Intro
Resolve the VBA User Defined Type Not Defined error with expert solutions. Discover how to fix UDT issues in Visual Basic for Applications, troubleshoot compiler errors, and master type definitions to streamline your VBA projects. Learn from examples and debugging tips to overcome type mismatch errors and enhance your coding skills.
VBA User Defined Type Not Defined Error: Causes and Solutions
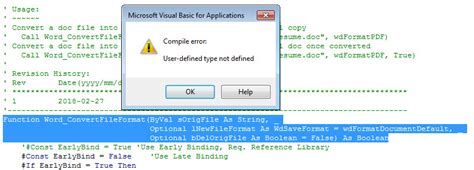
When working with Visual Basic for Applications (VBA), you may encounter the "User Defined Type Not Defined" error. This error can be frustrating, especially if you're new to VBA programming. In this article, we'll explore the causes of this error and provide step-by-step solutions to fix it.
What is a User Defined Type in VBA?
In VBA, a User Defined Type (UDT) is a custom data type that you can create to store multiple values. A UDT is defined using the Type
statement, and it can contain one or more elements, such as integers, strings, or other data types.
Causes of the User Defined Type Not Defined Error
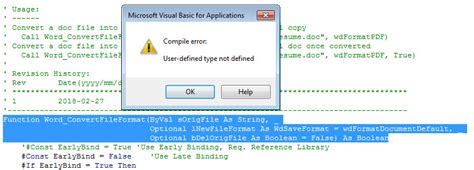
The "User Defined Type Not Defined" error typically occurs when VBA encounters a UDT that it doesn't recognize. Here are some common causes of this error:
- Missing or incomplete UDT definition: If the UDT definition is missing or incomplete, VBA won't recognize it, and you'll get this error.
- Typo or incorrect UDT name: If you mistype the UDT name or use an incorrect name, VBA won't be able to find the definition.
- UDT definition is not in scope: If the UDT definition is not in the same scope as the code that's using it, VBA won't be able to find it.
- Code is not compiled: If the code is not compiled, VBA won't be able to recognize the UDT.
Fixing the User Defined Type Not Defined Error
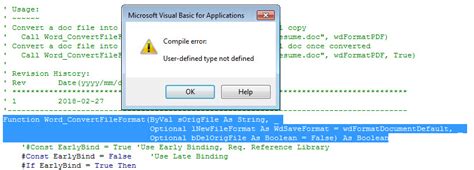
To fix the "User Defined Type Not Defined" error, follow these steps:
Step 1: Check the UDT definition
- Make sure the UDT definition is complete and correct.
- Check for any typos or incorrect UDT names.
Step 2: Verify the UDT definition is in scope
- Ensure the UDT definition is in the same scope as the code that's using it.
- If the UDT definition is in a different module, make sure it's properly declared and accessible.
Step 3: Compile the code
- Compile the code by clicking on the "Debug" menu and selecting "Compile VBAProject" or pressing Alt+F11.
- This will help VBA recognize the UDT definition.
Step 4: Check for conflicts with built-in VBA types
- Make sure the UDT name doesn't conflict with any built-in VBA types.
- If there's a conflict, rename the UDT to avoid the conflict.
Example: Creating and Using a UDT in VBA
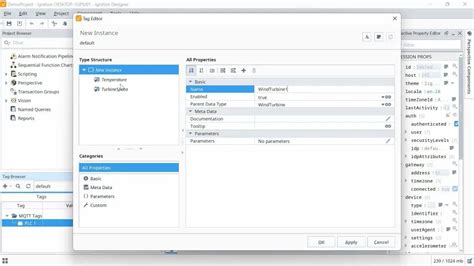
Here's an example of creating and using a UDT in VBA:
' Define a UDT called "Person"
Type Person
Name As String
Age As Integer
End Type
Sub TestUDT()
' Declare a variable of type Person
Dim person As Person
' Assign values to the UDT elements
person.Name = "John Doe"
person.Age = 30
' Print the UDT elements
Debug.Print person.Name, person.Age
End Sub
In this example, we define a UDT called "Person" with two elements: "Name" and "Age". We then declare a variable of type Person and assign values to the UDT elements. Finally, we print the UDT elements using the Debug.Print
statement.
Gallery of VBA User Defined Type Not Defined Error Images
VBA User Defined Type Not Defined Error Images
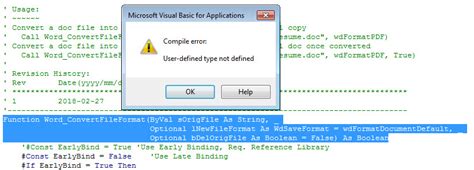
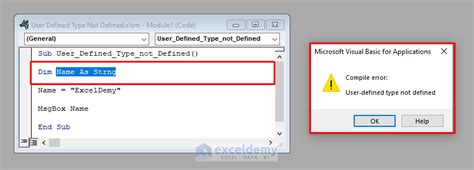
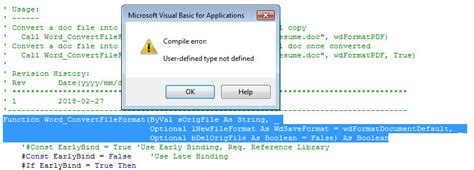
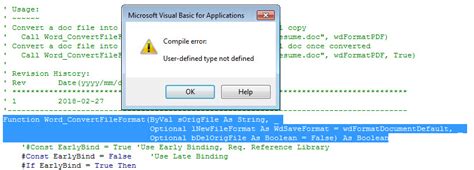
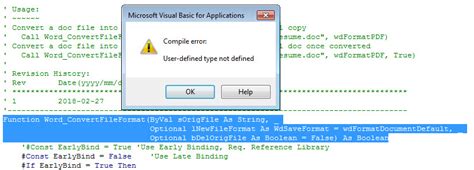
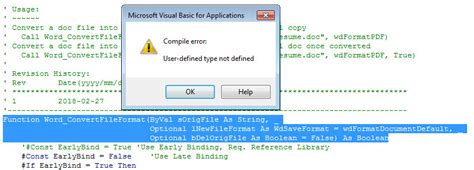
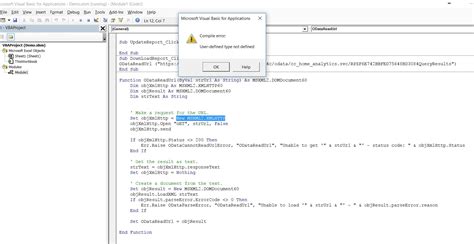
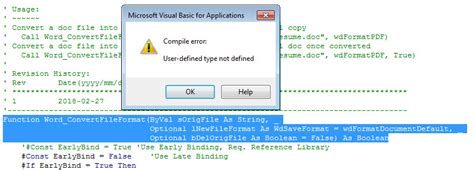
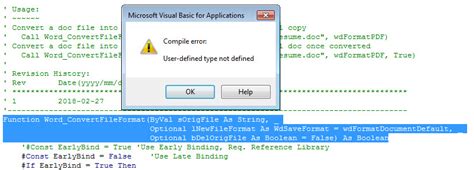
We hope this article has helped you understand the causes and solutions for the "User Defined Type Not Defined" error in VBA. If you have any further questions or need help with a specific issue, feel free to ask in the comments below.