Intro
Discover how to simplify date calculations in Excel using VBA Day of Week functions. Master the art of determining weekdays, weekends, and specific days of the week with ease. Learn VBA code snippets and examples to automate date-related tasks, boost productivity, and streamline your workflow. Unleash the power of VBA to work smarter, not harder.
Calculating the day of the week for a given date can be a daunting task, especially when working with dates in different formats. However, with VBA (Visual Basic for Applications), you can simplify date calculations with ease. In this article, we will explore the various ways to calculate the day of the week using VBA, including the built-in functions and custom formulas.
Understanding the Weekday Function
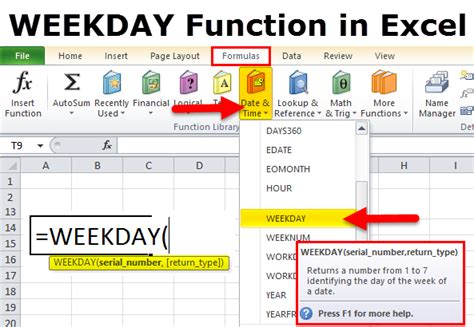
The Weekday function in VBA returns the day of the week for a given date. The function takes two arguments: the date and the first day of the week. The first day of the week can be either Sunday (1) or Monday (2). The function returns a number between 1 and 7, where 1 represents Sunday and 7 represents Saturday.
Using the Weekday Function
Here's an example of how to use the Weekday function:
Sub CalculateDayOfWeek()
Dim myDate As Date
myDate = #2/15/2022#
MsgBox Weekday(myDate) ' Returns 3, which represents Tuesday
End Sub
In this example, the Weekday function returns the day of the week for the date February 15, 2022.
Custom Formulas for Calculating the Day of the Week
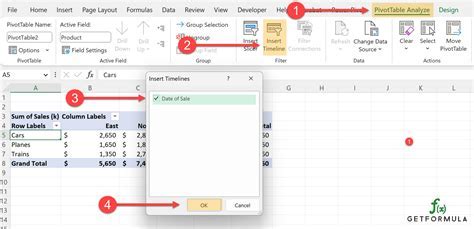
If you prefer not to use the built-in Weekday function, you can create a custom formula to calculate the day of the week. One way to do this is by using the following formula:
DayOfWeek = (Date - Int(Date)) * 7 + 2
This formula works by subtracting the integer part of the date from the date itself, multiplying the result by 7, and then adding 2. The result is a number between 1 and 7, where 1 represents Sunday and 7 represents Saturday.
Example Usage of Custom Formula
Here's an example of how to use the custom formula:
Sub CalculateDayOfWeekCustom()
Dim myDate As Date
myDate = #2/15/2022#
Dim DayOfWeek As Integer
DayOfWeek = (myDate - Int(myDate)) * 7 + 2
MsgBox DayOfWeek ' Returns 3, which represents Tuesday
End Sub
In this example, the custom formula returns the day of the week for the date February 15, 2022.
Using VBA to Determine the Day of the Week for a Range of Dates
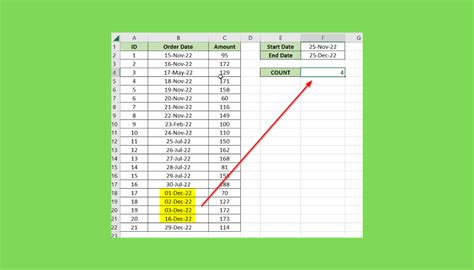
If you need to determine the day of the week for a range of dates, you can use VBA to loop through the dates and calculate the day of the week for each date.
Example Code for Determining Day of the Week for a Range of Dates
Here's an example of how to use VBA to determine the day of the week for a range of dates:
Sub CalculateDayOfWeekForDateRange()
Dim startDate As Date
Dim endDate As Date
Dim currentDate As Date
Dim DayOfWeek As Integer
startDate = #1/1/2022#
endDate = #12/31/2022#
For currentDate = startDate To endDate
DayOfWeek = Weekday(currentDate)
' Do something with the day of the week
MsgBox "The day of the week for " & currentDate & " is " & DayOfWeek
Next currentDate
End Sub
In this example, the VBA code loops through the dates from January 1, 2022, to December 31, 2022, and calculates the day of the week for each date using the Weekday function.
Gallery of VBA Date Calculations
VBA Date Calculations
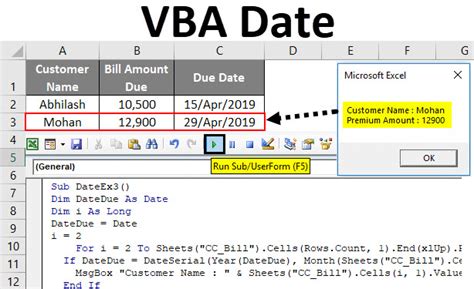
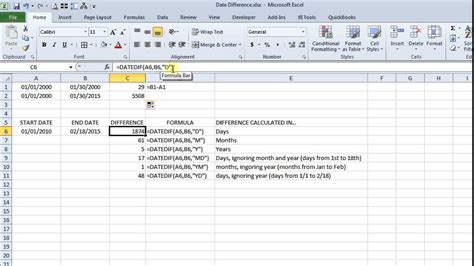
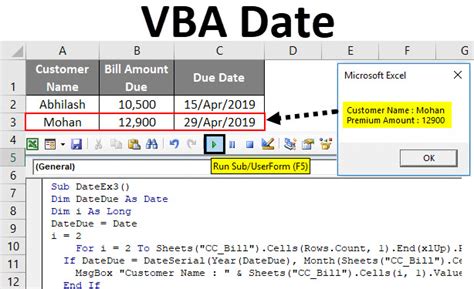
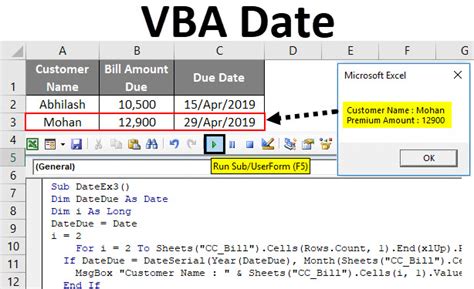
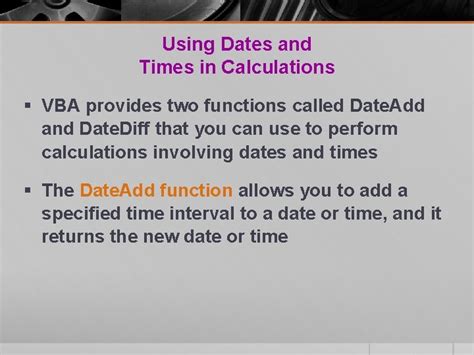
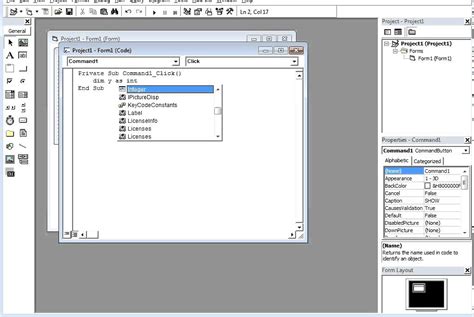
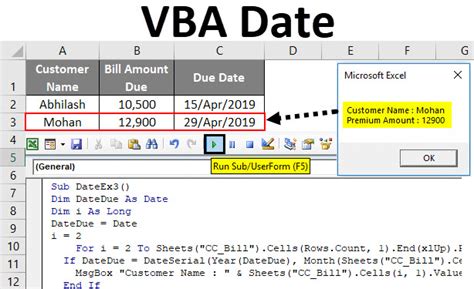
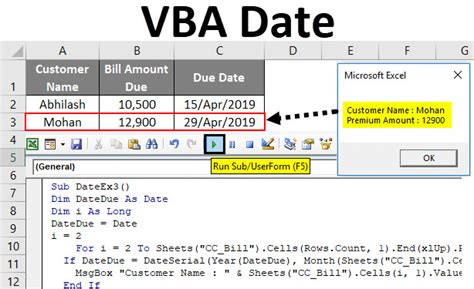
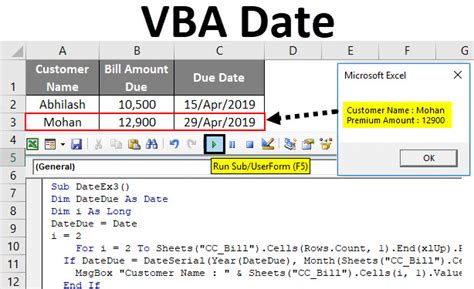
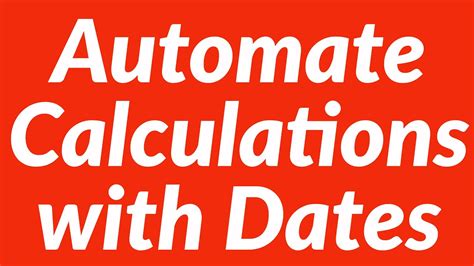
We hope this article has helped you understand the different ways to calculate the day of the week using VBA. Whether you prefer to use the built-in Weekday function or create a custom formula, VBA provides a powerful and flexible way to work with dates and perform calculations. If you have any questions or need further assistance, please don't hesitate to ask.