Intro
Master VBA file handling with our expert guide on 5 ways to open a text file in VBA. Learn how to read, write, and manipulate text files using VBA coding techniques, including file input/output operations, file system objects, and text stream methods. Boost your VBA skills and automate file processing tasks with ease.
Opening a text file in VBA can be a straightforward process, but there are several methods to achieve this, each with its own advantages and use cases. This article will guide you through five ways to open a text file in VBA, providing you with a comprehensive understanding of the techniques involved.
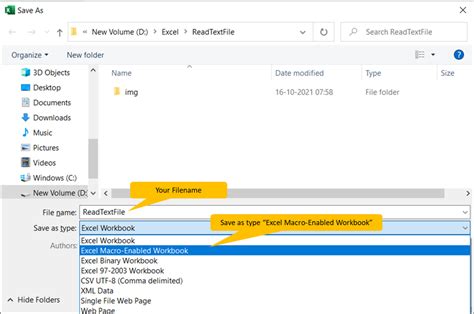
1. Using the Open
Statement
The most basic way to open a text file in VBA is by using the Open
statement. This method is straightforward and allows you to open a file in various modes, such as read-only or write.
Sub OpenTextFileUsingOpenStatement()
Dim fileNumber As Integer
fileNumber = FreeFile
Open "C:\example.txt" For Input As #fileNumber
' Code to read from the file
Close #fileNumber
End Sub
2. Using the FileSystemObject
The FileSystemObject
is a more powerful and flexible way to interact with files in VBA. This object is part of the Microsoft Scripting Runtime library, which you can add to your VBA project by going to Tools > References > Microsoft Scripting Runtime.
Sub OpenTextFileUsingFileSystemObject()
Dim fso As New FileSystemObject
Dim textStream As TextStream
Set textStream = fso.OpenTextFile("C:\example.txt", ForReading)
' Code to read from the file
textStream.Close
Set textStream = Nothing
Set fso = Nothing
End Sub
3. Using Late Binding
If you don't want to set a reference to the Microsoft Scripting Runtime library, you can use late binding to create a FileSystemObject
.
Sub OpenTextFileUsingLateBinding()
Dim fso As Object
Dim textStream As Object
Set fso = CreateObject("Scripting.FileSystemObject")
Set textStream = fso.OpenTextFile("C:\example.txt", 1)
' Code to read from the file
textStream.Close
Set textStream = Nothing
Set fso = Nothing
End Sub
4. Using Workbooks.OpenText
If you need to open a text file in Excel VBA, you can use the Workbooks.OpenText
method. This method allows you to open a text file as an Excel workbook.
Sub OpenTextFileUsingWorkbooksOpenText()
Workbooks.OpenText "C:\example.txt"
End Sub
5. Using ADODB.Stream
Another way to open a text file in VBA is by using the ADODB.Stream
object. This object allows you to read and write binary data to a file.
Sub OpenTextFileUsingADODBStream()
Dim stream As New ADODB.Stream
stream.Open
stream.Type = adTypeText
stream.LoadFromFile "C:\example.txt"
' Code to read from the file
stream.Close
Set stream = Nothing
End Sub
In conclusion, there are several ways to open a text file in VBA, each with its own strengths and weaknesses. By understanding these different methods, you can choose the best approach for your specific needs.
Gallery of Opening Text Files in VBA
Opening Text Files in VBA

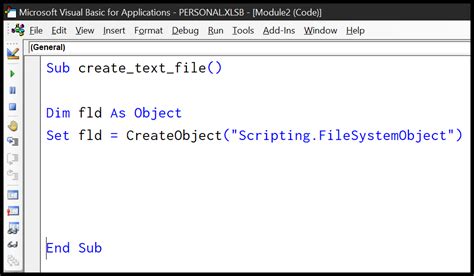
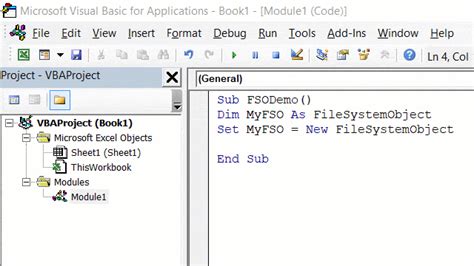
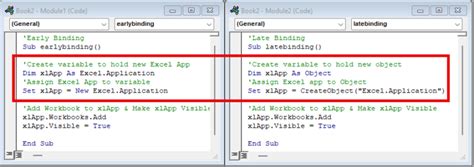
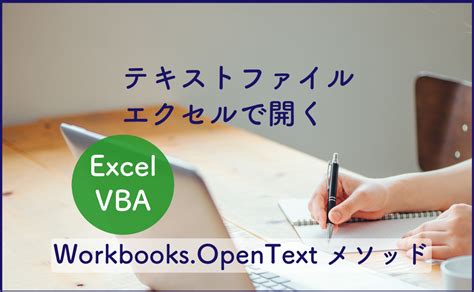
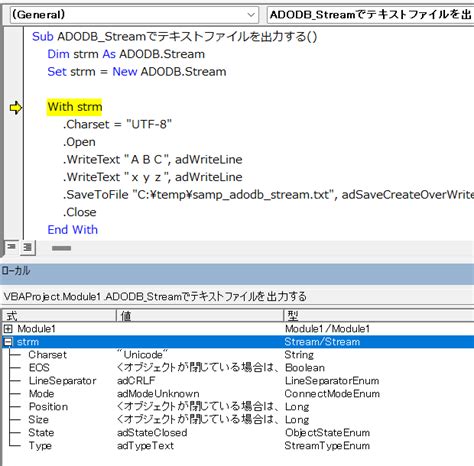
We hope this article has provided you with a comprehensive understanding of the different ways to open a text file in VBA. If you have any questions or need further clarification, please don't hesitate to ask.