Intro
Discover 5 efficient ways to check if a cell is empty in VBA, including using the ISBLANK function, checking for null values, and leveraging VBAs built-in cell properties. Learn how to optimize your VBA code with these expert-approved methods, improving your Excel automation workflows and data management tasks.
Determining whether a cell is empty or not is a common task in VBA (Visual Basic for Applications) programming. There are several ways to achieve this, and the approach you choose may depend on your specific requirements and the version of Excel you are using. Here are five ways to check if a cell is empty in VBA:
Method 1: Using the IsEmpty Function
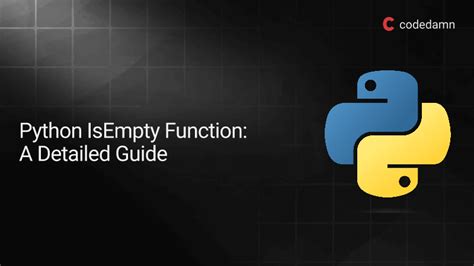
The IsEmpty
function is a built-in VBA function that returns True
if a variable or cell is empty, and False
otherwise. You can use this function to check if a cell is empty like this:
If IsEmpty(Range("A1").Value) Then
MsgBox "Cell A1 is empty"
Else
MsgBox "Cell A1 is not empty"
End If
Method 2: Checking the Cell Value Directly
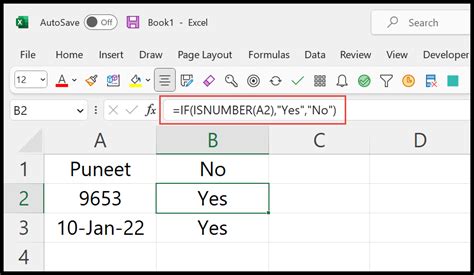
You can also check if a cell is empty by comparing its value to an empty string. Here's an example:
If Range("A1").Value = "" Then
MsgBox "Cell A1 is empty"
Else
MsgBox "Cell A1 is not empty"
End If
Method 3: Using the Len Function
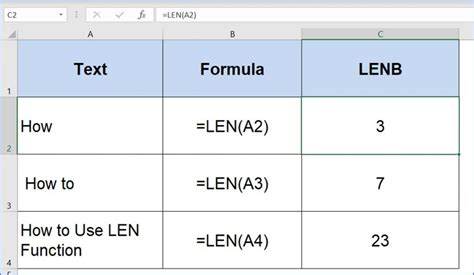
The Len
function returns the length of a string. If the length of the cell value is 0, then the cell is empty. Here's how you can use this function:
If Len(Range("A1").Value) = 0 Then
MsgBox "Cell A1 is empty"
Else
MsgBox "Cell A1 is not empty"
End If
Method 4: Checking for Errors
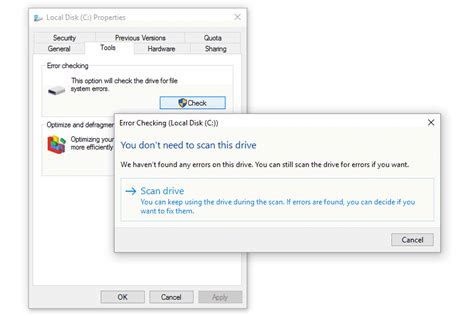
If the cell contains an error value (such as #N/A or #VALUE!), you may want to consider it empty. You can use the IsError
function to check for errors:
If IsError(Range("A1").Value) Then
MsgBox "Cell A1 contains an error"
ElseIf Range("A1").Value = "" Then
MsgBox "Cell A1 is empty"
Else
MsgBox "Cell A1 is not empty"
End If
Method 5: Using a Custom Function
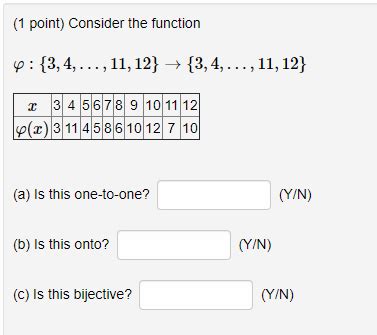
You can create a custom function to check if a cell is empty. This function can use any of the methods above and can be reused throughout your code:
Function IsCellEmpty(cell As Range) As Boolean
If IsEmpty(cell.Value) Or cell.Value = "" Then
IsCellEmpty = True
Else
IsCellEmpty = False
End If
End Function
You can then use this function in your code like this:
If IsCellEmpty(Range("A1")) Then
MsgBox "Cell A1 is empty"
Else
MsgBox "Cell A1 is not empty"
End If
In conclusion, there are several ways to check if a cell is empty in VBA. The method you choose will depend on your specific needs and the version of Excel you are using.
Gallery of VBA Images
VBA Image Gallery
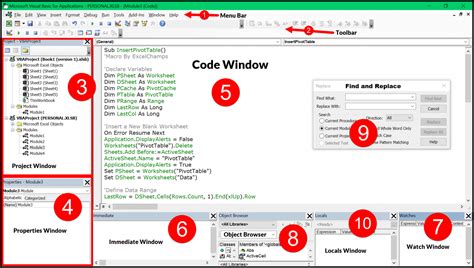
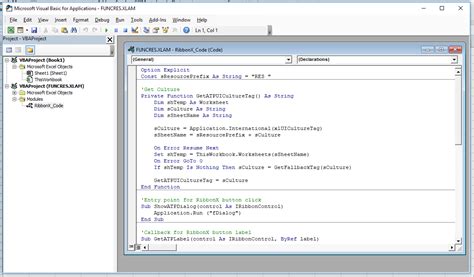
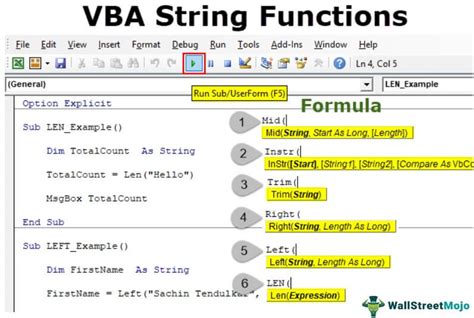
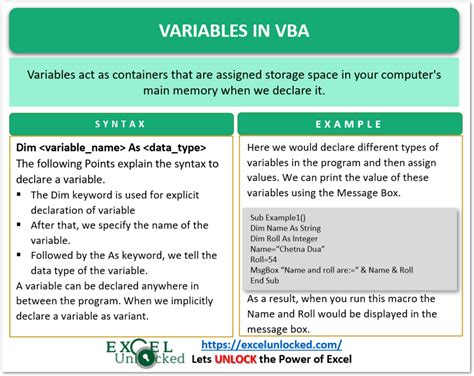
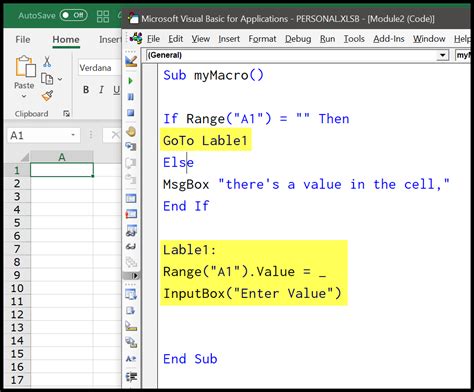
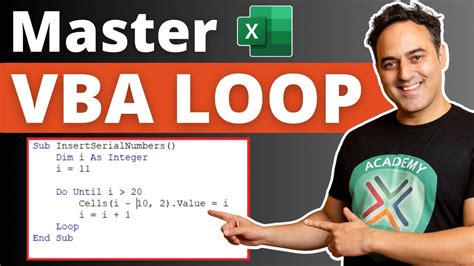
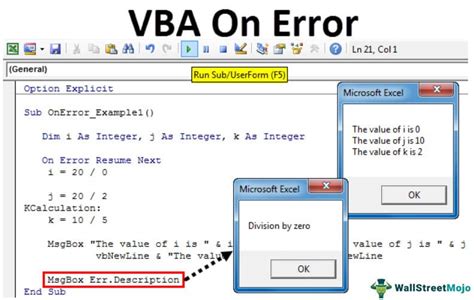
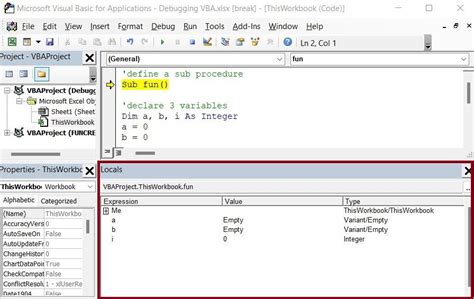
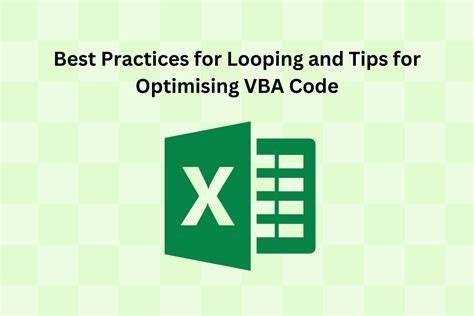
FAQ
Q: What is the difference between IsEmpty
and Len
functions in VBA?
A: IsEmpty
checks if a variable or cell is empty, while Len
returns the length of a string.
Q: Can I use IsEmpty
function to check if a range of cells is empty?
A: No, IsEmpty
function only checks a single cell or variable. To check a range of cells, you need to loop through each cell in the range.
Q: How do I check if a cell contains an error value in VBA?
A: You can use the IsError
function to check if a cell contains an error value.
Q: Can I create a custom function to check if a cell is empty in VBA? A: Yes, you can create a custom function using VBA to check if a cell is empty.