Intro
Master the art of opening Excel files with VBA effortlessly. Learn how to automate file opening using Visual Basic for Applications, streamlining your workflow. Discover the step-by-step process, including enabling VBA, writing macros, and troubleshooting common errors. Unlock the power of VBA to enhance your Excel productivity and efficiency.
Opening an Excel file using VBA (Visual Basic for Applications) can be a straightforward process, but it can also be a bit tricky if you're new to VBA. In this article, we'll cover the basics of opening an Excel file with VBA and provide some examples to get you started.
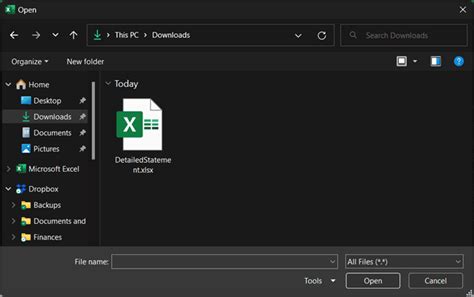
Why Open Excel Files with VBA?
There are several reasons why you might want to open an Excel file with VBA. For example, you might want to automate a task that involves reading or writing data to an Excel file, or you might want to integrate Excel with another application or system. Whatever your reason, VBA provides a powerful and flexible way to interact with Excel files programmatically.
Basic Steps to Open an Excel File with VBA
To open an Excel file with VBA, you'll need to follow these basic steps:
- Create a new VBA module in your Excel workbook or in a separate VBA project.
- Declare a variable to hold the Excel application object.
- Use the
Workbooks.Open
method to open the Excel file. - Optionally, you can also specify additional parameters to control how the file is opened.
Here's an example of how you might open an Excel file using VBA:
Sub OpenExcelFile()
Dim xlApp As Excel.Application
Dim xlWorkbook As Excel.Workbook
' Create a new instance of the Excel application
Set xlApp = New Excel.Application
' Open the Excel file
Set xlWorkbook = xlApp.Workbooks.Open("C:\Path\To\Your\File.xlsx")
' Do something with the workbook...
' Close the workbook and quit the application
xlWorkbook.Close
xlApp.Quit
End Sub
Tips and Variations
Here are a few tips and variations to keep in mind when opening Excel files with VBA:
- Specify the file path and name: When using the
Workbooks.Open
method, you'll need to specify the full path and name of the Excel file you want to open. - Use the
ReadOnly
parameter: If you want to open the file in read-only mode, you can pass theReadOnly
parameter to theWorkbooks.Open
method. - Use the
Password
parameter: If the Excel file is password-protected, you'll need to pass the password to theWorkbooks.Open
method using thePassword
parameter. - Use late binding: If you're using VBA in a non-Excel application, you might need to use late binding to interact with the Excel application object.
Common Errors and Solutions
When working with VBA and Excel files, you might encounter a few common errors. Here are some solutions to help you troubleshoot:
- Error: "File not found": Make sure the file path and name are correct, and that the file exists in the specified location.
- Error: "Permission denied": Check the file permissions to ensure that the user account running the VBA code has read and write access to the file.
- Error: "Excel is not responding": Try closing any other instances of Excel that might be running, and then try opening the file again.
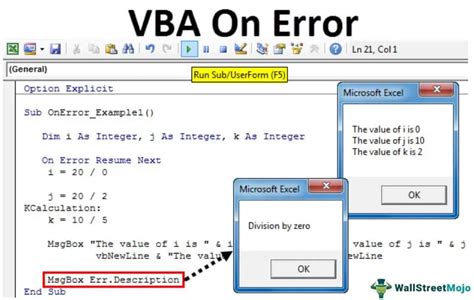
Advanced Techniques
Once you've mastered the basics of opening Excel files with VBA, you can explore more advanced techniques to automate and customize your workflows. Here are a few ideas:
- Use VBA to automate tasks: Use VBA to automate repetitive tasks, such as formatting data or generating reports.
- Use VBA to integrate with other applications: Use VBA to integrate Excel with other applications, such as Word or PowerPoint.
- Use VBA to create custom tools: Use VBA to create custom tools and add-ins for Excel, such as custom menus or buttons.
Gallery of VBA and Excel
VBA and Excel Gallery
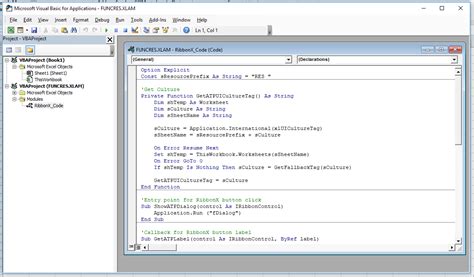
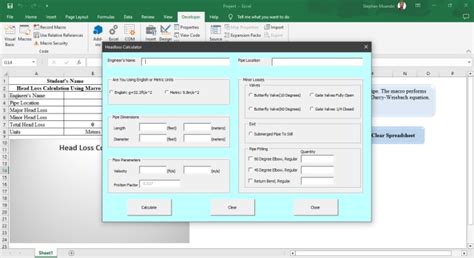
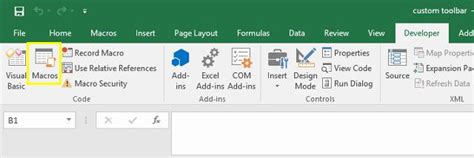
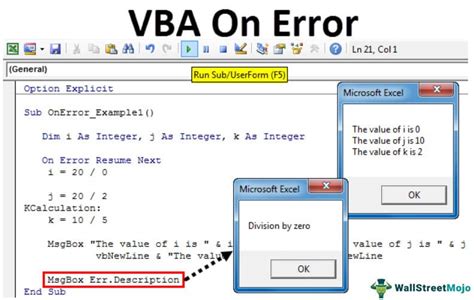
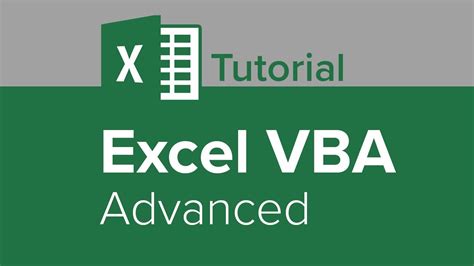
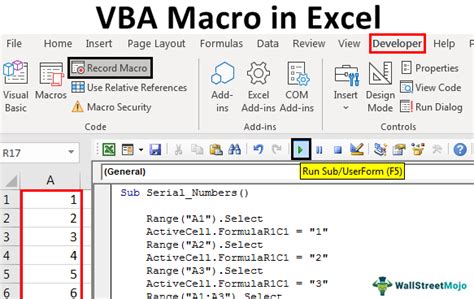
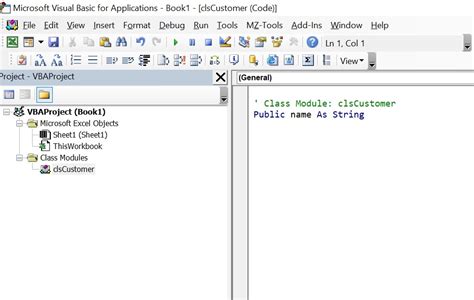
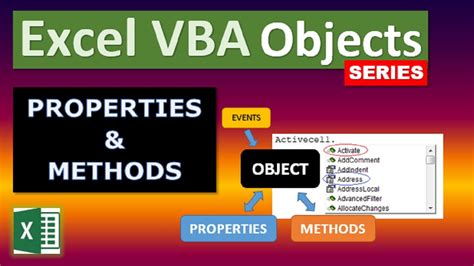
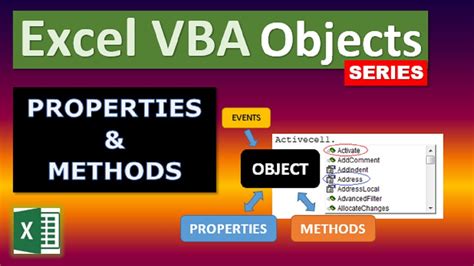
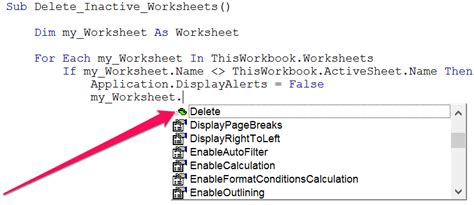
By following these tips and techniques, you can master the art of opening Excel files with VBA and take your automation and integration skills to the next level. Happy coding!