Intro
Troubleshoot Excel macro errors with ease. Discover 5 proven methods to fix Subscript Out Of Range errors in Excel VBA, including debugging techniques, error handling, and code optimization. Learn how to resolve runtime errors, fix array indices, and improve macro performance. Get back to automating tasks with confidence.
When working with Excel macros, errors can be frustrating and disrupt your workflow. One common error is the "Subscript out of range" error, which occurs when your macro tries to access an element in an array or collection that doesn't exist. In this article, we'll explore five ways to fix this error and get your macro running smoothly.
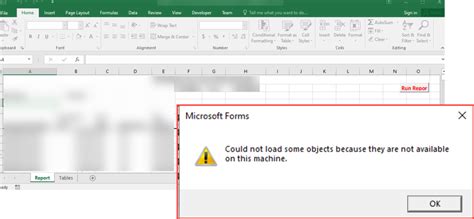
Understanding the Subscript Out of Range Error
Before we dive into the solutions, it's essential to understand what causes the "Subscript out of range" error. This error typically occurs when your macro tries to access an element in an array or collection using an index that is outside the valid range. For example, if you have an array with 10 elements, trying to access the 11th element will result in a "Subscript out of range" error.
Common Causes of the Subscript Out of Range Error
Here are some common causes of the "Subscript out of range" error:
- Incorrect array or collection indexing
- Missing or mismatched data
- Incorrect loop iteration
- Wrong data type or variable declaration
1. Check Your Array or Collection Indexing
One of the most common causes of the "Subscript out of range" error is incorrect array or collection indexing. Make sure you're using the correct index to access the desired element. Here's an example:
Dim myArray(10) As Integer
myArray(10) = 10 ' This will cause a Subscript out of range error
In this example, the array myArray
has 11 elements ( indexed from 0 to 10), but the code tries to access the 11th element, which doesn't exist.
To fix this, ensure you're using the correct index:
Dim myArray(10) As Integer
myArray(9) = 10 ' This is the correct index
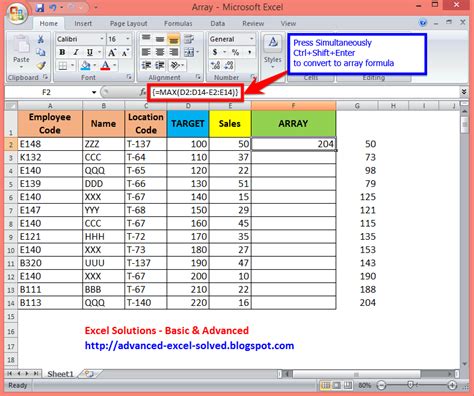
2. Verify Your Data
Missing or mismatched data can also cause the "Subscript out of range" error. Ensure that your data is complete and correctly formatted. Here's an example:
Dim myArray(10) As Integer
For i = 1 To 11
myArray(i) = i ' This will cause a Subscript out of range error
Next i
In this example, the loop tries to access the 11th element of the array, but it only has 10 elements.
To fix this, ensure your data is correctly formatted and complete:
Dim myArray(10) As Integer
For i = 0 To 9
myArray(i) = i ' This is the correct loop iteration
Next i
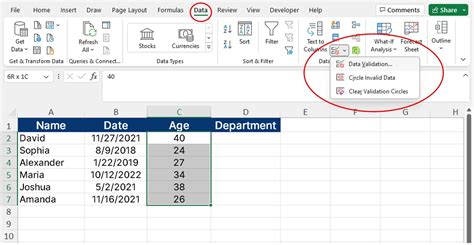
3. Use Correct Loop Iteration
Incorrect loop iteration can also cause the "Subscript out of range" error. Ensure that your loop iterates over the correct range. Here's an example:
Dim myArray(10) As Integer
For i = 1 To 10
myArray(i) = i ' This is correct, but the loop can be improved
Next i
In this example, the loop iterates over the correct range, but it can be improved:
Dim myArray(10) As Integer
For i = LBound(myArray) To UBound(myArray)
myArray(i) = i ' This is the correct loop iteration
Next i
In this improved example, the loop uses the LBound
and UBound
functions to iterate over the correct range of the array.
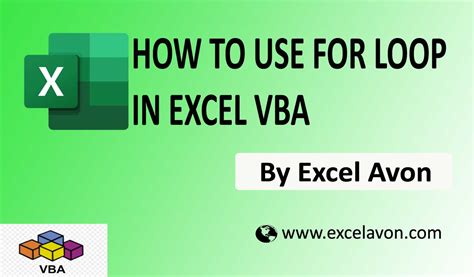
4. Check Your Variable Declarations
Incorrect variable declarations can also cause the "Subscript out of range" error. Ensure that your variables are declared correctly. Here's an example:
Dim myArray As Variant
myArray = Array(1, 2, 3)
myArray(3) = 4 ' This will cause a Subscript out of range error
In this example, the variable myArray
is declared as a Variant
, but it's used as an array.
To fix this, ensure your variables are declared correctly:
Dim myArray(2) As Integer
myArray(0) = 1
myArray(1) = 2
myArray(2) = 3
In this corrected example, the variable myArray
is declared as an array with 3 elements.
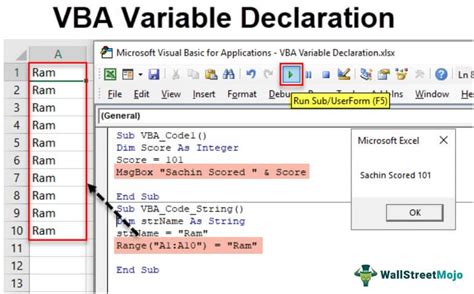
5. Use Error Handling
Finally, use error handling to catch and handle the "Subscript out of range" error. Here's an example:
On Error Resume Next
Dim myArray(10) As Integer
myArray(10) = 10
If Err.Number <> 0 Then
MsgBox "Error: " & Err.Description
End If
In this example, the On Error Resume Next
statement is used to catch and handle any errors that occur. If an error occurs, the code displays a message box with the error description.
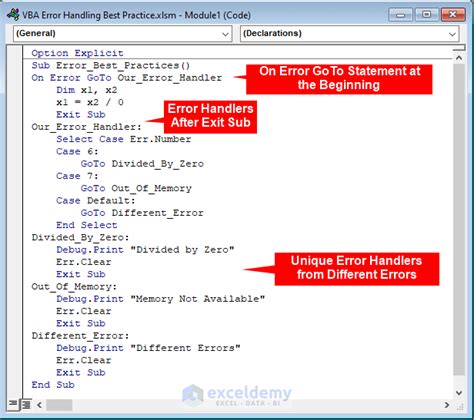
Excel Macro Errors Gallery
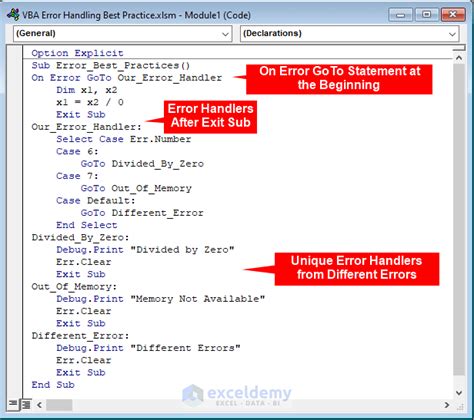
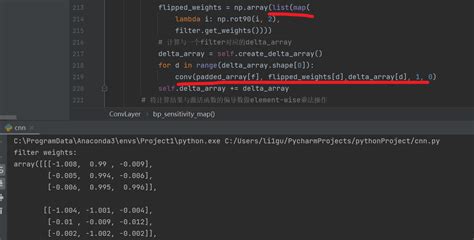
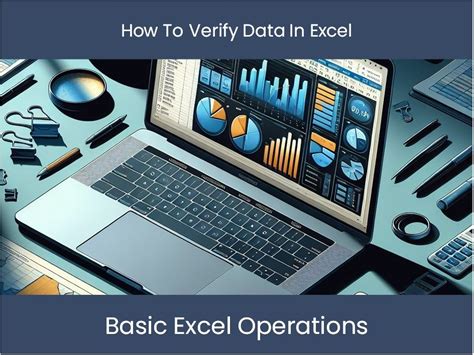
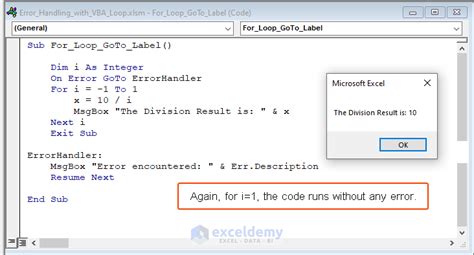
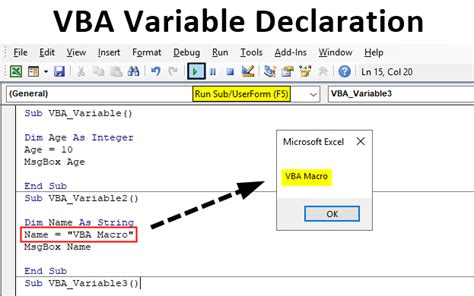
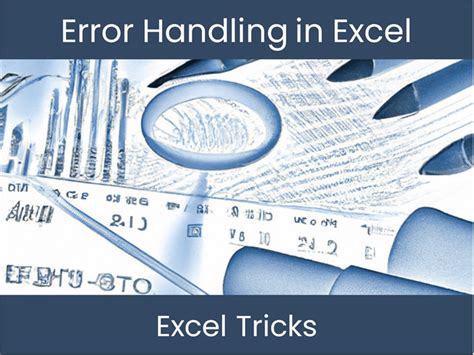
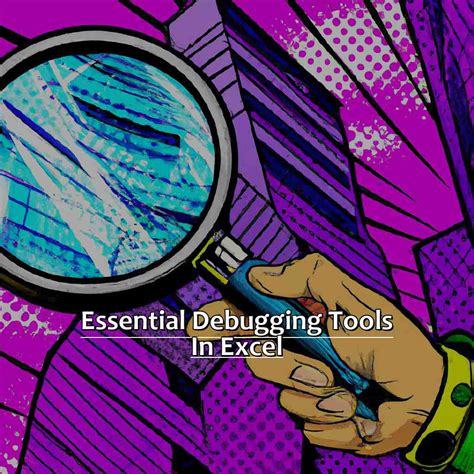
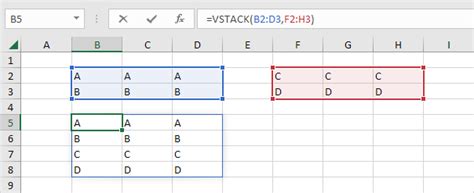
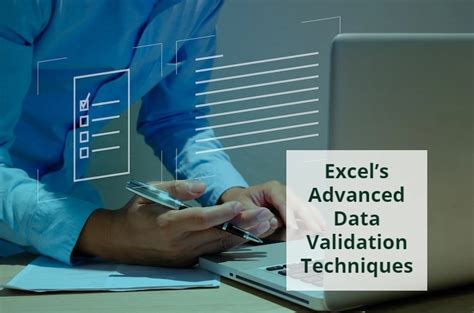
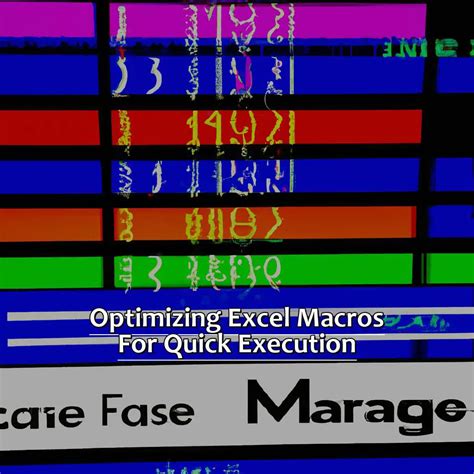
If you've reached this point, you should have a good understanding of how to fix the "Subscript out of range" error in your Excel macros. Remember to check your array or collection indexing, verify your data, use correct loop iteration, check your variable declarations, and use error handling to catch and handle any errors that occur. By following these tips, you'll be able to write more robust and reliable Excel macros.