Intro
Boost your Excel productivity with a powerful VBA macro to delete columns quickly and efficiently. Learn how to automate column deletion tasks using VBA scripting, covering topics like range selection, column indexing, and error handling. Streamline your workflow with this step-by-step guide and master Excel column management.
Delete Columns in Excel Quickly with VBA Macro
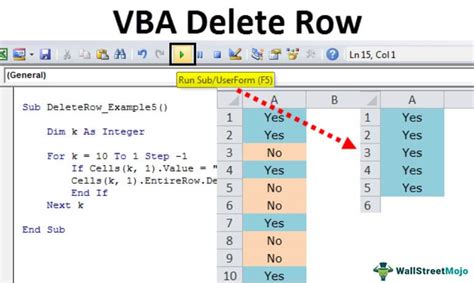
Are you tired of manually deleting columns in Excel? Do you find yourself wasting time selecting and deleting columns one by one? Look no further! With a simple VBA macro, you can quickly delete columns in Excel and save yourself a lot of time and effort.
In this article, we will explore the benefits of using VBA macros to delete columns in Excel, how to create a VBA macro to delete columns, and provide some practical examples and tips to get you started.
Benefits of Using VBA Macro to Delete Columns in Excel
Using a VBA macro to delete columns in Excel offers several benefits, including:
- Time-saving: With a VBA macro, you can delete multiple columns at once, saving you time and effort.
- Efficient: VBA macros can automate repetitive tasks, making it easier to manage large datasets.
- Accurate: VBA macros can help reduce errors caused by manual deletion of columns.
- Customizable: You can customize the VBA macro to suit your specific needs and delete columns based on specific conditions.
How to Create a VBA Macro to Delete Columns in Excel
To create a VBA macro to delete columns in Excel, follow these steps:
- Open the Visual Basic Editor by pressing
Alt + F11
or by navigating toDeveloper
>Visual Basic
in the ribbon. - In the Visual Basic Editor, click
Insert
>Module
to insert a new module. - In the module, paste the following code:
Sub DeleteColumns() Dim ws As Worksheet Set ws = ActiveSheet
' Specify the column numbers to delete
Dim columnsToDelete As Variant
columnsToDelete = Array(1, 3, 5)
' Delete the columns
For i = UBound(columnsToDelete) To LBound(columnsToDelete) Step -1
ws.Columns(columnsToDelete(i)).Delete
Next i
End Sub
This code deletes columns 1, 3, and 5 in the active worksheet.
4. Save the module by clicking `File` > `Save` or by pressing `Ctrl + S`.
5. To run the macro, click `Developer` > `Macros` in the ribbon, select the `DeleteColumns` macro, and click `Run`.
Practical Examples and Tips
Here are some practical examples and tips to help you get the most out of your VBA macro:
* **Delete columns based on header**: To delete columns based on the header, you can modify the code to search for the header and then delete the column. For example:
```vb
Sub DeleteColumnsBasedOnHeader()
Dim ws As Worksheet
Set ws = ActiveSheet
' Specify the header to search for
Dim headerToSearch As String
headerToSearch = "Header"
' Find the column with the header
Dim columnToFind As Range
Set columnToFind = ws.Rows(1).Find(headerToSearch)
' Delete the column
If Not columnToFind Is Nothing Then
columnToFind.EntireColumn.Delete
End If
End Sub
- Delete columns based on condition: To delete columns based on a condition, you can modify the code to check for the condition and then delete the column. For example:
Sub DeleteColumnsBasedOnCondition() Dim ws As Worksheet Set ws = ActiveSheet
' Specify the condition to check
Dim conditionToCheck As String
conditionToCheck = "=A1>10"
' Find the column with the condition
Dim columnToFind As Range
Set columnToFind = ws.Rows(1).Find(conditionToCheck)
' Delete the column
If Not columnToFind Is Nothing Then
columnToFind.EntireColumn.Delete
End If
End Sub
* **Delete multiple columns at once**: To delete multiple columns at once, you can modify the code to specify multiple column numbers or headers. For example:
```vb
Sub DeleteMultipleColumns()
Dim ws As Worksheet
Set ws = ActiveSheet
' Specify the column numbers to delete
Dim columnsToDelete As Variant
columnsToDelete = Array(1, 3, 5, 7, 9)
' Delete the columns
For i = UBound(columnsToDelete) To LBound(columnsToDelete) Step -1
ws.Columns(columnsToDelete(i)).Delete
Next i
End Sub
Best Practices for Using VBA Macros to Delete Columns in Excel
Here are some best practices to keep in mind when using VBA macros to delete columns in Excel:
- Backup your data: Before running a VBA macro to delete columns, make sure to backup your data to avoid losing important information.
- Test the macro: Before running the macro on a large dataset, test it on a small sample to ensure it works as expected.
- Use error handling: Use error handling to handle any errors that may occur during the execution of the macro.
- Document the macro: Document the macro to explain what it does and how it works.
Gallery of VBA Macros for Deleting Columns in Excel
VBA Macros for Deleting Columns in Excel
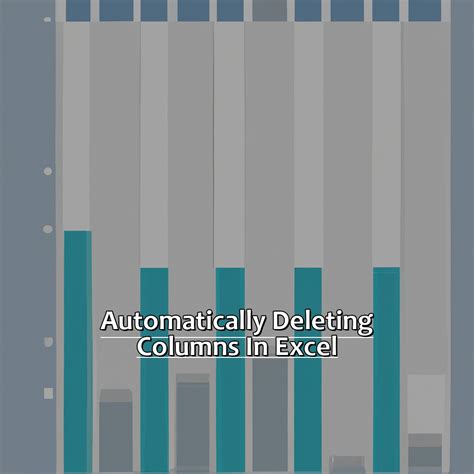
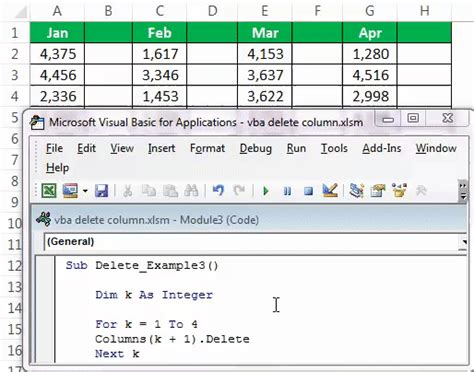
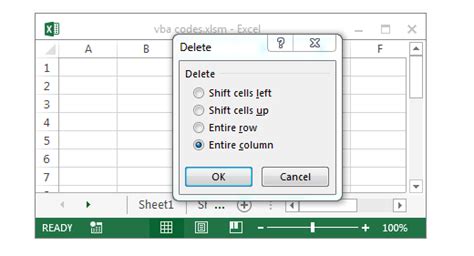
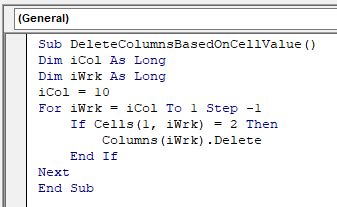
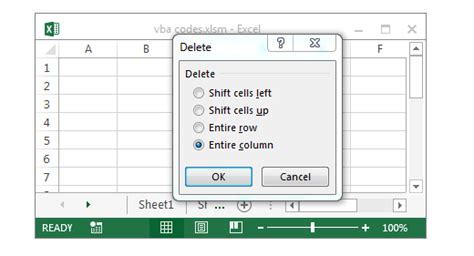
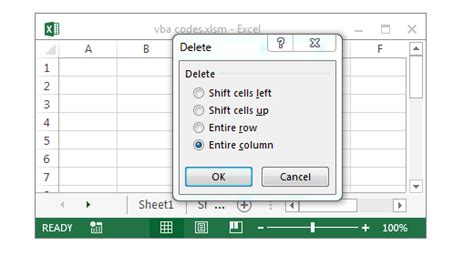
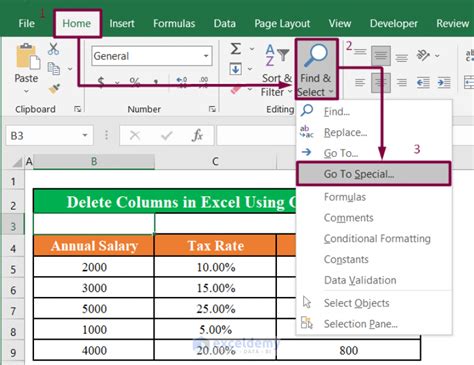
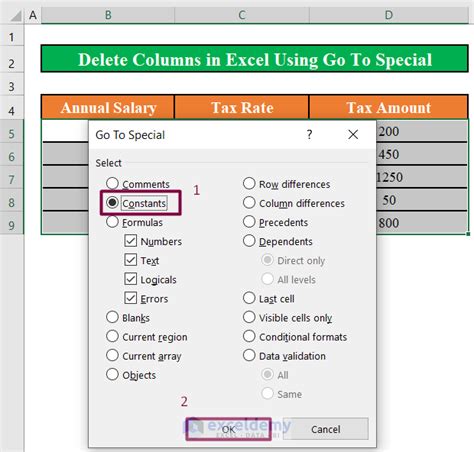
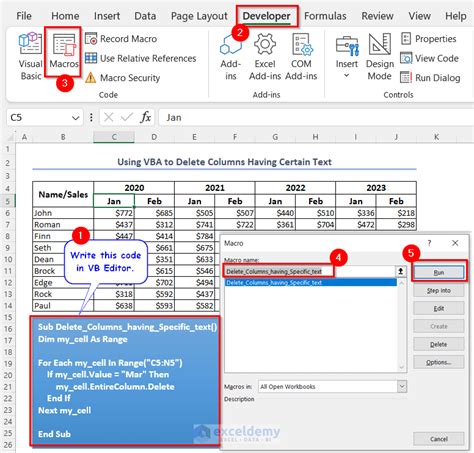
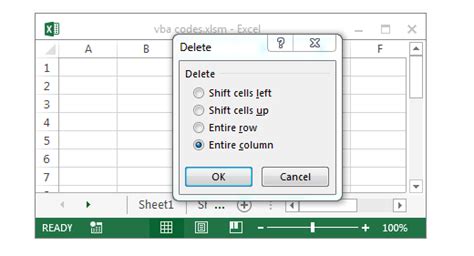
We hope this article has helped you learn how to create a VBA macro to delete columns in Excel quickly and efficiently. By following the steps and best practices outlined in this article, you can automate the process of deleting columns and save yourself a lot of time and effort.