Intro
Mastering VBA range selection is crucial for efficient data manipulation. Discover 5 expert-approved methods to select a range in VBA, including using Range.Find, Range.Offset, and Range.Resize. Learn how to define and manipulate ranges with ease, optimize your code, and improve your Excel automation skills with this comprehensive guide.
When working with Excel VBA, one of the most common tasks is selecting a range of cells. This can be a simple task, but it's essential to do it correctly to avoid errors and ensure your code runs efficiently. In this article, we'll explore five ways to select a range in VBA, along with practical examples and tips to help you master this skill.
The Importance of Selecting a Range in VBA
Before we dive into the methods, let's understand why selecting a range is crucial in VBA. When you select a range, you're telling VBA which cells to perform actions on. This can include formatting, calculations, data manipulation, and more. If you don't select the correct range, your code may produce incorrect results or throw errors.
Method 1: Using the Range Object
The Range object is the most common way to select a range in VBA. You can use the Range property to specify the range of cells you want to work with.
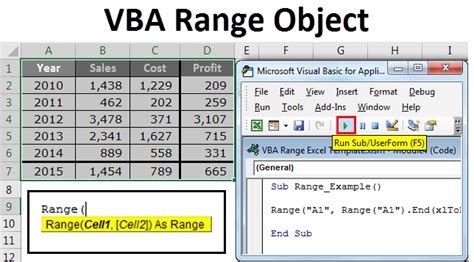
Here's an example:
Sub SelectRange()
Dim myRange As Range
Set myRange = Range("A1:B2")
myRange.Select
End Sub
In this example, we declare a Range object called myRange and set it to the range A1:B2 using the Range property. Finally, we use the Select method to select the range.
Method 2: Using the Cells Property
The Cells property is another way to select a range in VBA. This method allows you to specify the range using row and column numbers.
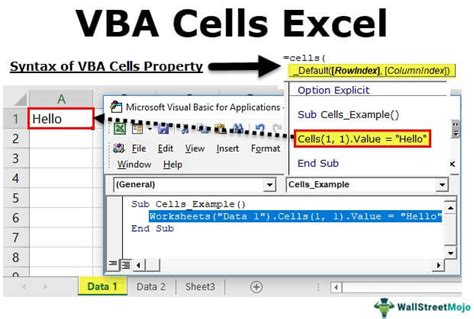
Here's an example:
Sub SelectRange()
Dim myRange As Range
Set myRange = Range(Cells(1, 1), Cells(2, 2))
myRange.Select
End Sub
In this example, we use the Cells property to specify the range A1:B2 using row and column numbers.
Method 3: Using the Offset Property
The Offset property allows you to select a range relative to another range. This method is useful when you need to select a range that is offset from another range.
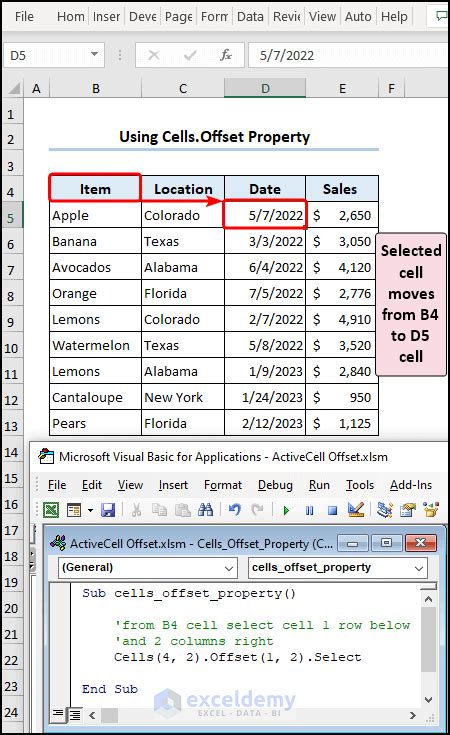
Here's an example:
Sub SelectRange()
Dim myRange As Range
Set myRange = Range("A1").Offset(1, 1).Resize(2, 2)
myRange.Select
End Sub
In this example, we use the Offset property to select a range that is offset from cell A1 by one row and one column. We also use the Resize method to set the size of the range.
Method 4: Using the Union Method
The Union method allows you to select multiple ranges at once. This method is useful when you need to perform actions on multiple ranges simultaneously.
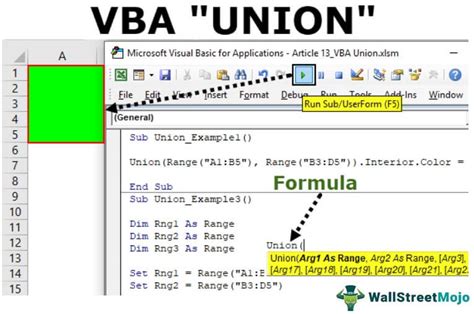
Here's an example:
Sub SelectRange()
Dim myRange As Range
Set myRange = Union(Range("A1:B2"), Range("C3:D4"))
myRange.Select
End Sub
In this example, we use the Union method to select two ranges: A1:B2 and C3:D4.
Method 5: Using the Find Method
The Find method allows you to select a range based on specific criteria, such as a value or format. This method is useful when you need to select a range that meets specific conditions.
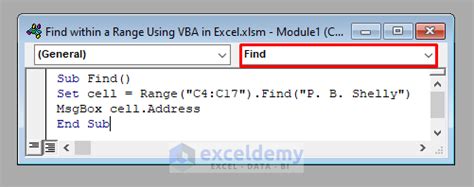
Here's an example:
Sub SelectRange()
Dim myRange As Range
Set myRange = Range("A1:B2").Find("Hello")
myRange.Select
End Sub
In this example, we use the Find method to select a range that contains the value "Hello".
Gallery of VBA Range Selection Methods
VBA Range Selection Methods
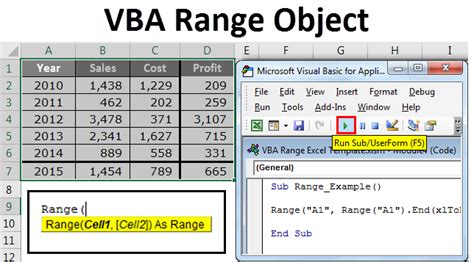
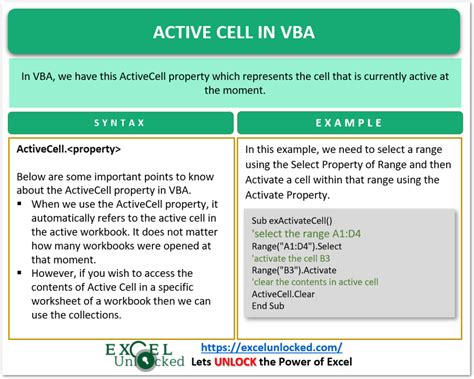
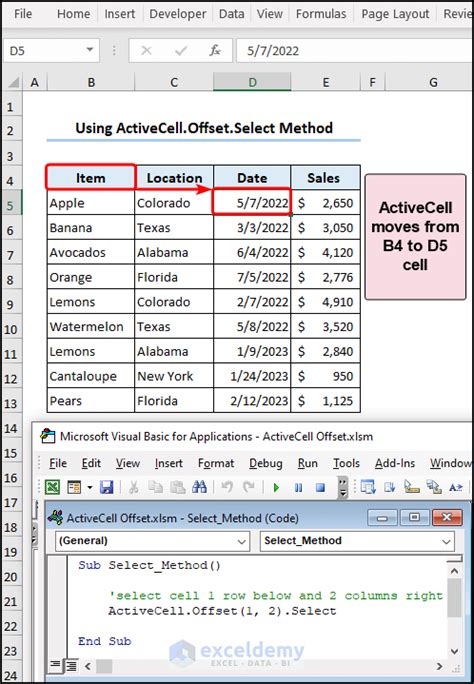
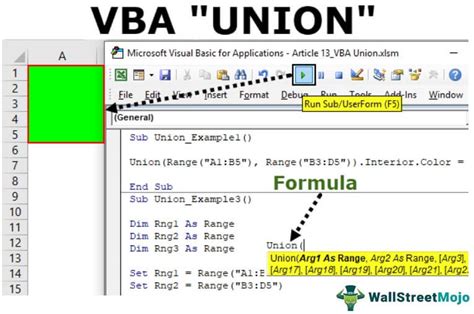
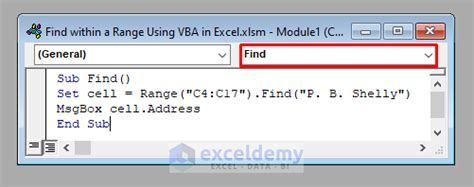
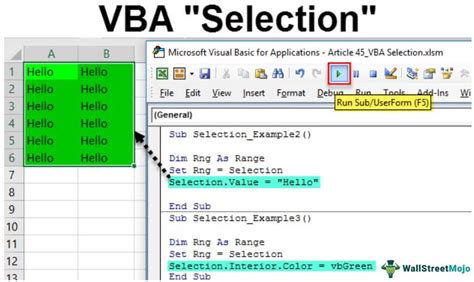
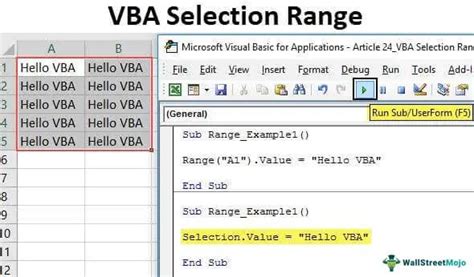
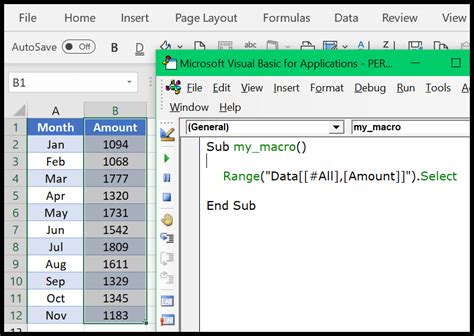
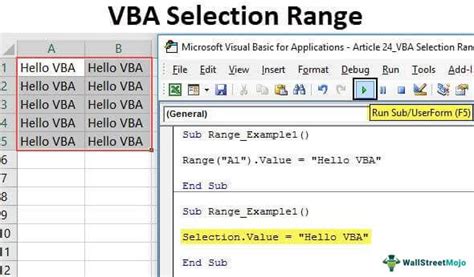
Conclusion
Selecting a range in VBA is a crucial skill that can help you automate tasks and work more efficiently in Excel. By mastering the five methods outlined in this article, you'll be able to select ranges with confidence and accuracy. Remember to always use the correct method for the task at hand, and don't hesitate to experiment with different techniques to find what works best for you.
What's your favorite method for selecting a range in VBA? Share your tips and experiences in the comments below!