Intro
Master VBA with 5 simple methods to check if a cell is empty. Learn how to use IsEmpty, IsNull, IF statements, and more to efficiently handle blank cells in your Excel spreadsheets. Improve your VBA skills with these easy-to-implement techniques and optimize your code for seamless data processing.
Understanding the Importance of Checking Empty Cells in VBA
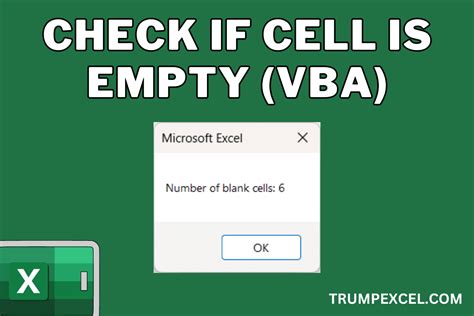
When working with Excel and VBA, it's not uncommon to encounter empty cells. These cells can cause issues with your code, leading to errors or unexpected behavior. To avoid these problems, it's crucial to check if a cell is empty before attempting to manipulate its contents. In this article, we'll explore five ways to check if a cell is empty in VBA.
The Need for Empty Cell Checks
Before diving into the methods, let's discuss why checking for empty cells is essential. When a cell is empty, it can cause problems with:
- Formula calculations
- Data analysis
- Conditional statements
- Error handling
By checking for empty cells, you can prevent these issues and ensure your code runs smoothly.
Method 1: Using the `IsEmpty` Function
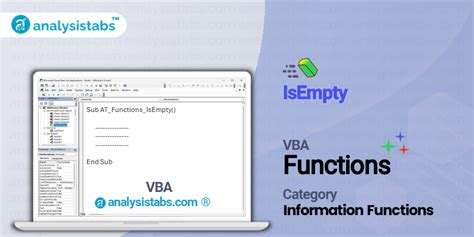
The IsEmpty
function is a straightforward way to check if a cell is empty. Here's an example:
If IsEmpty(Range("A1").Value) Then
MsgBox "Cell A1 is empty"
End If
This code checks if the value in cell A1 is empty. If it is, the message box will display "Cell A1 is empty".
Method 2: Using the `""` Operator
Another way to check for empty cells is to use the ""
operator. This method is useful when working with ranges:
If Range("A1").Value = "" Then
MsgBox "Cell A1 is empty"
End If
This code checks if the value in cell A1 is equal to an empty string. If it is, the message box will display "Cell A1 is empty".
Method 3: Using the `Len` Function
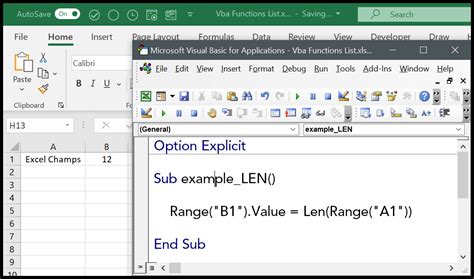
The Len
function returns the length of a string. If the length is 0, the cell is empty:
If Len(Range("A1").Value) = 0 Then
MsgBox "Cell A1 is empty"
End If
This code checks the length of the value in cell A1. If it's 0, the message box will display "Cell A1 is empty".
Method 4: Using the `CountA` Function
The CountA
function counts the number of cells in a range that contain text. If the count is 0, the cell is empty:
If Application.WorksheetFunction.CountA(Range("A1")) = 0 Then
MsgBox "Cell A1 is empty"
End If
This code uses the CountA
function to count the number of cells in range A1 that contain text. If the count is 0, the message box will display "Cell A1 is empty".
Method 5: Using the `Cells.Find` Method
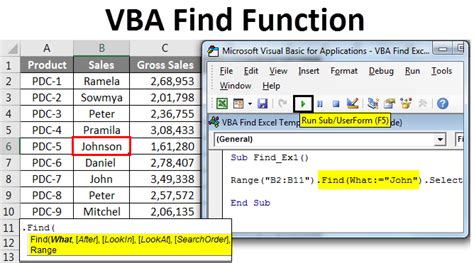
The Cells.Find
method searches for a value in a range. If the value is not found, the cell is empty:
If Range("A1").Find("") Is Nothing Then
MsgBox "Cell A1 is empty"
End If
This code uses the Cells.Find
method to search for an empty string in cell A1. If the value is not found, the message box will display "Cell A1 is empty".
Conclusion: Choosing the Right Method
When checking for empty cells in VBA, it's essential to choose the right method for your specific use case. The IsEmpty
function is a straightforward way to check for empty cells, while the ""
operator and Len
function provide alternative methods. The CountA
function and Cells.Find
method offer more advanced ways to check for empty cells.
VBA Empty Cell Check Gallery
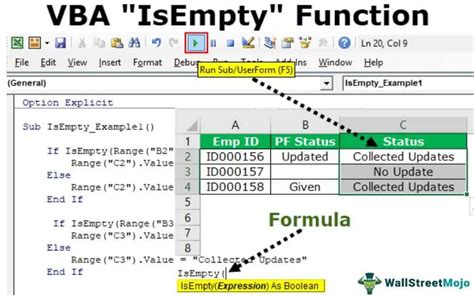
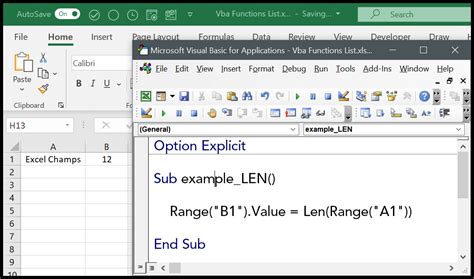
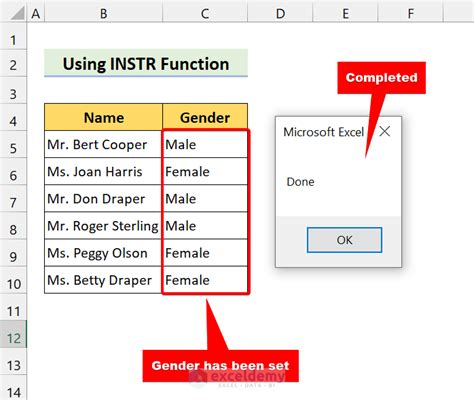
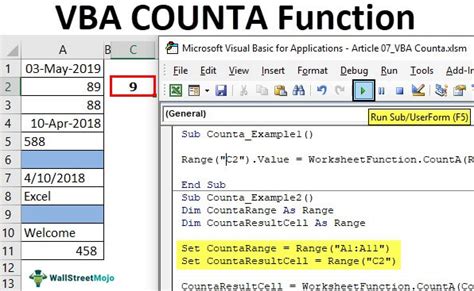
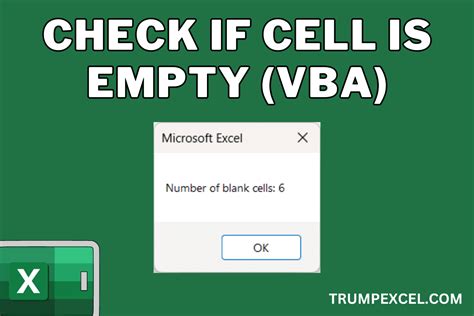
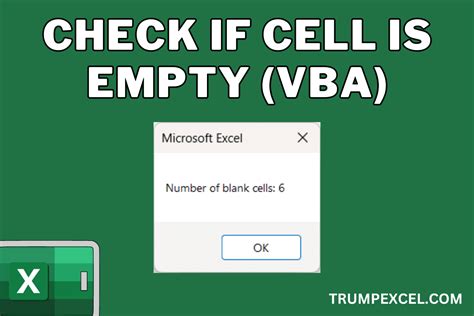
We hope this article has helped you understand the importance of checking for empty cells in VBA and provided you with the necessary tools to do so. If you have any questions or need further assistance, please don't hesitate to ask in the comments below.