Intro
Unlock the power of Django URL templates with our expert guide. Discover 5 ways to use Django URL in templates, including reversing URLs, using URL names, and more. Master URL routing, template tags, and resolver functions to boost your Django development skills. Learn how to simplify complex URLs and improve your web apps performance.
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. It provides an excellent way to handle URLs in templates, making it easier to manage and maintain your website's navigation. In this article, we will explore five ways to use Django URLs in templates, making it easier for you to create a seamless user experience.
Understanding Django URL Resolvers
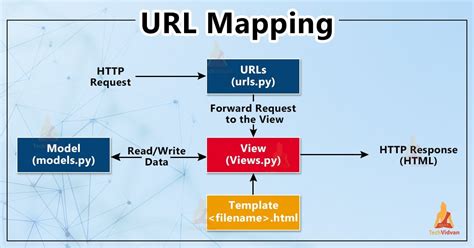
Before we dive into the ways to use Django URLs in templates, it's essential to understand how Django URL resolvers work. Django's URL resolver is a component that maps URLs to views. It takes a URL pattern and returns a view function that handles the request. The resolver also passes any additional arguments captured from the URL to the view function.
1. Using the url Template Tag
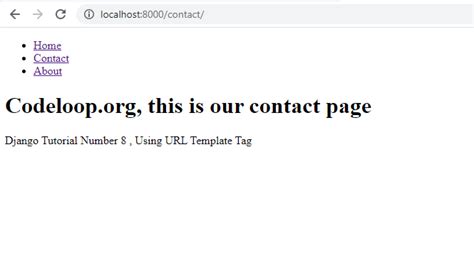
One of the most common ways to use Django URLs in templates is by using the {% url %}
template tag. This tag takes the name of the URL pattern and any additional arguments as parameters. It returns the absolute URL of the view function associated with the given URL pattern.
For example, if you have a URL pattern named my_view
defined in your urls.py
file:
from django.urls import path
from. import views
urlpatterns = [
path('my-view/', views.my_view, name='my_view'),
]
You can use the {% url %}
template tag in your template file like this:
Go to my view
This will generate an HTML anchor tag with the absolute URL of the my_view
view function.
Reverse URL Resolution
Django also provides a way to reverse URL resolution using the {% url %}
template tag. This means you can generate URLs from view functions without having to hardcode the URL pattern. To use reverse URL resolution, you need to define a URL pattern with a name.
For example, if you have a view function defined in your views.py
file:
from django.shortcuts import render
def my_view(request):
return render(request, 'my_template.html')
You can define a URL pattern with a name in your urls.py
file:
from django.urls import path
from. import views
urlpatterns = [
path('my-view/', views.my_view, name='my_view'),
]
Then, you can use the {% url %}
template tag in your template file to generate the URL:
Go to my view
This will generate an HTML anchor tag with the absolute URL of the my_view
view function.
2. Using the url Template Filter

Another way to use Django URLs in templates is by using the url
template filter. This filter takes a URL pattern name and any additional arguments as parameters. It returns the absolute URL of the view function associated with the given URL pattern.
For example, if you have a URL pattern named my_view
defined in your urls.py
file:
from django.urls import path
from. import views
urlpatterns = [
path('my-view/', views.my_view, name='my_view'),
]
You can use the url
template filter in your template file like this:
Go to my view
This will generate an HTML anchor tag with the absolute URL of the my_view
view function.
Using the url Template Filter with Arguments
The url
template filter also supports passing additional arguments to the view function. You can pass arguments as a dictionary or as positional arguments.
For example, if you have a view function defined in your views.py
file:
from django.shortcuts import render
def my_view(request, pk):
return render(request, 'my_template.html')
You can define a URL pattern with a name in your urls.py
file:
from django.urls import path
from. import views
urlpatterns = [
path('my-view//', views.my_view, name='my_view'),
]
Then, you can use the url
template filter in your template file to generate the URL with arguments:
Go to my view with pk 1
This will generate an HTML anchor tag with the absolute URL of the my_view
view function with the pk
argument set to 1.
3. Using the reverse Function

Django also provides a way to reverse URL resolution using the reverse
function. This function takes a URL pattern name and any additional arguments as parameters. It returns the absolute URL of the view function associated with the given URL pattern.
For example, if you have a view function defined in your views.py
file:
from django.shortcuts import render
def my_view(request):
return render(request, 'my_template.html')
You can define a URL pattern with a name in your urls.py
file:
from django.urls import path
from. import views
urlpatterns = [
path('my-view/', views.my_view, name='my_view'),
]
Then, you can use the reverse
function in your Python code to generate the URL:
from django.urls import reverse
url = reverse('my_view')
This will return the absolute URL of the my_view
view function.
Using the reverse Function with Arguments
The reverse
function also supports passing additional arguments to the view function. You can pass arguments as a dictionary or as positional arguments.
For example, if you have a view function defined in your views.py
file:
from django.shortcuts import render
def my_view(request, pk):
return render(request, 'my_template.html')
You can define a URL pattern with a name in your urls.py
file:
from django.urls import path
from. import views
urlpatterns = [
path('my-view//', views.my_view, name='my_view'),
]
Then, you can use the reverse
function in your Python code to generate the URL with arguments:
from django.urls import reverse
url = reverse('my_view', kwargs={'pk': 1})
This will return the absolute URL of the my_view
view function with the pk
argument set to 1.
4. Using the {% url %} Template Tag with Namespaces
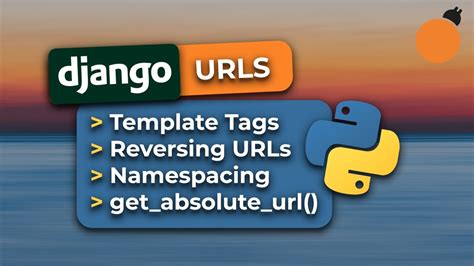
Django also provides a way to use the {% url %}
template tag with namespaces. Namespaces allow you to organize your URL patterns into logical groups.
For example, if you have a URL pattern named my_view
defined in your urls.py
file:
from django.urls import path, include
from. import views
urlpatterns = [
path('my-app/', include('my_app.urls', namespace='my_app')),
]
And in your my_app/urls.py
file:
from django.urls import path
from. import views
urlpatterns = [
path('my-view/', views.my_view, name='my_view'),
]
You can use the {% url %}
template tag in your template file to generate the URL with a namespace:
Go to my view
This will generate an HTML anchor tag with the absolute URL of the my_view
view function in the my_app
namespace.
5. Using the {% url %} Template Tag with URL Pattern Names
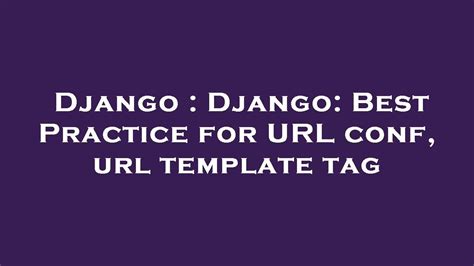
Finally, Django also provides a way to use the {% url %}
template tag with URL pattern names. This allows you to generate URLs from view functions without having to hardcode the URL pattern.
For example, if you have a view function defined in your views.py
file:
from django.shortcuts import render
def my_view(request):
return render(request, 'my_template.html')
You can define a URL pattern with a name in your urls.py
file:
from django.urls import path
from. import views
urlpatterns = [
path('my-view/', views.my_view, name='my_view'),
]
Then, you can use the {% url %}
template tag in your template file to generate the URL:
Go to my view
This will generate an HTML anchor tag with the absolute URL of the my_view
view function.
Django URL Template Tag Examples
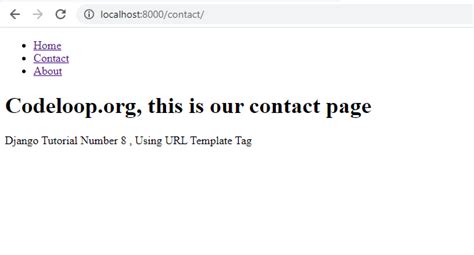
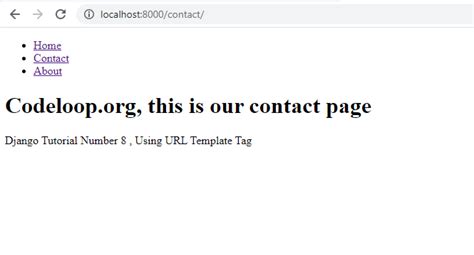
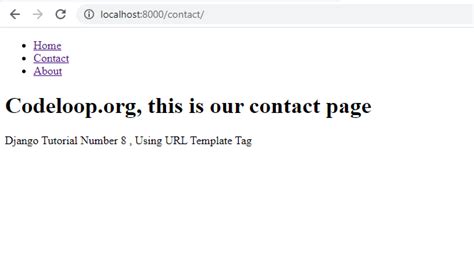

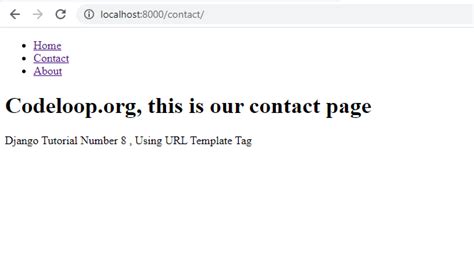
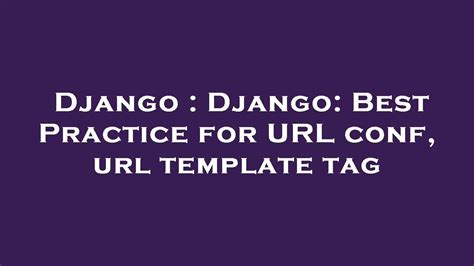
We hope this article has helped you understand the different ways to use Django URLs in templates. Whether you're using the {% url %}
template tag, the url
template filter, or the reverse
function, Django provides a flexible and powerful way to manage your website's navigation. Do you have any questions or need further clarification on any of the topics covered in this article? Please feel free to ask in the comments section below.