Intro
Master Excel VBA with our expert guide on how to select a sheet in Excel VBA. Discover 5 efficient ways to manipulate worksheets, including activating sheets, using worksheet objects, and iterating through sheets. Learn how to work with worksheets, ranges, and cells in VBA, and improve your Excel automation skills.
Selecting a sheet in Excel VBA is an essential task when working with multiple worksheets in a single workbook. Properly referencing the desired sheet enables you to manipulate its data, modify its layout, and perform various other operations efficiently. In this article, we'll delve into five distinct methods to select a sheet in Excel VBA, providing you with the flexibility to choose the approach that best suits your specific needs.
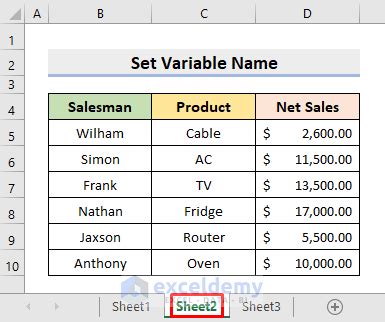
1. Using the Worksheets
Collection
One of the most straightforward methods to select a sheet in Excel VBA is by using the Worksheets
collection. This approach involves referencing the worksheet by its index number or its name.
Sub SelectSheetByIndex()
' Select the first worksheet in the workbook
Worksheets(1).Select
End Sub
Sub SelectSheetByName()
' Select the worksheet named "Sheet1"
Worksheets("Sheet1").Select
End Sub
2. Using the Sheets
Collection
Similar to the Worksheets
collection, the Sheets
collection can also be used to select a sheet in Excel VBA. The primary difference between the two collections is that Sheets
includes all types of sheets (worksheets and chart sheets), while Worksheets
only includes worksheets.
Sub SelectSheetByIndexUsingSheets()
' Select the first sheet in the workbook (can be a worksheet or chart sheet)
Sheets(1).Select
End Sub
Sub SelectSheetByNameUsingSheets()
' Select the sheet named "Sheet1" (can be a worksheet or chart sheet)
Sheets("Sheet1").Select
End Sub
3. Using the Activate
Method
Another way to select a sheet in Excel VBA is by using the Activate
method. This approach is particularly useful when you need to switch between different sheets and perform actions on the active sheet.
Sub ActivateSheet()
' Activate the worksheet named "Sheet1"
Worksheets("Sheet1").Activate
End Sub
4. Using the Select
Method with Range
You can also select a sheet by selecting a range on that sheet. This method is helpful when you want to perform actions on a specific range within a sheet.
Sub SelectRangeOnSheet()
' Select cell A1 on the worksheet named "Sheet1"
Worksheets("Sheet1").Range("A1").Select
End Sub
5. Using the For Each
Loop
If you need to iterate through all sheets in a workbook and perform actions on each one, you can use a For Each
loop to select each sheet.
Sub IterateThroughSheets()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
' Perform actions on the current worksheet
ws.Select
' Your code here...
Next ws
End Sub
In conclusion, selecting a sheet in Excel VBA can be accomplished in various ways, depending on your specific requirements. By mastering these different methods, you can efficiently navigate and manipulate your worksheets, ultimately streamlining your VBA coding tasks.
Gallery of Excel VBA Select Sheet Examples
Excel VBA Select Sheet Image Gallery
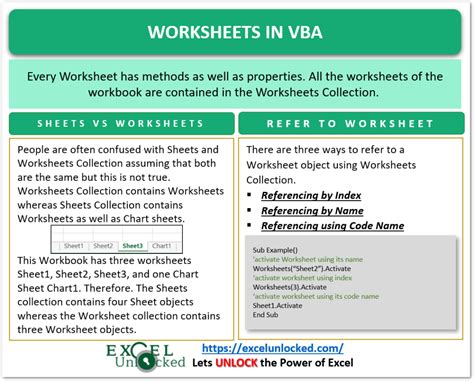
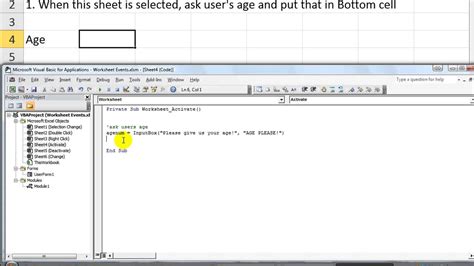
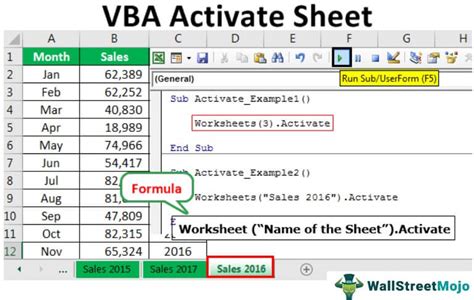
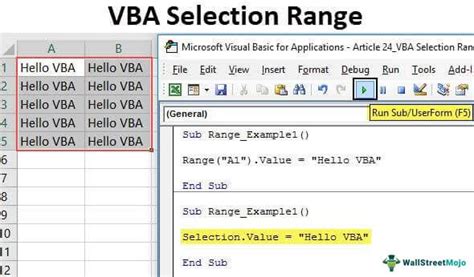
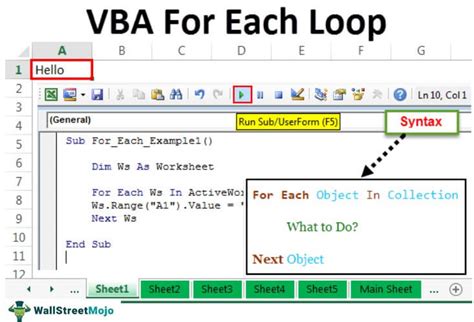
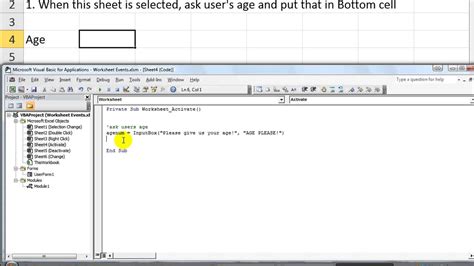
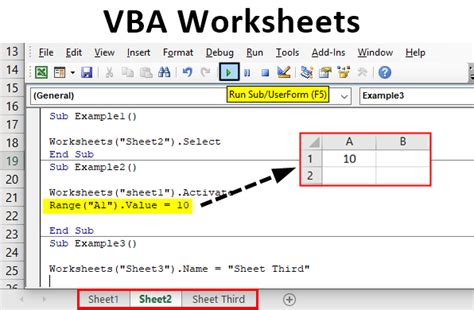
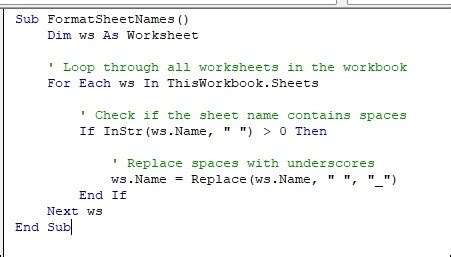
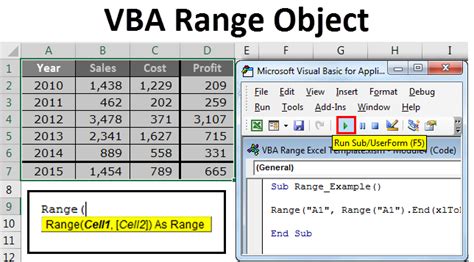
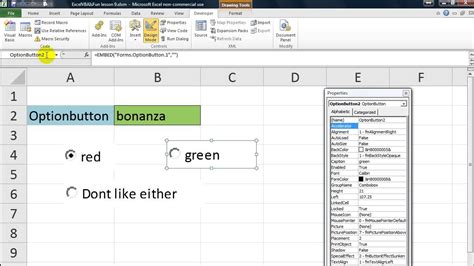
Do you have any questions or need further clarification on selecting a sheet in Excel VBA? Share your thoughts and experiences in the comments section below!