Intro
Learn how to efficiently remove blank rows in Excel using VBA with our expert guide. Discover 5 effective methods to delete empty rows, including using loops, filters, and range manipulation. Master Excel VBA programming and improve your data cleaning skills. Say goodbye to blank rows and hello to organized spreadsheets with these simple and powerful VBA techniques.
Excel is a powerful tool for data analysis, but it can be frustrating to work with datasets that contain blank rows. Not only do these blank rows make your data look messy, but they can also cause problems when you're trying to analyze or manipulate your data. Fortunately, there are several ways to delete blank rows in Excel using VBA. In this article, we'll explore five different methods to help you get rid of those pesky blank rows.
The Importance of Deleting Blank Rows
Before we dive into the methods, let's talk about why deleting blank rows is important. Blank rows can cause a range of problems, including:
- Incorrect calculations: If you're using formulas that reference entire columns or rows, blank rows can cause errors or incorrect results.
- Difficulty selecting data: Blank rows can make it harder to select the data you want to work with, especially if you're using keyboard shortcuts like Ctrl+A to select all data.
- Increased file size: Blank rows can increase the size of your Excel file, making it slower to open and close.
By deleting blank rows, you can make your data more manageable, reduce errors, and improve the overall performance of your Excel file.
Method 1: Using the "Go To Special" Feature
One of the quickest ways to delete blank rows is to use the "Go To Special" feature in Excel. Here's how:
Sub DeleteBlankRows_GoToSpecial()
Range("A1").CurrentRegion.SpecialCells(xlCellTypeBlanks).EntireRow.Delete
End Sub
This code selects all blank cells in the current region (i.e., the range of cells that contains data) and deletes the entire row. The CurrentRegion
property is used to select all cells that contain data, and the SpecialCells
method is used to select only blank cells.
Method 2: Using a Loop
Another way to delete blank rows is to use a loop that checks each row for blank cells. Here's an example:
Sub DeleteBlankRows_Loop()
Dim ws As Worksheet
Set ws = ActiveSheet
Dim lastRow As Long
lastRow = ws.Cells(ws.Rows.Count, 1).End(xlUp).Row
Dim i As Long
For i = lastRow To 1 Step -1
If ws.Cells(i, 1).Value = "" Then
ws.Rows(i).Delete
End If
Next i
End Sub
This code uses a loop to check each row in the active sheet for blank cells in column A. If a row is blank, it is deleted. The loop starts from the last row and works its way up to avoid skipping rows.
Method 3: Using the "Find" Method
You can also use the "Find" method to delete blank rows. Here's an example:
Sub DeleteBlankRows_Find()
Dim ws As Worksheet
Set ws = ActiveSheet
Dim lastRow As Long
lastRow = ws.Cells(ws.Rows.Count, 1).End(xlUp).Row
Dim rng As Range
Set rng = ws.Range("A1:A" & lastRow)
rng.Find("").EntireRow.Delete
End Sub
This code uses the "Find" method to select all blank cells in column A and deletes the entire row.
Method 4: Using the "Filter" Method
Another way to delete blank rows is to use the "Filter" method. Here's an example:
Sub DeleteBlankRows_Filter()
Dim ws As Worksheet
Set ws = ActiveSheet
Dim lastRow As Long
lastRow = ws.Cells(ws.Rows.Count, 1).End(xlUp).Row
ws.Range("A1:A" & lastRow).AutoFilter Field:=1, Criteria1:="="
ws.Range("A1:A" & lastRow).SpecialCells(xlCellTypeVisible).EntireRow.Delete
ws.AutoFilterMode = False
End Sub
This code applies a filter to column A to select only blank cells and then deletes the entire row.
Method 5: Using the "WorksheetFunction.CountA" Method
Finally, you can use the WorksheetFunction.CountA
method to delete blank rows. Here's an example:
Sub DeleteBlankRows_CountA()
Dim ws As Worksheet
Set ws = ActiveSheet
Dim lastRow As Long
lastRow = ws.Cells(ws.Rows.Count, 1).End(xlUp).Row
Dim rng As Range
Set rng = ws.Range("A1:A" & lastRow)
For Each cell In rng
If Application.WorksheetFunction.CountA(cell) = 0 Then
cell.EntireRow.Delete
End If
Next cell
End Sub
This code uses the WorksheetFunction.CountA
method to count the number of non-blank cells in each row. If a row is blank, it is deleted.
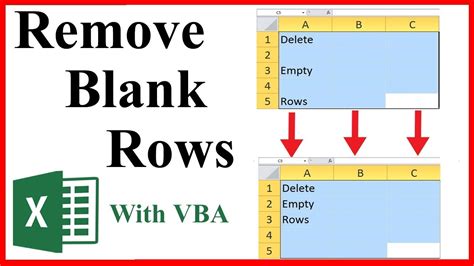
Conclusion
In this article, we've explored five different methods to delete blank rows in Excel using VBA. Each method has its own advantages and disadvantages, and the best method for you will depend on your specific needs and preferences. Whether you're a beginner or an experienced VBA user, these methods will help you get rid of those pesky blank rows and make your data more manageable.
Gallery of Delete Blank Rows in Excel VBA
Delete Blank Rows in Excel VBA Image Gallery
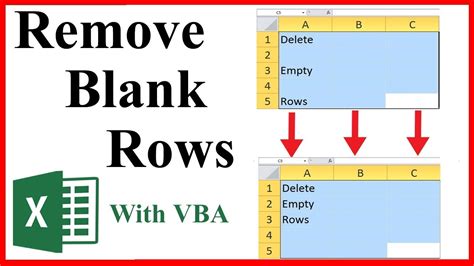
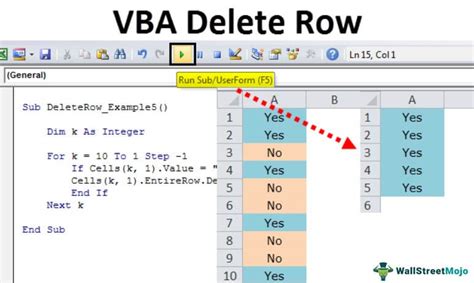
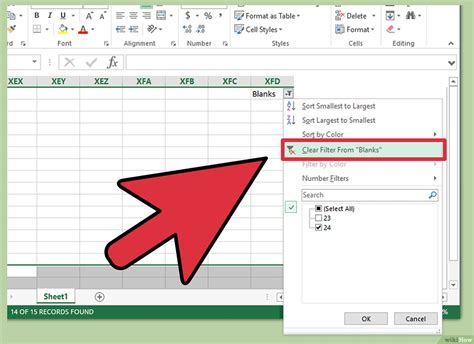
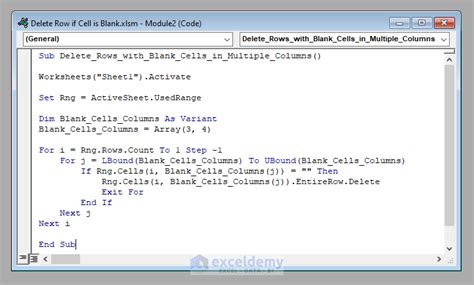
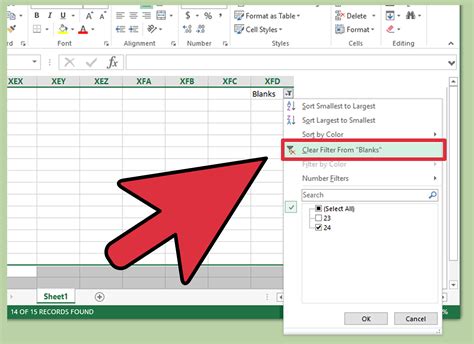
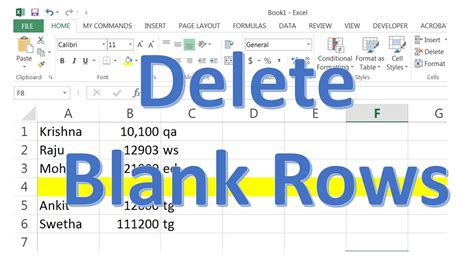
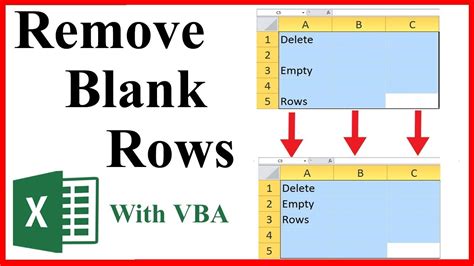
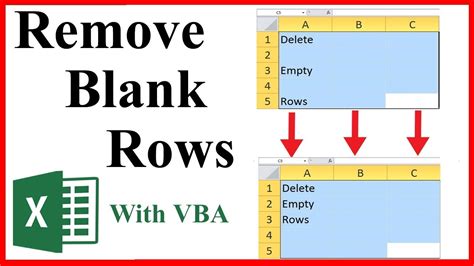
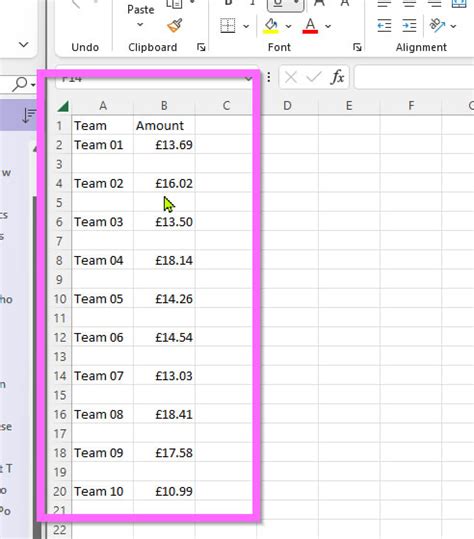
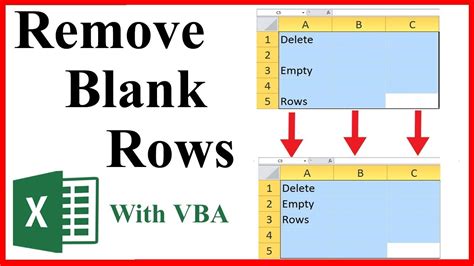
Share Your Thoughts
We hope this article has helped you learn how to delete blank rows in Excel using VBA. Do you have any favorite methods or tips for deleting blank rows? Share your thoughts with us in the comments below!