Intro
Master VBA column selection with ease! Discover 5 efficient ways to select a column in VBA, including referencing by index, header, and range. Learn how to automate tasks, optimize code, and improve productivity. Get expert tips on VBA column selection, range manipulation, and spreadsheet automation to streamline your workflow.
When working with Excel VBA, selecting a column can be a crucial step in automating tasks. Whether you're trying to manipulate data, perform calculations, or create reports, selecting the right column can make all the difference. In this article, we'll explore five different ways to select a column in VBA, highlighting the benefits and drawbacks of each method.
Why Select a Column in VBA?
Before we dive into the different methods, it's essential to understand why selecting a column in VBA is important. By selecting a column, you can:
- Perform calculations and data manipulation on a specific set of data
- Create charts and reports based on specific columns
- Use VBA to automate tasks, such as data validation and formatting
- Improve code readability and maintainability by working with specific columns
Method 1: Using the Range
Object
One of the most common ways to select a column in VBA is by using the Range
object. You can specify the column letter or number, and the Range
object will select the entire column.
Sub SelectColumnUsingRange()
Dim columnRange As Range
Set columnRange = Range("A:A")
columnRange.Select
End Sub
In this example, the code selects the entire column A.
Pros: Easy to use, flexible, and works with most Excel versions.
Cons: Can be slow for large datasets, and may cause issues if the worksheet is protected.
Method 2: Using the Columns
Object
Another way to select a column is by using the Columns
object. This method allows you to specify the column number, and the Columns
object will select the entire column.
Sub SelectColumnUsingColumns()
Dim columnNumber As Long
columnNumber = 1
Columns(columnNumber).Select
End Sub
In this example, the code selects the first column (column A).
Pros: Fast and efficient, works well with large datasets.
Cons: Limited flexibility, as you can only specify column numbers.
Method 3: Using the ListObjects
Object
If you're working with tables in Excel, you can use the ListObjects
object to select a column. This method allows you to specify the table name and column name, and the ListObjects
object will select the entire column.
Sub SelectColumnUsingListObjects()
Dim tableName As String
Dim columnName As String
tableName = "Table1"
columnName = "Column1"
ListObjects(tableName).ListColumns(columnName).Range.Select
End Sub
In this example, the code selects the "Column1" column in the "Table1" table.
Pros: Works well with tables, allows for easy data manipulation.
Cons: Limited flexibility, as you can only work with tables.
Method 4: Using the Offset
Property
You can also select a column using the Offset
property. This method allows you to specify the starting cell and offset, and the Offset
property will select the entire column.
Sub SelectColumnUsingOffset()
Dim startCell As Range
Set startCell = Range("A1")
startCell.Offset(0, 0).Resize(1048576, 1).Select
End Sub
In this example, the code selects the entire column A, starting from cell A1.
Pros: Fast and efficient, works well with large datasets.
Cons: Can be tricky to use, requires knowledge of the Offset
property.
Method 5: Using the SpecialCells
Method
Finally, you can select a column using the SpecialCells
method. This method allows you to specify the cell type and area, and the SpecialCells
method will select the entire column.
Sub SelectColumnUsingSpecialCells()
Range("A1").SpecialCells(xlCellTypeConstants).EntireColumn.Select
End Sub
In this example, the code selects the entire column A, starting from cell A1, and containing only constant values.
Pros: Fast and efficient, works well with large datasets.
Cons: Limited flexibility, as you can only select specific cell types.
Conclusion
Selecting a column in VBA can be a crucial step in automating tasks and working with data. In this article, we explored five different ways to select a column, highlighting the benefits and drawbacks of each method. Whether you're using the Range
object, Columns
object, ListObjects
object, Offset
property, or SpecialCells
method, the key is to choose the method that best fits your needs and data.
Gallery Section
VBA Column Selection Gallery
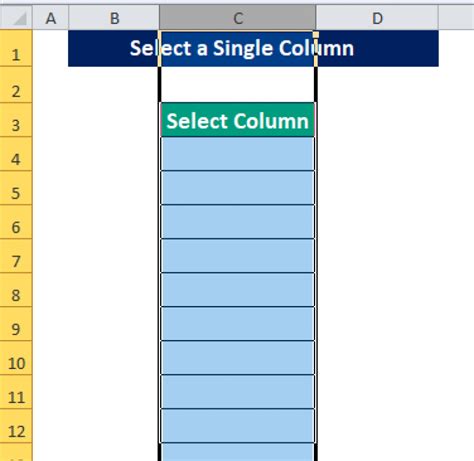
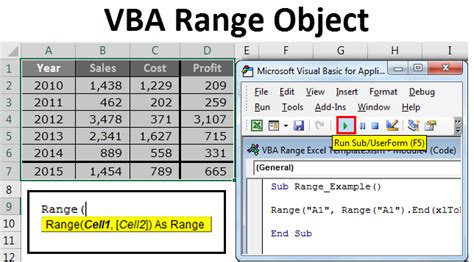
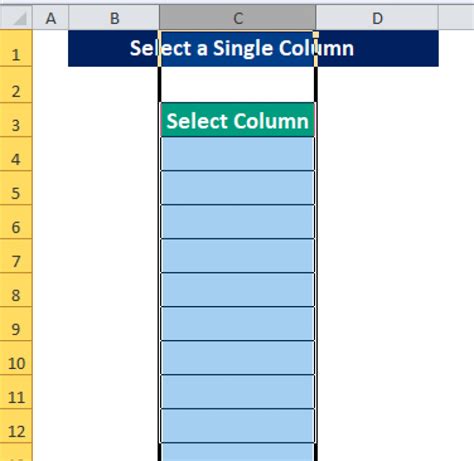
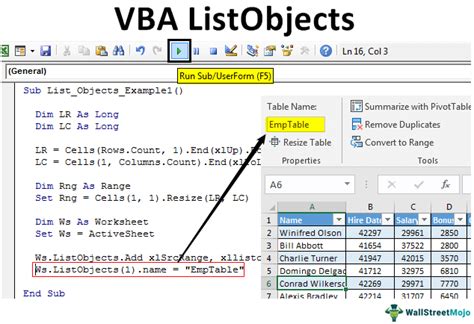
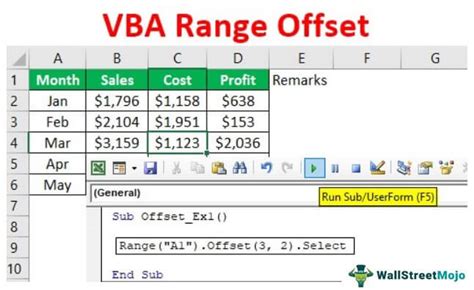
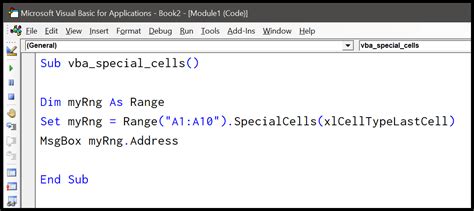
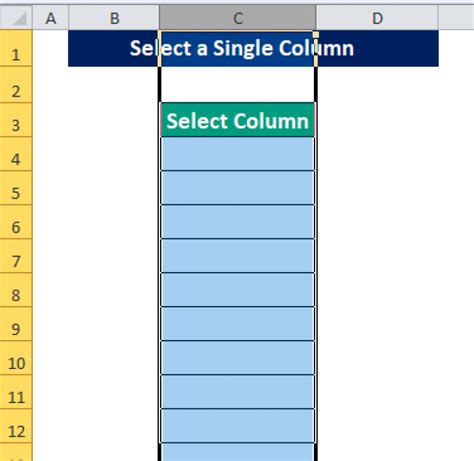
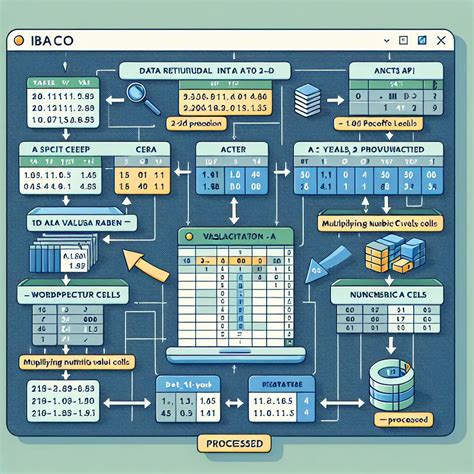
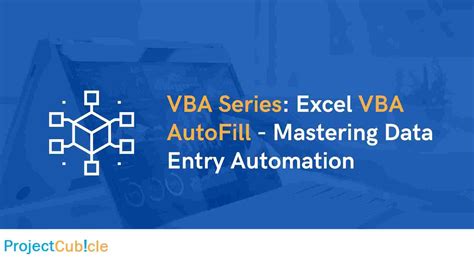
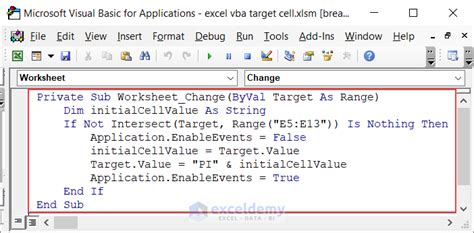
We hope this article has helped you learn more about selecting columns in VBA. Whether you're a beginner or an expert, we encourage you to share your thoughts and experiences in the comments section below.