Intro
Automate repetitive tasks in Excel with a VBA macro that runs every 5 minutes. Learn how to schedule a macro to execute at regular intervals using Excel VBA programming. Discover the power of Application.OnTime and scheduling scripts to streamline workflows, boost productivity, and save time. Get expert tips on setting up and troubleshooting your VBA macro.
Running Excel macros automatically at regular intervals can be a great way to automate tasks, update data, or perform other repetitive actions. In this article, we'll explore how to run an Excel macro automatically every 5 minutes using VBA.
Why Run a Macro Automatically?
Before we dive into the details, let's quickly discuss why you might want to run a macro automatically. Here are a few scenarios:
- Updating data from external sources, such as databases or web APIs
- Performing calculations or analysis on a regular basis
- Sending reports or notifications to stakeholders
- Maintaining data consistency and accuracy
Setting Up the Macro
To run a macro automatically, you'll need to create the macro itself. Here's a simple example:
Sub UpdateData()
' Code to update data goes here
MsgBox "Data updated!"
End Sub
This macro, UpdateData
, simply displays a message box with the text "Data updated!". You can replace this code with your own logic to update data, perform calculations, or take other actions.
Using the `OnTime` Method
To run the macro automatically every 5 minutes, you can use the OnTime
method in VBA. This method allows you to schedule a macro to run at a specific time or after a certain delay.
Here's an example of how to use OnTime
to run the UpdateData
macro every 5 minutes:
Sub RunMacroEvery5Minutes()
Application.OnTime Now + TimeValue("00:05:00"), "UpdateData"
End Sub
This code schedules the UpdateData
macro to run 5 minutes from the current time. When the macro finishes running, you'll need to schedule the next run using the OnTime
method again. To do this, you can modify the UpdateData
macro to schedule the next run:
Sub UpdateData()
' Code to update data goes here
MsgBox "Data updated!"
Application.OnTime Now + TimeValue("00:05:00"), "UpdateData"
End Sub
Now, when the UpdateData
macro finishes running, it will schedule the next run 5 minutes from the current time.
Handling Errors and Interruptions
When running a macro automatically, it's essential to handle errors and interruptions gracefully. Here are a few tips:
- Use error handling to catch and log errors, so you can investigate and fix issues later.
- Use a try-catch block to catch specific exceptions and handle them accordingly.
- Consider using a backup or recovery mechanism to ensure data integrity in case of errors or interruptions.
Here's an updated version of the UpdateData
macro with basic error handling:
Sub UpdateData()
On Error GoTo ErrorHandler
' Code to update data goes here
MsgBox "Data updated!"
Application.OnTime Now + TimeValue("00:05:00"), "UpdateData"
Exit Sub
ErrorHandler:
MsgBox "Error occurred: " & Err.Description
' Log error or take other actions
End Sub
Security Considerations
When running macros automatically, it's crucial to consider security implications. Here are a few tips:
- Ensure that your macro is signed with a digital certificate to prevent security warnings.
- Use secure coding practices to avoid vulnerabilities and exploits.
- Limit the scope of the macro to the specific task or data it needs to access.
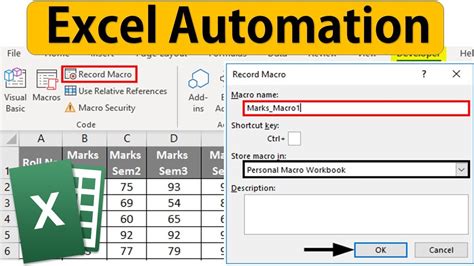
Best Practices and Additional Tips
Here are some best practices and additional tips to keep in mind:
- Test your macro thoroughly before scheduling it to run automatically.
- Use a consistent naming convention and organization for your macros and modules.
- Consider using a centralized logging mechanism to track macro execution and errors.
- Keep your macros up-to-date with the latest security patches and best practices.
Conclusion
In this article, we've explored how to run an Excel macro automatically every 5 minutes using VBA. We've covered the basics of setting up the macro, using the OnTime
method, handling errors and interruptions, and security considerations. By following these tips and best practices, you can automate tasks, update data, and perform other repetitive actions with ease.
Excel Macro Automation Image Gallery
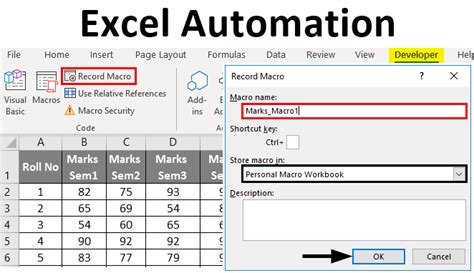
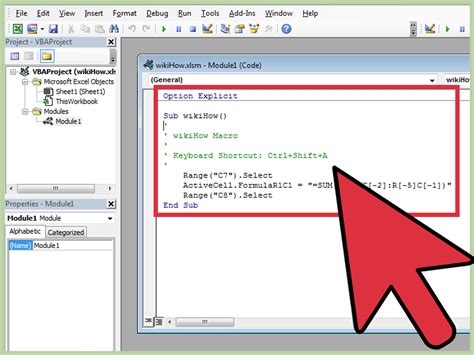
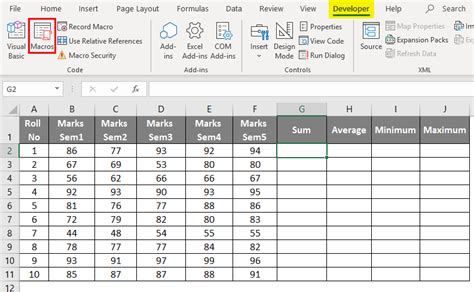
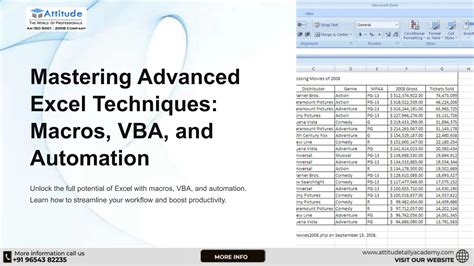
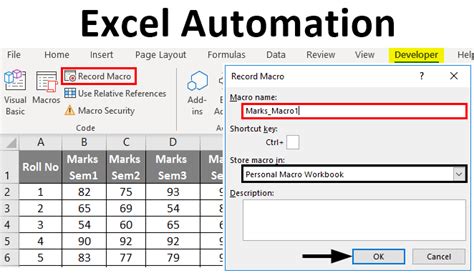
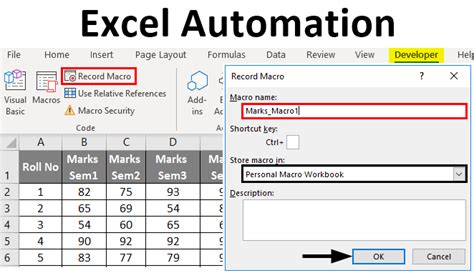
What's your experience with running Excel macros automatically? Share your tips and best practices in the comments below!