Intro
Secure user input in Excel VBA with these 3 methods to mask passwords in InputBox. Learn how to conceal sensitive information, protect data integrity, and enhance security using VBA coding techniques. Discover expert tips for creating masked password inputs, improving data protection, and ensuring compliance with industry standards.
As Excel VBA developers, we often come across situations where we need to prompt users to enter sensitive information, such as passwords or credit card numbers. However, the standard InputBox function in Excel VBA displays the input text in plain sight, which can be a security risk. In this article, we will explore three ways to mask passwords in Excel VBA InputBox, ensuring that sensitive information remains confidential.
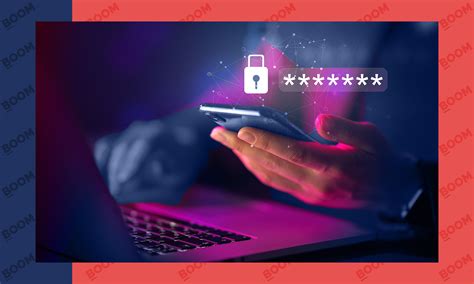
Why Mask Passwords in Excel VBA InputBox?
Before we dive into the solutions, it's essential to understand why masking passwords is crucial. When users enter sensitive information, it's visible to anyone who might be watching, which can lead to security breaches. By masking passwords, we can protect users' sensitive information and maintain confidentiality.
Method 1: Using the Application.InputBox
Method with a Masked Input
One way to mask passwords in Excel VBA InputBox is to use the Application.InputBox
method with a masked input. This method allows us to specify the input type and mask the input text.
Sub MaskedInputBox()
Dim password As String
password = Application.InputBox("Enter your password", "Password", "", Type:=2)
MsgBox "You entered: " & password
End Sub
In this example, the Type:=2
argument specifies that the input should be a string, and the input text will be masked.
Method 2: Creating a Custom InputBox with a Masked Input
Another way to mask passwords in Excel VBA InputBox is to create a custom InputBox with a masked input. We can use a UserForm to create a custom InputBox and set the PasswordChar
property to mask the input text.
Sub CustomInputBox()
Dim uf As UserForm
Set uf = New UserForm1
uf.Show
Dim password As String
password = uf.TextBox1.Value
MsgBox "You entered: " & password
End Sub
In this example, we create a new instance of the UserForm1
and show it using the Show
method. The PasswordChar
property is set to mask the input text in the TextBox control.
Method 3: Using the MSComctlLib
Library to Mask Passwords
The third method involves using the MSComctlLib
library to mask passwords in Excel VBA InputBox. This library provides a TextBox
control that can be used to mask passwords.
Sub MaskedInputBox_MSComctlLib()
Dim password As String
password = InputBox("Enter your password", "Password", "", Type:=2)
Dim tb As MSComctlLib.TextBox
Set tb = New MSComctlLib.TextBox
tb.PasswordChar = "*"
tb.Text = password
MsgBox "You entered: " & tb.Text
End Sub
In this example, we use the MSComctlLib
library to create a new instance of the TextBox
control. We set the PasswordChar
property to mask the input text and assign the input text to the Text
property.
Benefits of Masking Passwords in Excel VBA InputBox
Masking passwords in Excel VBA InputBox provides several benefits, including:
- Security: Masking passwords protects sensitive information from being visible to others.
- Confidentiality: By masking passwords, we can maintain confidentiality and ensure that sensitive information remains private.
- Compliance: Masking passwords can help organizations comply with security regulations and standards.
Common Challenges and Solutions
When implementing password masking in Excel VBA InputBox, developers may encounter several challenges, including:
- Compatibility Issues: Some methods may not work on all versions of Excel or Windows.
- User Experience: Masking passwords can affect the user experience, especially if the input text is not visible.
To overcome these challenges, developers can use a combination of methods, such as using a custom InputBox with a masked input or utilizing the MSComctlLib
library.
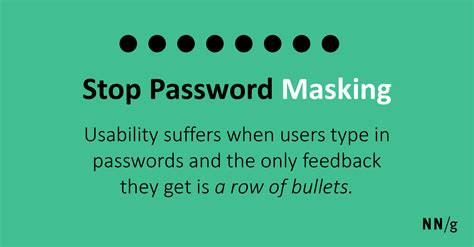
Best Practices for Masking Passwords in Excel VBA InputBox
To ensure secure and effective password masking in Excel VBA InputBox, follow these best practices:
- Use a combination of methods: Use a combination of methods, such as using a custom InputBox with a masked input or utilizing the
MSComctlLib
library. - Test for compatibility: Test the password masking method for compatibility with different versions of Excel and Windows.
- Consider user experience: Consider the user experience and ensure that the input text is not visible but still accessible.
Conclusion
Masking passwords in Excel VBA InputBox is crucial for protecting sensitive information and maintaining confidentiality. By using one of the three methods outlined in this article, developers can ensure that passwords are masked and secure. Remember to follow best practices and test for compatibility to ensure a seamless user experience.
Password Masking Gallery
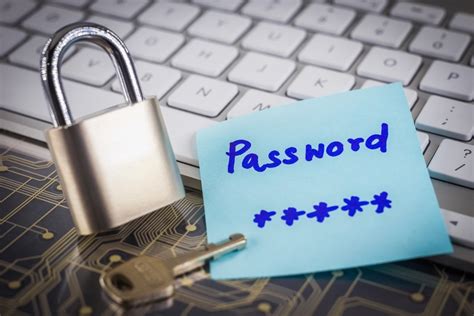
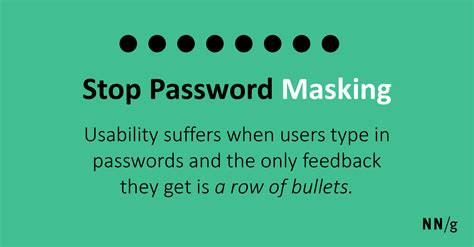
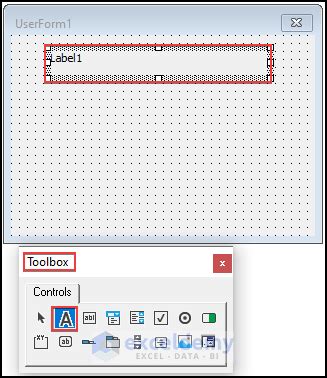
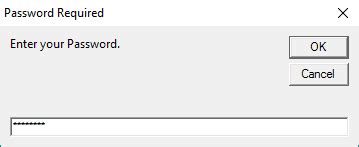
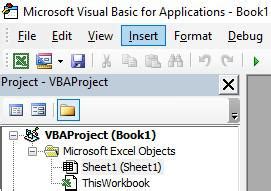
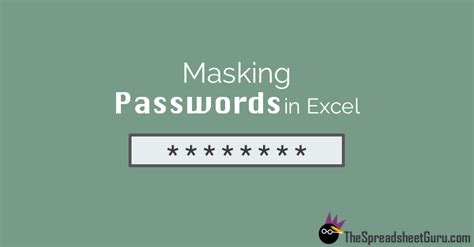
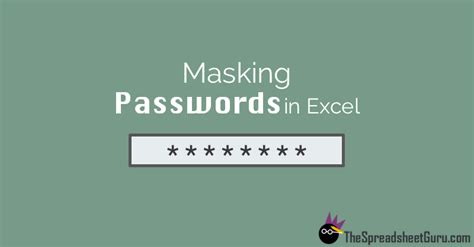
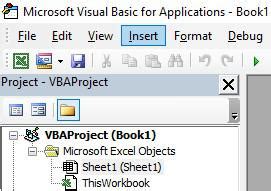
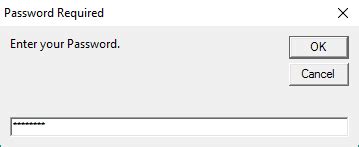
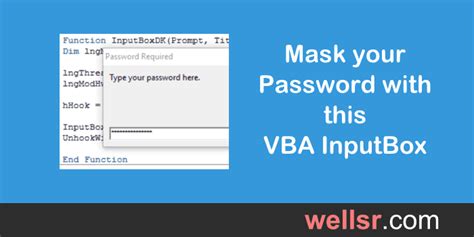