Intro
Boost your Excel VBA skills with efficient find and replace techniques. Learn 5 expert methods to streamline your workflow, including pattern matching, regular expressions, and dynamic range adjustments. Optimize your VBA code and master text manipulation with these actionable tips and tricks.
If you're an Excel power user, you're probably familiar with the frustration of manually searching for and replacing specific values or text within your worksheets. Fortunately, Excel VBA provides a robust Find and Replace functionality that can save you a significant amount of time and effort. In this article, we'll explore five ways to excel in VBA Find and Replace efficiently.
The Importance of Efficient Find and Replace
When working with large datasets, finding and replacing specific values or text can be a daunting task. Manual searches can be time-consuming, prone to errors, and may lead to inconsistencies in your data. By leveraging VBA's Find and Replace capabilities, you can automate this process, ensuring accuracy, speed, and efficiency.
Method 1: Using the Range.Find
Method
The Range.Find
method is a powerful tool for finding specific values or text within a range of cells. This method returns a Range
object that represents the first cell where the value is found.
Sub FindValue()
Dim rng As Range
Set rng = Range("A1:A100").Find("SearchValue")
If Not rng Is Nothing Then
MsgBox "Value found in cell " & rng.Address
Else
MsgBox "Value not found"
End If
End Sub
Method 2: Using the Range.FindNext
Method
The Range.FindNext
method is used to find the next occurrence of a value or text within a range of cells. This method returns a Range
object that represents the next cell where the value is found.
Sub FindNextValue()
Dim rng As Range
Set rng = Range("A1:A100").Find("SearchValue")
If Not rng Is Nothing Then
Do
MsgBox "Value found in cell " & rng.Address
Set rng = Range("A1:A100").FindNext(rng)
Loop Until rng Is Nothing
Else
MsgBox "Value not found"
End If
End Sub
Method 3: Using the Range.Replace
Method
The Range.Replace
method is used to replace a specific value or text within a range of cells.
Sub ReplaceValue()
Range("A1:A100").Replace "SearchValue", "ReplaceValue"
End Sub
Method 4: Using the WorksheetFunction.Find
Function
The WorksheetFunction.Find
function is a more flexible alternative to the Range.Find
method. This function returns the relative position of the value or text within the range.
Sub FindValueWF()
Dim rng As Range
Set rng = Range("A1:A100")
Dim findPos As Integer
findPos = WorksheetFunction.Find("SearchValue", rng)
If findPos > 0 Then
MsgBox "Value found in cell " & rng.Cells(findPos).Address
Else
MsgBox "Value not found"
End If
End Sub
Method 5: Using Regular Expressions
Regular expressions (RegEx) provide a powerful way to search for patterns within text. Excel VBA supports RegEx through the VBScript_RegExp_1_0
library.
Sub FindValueRegEx()
Dim regex As New RegExp
regex.Pattern = "SearchValue"
regex.IgnoreCase = True
Dim rng As Range
Set rng = Range("A1:A100")
If regex.Test(rng.Value) Then
MsgBox "Value found in cell " & rng.Address
Else
MsgBox "Value not found"
End If
End Sub
Gallery of VBA Find and Replace Examples
VBA Find and Replace Image Gallery
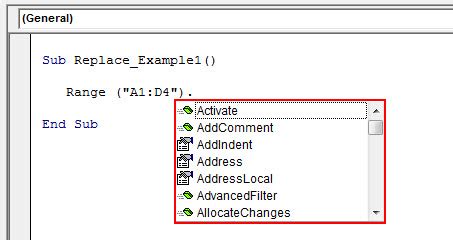
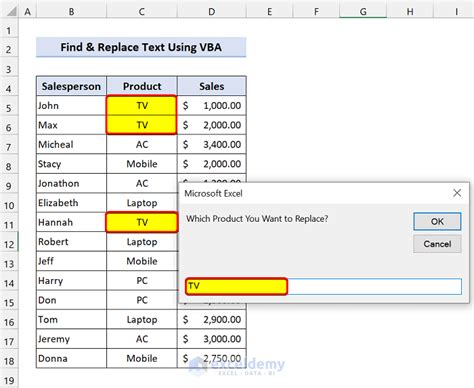
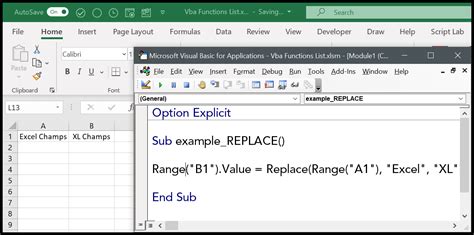
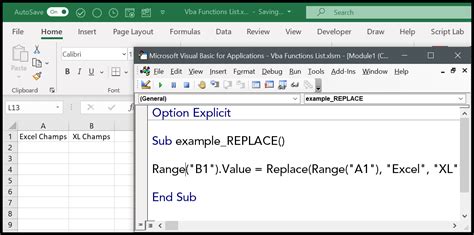
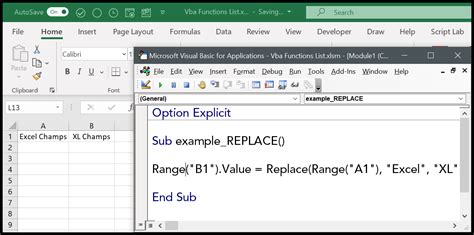
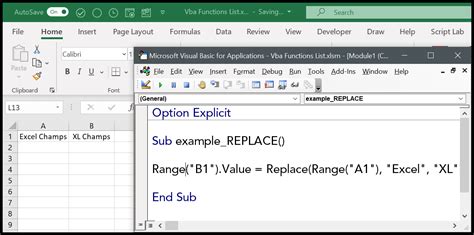
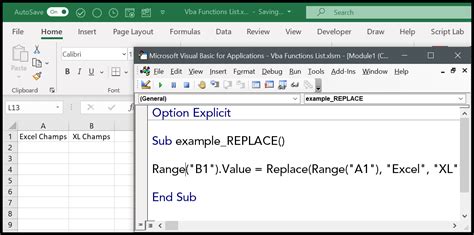
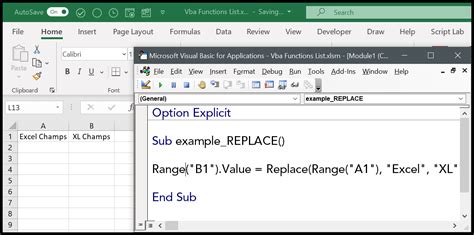
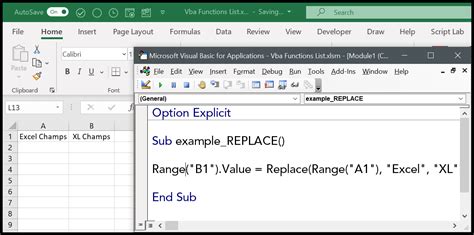
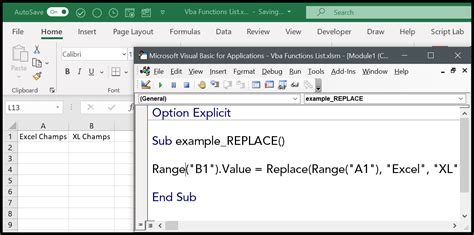
By mastering these five methods, you'll be able to efficiently find and replace values or text within your Excel worksheets using VBA. Remember to practice and experiment with different techniques to find what works best for your specific use case.
If you have any questions or would like to share your own VBA Find and Replace experiences, please leave a comment below. Don't forget to share this article with your fellow Excel enthusiasts and help them unlock the power of VBA!