The humble Find and Replace function in Excel - a staple of data manipulation and cleanup. While the built-in Find and Replace dialog box is powerful, it can be limited in its capabilities. That's where Visual Basic for Applications (VBA) comes in - a programming language that allows you to automate and extend Excel's functionality. In this article, we'll explore five ways to use VBA for Excel Find and Replace, taking your data manipulation skills to the next level.
The Power of VBA in Excel
Before we dive into the examples, let's briefly discuss the benefits of using VBA in Excel. VBA allows you to:
- Automate repetitive tasks, freeing up time for more strategic work
- Create custom tools and interfaces to simplify complex tasks
- Extend Excel's functionality, allowing you to perform tasks that would be impossible or impractical with the built-in features
- Interact with other Office applications, such as Word and Outlook
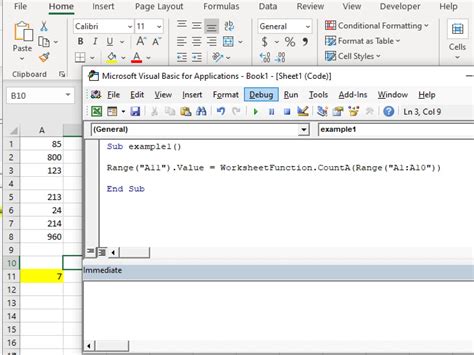
Method 1: Simple Find and Replace
Let's start with a basic example of using VBA for Find and Replace. Suppose you want to replace all instances of "old text" with "new text" in a worksheet. You can use the following code:
Sub SimpleFindReplace()
Cells.Replace What:="old text", Replacement:="new text", _
LookAt:=xlPart, SearchOrder:=xlByRows, MatchCase:=False
End Sub
This code uses the Cells.Replace
method to search for "old text" and replace it with "new text". The LookAt
parameter specifies whether to search for whole words or partial matches, while the SearchOrder
parameter determines the direction of the search.
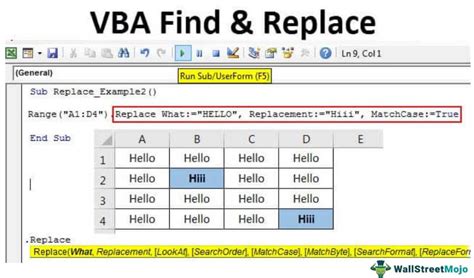
Method 2: Find and Replace with Multiple Criteria
What if you need to replace multiple strings at once? You can use an array to store the search and replacement values, and then loop through the array to perform the replacements. Here's an example:
Sub FindReplaceMultiple()
Dim searchArray As Variant
Dim replaceArray As Variant
Dim i As Long
searchArray = Array("old text 1", "old text 2", "old text 3")
replaceArray = Array("new text 1", "new text 2", "new text 3")
For i = LBound(searchArray) To UBound(searchArray)
Cells.Replace What:=searchArray(i), Replacement:=replaceArray(i), _
LookAt:=xlPart, SearchOrder:=xlByRows, MatchCase:=False
Next i
End Sub
This code uses two arrays to store the search and replacement values, and then loops through the arrays using a For
loop. The LBound
and UBound
functions are used to determine the bounds of the arrays.
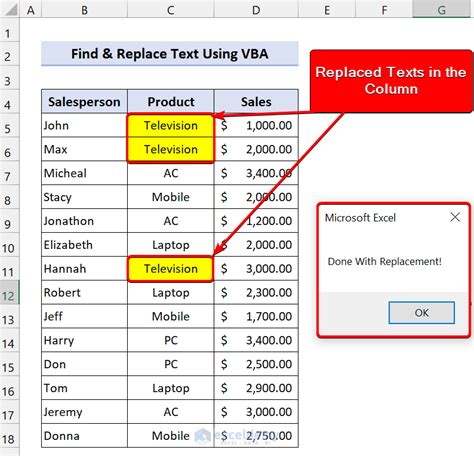
Method 3: Find and Replace with Regular Expressions
Regular expressions are a powerful tool for searching and replacing text patterns. Excel VBA supports regular expressions through the RegExp
object. Here's an example of using regular expressions to find and replace text:
Sub FindReplaceRegex()
Dim regex As Object
Set regex = CreateObject("VBScript.RegExp")
regex.Pattern = "old text \d+"
regex.Replace Cells.Value, "new text $1"
End Sub
This code uses the RegExp
object to search for the pattern "old text \d+", which matches the string "old text" followed by one or more digits. The $1
placeholder in the replacement string refers to the first captured group in the search pattern.
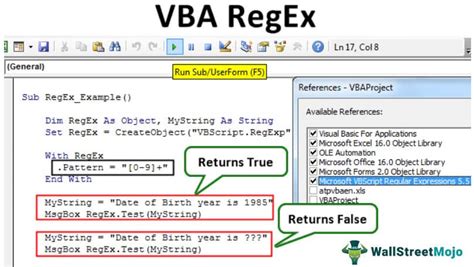
Method 4: Find and Replace with User Input
What if you want to prompt the user to enter the search and replacement values? You can use the InputBox
function to get user input, and then use the Cells.Replace
method to perform the replacement. Here's an example:
Sub FindReplaceUserInput()
Dim searchValue As String
Dim replaceValue As String
searchValue = InputBox("Enter the search value:", "Find and Replace")
replaceValue = InputBox("Enter the replacement value:", "Find and Replace")
Cells.Replace What:=searchValue, Replacement:=replaceValue, _
LookAt:=xlPart, SearchOrder:=xlByRows, MatchCase:=False
End Sub
This code uses the InputBox
function to prompt the user to enter the search and replacement values, and then uses the Cells.Replace
method to perform the replacement.
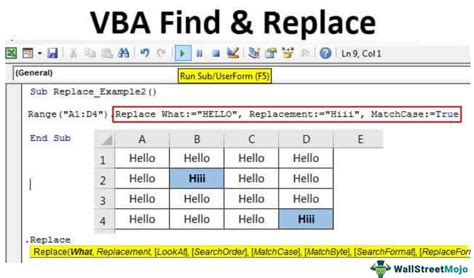
Method 5: Find and Replace with Multiple Worksheets
What if you need to find and replace text across multiple worksheets? You can use the Worksheets
collection to loop through the worksheets, and then use the Cells.Replace
method to perform the replacement. Here's an example:
Sub FindReplaceMultipleWorksheets()
Dim ws As Worksheet
Dim searchValue As String
Dim replaceValue As String
searchValue = "old text"
replaceValue = "new text"
For Each ws In ThisWorkbook.Worksheets
ws.Cells.Replace What:=searchValue, Replacement:=replaceValue, _
LookAt:=xlPart, SearchOrder:=xlByRows, MatchCase:=False
Next ws
End Sub
This code uses the Worksheets
collection to loop through the worksheets, and then uses the Cells.Replace
method to perform the replacement.
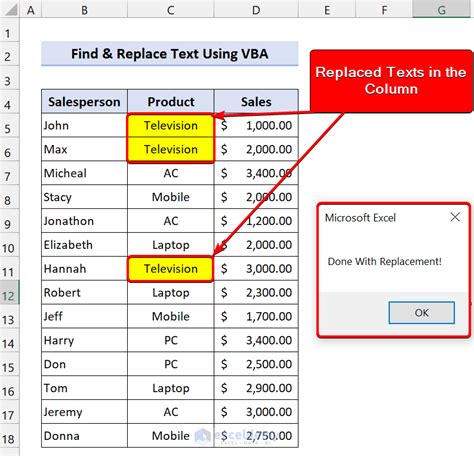
Gallery of Excel VBA Find and Replace
Excel VBA Find and Replace Gallery
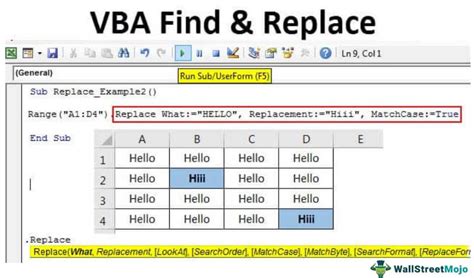
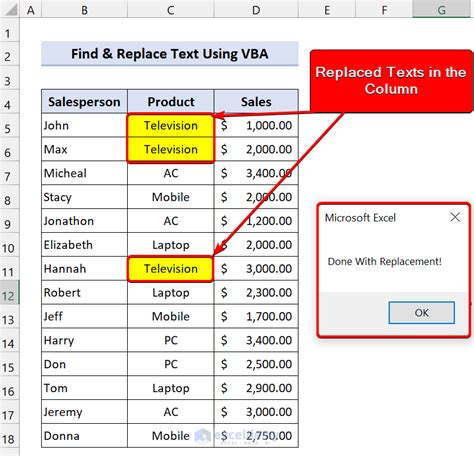
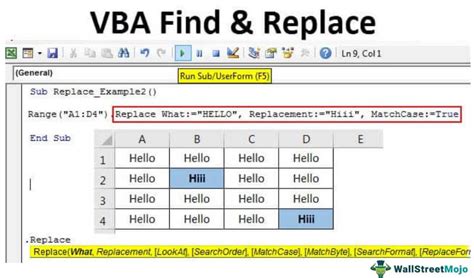
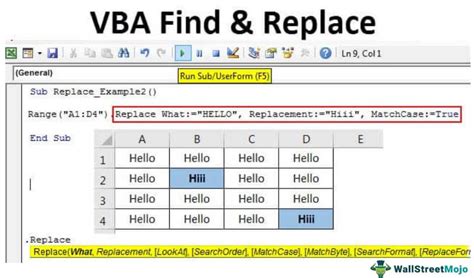
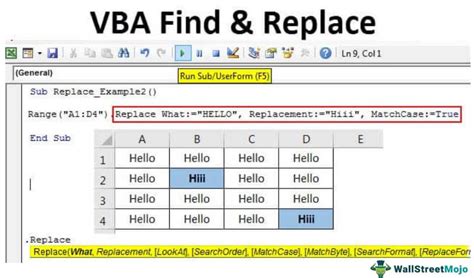
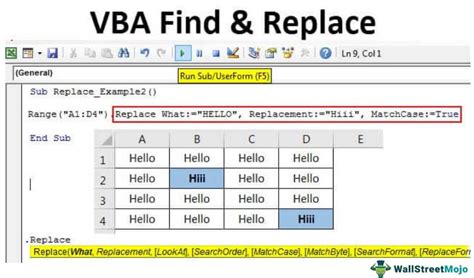
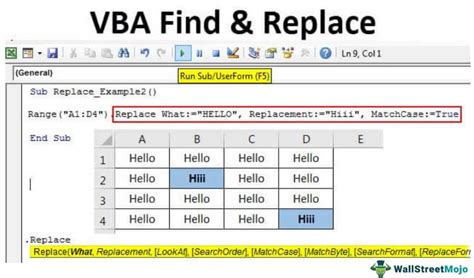
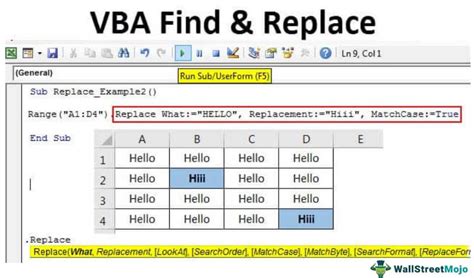
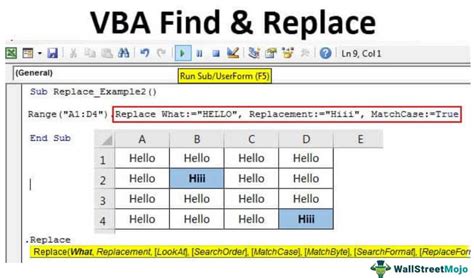
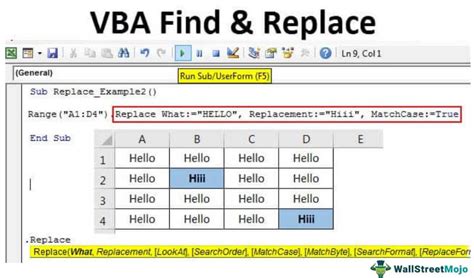
Conclusion
In conclusion, VBA can be a powerful tool for automating and extending Excel's Find and Replace functionality. By using the methods outlined in this article, you can take your data manipulation skills to the next level and perform complex tasks with ease. Whether you're a beginner or an advanced user, VBA can help you achieve your goals and increase your productivity. So why not give it a try?