Intro
Discover how to transpose ranges in Excel VBA with ease. Learn 5 powerful methods to rotate, flip, and transform your data with VBA code. Master techniques for transposing tables, arrays, and ranges, and improve your Excel automation skills. Get expert tips on data manipulation, range offset, and worksheet looping. Transform your data with Excel VBA transposition.
Transpose Range in Excel VBA
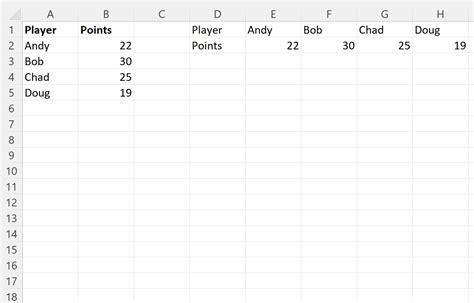
Excel VBA is a powerful tool for automating tasks in Excel. One common task is transposing a range of cells, which can be useful for rearranging data or preparing it for analysis. In this article, we'll explore five ways to transpose a range in Excel VBA.
Transposing a range involves swapping the rows and columns of a selection of cells. For example, if you have a range of cells with three rows and four columns, transposing it would result in a range with four rows and three columns. This can be useful for a variety of tasks, such as rearranging data for a pivot table or preparing data for a chart.
Method 1: Using the Transpose Function
The most straightforward way to transpose a range in Excel VBA is to use the Transpose function. This function takes a range of cells as input and returns a new range with the rows and columns swapped.Here's an example of how to use the Transpose function in VBA:
Sub TransposeRange()
Dim originalRange As Range
Dim transposedRange As Range
Set originalRange = Range("A1:C4")
Set transposedRange = Application.WorksheetFunction.Transpose(originalRange)
transposedRange.Resize(UBound(transposedRange, 1), UBound(transposedRange, 2)).Value = transposedRange
End Sub
This code defines a subroutine called TransposeRange that takes a range of cells (A1:C4) and transposes it using the Transpose function. The resulting transposed range is then assigned to a new range of cells.
Method 2: Using a Loop
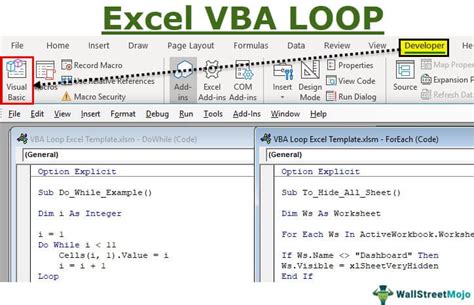
Another way to transpose a range in Excel VBA is to use a loop to iterate over the cells in the original range and assign their values to a new range with the rows and columns swapped.
Here's an example of how to use a loop to transpose a range in VBA:
Sub TransposeRangeLoop()
Dim originalRange As Range
Dim transposedRange As Range
Dim i As Long
Dim j As Long
Set originalRange = Range("A1:C4")
Set transposedRange = Range("E1")
For i = 1 To originalRange.Rows.Count
For j = 1 To originalRange.Columns.Count
transposedRange.Offset(j - 1, i - 1).Value = originalRange.Cells(i, j).Value
Next j
Next i
End Sub
This code defines a subroutine called TransposeRangeLoop that uses two nested loops to iterate over the cells in the original range and assign their values to a new range with the rows and columns swapped.
Method 3: Using an Array
A third way to transpose a range in Excel VBA is to use an array to store the values from the original range and then assign them to a new range with the rows and columns swapped.Here's an example of how to use an array to transpose a range in VBA:
Sub TransposeRangeArray()
Dim originalRange As Range
Dim transposedRange As Range
Dim dataArray As Variant
Set originalRange = Range("A1:C4")
dataArray = originalRange.Value
Set transposedRange = Range("E1")
transposedRange.Resize(UBound(dataArray, 2), UBound(dataArray, 1)).Value = Application.WorksheetFunction.Transpose(dataArray)
End Sub
This code defines a subroutine called TransposeRangeArray that uses an array to store the values from the original range and then assigns them to a new range with the rows and columns swapped using the Transpose function.
Method 4: Using a Worksheet Function
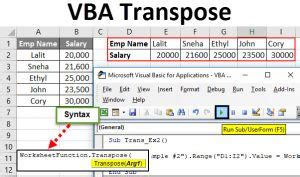
A fourth way to transpose a range in Excel VBA is to use a worksheet function to transpose the range.
Here's an example of how to use a worksheet function to transpose a range in VBA:
Sub TransposeRangeWorksheetFunction()
Dim originalRange As Range
Dim transposedRange As Range
Set originalRange = Range("A1:C4")
Set transposedRange = Range("E1")
transposedRange.FormulaArray = "=TRANSPOSE(" & originalRange.Address & ")"
transposedRange.Resize(UBound(transposedRange, 1), UBound(transposedRange, 2)).Value = transposedRange.Value
End Sub
This code defines a subroutine called TransposeRangeWorksheetFunction that uses a worksheet function to transpose the range.
Method 5: Using Power Query
A fifth way to transpose a range in Excel VBA is to use Power Query.Here's an example of how to use Power Query to transpose a range in VBA:
Sub TransposeRangePowerQuery()
Dim originalRange As Range
Dim transposedRange As Range
Set originalRange = Range("A1:C4")
Set transposedRange = Range("E1")
Dim pq As Object
Set pq = originalRange.Worksheet.ListObjects.Add(xlSrcRange, originalRange,, xlYes)
pq.Query.Table.Transpose(1).Load Destination:=transposedRange
End Sub
This code defines a subroutine called TransposeRangePowerQuery that uses Power Query to transpose the range.
Excel VBA Transpose Range Image Gallery
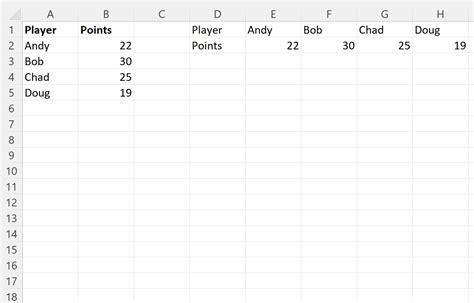
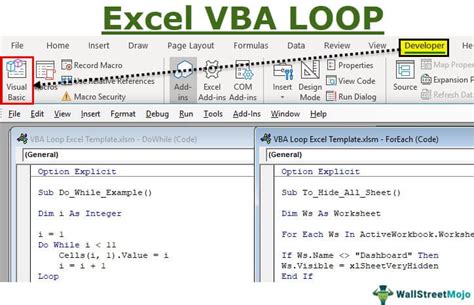
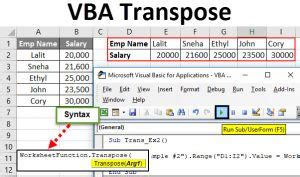
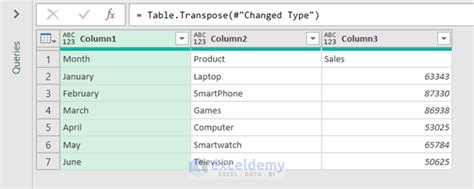
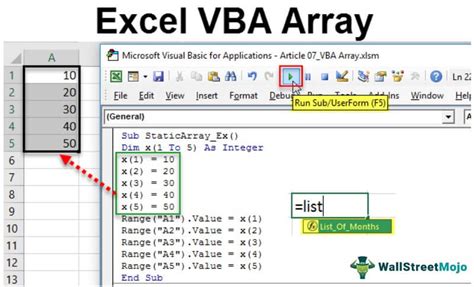
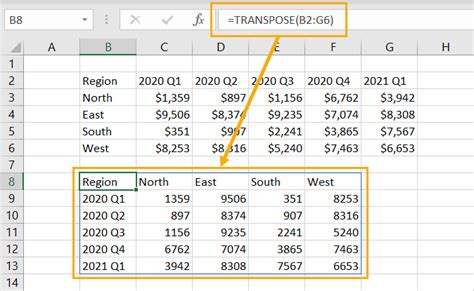
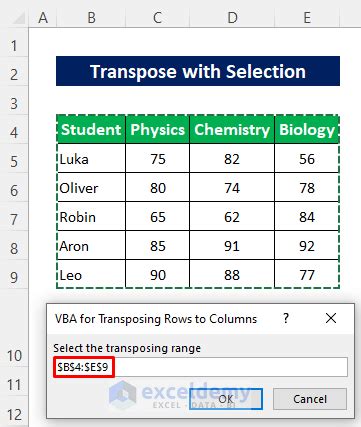
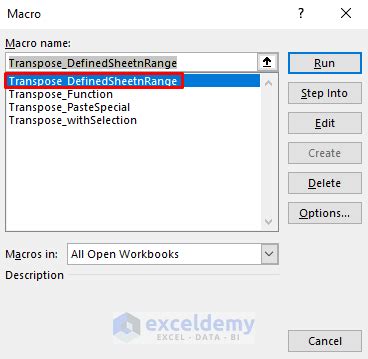
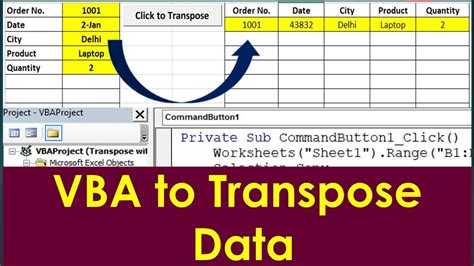
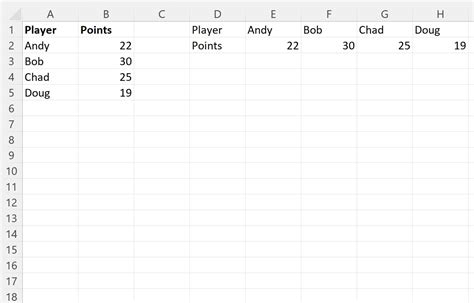
In conclusion, there are several ways to transpose a range in Excel VBA, each with its own strengths and weaknesses. By choosing the right method for your needs, you can easily transpose ranges and perform complex data manipulation tasks in Excel. Whether you're a beginner or an experienced VBA user, we hope this article has provided you with the knowledge and tools you need to get the job done. If you have any questions or need further assistance, please don't hesitate to ask.