Intro
Discover 5 effective methods to determine if a date falls within multiple date ranges. Learn how to use logical operators, conditional statements, and date functions to check if a date is between multiple ranges in various programming languages and applications, improving your data analysis and management skills.
Checking if a date falls between multiple ranges can be a useful skill in various applications, such as scheduling, data analysis, and software development. In this article, we will explore five different ways to achieve this, along with examples and code snippets.
Understanding Date Ranges
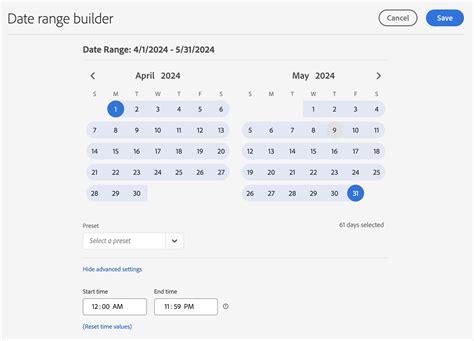
Before diving into the methods, let's define what we mean by "date ranges." A date range is a period of time between two specific dates, such as "2022-01-01" to "2022-01-31." When checking if a date falls between multiple ranges, we need to ensure that the date is within at least one of the ranges.
Method 1: Using Nested IF Statements
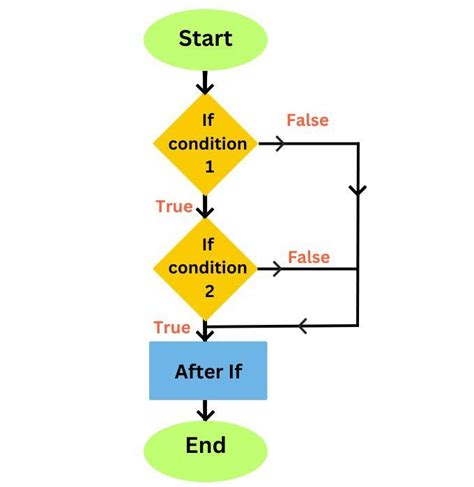
One way to check if a date is between multiple ranges is to use nested IF statements. This method involves creating multiple conditions to check if the date falls within each range.
Here's an example code snippet in Python:
def is_date_between_ranges(date, ranges):
for range in ranges:
start_date = range[0]
end_date = range[1]
if start_date <= date <= end_date:
return True
return False
ranges = [
("2022-01-01", "2022-01-31"),
("2022-02-01", "2022-02-28"),
("2022-03-01", "2022-03-31")
]
date = "2022-02-15"
print(is_date_between_ranges(date, ranges)) # Output: True
Method 2: Using List Comprehensions
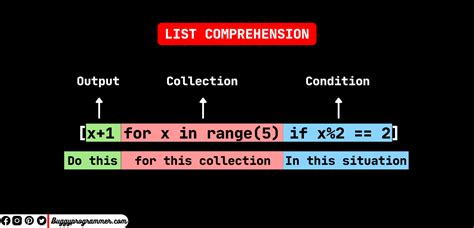
Another way to check if a date is between multiple ranges is to use list comprehensions. This method involves creating a list of boolean values indicating whether the date falls within each range.
Here's an example code snippet in Python:
def is_date_between_ranges(date, ranges):
return any(start_date <= date <= end_date for start_date, end_date in ranges)
ranges = [
("2022-01-01", "2022-01-31"),
("2022-02-01", "2022-02-28"),
("2022-03-01", "2022-03-31")
]
date = "2022-02-15"
print(is_date_between_ranges(date, ranges)) # Output: True
Method 3: Using Pandas DateRange
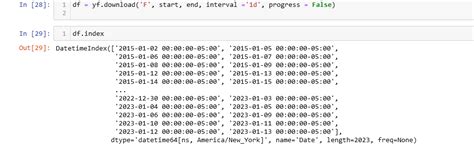
If you're working with Pandas, you can use the DateRange
function to create a range of dates and check if a specific date falls within that range.
Here's an example code snippet in Python:
import pandas as pd
def is_date_between_ranges(date, ranges):
for range in ranges:
start_date = pd.to_datetime(range[0])
end_date = pd.to_datetime(range[1])
if start_date <= pd.to_datetime(date) <= end_date:
return True
return False
ranges = [
("2022-01-01", "2022-01-31"),
("2022-02-01", "2022-02-28"),
("2022-03-01", "2022-03-31")
]
date = "2022-02-15"
print(is_date_between_ranges(date, ranges)) # Output: True
Method 4: Using NumPy
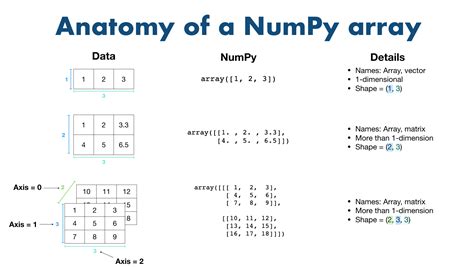
NumPy provides a powerful way to perform date calculations using the datetime64
data type.
Here's an example code snippet in Python:
import numpy as np
def is_date_between_ranges(date, ranges):
date = np.datetime64(date)
for range in ranges:
start_date = np.datetime64(range[0])
end_date = np.datetime64(range[1])
if start_date <= date <= end_date:
return True
return False
ranges = [
("2022-01-01", "2022-01-31"),
("2022-02-01", "2022-02-28"),
("2022-03-01", "2022-03-31")
]
date = "2022-02-15"
print(is_date_between_ranges(date, ranges)) # Output: True
Method 5: Using SQL
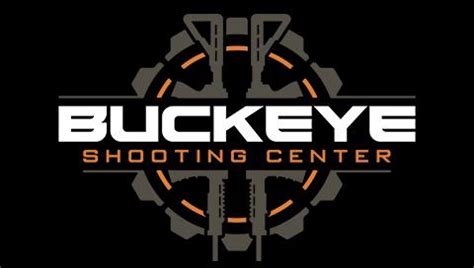
If you're working with a database, you can use SQL to check if a date falls between multiple ranges.
Here's an example SQL query:
SELECT *
FROM dates
WHERE date BETWEEN '2022-01-01' AND '2022-01-31'
OR date BETWEEN '2022-02-01' AND '2022-02-28'
OR date BETWEEN '2022-03-01' AND '2022-03-31'
Date Range Gallery
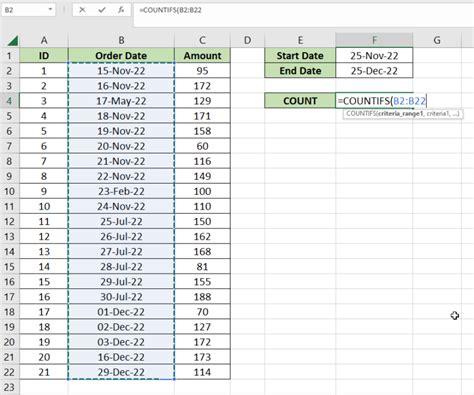
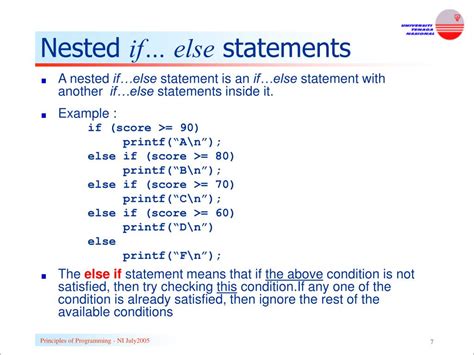
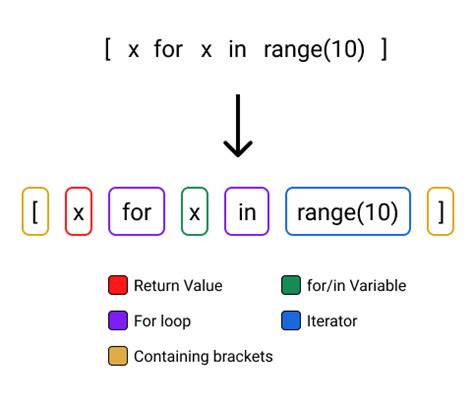
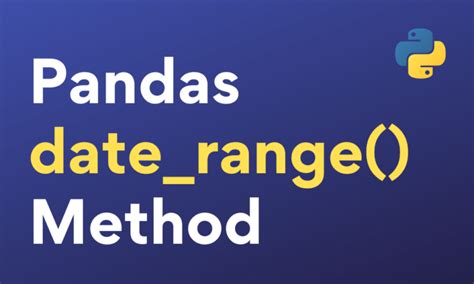
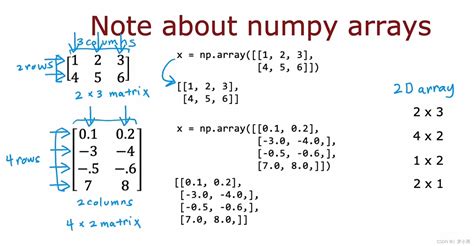
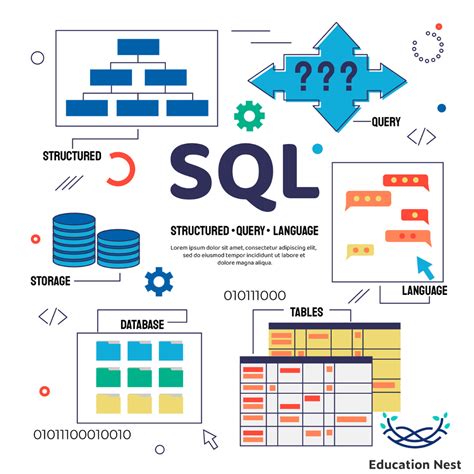
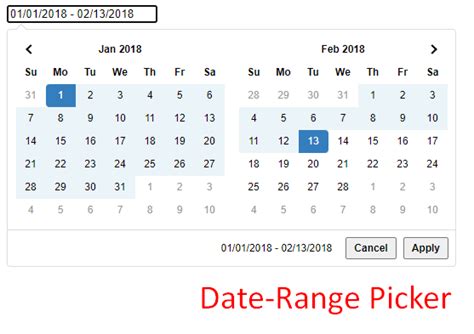
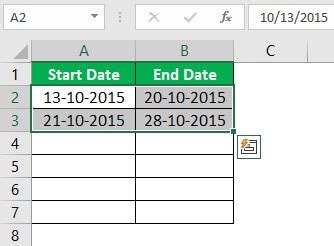
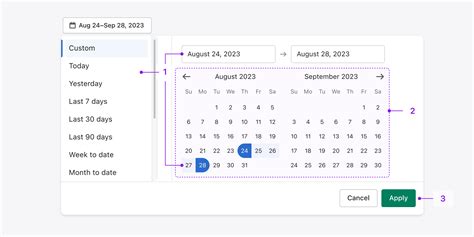
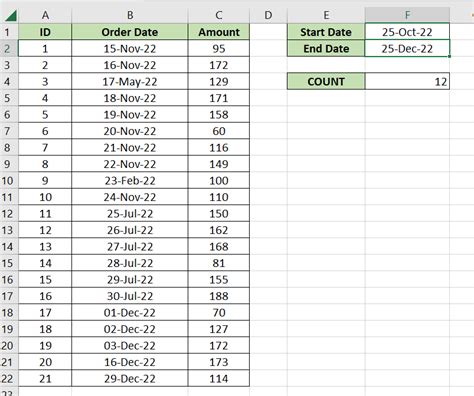
In conclusion, checking if a date falls between multiple ranges can be achieved using various methods, including nested IF statements, list comprehensions, Pandas DateRange, NumPy, and SQL. Each method has its own strengths and weaknesses, and the choice of method depends on the specific use case and requirements.
We hope this article has been helpful in providing you with the knowledge and tools to tackle date range calculations with confidence. If you have any further questions or need more assistance, please don't hesitate to comment below.