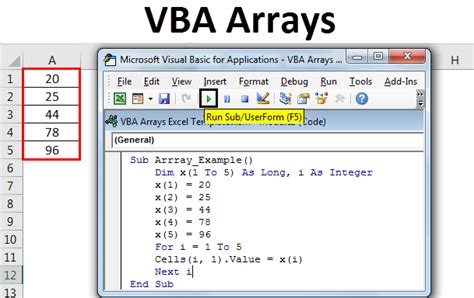
Conclusion
In this article, we explored the world of arrays in VBA, covering the basics, best practices, and example use cases. By following the steps outlined in this guide, you should now be able to create and work with arrays in VBA like a pro. Remember to dimension your arrays carefully, use loops efficiently, and test your code thoroughly to ensure it works as expected. Happy coding!
We hope you found this article helpful. If you have any questions or need further assistance, please don't hesitate to ask. Share your thoughts and experiences with arrays in VBA in the comments below.