Working with Excel VBA can greatly enhance your productivity and automation capabilities within the Excel environment. One of the fundamental actions you might want to automate is creating new sheets. Whether you're managing large datasets, organizing reports, or simply streamlining your workflow, knowing how to add new sheets programmatically can be very useful. This guide will walk you through the steps and provide examples of how to create a new sheet in Excel VBA easily.
Understanding Excel VBA Basics
Before diving into the specifics of creating new sheets, ensure you have a basic understanding of Excel VBA. VBA stands for Visual Basic for Applications, and it's the programming language used to create and automate tasks in Microsoft Office applications, including Excel.
-
Accessing the VBA Editor: To start working with VBA in Excel, press
Alt + F11
or navigate toDeveloper
>Visual Basic
in the ribbon. If you don't see the Developer tab, you can add it by going toFile
>Options
>Customize Ribbon
, then check theDeveloper
checkbox. -
Writing VBA Code: VBA code is written in modules. You can insert a new module by right-clicking on any of the objects for your workbook listed in the Project Explorer on the left side of the VBA Editor, then choosing
Insert
>Module
.
Creating a New Sheet Using VBA
Here's a simple example of how to create a new sheet using VBA:
Sub CreateNewSheet()
' Declare a variable to hold the new worksheet
Dim newSheet As Worksheet
' Create a new worksheet and assign it to the variable
Set newSheet = ThisWorkbook.Worksheets.Add
' Name the new worksheet
newSheet.Name = "MyNewSheet"
End Sub
To run this code, simply open the VBA Editor, paste the code into a module, then press F5
or click Run
> Run Sub/UserForm
. This will create a new sheet named "MyNewSheet" in your workbook.
Advanced Options for Creating Sheets
Sometimes, you might need more control over the creation process. Here are a few advanced options:
- Specifying the Position: You can specify where the new sheet should be inserted. For example, to insert a new sheet before the first sheet in your workbook, you can modify the code like this:
Sub CreateNewSheetBeforeFirst()
ThisWorkbook.Worksheets.Add Before:=ThisWorkbook.Worksheets(1)
End Sub
- Creating Multiple Sheets: If you need to create multiple sheets at once, you can use a loop. Here's an example:
Sub CreateMultipleSheets()
Dim i As Integer
For i = 1 To 5
ThisWorkbook.Worksheets.Add.Name = "Sheet" & i
Next i
End Sub
This code will create five new sheets named "Sheet1", "Sheet2", etc.
- Error Handling: When automating tasks, it's a good practice to include error handling to ensure your program doesn't crash if something unexpected happens. For example, if the user tries to create a sheet with a name that already exists, you might want to handle that situation gracefully.
Sub CreateNewSheetWithErrorHandling()
Dim newSheet As Worksheet
Dim sheetName As String
sheetName = "MyNewSheet"
On Error Resume Next
Set newSheet = ThisWorkbook.Worksheets.Add
newSheet.Name = sheetName
If Err.Number <> 0 Then
MsgBox "A sheet with the name '" & sheetName & "' already exists.", vbExclamation
Err.Clear
End If
On Error GoTo 0
End Sub
Conclusion
Creating new sheets in Excel using VBA is a straightforward process that can greatly enhance your productivity. By understanding how to automate this task, you can simplify your workflow and focus on more complex aspects of your work. Whether you're a beginner or an advanced user, mastering VBA can open up new possibilities for managing and analyzing your data in Excel.
Encourage Engagement
If you have any questions about creating new sheets in Excel VBA or need further assistance with a specific task, feel free to ask in the comments. Sharing your experiences and tips with VBA can also help others in the community, so don't hesitate to contribute. Let's explore the world of Excel VBA together!
Gallery of Excel VBA Images
Excel VBA Gallery
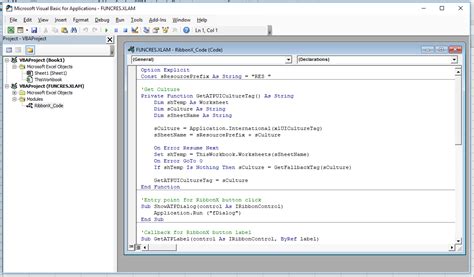
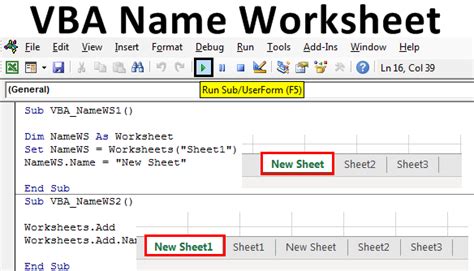


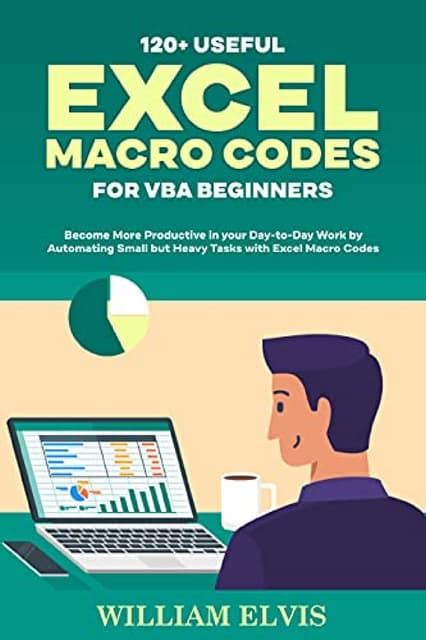
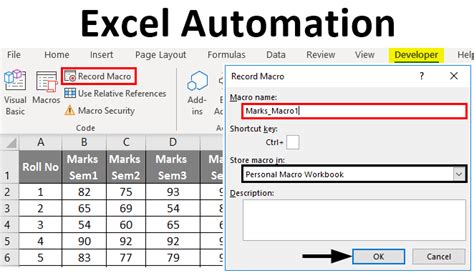
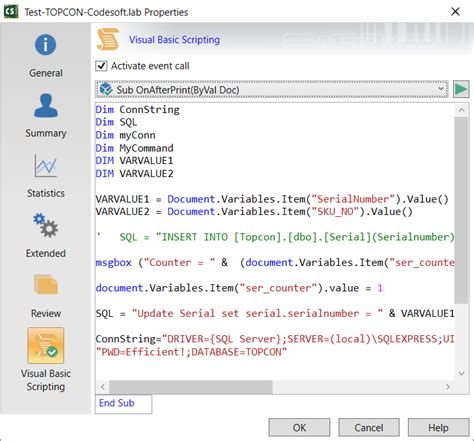
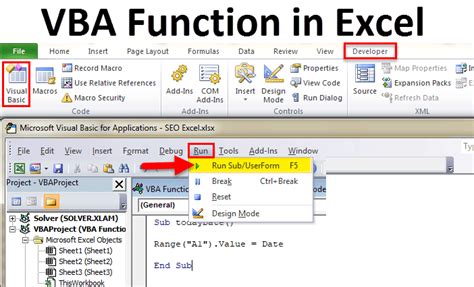
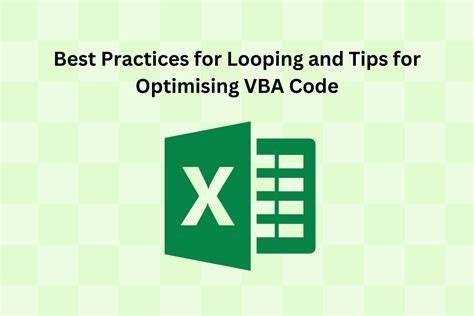
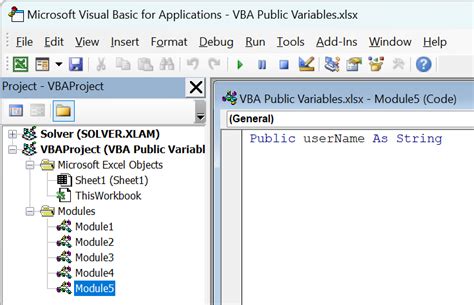