Intro
Boost VBA performance by learning how to disable screen updating in Excel VBA. Discover the benefits of disabling screen updating, including faster execution and reduced flicker. Get step-by-step code examples and expert tips on how to optimize your VBA macros by turning off screen updates, improving user experience and productivity.
Disabling screen updating in VBA is a technique used to improve the performance and speed of your VBA code. When screen updating is enabled, Excel updates the screen after each line of code is executed, which can slow down your code significantly. By disabling screen updating, you can prevent Excel from updating the screen unnecessarily, allowing your code to run much faster.
Why Disable Screen Updating?
Disabling screen updating can benefit your VBA code in several ways:
- Improved Performance: By preventing Excel from updating the screen after each line of code, you can significantly improve the performance of your code.
- Reduced Flickering: Disabling screen updating can reduce the flickering effect that occurs when Excel updates the screen rapidly.
- Better User Experience: By preventing unnecessary screen updates, you can provide a better user experience, especially when running long or complex VBA code.
How to Disable Screen Updating in VBA
To disable screen updating in VBA, you can use the following code:
Application.ScreenUpdating = False
This line of code sets the ScreenUpdating
property of the Application
object to False
, effectively disabling screen updating.
Example Code
Here's an example code that demonstrates how to disable screen updating:
Sub DisableScreenUpdating()
' Disable screen updating
Application.ScreenUpdating = False
' Your code here...
' Enable screen updating
Application.ScreenUpdating = True
End Sub
In this example, we disable screen updating at the beginning of the code, execute our code, and then enable screen updating again at the end.
When to Disable Screen Updating
You should disable screen updating when:
- Running Long or Complex Code: If your code takes a long time to execute or performs complex operations, disabling screen updating can improve performance.
- Performing Frequent Updates: If your code updates cells or ranges frequently, disabling screen updating can reduce flickering.
- Improving User Experience: If your code runs in the background or performs tasks that don't require user interaction, disabling screen updating can provide a better user experience.
Best Practices
When disabling screen updating, keep the following best practices in mind:
- Enable Screen Updating Again: Always enable screen updating again after your code has finished executing to prevent issues with Excel's user interface.
- Use Try-Catch Blocks: Use try-catch blocks to catch any errors that may occur when disabling screen updating.
- Test Your Code: Test your code thoroughly after disabling screen updating to ensure it works as expected.
By disabling screen updating in VBA, you can significantly improve the performance and speed of your code, reduce flickering, and provide a better user experience.
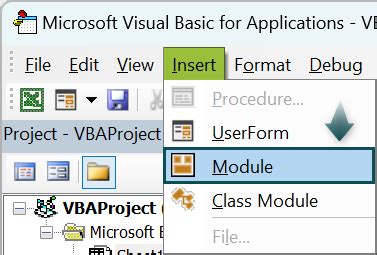
Common Issues and Troubleshooting
If you encounter issues after disabling screen updating, try the following:
- Check for Errors: Check your code for errors and ensure that screen updating is enabled again after your code has finished executing.
- Use Debugging Tools: Use debugging tools, such as the VBA debugger or Excel's built-in debugging tools, to identify issues.
- Consult Online Resources: Consult online resources, such as Microsoft documentation or VBA forums, for troubleshooting tips and solutions.
By following these best practices and troubleshooting tips, you can effectively disable screen updating in VBA and improve the performance of your code.
Disable Screen Updating in VBA Image Gallery
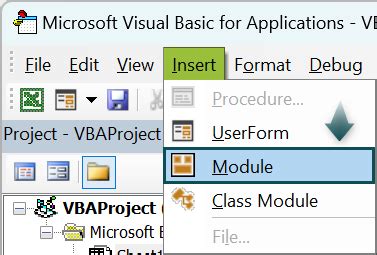
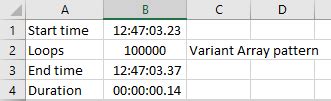
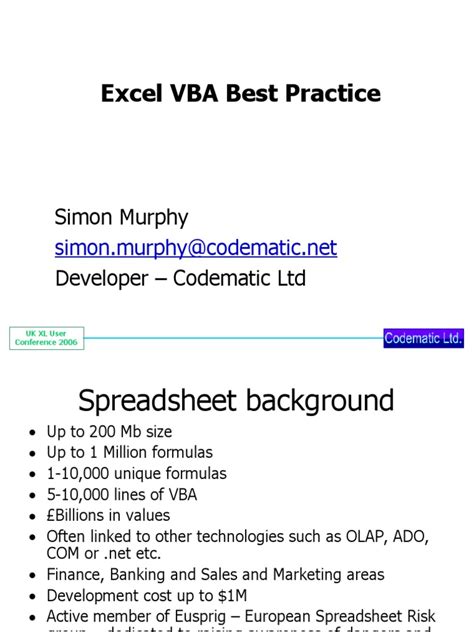
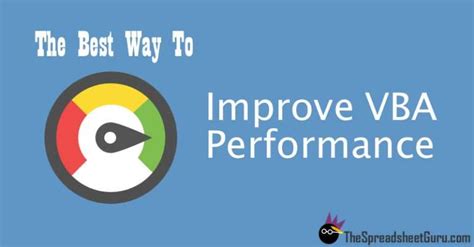
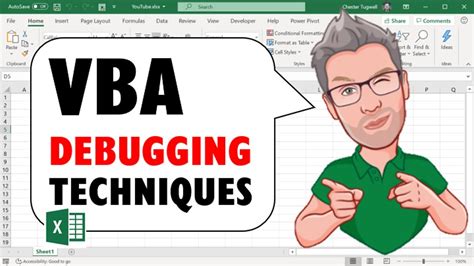
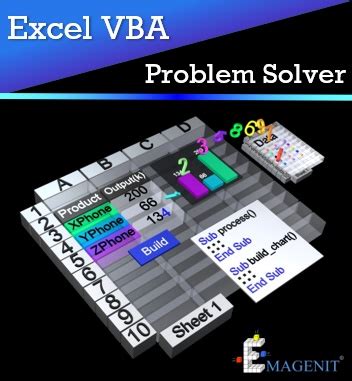
We hope this article has helped you understand the benefits of disabling screen updating in VBA and how to implement it effectively in your code. If you have any questions or need further assistance, please don't hesitate to ask.