Intro
Master VBA string manipulation with 5 efficient ways to replace characters. Learn how to use VBAs built-in functions, such as Replace, InStr, and Mid, to substitute characters in strings. Discover how to handle special cases, like multiple replacements, and optimize performance. Improve your VBA coding skills with these actionable tips and examples.
Replacing characters in VBA strings is an essential task in various programming scenarios, from data cleaning to generating customized output. VBA, which stands for Visual Basic for Applications, is a programming language used in Microsoft Office applications, including Excel. When working with strings in VBA, you might need to replace certain characters or sets of characters with others. This can be due to formatting requirements, data compatibility issues, or other reasons. Here are five ways to replace characters in VBA strings, each serving different purposes and offering various levels of control and complexity.
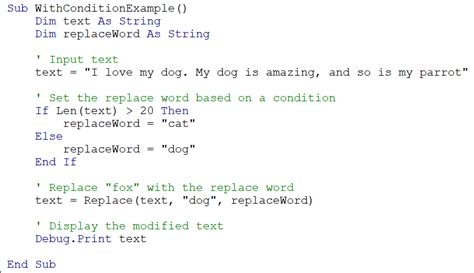
1. Using the Replace
Function
The most straightforward method to replace characters in a VBA string is by using the Replace
function. This function is intuitive and directly serves the purpose of replacing specified characters.
Sub ReplaceCharacters()
Dim originalString As String
Dim replacedString As String
originalString = "Hello, World!"
replacedString = Replace(originalString, "World", "Earth")
MsgBox "Original: " & originalString & vbCrLf & "Replaced: " & replacedString
End Sub
In this example, the Replace
function takes three arguments: the original string, the string to be replaced, and the replacement string. It then returns a new string with the replacements made.
2. Using RegExp
for Complex Patterns
For more complex character replacement tasks, especially those involving patterns (like regular expressions), VBA offers the RegExp
object. This object provides powerful pattern matching and replacement capabilities.
Sub RegExpReplace()
Dim originalString As String
Dim pattern As String
Dim replacement As String
Dim regEx As Object
Set regEx = CreateObject("VBScript.RegExp")
originalString = "Hello, 123 World 456"
pattern = "\d+" ' Matches one or more digits
replacement = "X"
regEx.Pattern = pattern
regEx.Global = True
MsgBox "Original: " & originalString & vbCrLf & "Replaced: " & regEx.Replace(originalString, replacement)
End Sub
In this example, the regular expression \d+
matches one or more digits, and these are replaced with an "X".
3. Using InStr
and String Manipulation
Sometimes, you might need to replace a character or a string that appears at a specific position within the original string. The InStr
function can help locate the position, and then you can use string manipulation functions to replace the characters.
Sub InStrReplace()
Dim originalString As String
Dim searchString As String
Dim replacement As String
Dim position As Integer
originalString = "Hello, World!"
searchString = "World"
replacement = "Earth"
position = InStr(1, originalString, searchString)
If position > 0 Then
originalString = Mid(originalString, 1, position - 1) & replacement & Mid(originalString, position + Len(searchString))
End If
MsgBox "Original: Hello, World!" & vbCrLf & "Replaced: " & originalString
End Sub
This method is more manual and can be less efficient for large strings or complex replacements but is useful for understanding the basics of string manipulation.
4. Looping Through Characters
For very specific requirements, especially when the replacement logic is complex or dynamic, looping through each character in the string might be the best approach.
Sub LoopReplace()
Dim originalString As String
Dim replacedString As String
Dim i As Integer
originalString = "Hello, World!"
replacedString = ""
For i = 1 To Len(originalString)
If Mid(originalString, i, 1) = "W" Then
replacedString = replacedString & "E"
Else
replacedString = replacedString & Mid(originalString, i, 1)
End If
Next i
MsgBox "Original: " & originalString & vbCrLf & "Replaced: " & replacedString
End Sub
This method gives you complete control over the replacement logic but can be slower and more cumbersome for large strings.
5. Using VBA's WorksheetFunction
for Non-VBA Formulas
Although not directly a VBA function, for users familiar with Excel formulas, the SUBSTITUTE
or REPLACE
worksheet functions can be used within VBA through the WorksheetFunction
object.
Sub WorksheetFunctionReplace()
Dim originalString As String
Dim replacedString As String
originalString = "Hello, World!"
' Using SUBSTITUTE
replacedString = Application.WorksheetFunction.Substitute(originalString, "World", "Earth")
MsgBox "Original: " & originalString & vbCrLf & "Replaced: " & replacedString
End Sub
This approach leverages Excel's built-in functions and can be particularly useful when working with data in worksheets.
VBA String Replacement Methods
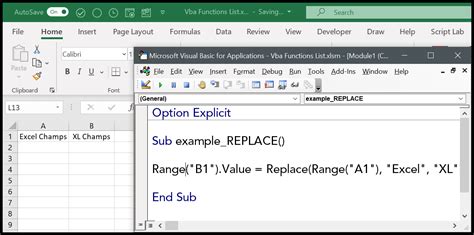
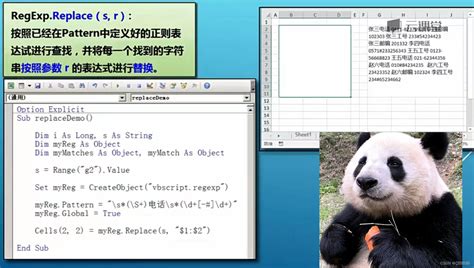
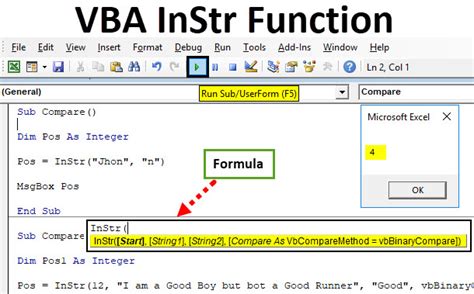
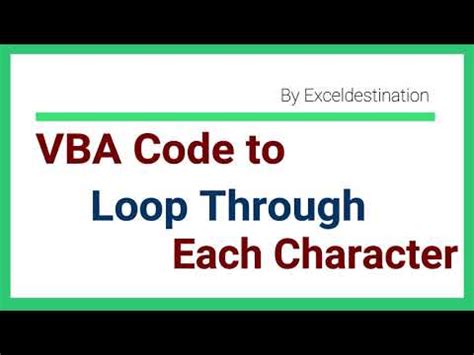
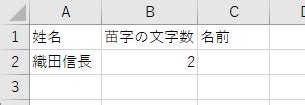
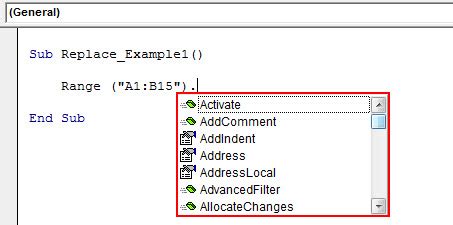
Each of these methods has its own use cases and can be applied based on the complexity and requirements of the string replacement task at hand. By mastering these techniques, you can efficiently manipulate strings in VBA to suit your programming needs.
If you have specific questions or would like to explore more about string manipulation in VBA, feel free to ask in the comments section.