Function traps are a fundamental concept in programming, particularly in languages that support functional programming paradigms. These traps play a crucial role in handling errors, making code more robust and reliable. In this article, we'll delve into five ways function traps errors, exploring the benefits and mechanisms behind this powerful programming technique.

Understanding Function Traps
Before diving into the ways function traps errors, it's essential to understand what function traps are. In simple terms, function traps are a way to wrap a function around another function, allowing for pre- and post-execution code to run. This wrapping mechanism enables developers to handle errors, log events, or perform other tasks without modifying the original function.
1. Try-Catch Blocks
One of the most common ways function traps errors is through try-catch blocks. By wrapping a function in a try-catch block, developers can catch and handle exceptions that occur during execution. This approach ensures that the program doesn't crash due to a single error, allowing for more robust error handling.
Example:
function divide(a, b) {
try {
return a / b;
} catch (error) {
console.error("Error:", error);
return null;
}
}
In this example, the divide
function is wrapped in a try-catch block, catching any errors that occur during division.

2. Error Handling Functions
Another way function traps errors is through error handling functions. These functions can be used to wrap other functions, catching and handling errors in a centralized manner. This approach makes it easier to manage error handling across multiple functions.
Example:
function errorHandler(fn) {
return function(...args) {
try {
return fn(...args);
} catch (error) {
console.error("Error:", error);
return null;
}
};
}
const divide = errorHandler((a, b) => a / b);
In this example, the errorHandler
function wraps the divide
function, catching and handling any errors that occur during execution.

3. Aspect-Oriented Programming (AOP)
Aspect-Oriented Programming (AOP) is a programming paradigm that allows developers to modularize cross-cutting concerns, such as error handling. AOP frameworks provide a way to wrap functions with aspects, which can handle errors and other concerns in a centralized manner.
Example:
const aspect = (fn) => {
return function(...args) {
try {
return fn(...args);
} catch (error) {
console.error("Error:", error);
return null;
}
};
};
const divide = aspect((a, b) => a / b);
In this example, the aspect
function wraps the divide
function, catching and handling any errors that occur during execution.

4. Higher-Order Functions
Higher-order functions are functions that take other functions as arguments or return functions as output. These functions can be used to wrap other functions, catching and handling errors in a functional programming style.
Example:
const withErrorHandling = (fn) => {
return function(...args) {
try {
return fn(...args);
} catch (error) {
console.error("Error:", error);
return null;
}
};
};
const divide = withErrorHandling((a, b) => a / b);
In this example, the withErrorHandling
function wraps the divide
function, catching and handling any errors that occur during execution.

5. Decorators
Decorators are a design pattern that allows developers to wrap functions with additional behavior, such as error handling. Decorators are commonly used in languages like Python and JavaScript.
Example:
function errorHandler(target, propertyKey, descriptor) {
const originalMethod = descriptor.value;
descriptor.value = function(...args) {
try {
return originalMethod.apply(this, args);
} catch (error) {
console.error("Error:", error);
return null;
}
};
return descriptor;
}
class Calculator {
@errorHandler
divide(a, b) {
return a / b;
}
}
In this example, the errorHandler
decorator wraps the divide
method, catching and handling any errors that occur during execution.

Conclusion
In conclusion, function traps are a powerful programming technique for handling errors and making code more robust. By using try-catch blocks, error handling functions, Aspect-Oriented Programming (AOP), higher-order functions, and decorators, developers can catch and handle errors in a centralized manner. Whether you're working with functional programming or object-oriented programming, understanding function traps can help you write more reliable and maintainable code.
Function Traps Errors Image Gallery






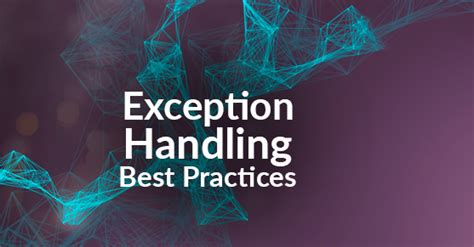


