When working with directories in VBA, it's essential to ensure that the directory exists before attempting to access or manipulate it. Failure to do so can lead to errors and crashes. In this article, we'll explore the importance of checking if a directory exists in VBA, and provide a simplified approach to error handling.
The Importance of Checking Directory Existence
When working with files and directories in VBA, it's crucial to verify that the directory exists before attempting to perform any operations. This is because VBA will throw an error if it tries to access a non-existent directory. By checking if the directory exists, you can avoid errors and ensure that your code runs smoothly.
Traditional Approach to Checking Directory Existence
The traditional approach to checking directory existence in VBA involves using the Dir
function. This function returns a string representing the name of the directory or file. If the directory does not exist, it returns an empty string. Here's an example of how to use the Dir
function to check if a directory exists:
Sub CheckDirectoryExistence()
Dim directoryPath As String
directoryPath = "C:\Example\Directory"
If Dir(directoryPath, vbDirectory) <> "" Then
MsgBox "Directory exists"
Else
MsgBox "Directory does not exist"
End If
End Sub
Simplified Approach to Error Handling
While the traditional approach works, it can be cumbersome to write and maintain. A simplified approach to error handling involves using the FileSystemObject
(FSO) library. The FSO library provides a more intuitive and efficient way to interact with the file system.
To use the FSO library, you need to set a reference to it in your VBA project. Here's how:
- Open the Visual Basic Editor (VBE) by pressing
Alt + F11
or navigating toDeveloper
>Visual Basic
in the ribbon. - In the VBE, click
Tools
>References
in the menu. - In the References dialog box, scroll down and check the box next to
Microsoft Scripting Runtime
. - Click
OK
to close the dialog box.
Once you've set the reference, you can use the FileSystemObject
to check if a directory exists. Here's an example:
Sub CheckDirectoryExistence()
Dim fso As FileSystemObject
Set fso = New FileSystemObject
Dim directoryPath As String
directoryPath = "C:\Example\Directory"
If fso.FolderExists(directoryPath) Then
MsgBox "Directory exists"
Else
MsgBox "Directory does not exist"
End If
Set fso = Nothing
End Sub
In this example, we create a new instance of the FileSystemObject
and use its FolderExists
method to check if the directory exists.
Benefits of Using the FSO Library
Using the FSO library provides several benefits, including:
- Simplified error handling: The FSO library provides a more intuitive and efficient way to interact with the file system, making it easier to handle errors.
- Improved performance: The FSO library is optimized for performance, making it faster than traditional approaches.
- Increased flexibility: The FSO library provides a wide range of methods and properties for interacting with the file system, making it more flexible than traditional approaches.
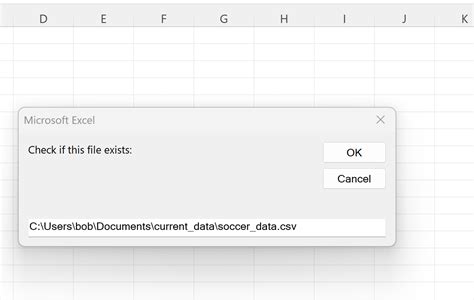
Conclusion
Checking if a directory exists is an essential step in VBA programming. By using the FileSystemObject
library, you can simplify error handling and improve performance. Remember to set a reference to the FSO library in your VBA project and use its FolderExists
method to check if a directory exists.
Gallery of VBA Directory Existence
VBA Directory Existence Image Gallery
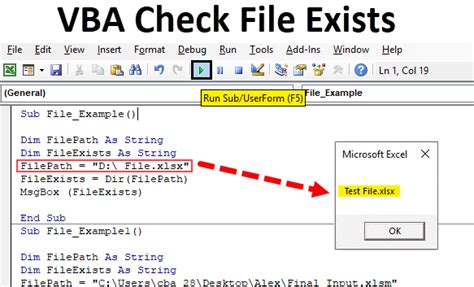
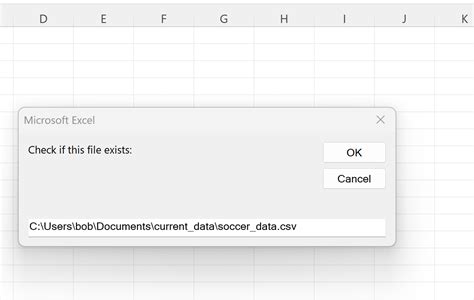
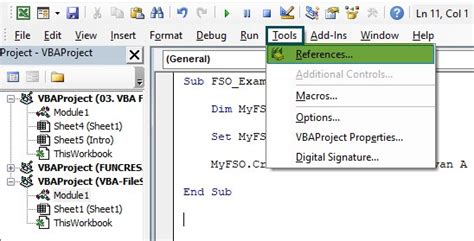
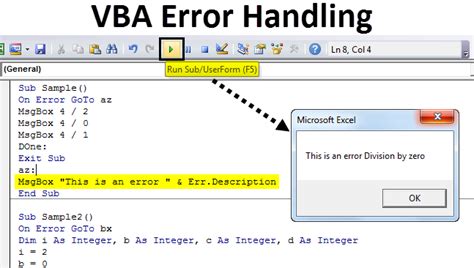
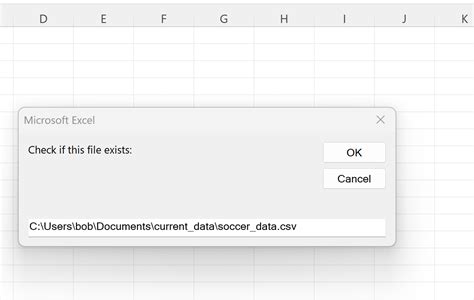
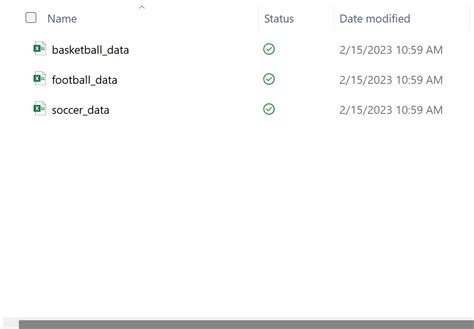
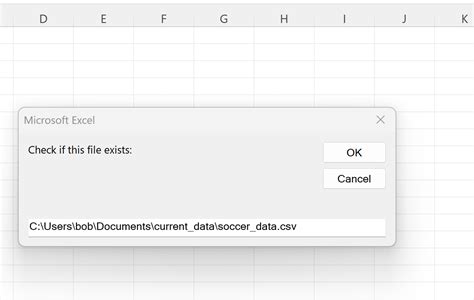
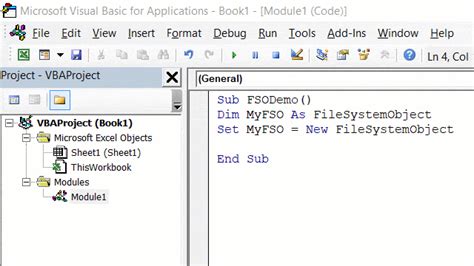
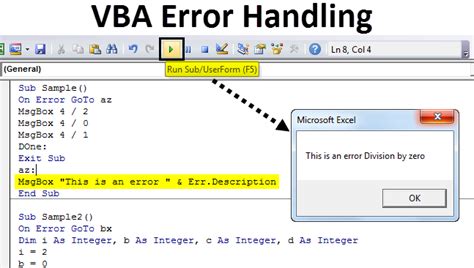
We hope this article has helped you understand the importance of checking if a directory exists in VBA and provided a simplified approach to error handling using the FileSystemObject
library. If you have any questions or need further assistance, please don't hesitate to ask.