File management is an essential aspect of any programming task, and Visual Basic for Applications (VBA) is no exception. When working with files in VBA, there may come a time when you need to delete a file. Deleting a file in VBA can be accomplished in several ways, each with its own set of benefits and considerations. In this article, we will explore three ways to delete a file in VBA.
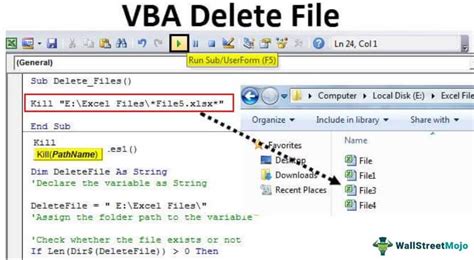
Method 1: Using the Kill Statement
The Kill statement is a straightforward method for deleting files in VBA. This statement is used to delete a file or a group of files. The syntax for the Kill statement is as follows:
Kill pathname
In this syntax, pathname
is the path and name of the file or files to be deleted. You can specify multiple files by separating them with commas.
For example:
Kill "C:\Users\Username\Documents\file.txt"
This code will delete the file file.txt
located in the Documents
folder of the user Username
.
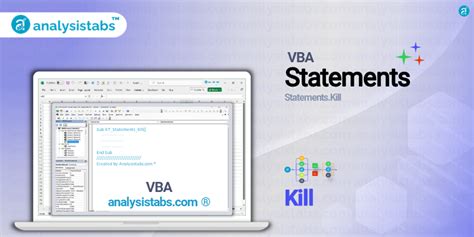
Benefits and Considerations
The Kill statement is a simple and efficient way to delete files in VBA. However, it does not provide any error handling or confirmation before deleting the file. This means that if the file does not exist or cannot be deleted, the code will raise an error.
Method 2: Using the FileSystemObject
Another way to delete a file in VBA is by using the FileSystemObject
. This object provides a more robust and flexible way to interact with the file system. To use the FileSystemObject
, you need to set a reference to the Microsoft Scripting Runtime
library.
The syntax for deleting a file using the FileSystemObject
is as follows:
Dim fso As New FileSystemObject
fso.DeleteFile pathname
In this syntax, pathname
is the path and name of the file to be deleted.
For example:
Dim fso As New FileSystemObject
fso.DeleteFile "C:\Users\Username\Documents\file.txt"
This code will delete the file file.txt
located in the Documents
folder of the user Username
.
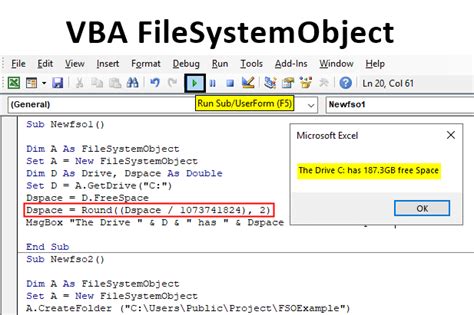
Benefits and Considerations
The FileSystemObject
provides more flexibility and control over the file deletion process. It also provides error handling and confirmation before deleting the file. However, it requires setting a reference to the Microsoft Scripting Runtime
library, which may not be desirable in all situations.
Method 3: Using the WScript.Shell Object
The WScript.Shell
object is another way to delete a file in VBA. This object provides a way to interact with the Windows shell and execute shell commands. To use the WScript.Shell
object, you need to set a reference to the Microsoft Shell Controls and Automation
library.
The syntax for deleting a file using the WScript.Shell
object is as follows:
Dim shell As New WScript.Shell
shell.Run "cmd /c del " & pathname
In this syntax, pathname
is the path and name of the file to be deleted.
For example:
Dim shell As New WScript.Shell
shell.Run "cmd /c del C:\Users\Username\Documents\file.txt"
This code will delete the file file.txt
located in the Documents
folder of the user Username
.
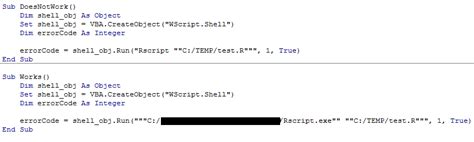
Benefits and Considerations
The WScript.Shell
object provides a way to execute shell commands, which can be useful in certain situations. However, it requires setting a reference to the Microsoft Shell Controls and Automation
library, which may not be desirable in all situations. Additionally, this method may not provide the same level of control and flexibility as the other two methods.
VBA File Deletion Gallery
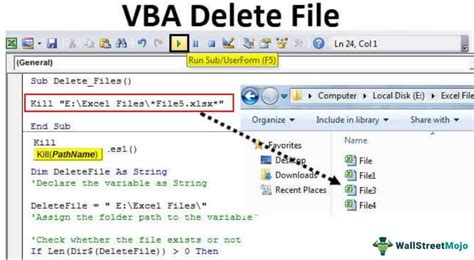
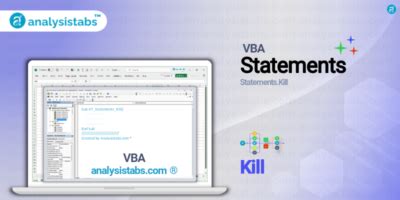
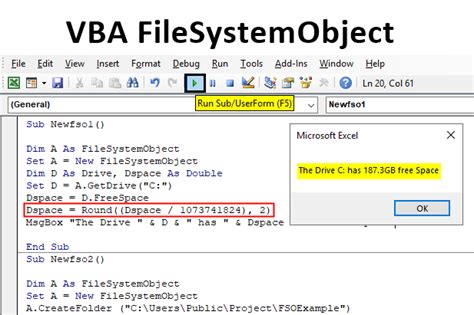
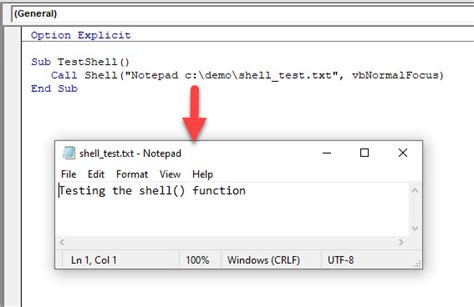
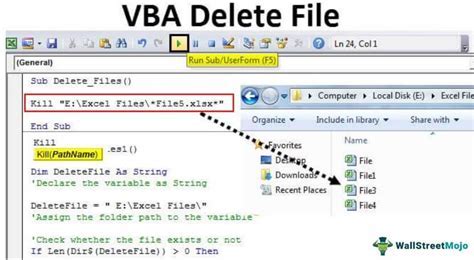
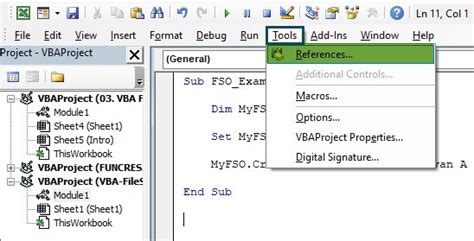
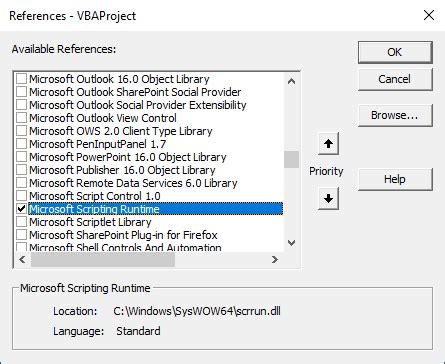
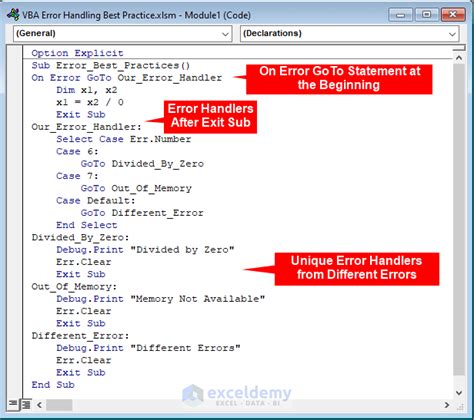
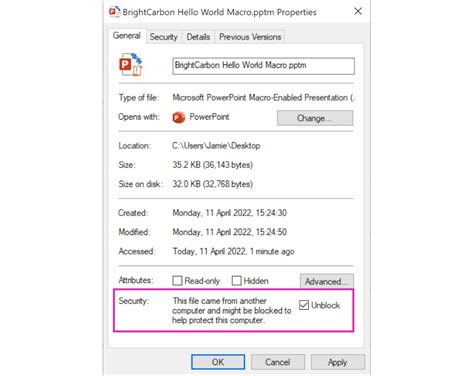
In conclusion, deleting a file in VBA can be accomplished in several ways, each with its own set of benefits and considerations. The choice of method depends on the specific requirements of your project and your personal preference. Whether you choose to use the Kill statement, the FileSystemObject, or the WScript.Shell object, make sure to follow best practices for file deletion and error handling.
We hope this article has provided you with the information you need to delete files in VBA with confidence. If you have any questions or comments, please don't hesitate to ask.