Intro
Mastering VBA range selection is crucial for efficient data manipulation. Discover 5 expert-approved methods to select a range in VBA, including using Range.Find, Range.Offset, and Range.Resize. Learn how to define and manipulate ranges with ease, optimize your code, and improve your Excel automation skills with this comprehensive guide.
Working with ranges is a fundamental aspect of programming in VBA (Visual Basic for Applications). A range in VBA refers to a group of cells that you can manipulate programmatically. Selecting a range allows you to perform various operations, such as formatting, data manipulation, and calculations. In this article, we will explore five ways to select a range in VBA.
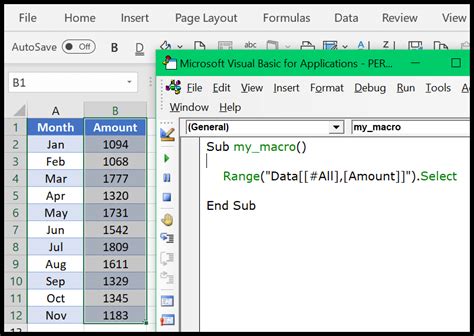
Understanding Range Selection
Before diving into the methods of selecting a range, it's essential to understand the basic syntax of range selection in VBA. The Range
object is used to represent a range of cells. You can select a range by specifying the starting and ending cell addresses.
1. Selecting a Range Using Cell Addresses
One of the most common ways to select a range is by specifying the cell addresses. You can use the Range
object to select a range by providing the starting and ending cell addresses.
Sub SelectRange()
Range("A1:B2").Select
End Sub
In this example, the range A1:B2 is selected.
2. Selecting a Range Using Variables
You can also select a range by using variables to store the cell addresses. This method is useful when you need to dynamically select a range based on user input or other conditions.
Sub SelectRangeUsingVariables()
Dim startCell As String
Dim endCell As String
startCell = "A1"
endCell = "B2"
Range(startCell & ":" & endCell).Select
End Sub
In this example, the variables startCell
and endCell
are used to store the cell addresses, which are then used to select the range.
3. Selecting a Range Using the Cells Property
The Cells
property is another way to select a range. This property allows you to specify the row and column numbers of the cells you want to select.
Sub SelectRangeUsingCells()
Range(Cells(1, 1), Cells(2, 2)).Select
End Sub
In this example, the range A1:B2 is selected using the Cells
property.
4. Selecting a Range Using the Offset Property
The Offset
property allows you to select a range by offsetting from a specific cell. This property is useful when you need to select a range that is relative to another cell.
Sub SelectRangeUsingOffset()
Range("A1").Offset(0, 1).Resize(2, 2).Select
End Sub
In this example, the range B1:C2 is selected by offsetting from cell A1.
5. Selecting a Range Using the CurrentRegion Property
The CurrentRegion
property allows you to select a range that is bounded by blank rows and columns. This property is useful when you need to select a range that contains data.
Sub SelectRangeUsingCurrentRegion()
Range("A1").CurrentRegion.Select
End Sub
In this example, the range A1:B2 is selected using the CurrentRegion
property.
Benefits of Each Method
Each method of selecting a range has its own benefits. Here are some benefits of each method:
- Selecting a range using cell addresses: This method is straightforward and easy to understand. It is useful when you know the exact cell addresses of the range you want to select.
- Selecting a range using variables: This method is useful when you need to dynamically select a range based on user input or other conditions.
- Selecting a range using the Cells property: This method is useful when you need to select a range based on row and column numbers.
- Selecting a range using the Offset property: This method is useful when you need to select a range that is relative to another cell.
- Selecting a range using the CurrentRegion property: This method is useful when you need to select a range that contains data.
Common Mistakes to Avoid
Here are some common mistakes to avoid when selecting a range in VBA:
- Using the Select method unnecessarily: The Select method can slow down your code and cause errors. Avoid using it unless it is necessary.
- Not qualifying the Range object: Make sure to qualify the Range object with the worksheet object to avoid errors.
- Not checking for errors: Always check for errors when selecting a range to avoid runtime errors.
Range Selection in VBA Image Gallery
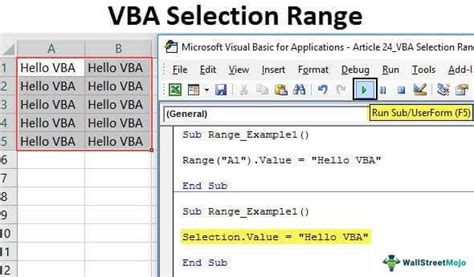
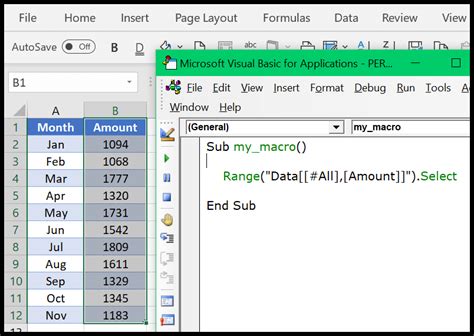
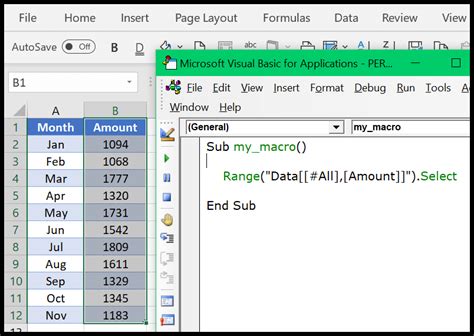
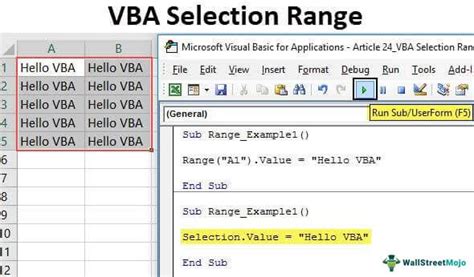
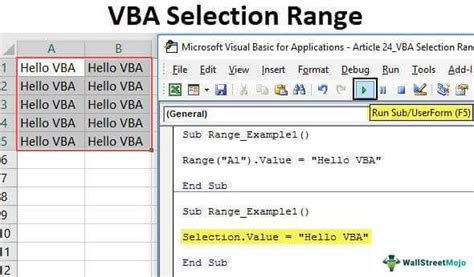
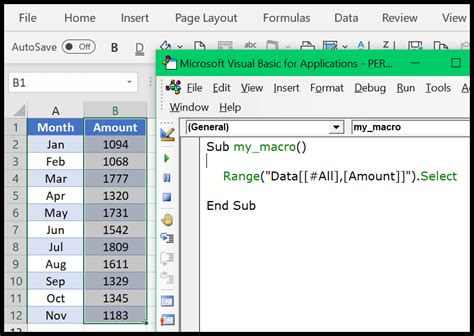
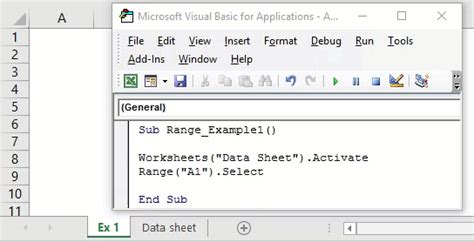
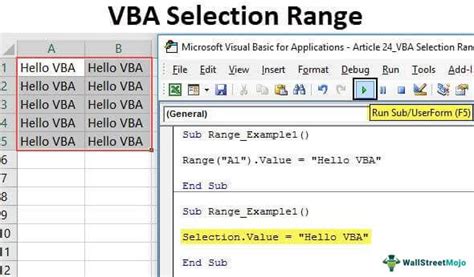
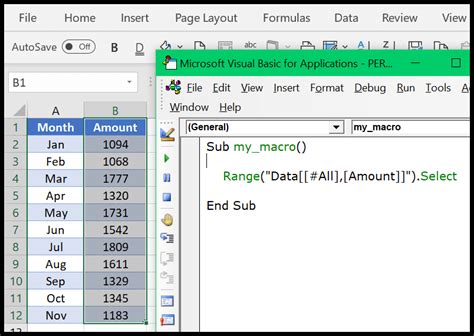
By following the methods and best practices outlined in this article, you can effectively select ranges in VBA and improve the efficiency of your code. Remember to avoid common mistakes and always check for errors to ensure that your code runs smoothly.