Renaming files is a common task in data management and automation, and Visual Basic for Applications (VBA) provides several ways to accomplish this task. In this article, we will explore three ways to rename a file in VBA, including using the Name
statement, the FileCopy
method, and the FileSystemObject
object.
Method 1: Using the `Name` Statement
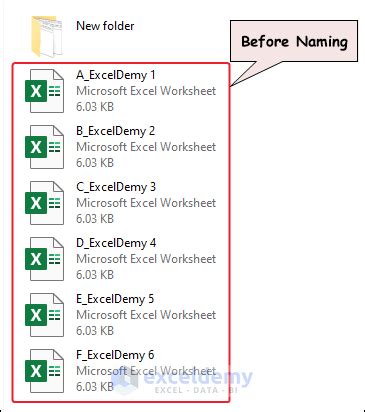
The Name
statement is a simple way to rename a file in VBA. This statement takes two arguments: the original file name and the new file name. Here is an example of how to use the Name
statement to rename a file:
Sub RenameFile_NameStatement()
Name "C:\OriginalFile.txt" As "C:\NewFileName.txt"
End Sub
In this example, the file "OriginalFile.txt" is renamed to "NewFileName.txt" using the Name
statement. Make sure to specify the full path to the file, including the file extension.
Method 2: Using the `FileCopy` Method
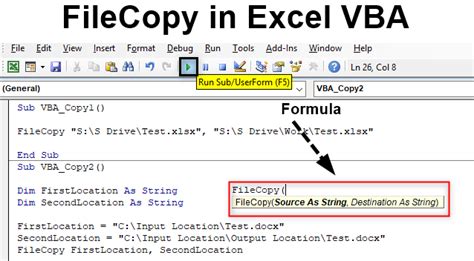
The FileCopy
method is another way to rename a file in VBA. This method takes two arguments: the source file name and the destination file name. To rename a file using the FileCopy
method, you need to copy the file to a new location with a new name, and then delete the original file. Here is an example of how to use the FileCopy
method to rename a file:
Sub RenameFile_FileCopyMethod()
FileCopy "C:\OriginalFile.txt", "C:\NewFileName.txt"
Kill "C:\OriginalFile.txt"
End Sub
In this example, the file "OriginalFile.txt" is copied to a new location with the name "NewFileName.txt" using the FileCopy
method. Then, the original file is deleted using the Kill
statement.
Method 3: Using the `FileSystemObject` Object
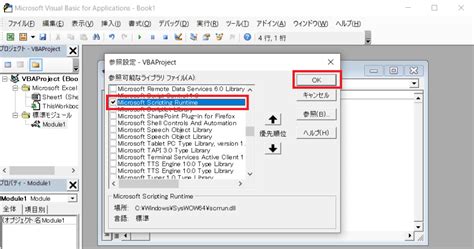
The FileSystemObject
object is a powerful object in VBA that provides a range of methods for working with files and folders. To rename a file using the FileSystemObject
object, you need to create an instance of the object and use the MoveFile
method. Here is an example of how to use the FileSystemObject
object to rename a file:
Sub RenameFile_FSO()
Dim fso As Object
Set fso = CreateObject("Scripting.FileSystemObject")
fso.MoveFile "C:\OriginalFile.txt", "C:\NewFileName.txt"
Set fso = Nothing
End Sub
In this example, an instance of the FileSystemObject
object is created using the CreateObject
function. Then, the MoveFile
method is used to rename the file "OriginalFile.txt" to "NewFileName.txt".
Renaming Files in VBA Image Gallery
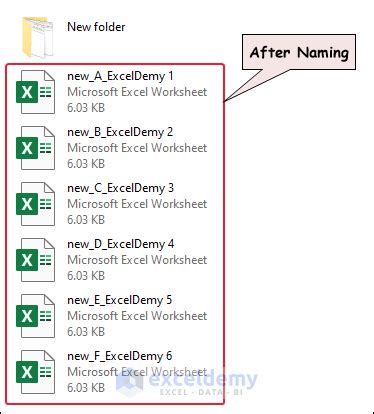
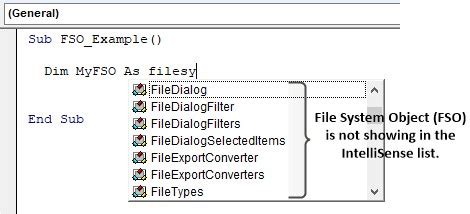
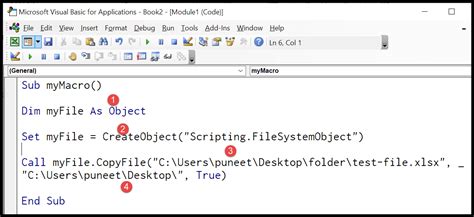
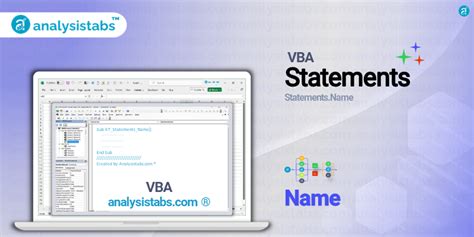
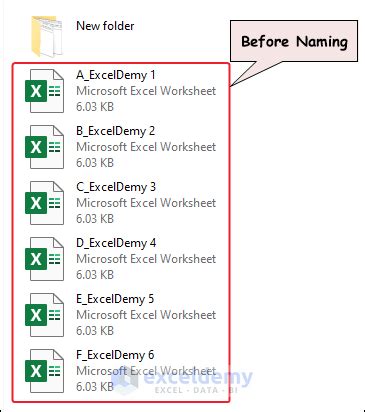
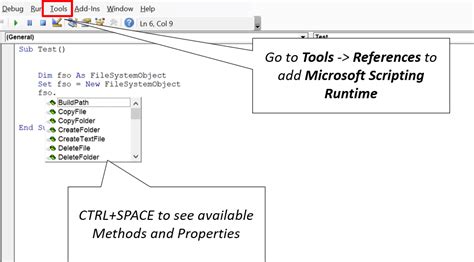
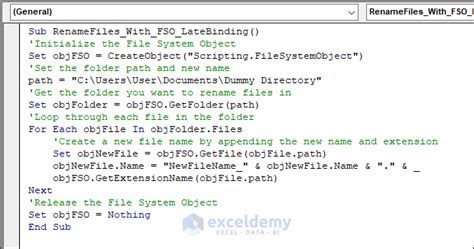
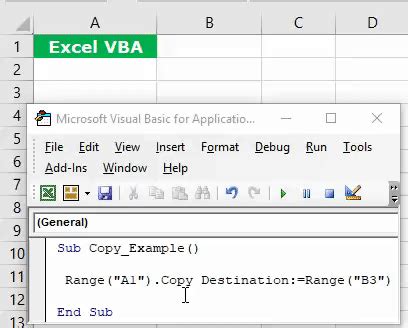
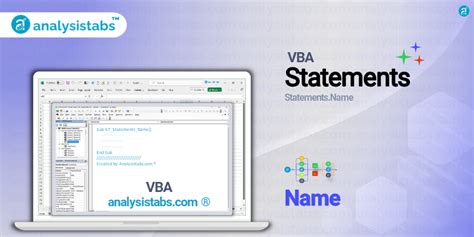
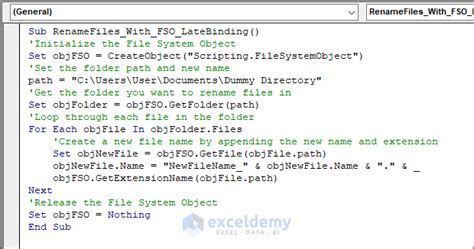
In conclusion, renaming a file in VBA can be accomplished using the Name
statement, the FileCopy
method, or the FileSystemObject
object. Each method has its own advantages and disadvantages, and the choice of method depends on the specific requirements of your project. By understanding the different ways to rename a file in VBA, you can improve your data management and automation skills.
If you have any questions or need further clarification on renaming files in VBA, please leave a comment below.