Intro
Unlock the power of VBA file dialogues! Discover how to easily retrieve the selected file name with VBA, making file interactions a breeze. Learn how to use the FileDialog object, SelectedItems property, and FileName property to get the file name, path, and more. Simplify your VBA coding with this easy-to-follow guide.
The importance of file dialogues in VBA cannot be overstated. They provide a simple and intuitive way for users to interact with files and folders, making it easier to automate tasks and streamline workflows. One of the most common uses of file dialogues is to allow users to select a file, and then use the selected file name in further processing. However, getting the selected file name can be a bit tricky, especially for beginners. In this article, we will explore how to make getting the selected file name easy in VBA file dialogues.
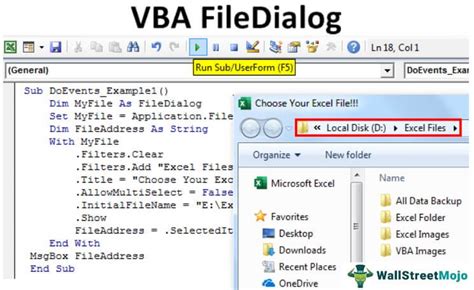
Understanding the FileDialogue Object
Before we dive into the nitty-gritty of getting the selected file name, it's essential to understand the FileDialogue object in VBA. The FileDialogue object is a part of the Application object and provides a way to interact with the file system. It allows you to open, save, and manipulate files, as well as display file dialogues to the user.
Getting the Selected File Name
Now that we have a basic understanding of the FileDialogue object, let's move on to getting the selected file name. There are a few ways to do this, but we will focus on the most common method, which is using the SelectedItems
property of the FileDialogue object.
Using the SelectedItems Property
The SelectedItems
property returns a collection of selected file names. To get the selected file name, you can use the following code:
Sub GetSelectedFileName()
Dim fd As FileDialog
Set fd = Application.FileDialog(msoFileDialogFilePicker)
With fd
.InitialFileName = "C:\"
.ButtonName = "Select File"
.AllowMultiSelect = False
If.Show Then
MsgBox.SelectedItems(1)
Else
MsgBox "No file selected"
End If
End With
End Sub
In this code, we first create a FileDialogue object and set its properties. We then display the file dialogue using the Show
method. If the user selects a file, the SelectedItems
property returns a collection containing the selected file name. We can then access the file name using the (1)
index.
Using a Variable to Store the Selected File Name
While the above code works, it's often more convenient to store the selected file name in a variable. We can do this by declaring a string variable and assigning the selected file name to it:
Sub GetSelectedFileName()
Dim fd As FileDialog
Dim selectedFileName As String
Set fd = Application.FileDialog(msoFileDialogFilePicker)
With fd
.InitialFileName = "C:\"
.ButtonName = "Select File"
.AllowMultiSelect = False
If.Show Then
selectedFileName =.SelectedItems(1)
MsgBox selectedFileName
Else
MsgBox "No file selected"
End If
End With
End Sub
Handling Multiple Selected Files
What if we want to allow the user to select multiple files? We can do this by setting the AllowMultiSelect
property to True
. However, this changes how we access the selected file names. Instead of using the (1)
index, we need to loop through the SelectedItems
collection:
Sub GetSelectedFileNames()
Dim fd As FileDialog
Dim selectedFileNames As Variant
Set fd = Application.FileDialog(msoFileDialogFilePicker)
With fd
.InitialFileName = "C:\"
.ButtonName = "Select Files"
.AllowMultiSelect = True
If.Show Then
selectedFileNames =.SelectedItems
For Each fileName In selectedFileNames
MsgBox fileName
Next fileName
Else
MsgBox "No files selected"
End If
End With
End Sub
Conclusion
Getting the selected file name in a VBA file dialogue is a common task, but it can be tricky, especially for beginners. By using the SelectedItems
property and understanding how to handle multiple selected files, you can make your VBA code more robust and user-friendly. Whether you're automating tasks or creating interactive applications, mastering the FileDialogue object is essential for any VBA developer.
VBA File Dialogue Selected File Name Made Easy Image Gallery
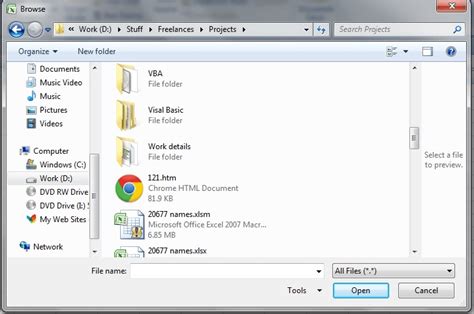
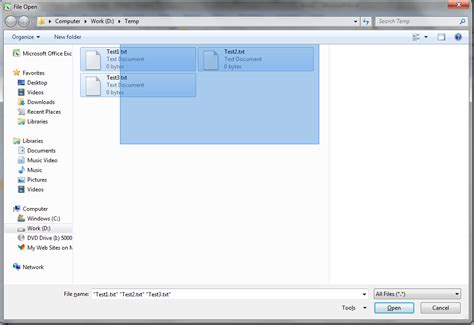
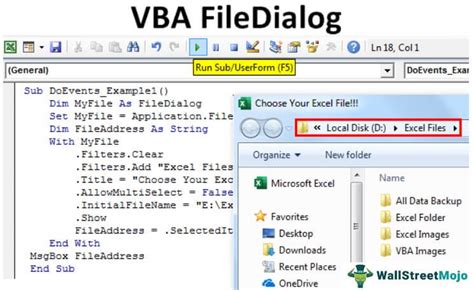
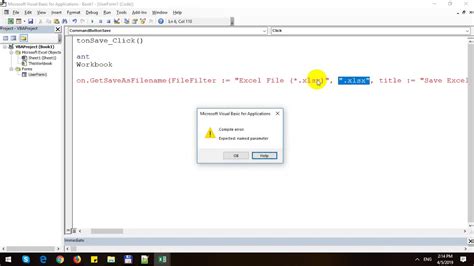
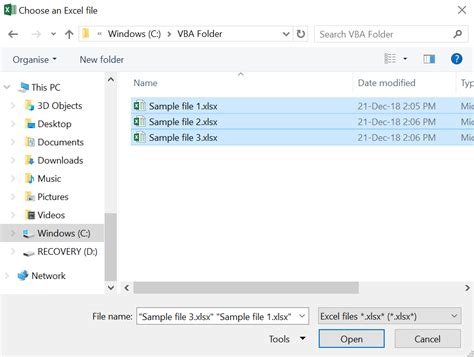
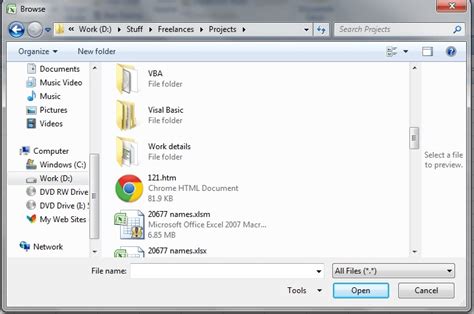
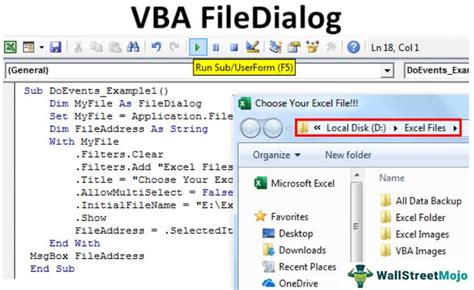
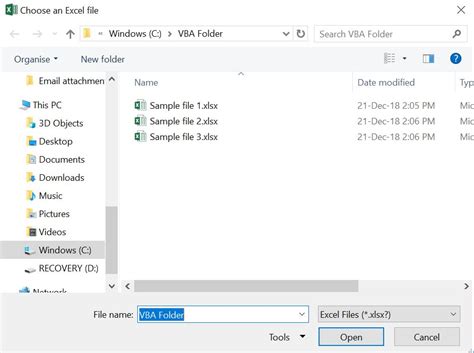
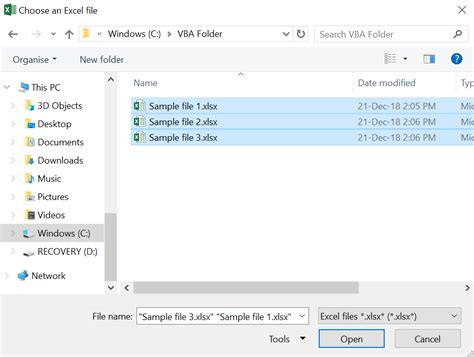
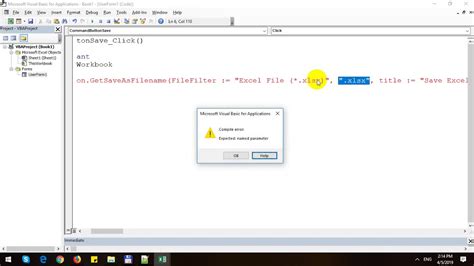
Share your thoughts! Have you ever struggled with getting the selected file name in a VBA file dialogue? How did you overcome the challenge? Share your experiences and tips in the comments below.