Intro
Discover 5 efficient ways to check empty cells in Excel VBA, streamlining your data analysis and automation. Learn how to identify and handle blank cells using VBAs built-in functions, loops, and conditional statements. Master VBAs IsEmpty, IsNull, and Range functions, and boost your Excel productivity with expert-approved methods.
In Microsoft Excel, empty cells can be a bit tricky to handle, especially when working with large datasets. Luckily, Excel VBA provides several ways to check for empty cells, each with its own strengths and use cases. In this article, we'll explore five methods to detect empty cells in Excel VBA, along with practical examples and explanations.
The Importance of Checking Empty Cells
Before we dive into the methods, let's understand why checking empty cells is crucial in Excel VBA. Empty cells can cause errors in calculations, formatting, and even data analysis. By identifying and handling empty cells, you can:
- Avoid errors in formulas and calculations
- Improve data quality and integrity
- Enhance data analysis and visualization
- Streamline data processing and automation
Method 1: Using the IsEmpty
Function
The IsEmpty
function is a straightforward way to check if a cell is empty. This function returns True
if the cell is empty and False
otherwise.
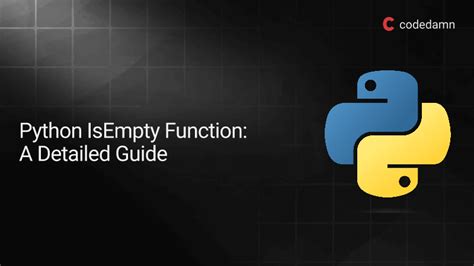
Example code:
Sub CheckEmptyCell()
If IsEmpty(Range("A1")) Then
MsgBox "Cell A1 is empty"
Else
MsgBox "Cell A1 is not empty"
End If
End Sub
Method 2: Using the Len
Function
The Len
function returns the length of a string. If a cell is empty, the Len
function will return 0.
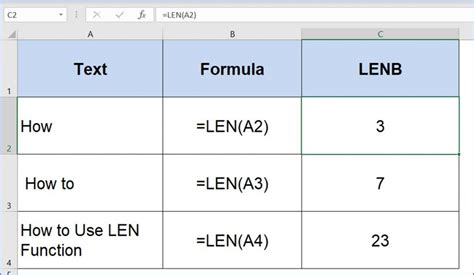
Example code:
Sub CheckEmptyCell()
If Len(Range("A1").Value) = 0 Then
MsgBox "Cell A1 is empty"
Else
MsgBox "Cell A1 is not empty"
End If
End Sub
Method 3: Using the Cells
Property
The Cells
property allows you to access a cell's value directly. If a cell is empty, the Cells
property will return an empty string.
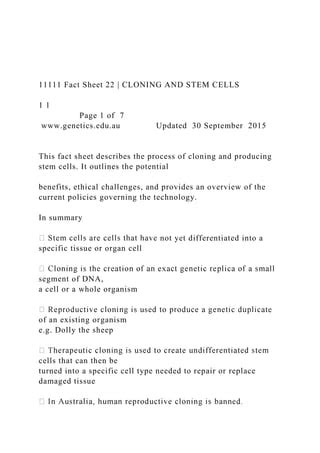
Example code:
Sub CheckEmptyCell()
If Range("A1").Cells.Value = "" Then
MsgBox "Cell A1 is empty"
Else
MsgBox "Cell A1 is not empty"
End If
End Sub
Method 4: Using the Range
Object
The Range
object provides a Value
property that can be used to check if a cell is empty. If a cell is empty, the Value
property will return an empty variant.
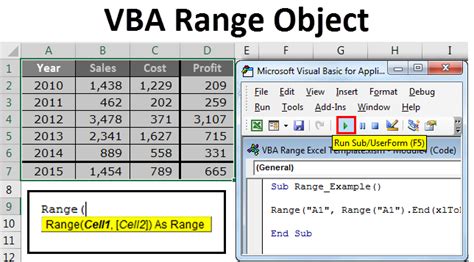
Example code:
Sub CheckEmptyCell()
If Range("A1").Value = vbNullString Then
MsgBox "Cell A1 is empty"
Else
MsgBox "Cell A1 is not empty"
End If
End Sub
Method 5: Using the SpecialCells
Method
The SpecialCells
method allows you to select cells based on specific criteria, including empty cells.
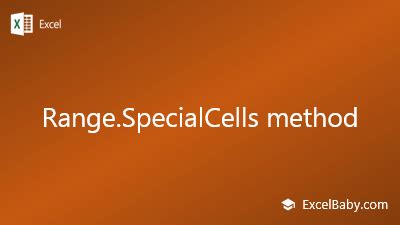
Example code:
Sub CheckEmptyCell()
If Range("A1").SpecialCells(xlCellTypeBlanks).Count > 0 Then
MsgBox "Cell A1 is empty"
Else
MsgBox "Cell A1 is not empty"
End If
End Sub
Gallery of Checking Empty Cells in Excel VBA
Checking Empty Cells in Excel VBA Image Gallery
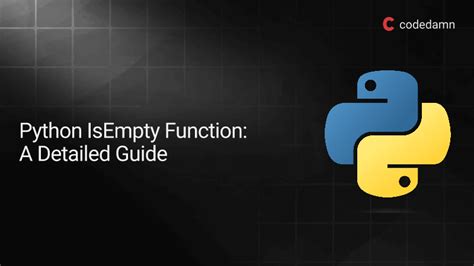
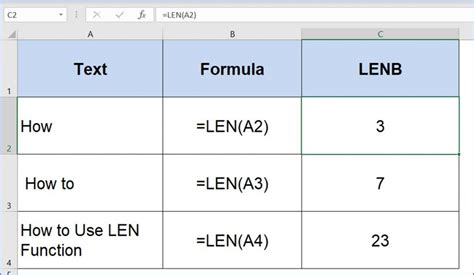
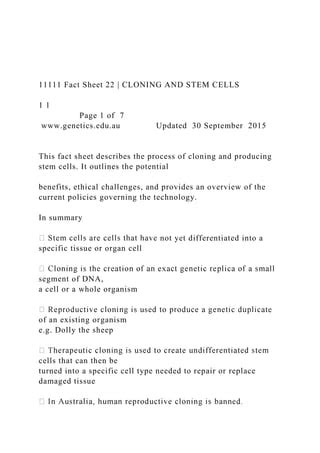
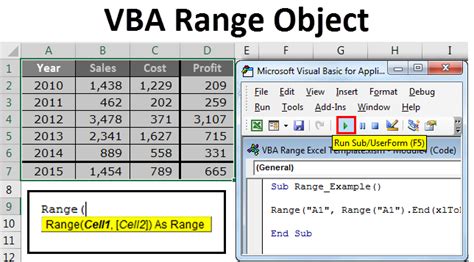
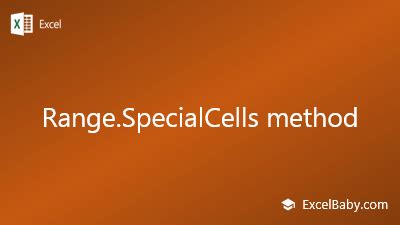
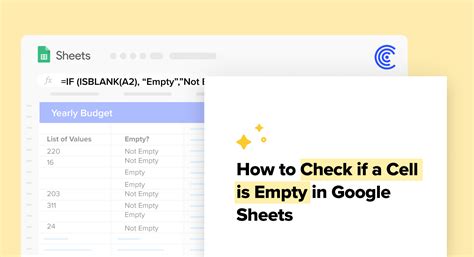
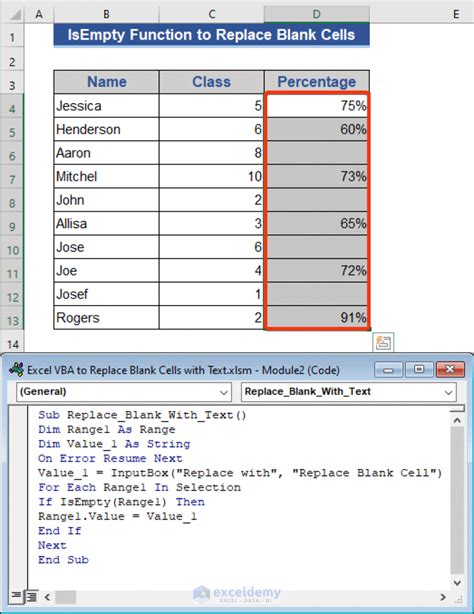
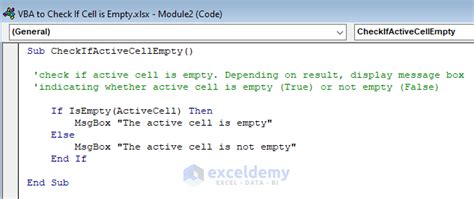
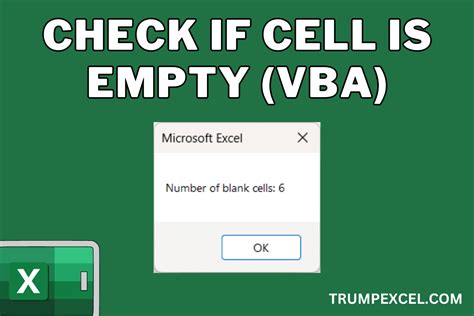
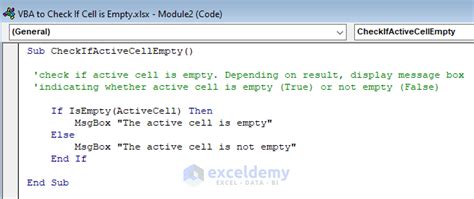
Conclusion
Checking empty cells is an essential task in Excel VBA, and there are several methods to achieve this. By using the IsEmpty
function, Len
function, Cells
property, Range
object, or SpecialCells
method, you can detect empty cells and handle them accordingly. Remember to choose the method that best suits your specific needs and use case. Happy coding!