Intro
Master Excel VBA with our expert guide on setting column width. Discover 5 efficient ways to adjust column width in VBA Excel, including using Range objects, ColumnDataRange, and more. Improve your spreadsheet skills with these practical tips and tricks, perfect for Excel VBA beginners and pros alike.
Working with Excel and VBA can be a powerful combination for automating tasks and customizing your spreadsheets. One common task you might encounter is adjusting the column width to better suit the data you're working with. Here's how you can set column width in VBA Excel.
Adjusting column widths is crucial for ensuring that your data is displayed clearly and that your spreadsheet looks organized. Excel provides several methods to achieve this, ranging from manual adjustments to more complex VBA coding solutions. Here, we'll explore five ways to set column width in VBA Excel, covering both basic and more advanced techniques.
Understanding Column Width in Excel
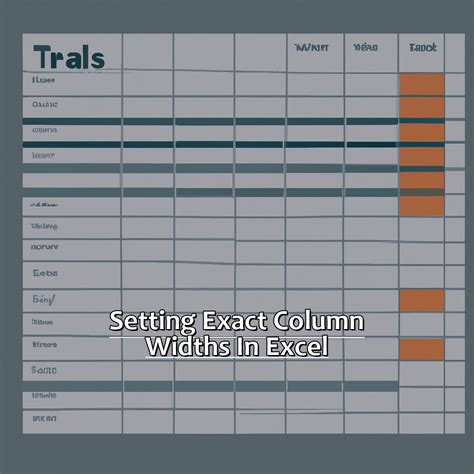
Before diving into VBA solutions, it's essential to understand how column width works in Excel. The width of a column is measured in points, with the default width being around 8.43 points for a standard font. Adjusting this width can significantly impact the readability of your spreadsheet.
Method 1: Using the `ColumnWidth` Property
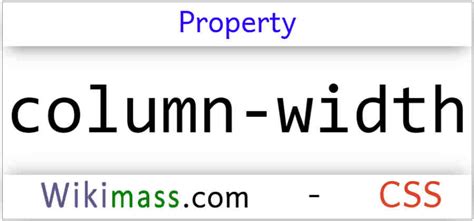
The most straightforward way to set column width in VBA is by using the ColumnWidth
property. This property can be applied to a Range
object or a Worksheet
object. For example, to set the width of column A to 10 points, you can use the following code:
Columns("A").ColumnWidth = 10
This method is direct and easy to implement, making it a favorite among VBA developers.
Adjusting Multiple Columns at Once
If you need to adjust the width of multiple columns simultaneously, you can specify a range of columns. For instance, to set the width of columns A to C, you would use:
Range("A:C").ColumnWidth = 10
This approach saves time and code when dealing with multiple columns.
Method 2: Using the `AutoFit` Method
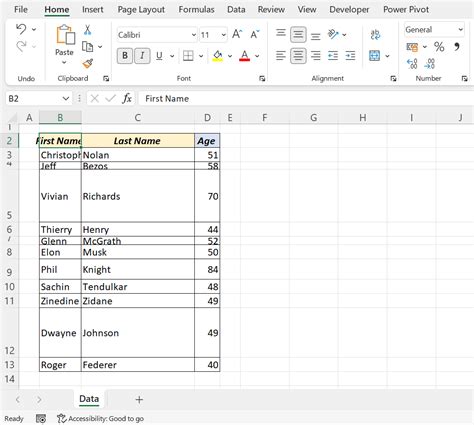
Sometimes, instead of setting a specific width, you might want to automatically adjust the column width based on the contents. The AutoFit
method does exactly that:
Columns("A").AutoFit
This code adjusts the width of column A to fit the contents of the cells in that column. Note that AutoFit
can be slower than setting a specific width, especially for large datasets.
Adjusting Column Width Based on Header or Contents
You can also specify whether to AutoFit
based on the headers or the entire contents of the column:
Columns("A").AutoFit ' Adjusts based on contents
Columns("A").AutoFit (-1) ' Adjusts based on header
The -1
argument tells VBA to only consider the header when calculating the width.
Method 3: Using a Loop to Adjust Widths
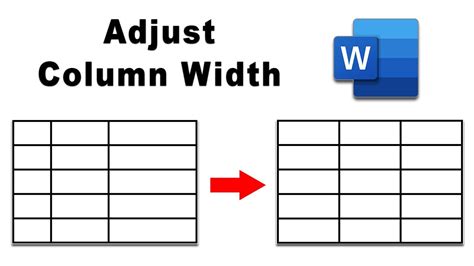
If you have a list of specific column widths you want to apply to different columns, a loop can be an efficient way to do this:
Dim columnWidths As Variant
columnWidths = Array(10, 15, 20) ' Widths for columns A, B, C
For i = 1 To UBound(columnWidths) + 1
Columns(i).ColumnWidth = columnWidths(i - 1)
Next i
This approach allows for flexibility when you have varying widths for different columns.
Method 4: Dynamically Adjusting Width Based on Data
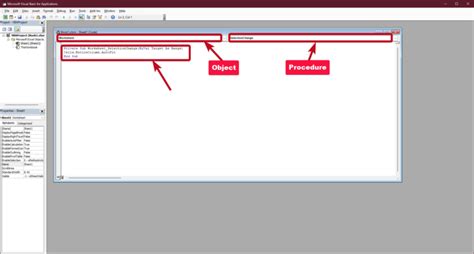
You might need to adjust column widths based on the maximum length of data in a column. Here's how you can achieve this dynamically:
Sub AdjustWidths Dynamically()
Dim ws As Worksheet
Set ws = ThisWorkbook.Sheets("Sheet1")
Dim lastRow As Long
lastRow = ws.Cells(ws.Rows.Count, "A").End(xlUp).Row
Dim col As Long
For col = 1 To 3 ' Adjust for columns A to C
Dim maxLength As Long
maxLength = 0
For i = 1 To lastRow
If Len(ws.Cells(i, col).Value) > maxLength Then
maxLength = Len(ws.Cells(i, col).Value)
End If
Next i
' Add 2 for padding
ws.Columns(col).ColumnWidth = maxLength + 2
Next col
End Sub
This code calculates the maximum length of data in each column and adjusts the width accordingly.
Method 5: Adjusting Width for All Columns in a Worksheet
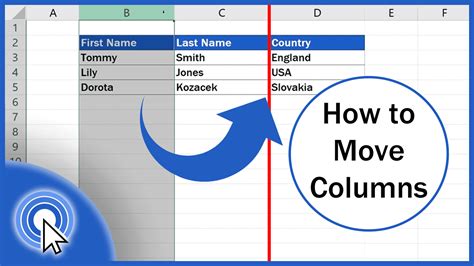
Finally, if you want to apply a uniform width to all columns in a worksheet, you can use the Cells
property to cover the entire range:
Cells.Columns.ColumnWidth = 12
This method ensures consistency across your spreadsheet.
Gallery of VBA Excel Column Width Adjustments
VBA Excel Column Width Adjustments Gallery
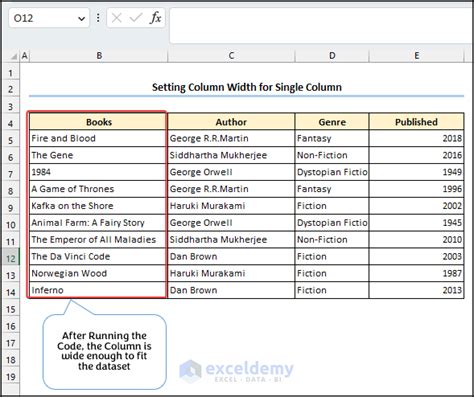
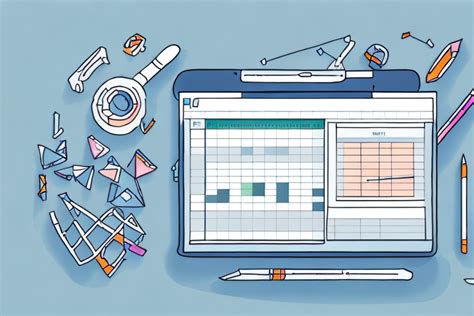
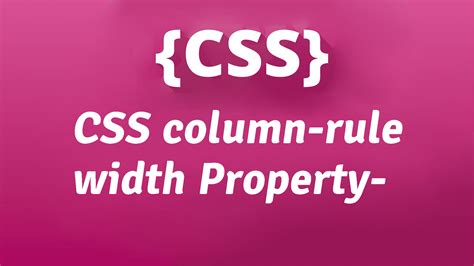
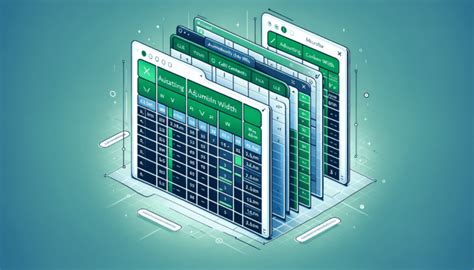
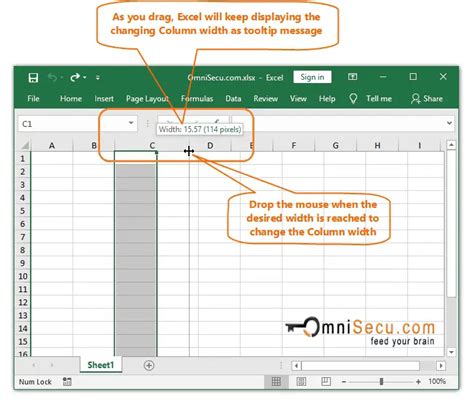
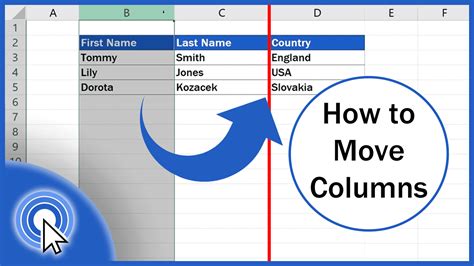
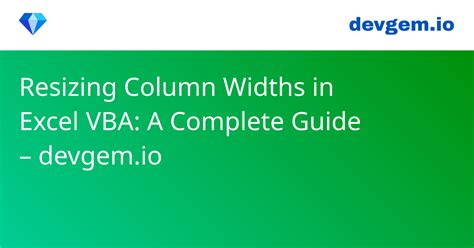
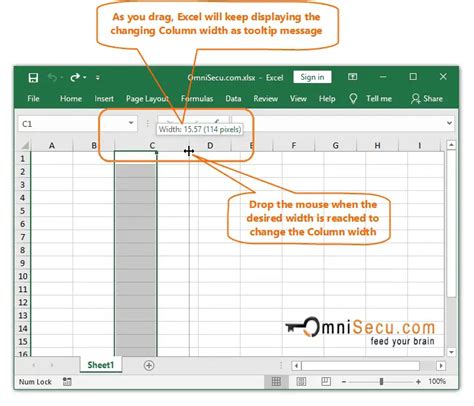
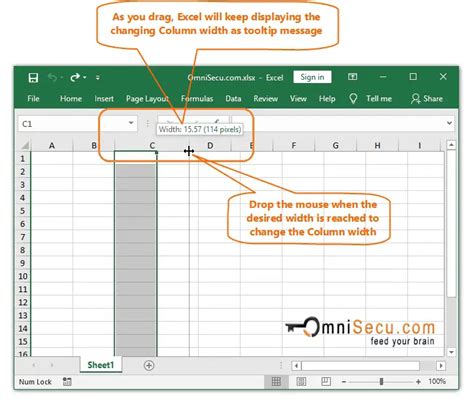
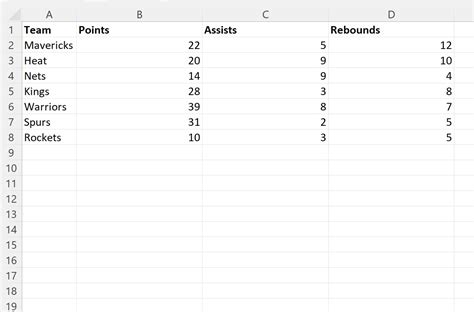
In conclusion, adjusting column widths in VBA Excel is a versatile task that can be accomplished through various methods, from simple property adjustments to more complex dynamic calculations. By mastering these techniques, you can significantly improve the readability and appearance of your spreadsheets.
What's your preferred method for adjusting column widths in Excel VBA? Have you encountered any challenges with column width adjustments that you'd like to discuss? Share your thoughts and experiences in the comments below.