Intro
Unlock the full potential of VBA programming with these 5 expert-approved methods to return value in VBA. Discover how to harness the power of user-defined functions, error handling, and data manipulation to create efficient and effective VBA code, driving business success through automation and data analysis.
Returning values in VBA is a crucial aspect of creating reusable and efficient code. Whether you're a beginner or an experienced programmer, understanding the different methods to return values can help you write better code and achieve your desired outcomes. In this article, we'll explore five ways to return values in VBA, along with examples and best practices to help you get the most out of your code.
Why Return Values Matter
Before we dive into the methods, it's essential to understand why returning values is important. In VBA, functions and subroutines are the building blocks of your code. When you write a function or subroutine, you want to be able to reuse it and make it flexible enough to handle different inputs and scenarios. Returning values allows you to achieve this flexibility and make your code more modular.
Method 1: Using the Return Statement
The most straightforward way to return a value in VBA is by using the Return statement. This statement is used to exit a function or subroutine and return a value to the calling procedure.
Example:
Function AddNumbers(a As Integer, b As Integer) As Integer
AddNumbers = a + b
End Function
In this example, the AddNumbers
function takes two integers as input, adds them together, and returns the result using the Return statement.
Method 2: Using the Function Name
Another way to return a value in VBA is by assigning the result to the function name. This method is commonly used when you need to return multiple values or complex data types.
Example:
Function GetEmployeeData() As Variant
GetEmployeeData = Array("John Doe", 30, "Sales")
End Function
In this example, the GetEmployeeData
function returns an array containing the employee's name, age, and department.
Method 3: Using a ByRef Parameter
You can also return a value in VBA by passing a variable as a ByRef parameter to a subroutine. This method is useful when you need to return multiple values or modify the input parameters.
Example:
Sub CalculateArea(length As Double, width As Double, ByRef area As Double)
area = length * width
End Sub
In this example, the CalculateArea
subroutine takes two input parameters (length and width) and returns the calculated area through the ByRef parameter.
Method 4: Using a Class Property
If you're working with classes in VBA, you can return values by exposing properties. This method is useful when you need to return complex data types or objects.
Example:
Class Employee
Private mName As String
Private mAge As Integer
Public Property Get Name() As String
Name = mName
End Property
Public Property Get Age() As Integer
Age = mAge
End Property
End Class
In this example, the Employee
class exposes two properties (Name and Age) that can be accessed through getters.
Method 5: Using a Collection or Array
Finally, you can return values in VBA by using collections or arrays. This method is useful when you need to return multiple values or a list of items.
Example:
Function GetEmployees() As Collection
Dim employees As New Collection
employees.Add "John Doe"
employees.Add "Jane Doe"
GetEmployees = employees
End Function
In this example, the GetEmployees
function returns a collection of employee names.
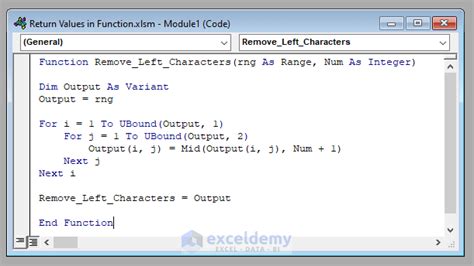
Best Practices
When returning values in VBA, keep the following best practices in mind:
- Use the Return statement to exit a function or subroutine and return a value.
- Use the function name to return values when working with complex data types or multiple returns.
- Pass variables as ByRef parameters to subroutines when you need to return multiple values or modify input parameters.
- Expose properties in classes to return complex data types or objects.
- Use collections or arrays to return multiple values or lists of items.
By following these best practices and using the methods outlined in this article, you can write more efficient and effective VBA code that returns values correctly.
Gallery of VBA Return Value Examples
VBA Return Value Examples
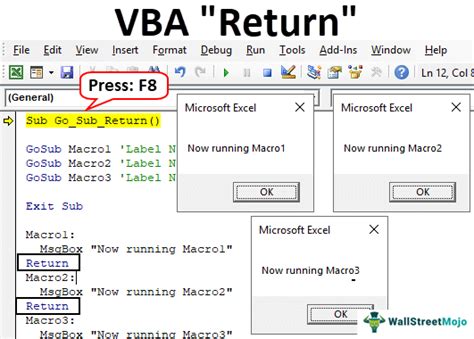
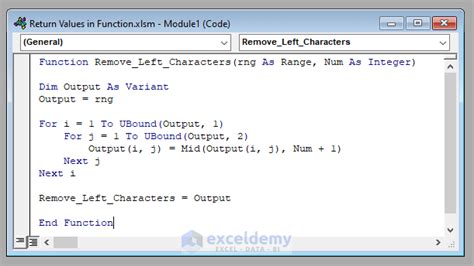
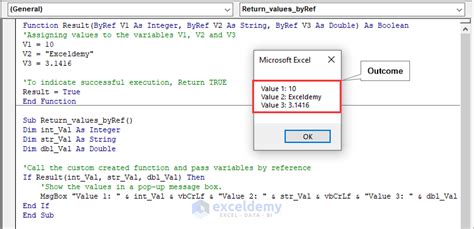
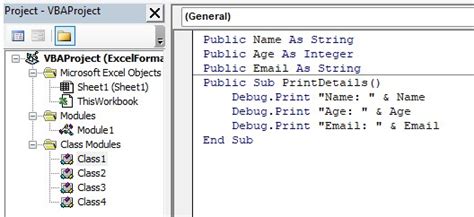
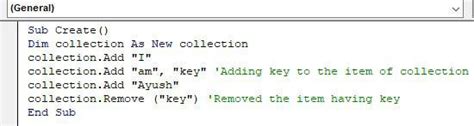
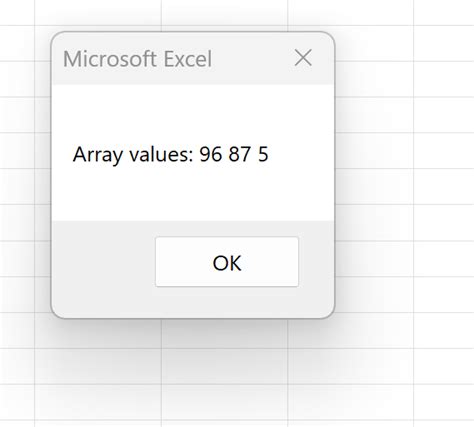
FAQ
Q: What is the difference between a function and a subroutine in VBA? A: A function returns a value, while a subroutine does not.
Q: How do I return multiple values from a VBA function? A: You can use a ByRef parameter, a class property, or a collection/array to return multiple values.
Q: What is the purpose of the Return statement in VBA? A: The Return statement is used to exit a function or subroutine and return a value to the calling procedure.
Q: Can I use a function name to return a value in VBA? A: Yes, you can assign the result to the function name to return a value.
We hope this article has helped you understand the different ways to return values in VBA. Whether you're a beginner or an experienced programmer, mastering these methods will help you write more efficient and effective code. If you have any questions or need further clarification, please don't hesitate to ask.