Intro
Discover the simplicity of VBA copy paste values with our expert guide. Master code examples to efficiently paste values in Excel, overcoming common errors and pitfalls. Learn to harness the power of VBA to streamline your workflows, improve data management, and boost productivity with our easy-to-follow tutorials and code snippets.
If you're a frequent user of Microsoft Excel, you've probably found yourself needing to copy and paste values from one cell or range to another. While this can be done manually, it can be a tedious and time-consuming process, especially when dealing with large datasets. Fortunately, VBA (Visual Basic for Applications) provides a powerful way to automate this process with ease.
In this article, we'll explore the world of VBA copy paste values, including the benefits, working mechanisms, and step-by-step code examples to get you started. By the end of this article, you'll be equipped with the knowledge and skills to streamline your workflow and boost productivity.
Why Use VBA Copy Paste Values?
Before we dive into the code examples, let's take a look at why using VBA copy paste values is a good idea:
- Efficiency: VBA allows you to automate repetitive tasks, freeing up your time for more strategic and creative work.
- Accuracy: By using code, you can minimize the risk of human error and ensure that your data is copied and pasted correctly.
- Speed: VBA can process large datasets much faster than manual copying and pasting.
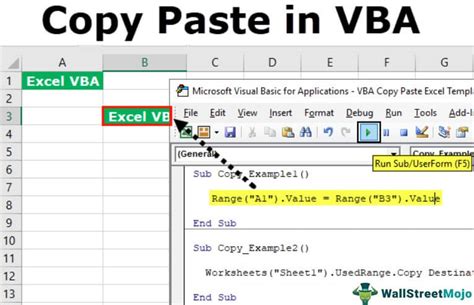
Basic VBA Copy Paste Values Code
To get started with VBA copy paste values, you'll need to open the Visual Basic Editor in Excel. To do this, press Alt + F11
or navigate to Developer > Visual Basic
in the ribbon.
Once you're in the Visual Basic Editor, create a new module by clicking Insert > Module
or press Alt + F11
again. Then, paste the following code into the module:
Sub CopyPasteValues()
Range("A1").Copy
Range("B1").PasteSpecial xlPasteValues
Application.CutCopyMode = False
End Sub
This code copies the value in cell A1
and pastes it into cell B1
. The xlPasteValues
argument specifies that we only want to paste the values, without formatting or formulas.
Copying Multiple Cells
To copy multiple cells, you can modify the code to use a range instead of a single cell:
Sub CopyPasteValuesMultipleCells()
Range("A1:B2").Copy
Range("C1:D2").PasteSpecial xlPasteValues
Application.CutCopyMode = False
End Sub
This code copies the values in the range A1:B2
and pastes them into the range C1:D2
.
Looping Through Cells
In many cases, you'll need to copy and paste values from multiple cells in a loop. Here's an example code snippet that demonstrates how to do this:
Sub CopyPasteValuesLoop()
Dim i As Long
For i = 1 To 10
Range("A" & i).Copy
Range("B" & i).PasteSpecial xlPasteValues
Next i
Application.CutCopyMode = False
End Sub
This code loops through cells A1:A10
and copies the values to cells B1:B10
.
Error Handling
When working with VBA copy paste values, it's essential to include error handling to ensure that your code runs smoothly and doesn't crash in case of errors. Here's an updated version of the previous code snippet with error handling:
Sub CopyPasteValuesLoopWithErrorHandling()
On Error GoTo ErrorHandler
Dim i As Long
For i = 1 To 10
Range("A" & i).Copy
Range("B" & i).PasteSpecial xlPasteValues
Next i
Application.CutCopyMode = False
Exit Sub
ErrorHandler:
MsgBox "Error " & Err.Number & ": " & Err.Description
End Sub
This code includes a basic error handling mechanism that displays a message box with the error number and description if an error occurs.
Conclusion
VBA copy paste values is a powerful technique that can help you streamline your workflow and boost productivity in Excel. With the code examples provided in this article, you should be able to automate many of your copy and paste tasks with ease.
Remember to always include error handling in your code to ensure that it runs smoothly and doesn't crash in case of errors. Happy coding!
VBA Copy Paste Values Code Examples
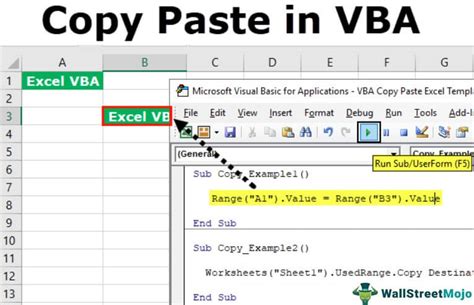
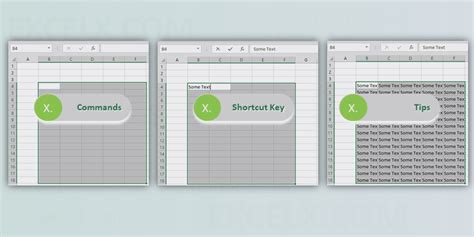
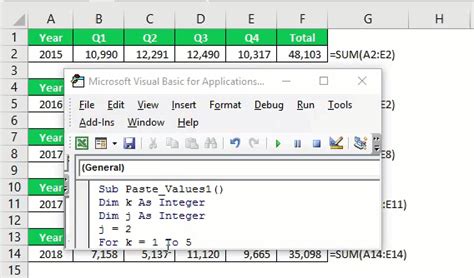
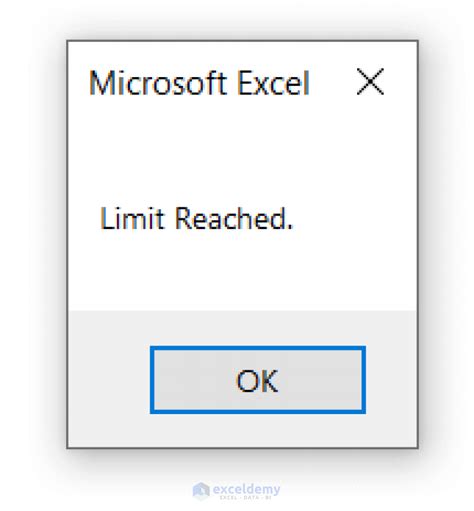
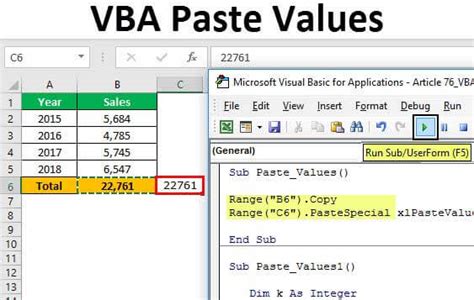
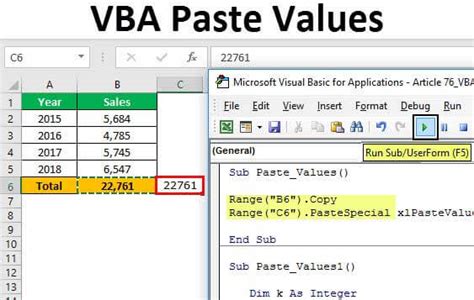