Intro
Master Excel VBA with ease! Learn 4 simple ways to get the current year in Excel VBA, including using the Year function, Now function, Date function, and Environ function. Discover how to automate year-based calculations and improve your VBA skills. Get the latest techniques for working with dates in Excel VBA.
Working with dates and years is a common task in Excel VBA, and getting the current year is a fundamental requirement in many applications. In this article, we will explore four different methods to get the current year in Excel VBA.
Why Do You Need to Get the Current Year in Excel VBA?
There are several scenarios where you might need to get the current year in Excel VBA. For instance, you might want to:
- Automatically update the year in a date column
- Create a dynamic range based on the current year
- Use the current year in a formula or calculation
- Update the year in a header or footer
Regardless of the reason, getting the current year in Excel VBA is a straightforward process. Let's dive into the four methods.
Method 1: Using the `Year` Function
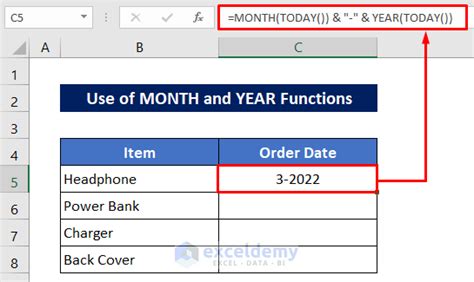
The Year
function is a built-in VBA function that returns the year of a given date. You can use this function to get the current year by passing the Date
function as an argument.
Sub GetCurrentYear()
Dim currentYear As Integer
currentYear = Year(Date)
MsgBox "The current year is: " & currentYear
End Sub
Method 2: Using the `Format` Function
Another way to get the current year is by using the Format
function, which returns a string representation of a date. You can format the date as "yyyy" to get the year.
Sub GetCurrentYear()
Dim currentYear As String
currentYear = Format(Date, "yyyy")
MsgBox "The current year is: " & currentYear
End Sub
Method 3: Using the `Now` Function
The Now
function returns the current date and time. You can use this function to get the current year by extracting the year from the returned date.
Sub GetCurrentYear()
Dim currentYear As Integer
currentYear = Year(Now)
MsgBox "The current year is: " & currentYear
End Sub
Method 4: Using the `DateSerial` Function
The DateSerial
function returns a date based on a year, month, and day. You can use this function to get the current year by creating a date with the current year and then extracting the year from it.
Sub GetCurrentYear()
Dim currentYear As Integer
currentYear = Year(DateSerial(Year(Date), 1, 1))
MsgBox "The current year is: " & currentYear
End Sub
Gallery of Excel VBA Date Functions
Excel VBA Date Functions
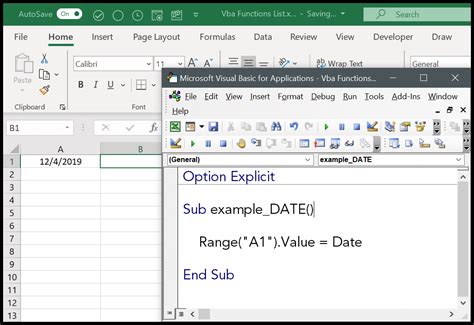
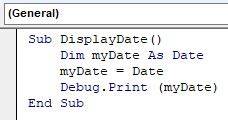
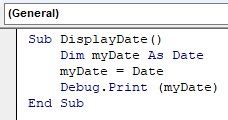
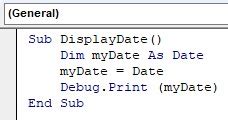
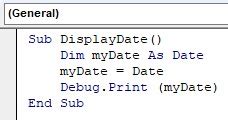
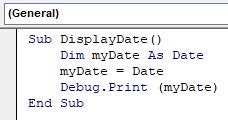
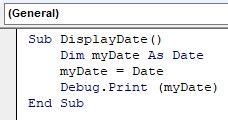
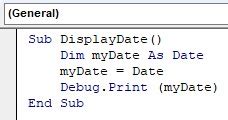
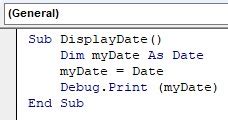
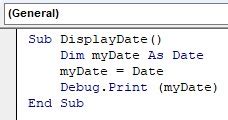
Conclusion
Getting the current year in Excel VBA is a simple process that can be achieved using four different methods. Whether you use the Year
function, Format
function, Now
function, or DateSerial
function, you can easily retrieve the current year in your VBA code.
If you have any questions or need further assistance, feel free to comment below. Share this article with your friends and colleagues who might find it useful.