Intro
Master Excel VBA delete columns with ease. Learn how to delete multiple columns, specific columns, and entire column ranges using VBA macros. Discover how to use VBA code to automate column deletion, including deleting columns based on criteria, and handle errors with try-except blocks for efficient spreadsheet management.
The world of Excel VBA! Deleting columns in Excel can be a tedious task, especially when dealing with large datasets. But fear not, dear readers, for we have the power of VBA to make our lives easier. In this article, we'll explore the various ways to delete columns in Excel using VBA, making your workflow more efficient and less prone to errors.
The Importance of Deleting Columns in Excel
Before we dive into the world of VBA, let's discuss why deleting columns is crucial in Excel. When working with large datasets, it's not uncommon to have unnecessary columns that take up space and slow down your workbook. By deleting these columns, you can:
- Improve data organization and readability
- Reduce file size and increase performance
- Prevent errors caused by unnecessary data
- Make data analysis and manipulation easier
Using VBA to Delete Columns
Now that we've covered the importance of deleting columns, let's get started with the fun part – using VBA to automate this process!
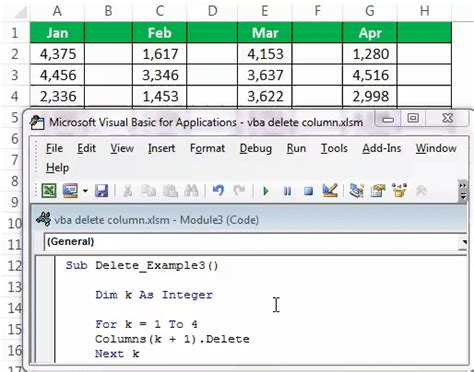
Method 1: Deleting a Single Column
To delete a single column using VBA, you can use the following code:
Sub DeleteColumn()
Columns("A").Delete
End Sub
This code deletes the entire column A. You can replace "A" with the column letter you want to delete.
Method 2: Deleting Multiple Columns
To delete multiple columns, you can use the following code:
Sub DeleteColumns()
Columns("A:C").Delete
End Sub
This code deletes columns A, B, and C. You can replace "A:C" with the range of columns you want to delete.
Method 3: Deleting Columns Based on Condition
Sometimes, you may want to delete columns based on a specific condition, such as deleting columns with blank headers. You can use the following code:
Sub DeleteColumnsWithBlankHeaders()
Dim ws As Worksheet
Set ws = ThisWorkbook.ActiveSheet
For i = ws.Cells(1, ws.Columns.Count).End(xlToLeft).Column To 1 Step -1
If ws.Cells(1, i).Value = "" Then
ws.Columns(i).Delete
End If
Next i
End Sub
This code deletes columns with blank headers. You can modify the condition to suit your needs.
Method 4: Deleting Columns Using a Loop
To delete columns using a loop, you can use the following code:
Sub DeleteColumnsUsingLoop()
Dim ws As Worksheet
Set ws = ThisWorkbook.ActiveSheet
For i = 1 To ws.Columns.Count
If ws.Columns(i).Hidden = True Then
ws.Columns(i).Delete
End If
Next i
End Sub
This code deletes hidden columns. You can modify the condition to suit your needs.
Gallery of Excel VBA Delete Columns
Excel VBA Delete Columns Image Gallery
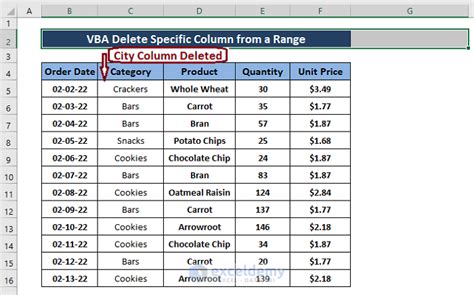
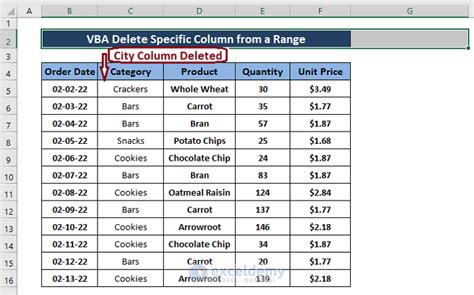
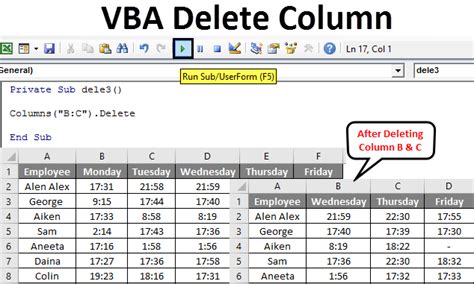
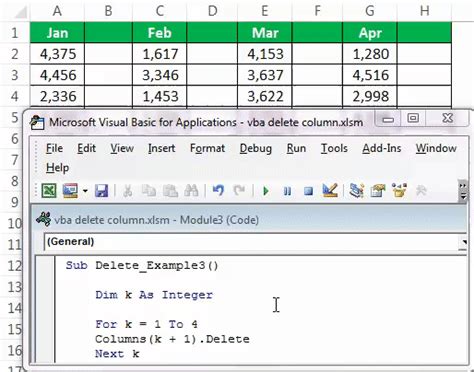
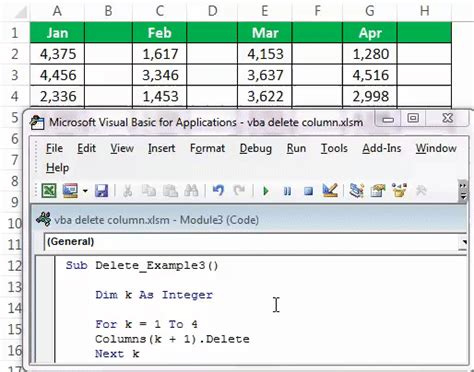
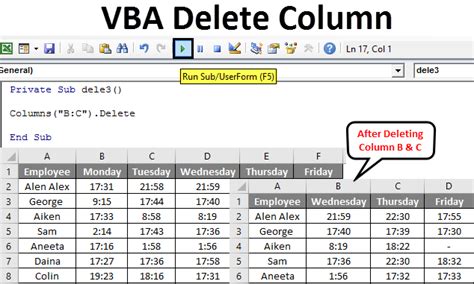
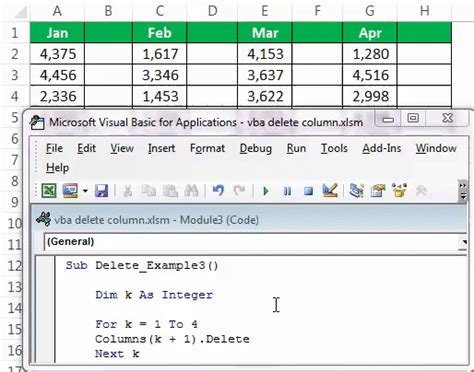
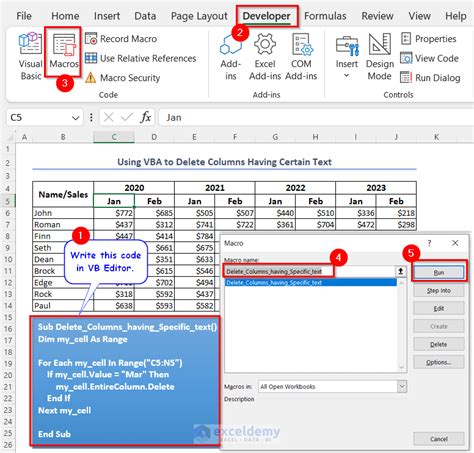
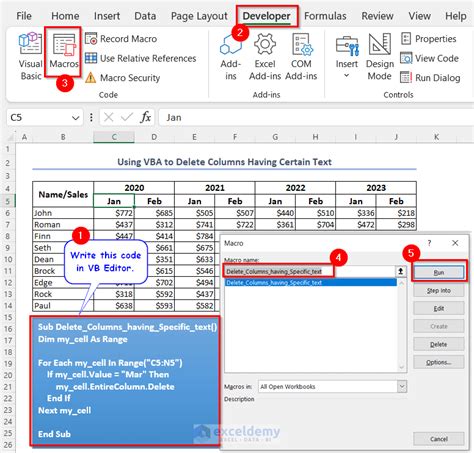
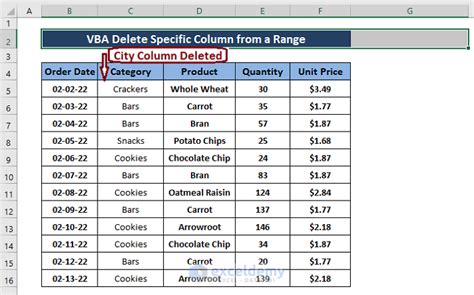
Frequently Asked Questions
Q: How do I delete columns in Excel using VBA?
A: You can use the Columns
object to delete columns in Excel using VBA.
Q: How do I delete multiple columns in Excel using VBA?
A: You can use the Columns
object with a range of columns to delete multiple columns.
Q: How do I delete columns based on a condition in Excel using VBA? A: You can use a loop to iterate through the columns and check for a specific condition before deleting the column.
Q: How do I troubleshoot errors when deleting columns in Excel using VBA?
A: You can use the On Error
statement to catch and handle errors when deleting columns.
Conclusion
Deleting columns in Excel using VBA can save you time and improve your workflow. By using the methods outlined in this article, you can delete single columns, multiple columns, and columns based on conditions. Remember to use best practices, debugging tips, and performance optimization techniques to ensure your code runs smoothly. Happy coding!