Intro
Unlock the power of dynamic range duplication in Excel with Range Copy VBA techniques. Master copying formulas, values, and formats with ease. Discover how to automate range copying, duplicate data, and apply formatting using VBA macros. Boost productivity and efficiency in your Excel workflows with these expert-driven range copy techniques and best practices.
In the world of Excel VBA, working with ranges is an essential skill for any developer or power user. One of the most versatile and powerful techniques in VBA is the ability to duplicate ranges dynamically. Whether you're creating a reporting tool, automating data entry, or building a dashboard, knowing how to copy ranges with VBA can save you time and effort.
The ability to dynamically duplicate ranges is especially useful when working with datasets that constantly change or when creating templates that need to adapt to different data sizes. In this article, we'll delve into the world of range copying in VBA, exploring various techniques, and providing practical examples to help you master this skill.
Understanding Range Copying in VBA
Before we dive into the techniques, it's essential to understand the basics of range copying in VBA. When you copy a range in VBA, you're essentially creating a new range that is an exact replica of the original. This new range can be located anywhere in the workbook, and it will inherit all the properties of the original range, including formatting, formulas, and data.
There are two primary methods of copying ranges in VBA: using the Range.Copy
method and using the Range.CopyDestination
method. We'll explore both methods in detail, along with some advanced techniques for dynamic range duplication.
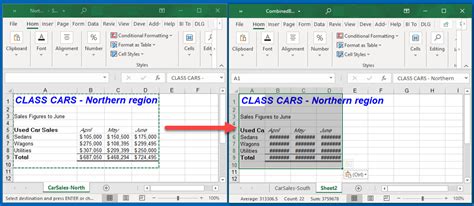
Method 1: Using the Range.Copy Method
The Range.Copy
method is the most straightforward way to copy a range in VBA. This method allows you to specify the destination range where you want to copy the original range. Here's an example:
Sub CopyRange()
Range("A1:B10").Copy Destination:=Range("C1")
End Sub
In this example, we're copying the range A1:B10
to the destination range C1
. Note that the destination range must be a single cell, not a range.
Method 2: Using the Range.CopyDestination Method
The Range.CopyDestination
method is similar to the Range.Copy
method, but it allows you to specify a destination range instead of a single cell. Here's an example:
Sub CopyRangeDestination()
Range("A1:B10").CopyDestination:=Range("C1:D10")
End Sub
In this example, we're copying the range A1:B10
to the destination range C1:D10
. Note that the destination range must be the same size as the original range.
Dynamic Range Duplication Techniques
Now that we've covered the basics, let's explore some advanced techniques for dynamic range duplication.
Technique 1: Using Variables to Specify the Range
One of the most powerful ways to dynamically copy ranges is to use variables to specify the range. You can use variables to store the range addresses, and then use those variables in your code. Here's an example:
Sub DynamicCopyRange()
Dim srcRange As Range
Dim dstRange As Range
Set srcRange = Range("A1:B10")
Set dstRange = Range("C1")
srcRange.Copy Destination:=dstRange
End Sub
In this example, we're using variables srcRange
and dstRange
to store the source and destination range addresses. We then use those variables to copy the range.
Technique 2: Using Loops to Copy Multiple Ranges
Another technique for dynamic range duplication is to use loops to copy multiple ranges. You can use a For
loop or a Do While
loop to iterate through a range of cells and copy each range to a new location. Here's an example:
Sub CopyMultipleRanges()
Dim i As Integer
Dim srcRange As Range
Dim dstRange As Range
For i = 1 To 10
Set srcRange = Range("A" & i & ":B" & i)
Set dstRange = Range("C" & i & ":D" & i)
srcRange.Copy Destination:=dstRange
Next i
End Sub
In this example, we're using a For
loop to iterate through the range A1:B10
and copy each range to a new location.
Conclusion
In this article, we've explored the world of range copying in VBA, covering the basics of range copying and advanced techniques for dynamic range duplication. We've seen how to use the Range.Copy
method and the Range.CopyDestination
method, as well as how to use variables and loops to dynamically copy ranges.
Whether you're a seasoned developer or a power user, mastering range copying in VBA can save you time and effort. By using the techniques outlined in this article, you'll be able to create dynamic and flexible solutions that can adapt to changing data sizes and requirements.
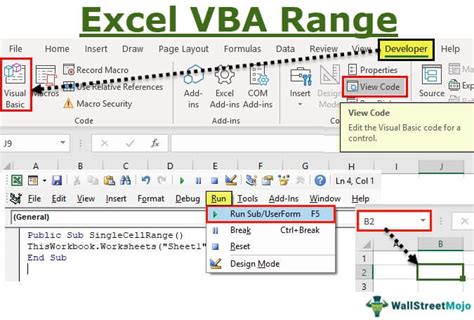
Gallery of Excel VBA Range Copying
Excel VBA Range Copying Image Gallery
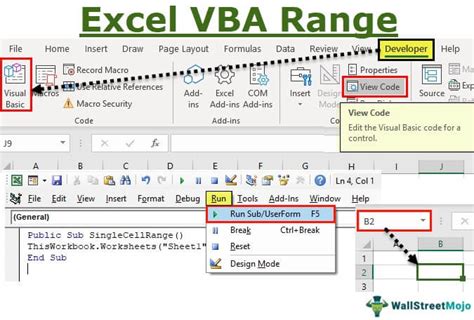
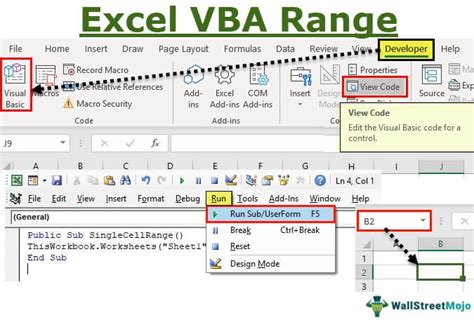
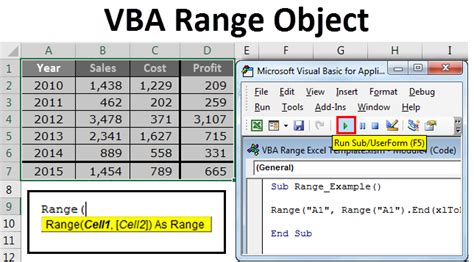
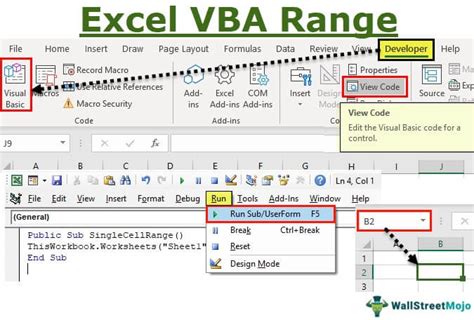
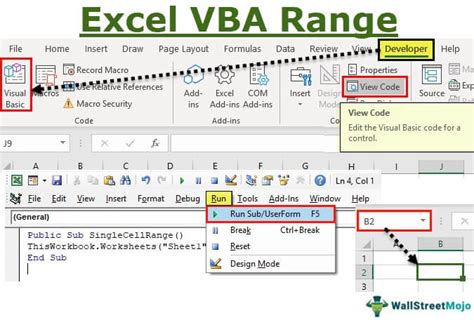
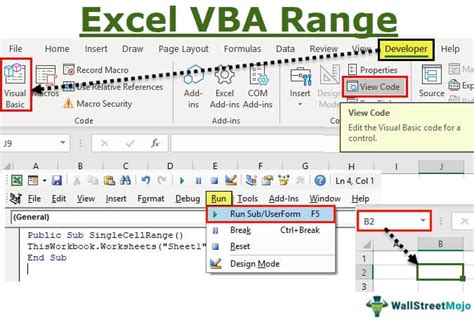
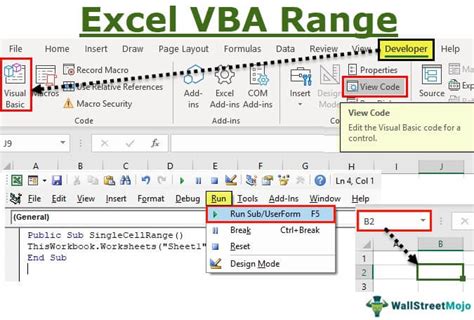
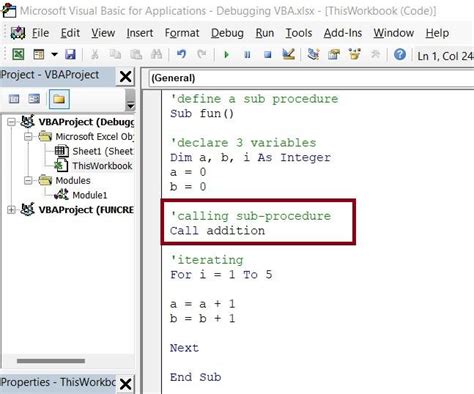
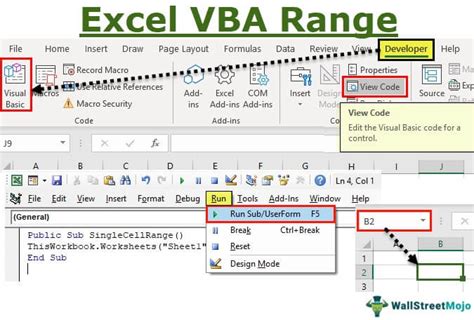
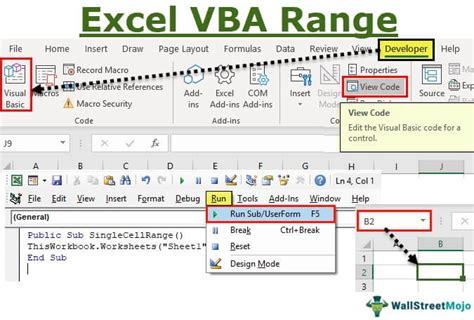
We hope you found this article helpful in mastering range copying in VBA. If you have any questions or need further assistance, please don't hesitate to ask.