Intro
Unlock the power of Excel VBA workbooks with our expert guide. Learn 5 ways to activate workbooks in Excel VBA, including setting active workbooks, using workbook objects, and more. Master VBA workbook activation and boost productivity with our step-by-step tutorials and code examples. Discover the best practices for Excel VBA workbook automation.
Activating a workbook in Excel VBA is a fundamental task that allows you to manipulate and interact with the workbook's contents programmatically. In this article, we will explore five different ways to activate a workbook in Excel VBA, along with examples and practical applications.
The Importance of Activating a Workbook
Before diving into the methods, it's essential to understand why activating a workbook is crucial in Excel VBA. When you activate a workbook, you're essentially telling VBA which workbook to focus on, allowing you to perform actions on that specific workbook. Without activating a workbook, your code may not work as intended, or it may interact with the wrong workbook.
Method 1: Using the Workbooks
Collection
One of the most common ways to activate a workbook is by using the Workbooks
collection. This collection contains all open workbooks in Excel.
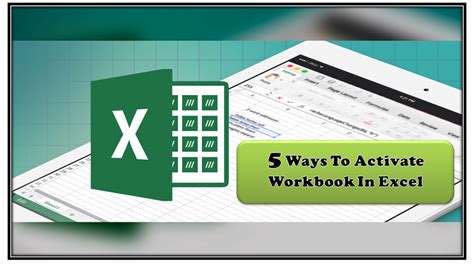
Sub ActivateWorkbook()
Dim wb As Workbook
Set wb = Workbooks("MyWorkbook.xlsx")
wb.Activate
End Sub
In this example, we're using the Workbooks
collection to access the workbook named "MyWorkbook.xlsx" and then activating it using the Activate
method.
Method 2: Using the ActiveWorkbook
Property
Another way to activate a workbook is by using the ActiveWorkbook
property. This property returns the currently active workbook.
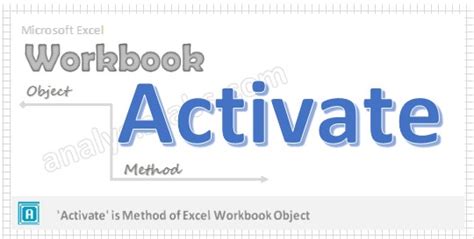
Sub ActivateWorkbook()
ActiveWorkbook.Activate
End Sub
In this example, we're using the ActiveWorkbook
property to activate the currently active workbook.
Method 3: Using the Workbooks.Open
Method
You can also activate a workbook by opening it using the Workbooks.Open
method.
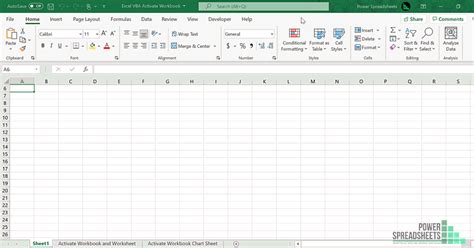
Sub ActivateWorkbook()
Dim filePath As String
filePath = "C:\Path\To\MyWorkbook.xlsx"
Workbooks.Open (filePath).Activate
End Sub
In this example, we're using the Workbooks.Open
method to open the workbook at the specified file path and then activating it.
Method 4: Using the Application.ActiveWorkbook
Property
This method is similar to Method 2, but uses the Application
object to access the active workbook.
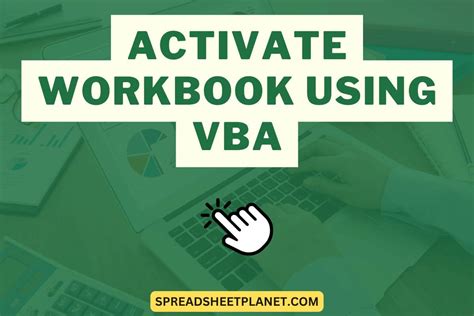
Sub ActivateWorkbook()
Application.ActiveWorkbook.Activate
End Sub
Method 5: Using the Workbooks.Add
Method
Finally, you can activate a new workbook by adding it using the Workbooks.Add
method.
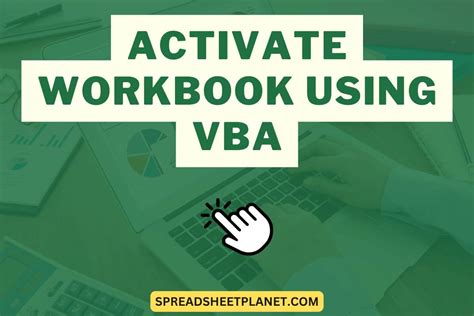
Sub ActivateWorkbook()
Workbooks.Add.Activate
End Sub
In this example, we're using the Workbooks.Add
method to add a new workbook and then activating it.
Gallery of Activate Workbook in Excel VBA
Activate Workbook in Excel VBA Image Gallery
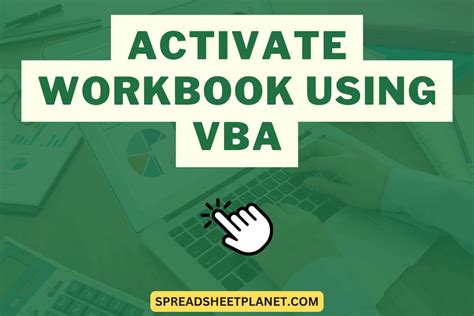
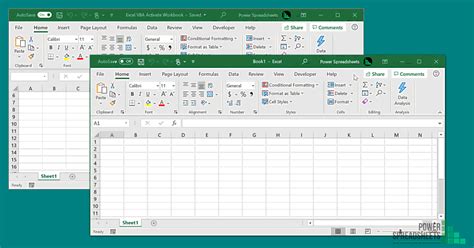
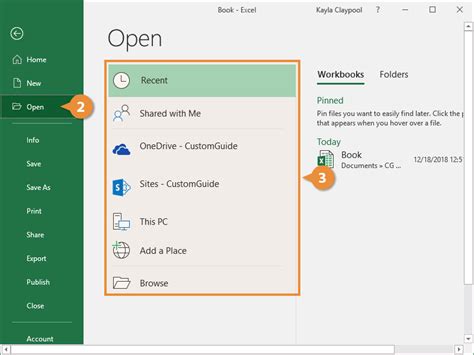
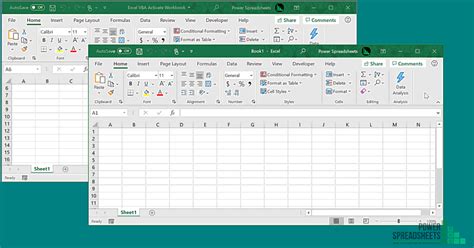
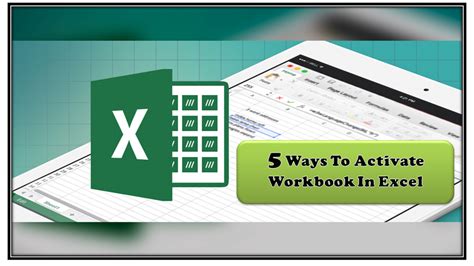
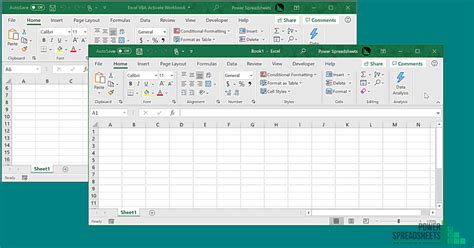
We hope this article has provided you with a comprehensive understanding of the different ways to activate a workbook in Excel VBA. Whether you're a beginner or an experienced developer, mastering these methods will help you to create more efficient and effective VBA code.
What's your favorite method for activating a workbook in Excel VBA? Share your thoughts and experiences in the comments below!