Intro
Working with strings in VBA can be a daunting task, especially when it comes to splitting them into arrays. However, with the right techniques and tools, this process can be made easy and efficient. In this article, we will explore the various methods of splitting a string into an array in VBA, including using the Split
function, regular expressions, and other creative approaches.
Understanding the Basics of String Splitting in VBA
Before diving into the various methods of splitting a string into an array, it's essential to understand the basics of string manipulation in VBA. In VBA, strings are sequences of characters that can be manipulated using various functions and techniques. One of the most common string manipulation tasks is splitting a string into an array of substrings.
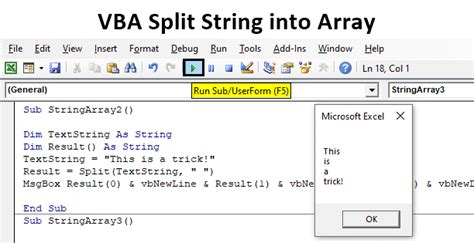
Why Split a String into an Array in VBA?
Splitting a string into an array in VBA can be useful in various scenarios, such as:
- Data processing: Splitting a string into an array can help you process data more efficiently, especially when working with large datasets.
- Text analysis: Splitting a string into an array can help you analyze text data, such as extracting specific words or phrases.
- Data visualization: Splitting a string into an array can help you visualize data more effectively, especially when working with charts and graphs.
Using the Split Function in VBA
The Split
function is one of the most commonly used methods of splitting a string into an array in VBA. This function takes two arguments: the string to be split and the delimiter used to split the string.
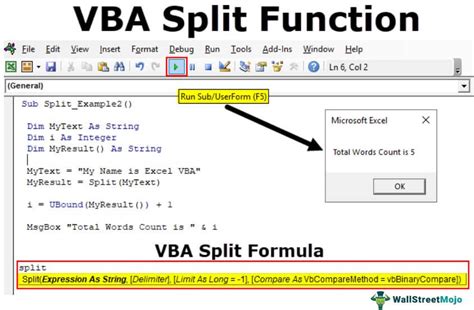
Here's an example of using the Split
function in VBA:
Dim str As String
str = "apple,banana,cherry"
Dim arr As Variant
arr = Split(str, ",")
In this example, the Split
function splits the string str
into an array arr
using the comma (,
) as the delimiter.
Using Regular Expressions in VBA
Regular expressions (regex) can also be used to split a string into an array in VBA. Regex is a powerful pattern-matching language that can be used to extract specific patterns from a string.
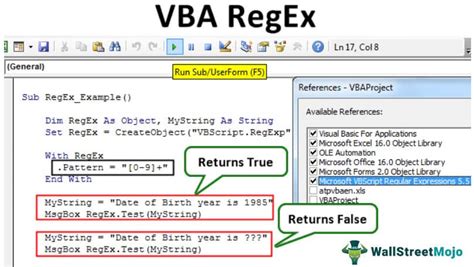
Here's an example of using regex to split a string into an array in VBA:
Dim str As String
str = "apple,banana,cherry"
Dim arr As Variant
arr = RegExp.Split(str, ",")
In this example, the RegExp
object is used to split the string str
into an array arr
using the comma (,
) as the delimiter.
Creative Approaches to Splitting a String into an Array in VBA
In addition to using the Split
function and regex, there are other creative approaches to splitting a string into an array in VBA. One such approach is using the InStr
function to find the position of the delimiter and then using the Mid
function to extract the substrings.
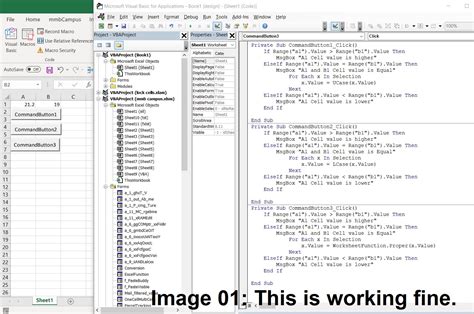
Here's an example of using the InStr
and Mid
functions to split a string into an array in VBA:
Dim str As String
str = "apple,banana,cherry"
Dim arr As Variant
Dim i As Integer
i = InStr(str, ",")
arr = Mid(str, 1, i - 1)
In this example, the InStr
function is used to find the position of the first comma (,
) in the string str
. The Mid
function is then used to extract the substring from the beginning of the string to the position of the comma.
Conclusion
Splitting a string into an array in VBA can be a challenging task, but with the right techniques and tools, it can be made easy and efficient. In this article, we explored various methods of splitting a string into an array in VBA, including using the Split
function, regex, and creative approaches. By understanding the basics of string manipulation in VBA and using the right techniques, you can efficiently split strings into arrays and perform various data processing tasks.
VBA String Splitting Gallery
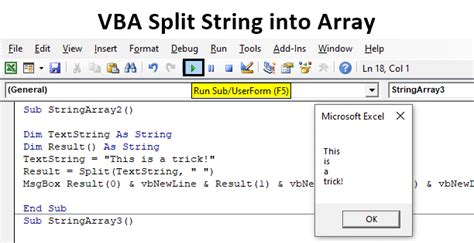
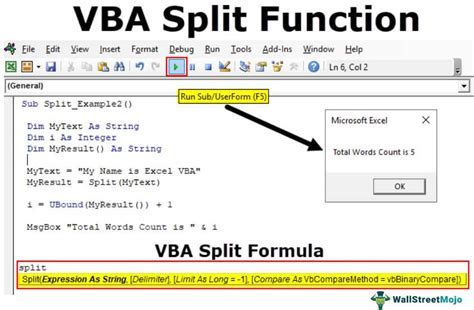
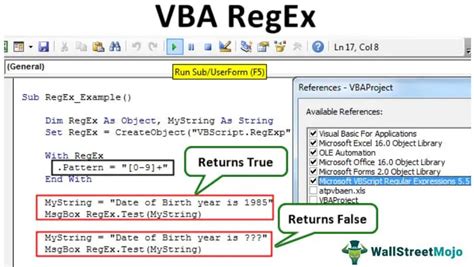
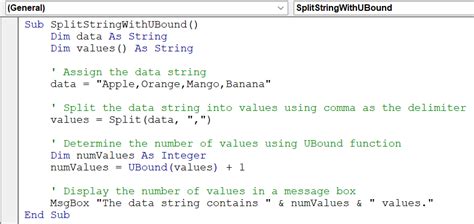
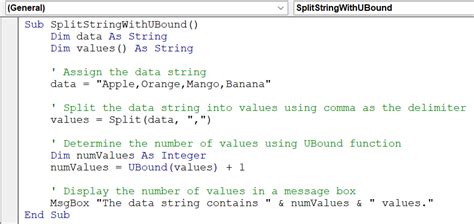
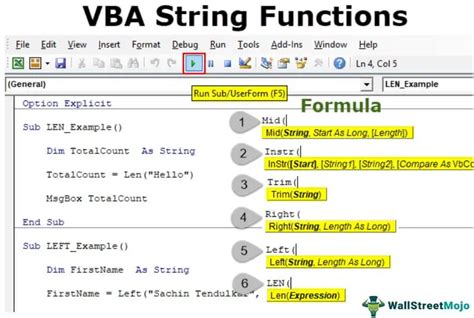
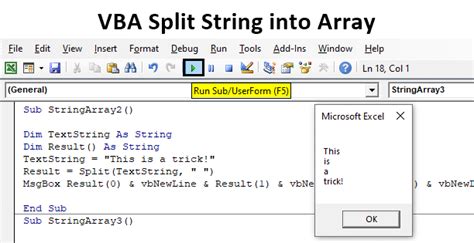
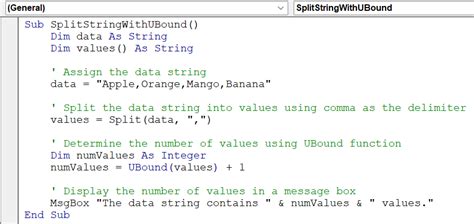
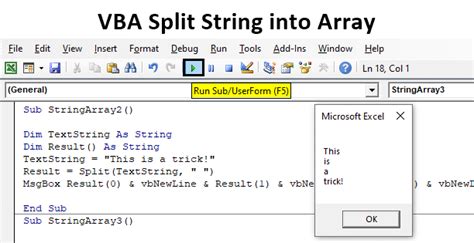
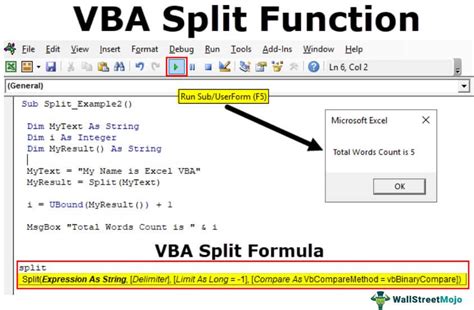
We hope this article has provided you with a comprehensive guide to splitting strings into arrays in VBA. If you have any further questions or would like to share your own experiences with string splitting in VBA, please leave a comment below!