Intro
Discover how to create a new Excel sheet with VBA made easy. Learn step-by-step techniques to automate worksheet creation, customize sheet properties, and enhance your workflow. Master VBA coding for Excel sheet generation, manipulation, and formatting, and boost your productivity with these actionable tips and tricks.
Creating a new Excel sheet can be a daunting task, especially for those who are new to VBA programming. However, with the right guidance, it can be made easy. In this article, we will explore the basics of creating a new Excel sheet using VBA and provide step-by-step instructions to help you get started.
Why Use VBA to Create a New Excel Sheet?
Before we dive into the world of VBA programming, let's first discuss why you might want to use VBA to create a new Excel sheet. VBA, or Visual Basic for Applications, is a programming language that allows you to automate tasks in Excel. By using VBA, you can create custom macros that can perform a variety of tasks, such as formatting cells, inserting charts, and even creating new worksheets.
One of the main advantages of using VBA to create a new Excel sheet is that it allows you to automate the process. This can be especially useful if you need to create multiple sheets with the same layout and formatting. With VBA, you can write a macro that creates a new sheet and applies the desired formatting, saving you time and effort.
Getting Started with VBA
To get started with VBA, you'll need to open the Visual Basic Editor in Excel. To do this, follow these steps:
- Open Excel and select the workbook where you want to create a new sheet.
- Press Alt + F11 to open the Visual Basic Editor.
- In the Visual Basic Editor, click on "Insert" and select "Module" to create a new module.
Creating a New Excel Sheet with VBA
Now that we have the Visual Basic Editor open, let's create a new Excel sheet using VBA. Here's an example code that creates a new sheet:
Sub CreateNewSheet()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets.Add
ws.Name = "My New Sheet"
End Sub
Let's break down this code:
Sub CreateNewSheet()
: This line declares a new subroutine calledCreateNewSheet
.Dim ws As Worksheet
: This line declares a variablews
as a worksheet object.Set ws = ThisWorkbook.Worksheets.Add
: This line creates a new worksheet and assigns it to thews
variable.ws.Name = "My New Sheet"
: This line sets the name of the new worksheet to "My New Sheet".
Running the Macro
To run the macro, follow these steps:
- In the Visual Basic Editor, click on "Run" and select "Run Sub/UserForm" or press F5.
- The macro will create a new sheet in your workbook with the name "My New Sheet".
Customizing the Macro
Now that we have a basic macro that creates a new sheet, let's customize it to apply some formatting. Here's an updated code:
Sub CreateNewSheet()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets.Add
ws.Name = "My New Sheet"
ws.Range("A1").Value = "My New Sheet"
ws.Range("A1").Font.Bold = True
ws.Range("A1").Font.Size = 18
End Sub
This updated code adds a title to the new sheet and applies some formatting to the title.
Adding Multiple Sheets
If you need to create multiple sheets with the same layout and formatting, you can modify the macro to create multiple sheets. Here's an example code:
Sub CreateMultipleSheets()
Dim ws As Worksheet
Dim i As Integer
For i = 1 To 5
Set ws = ThisWorkbook.Worksheets.Add
ws.Name = "Sheet " & i
ws.Range("A1").Value = "Sheet " & i
ws.Range("A1").Font.Bold = True
ws.Range("A1").Font.Size = 18
Next i
End Sub
This code creates 5 new sheets with the same layout and formatting.
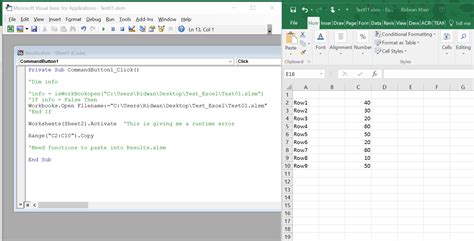
Gallery of Excel VBA Examples
Excel VBA Examples
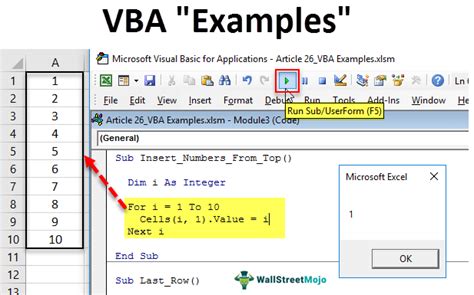
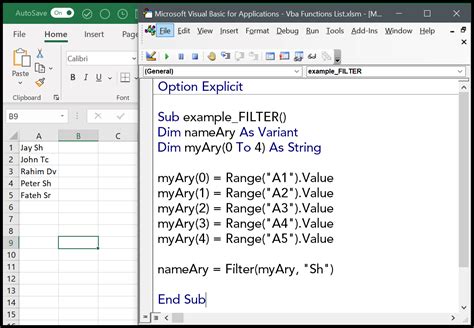
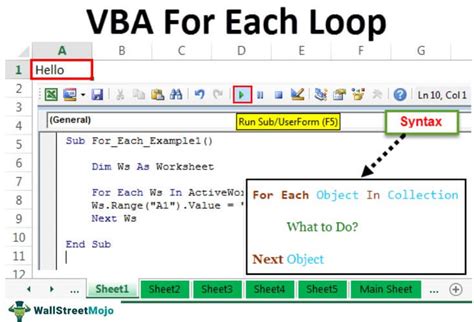
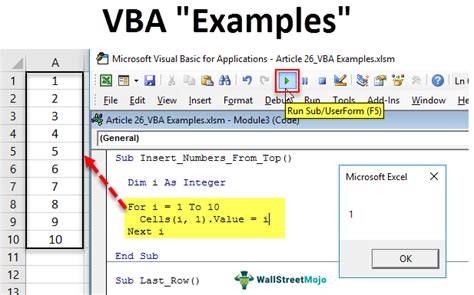
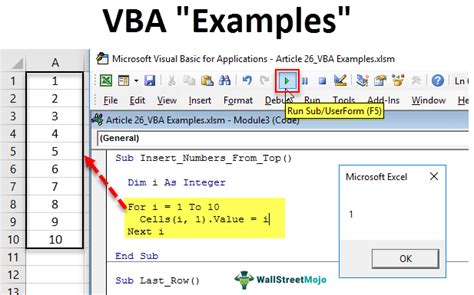
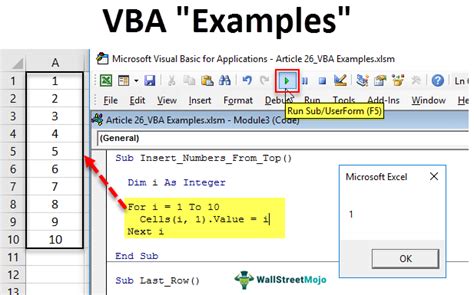
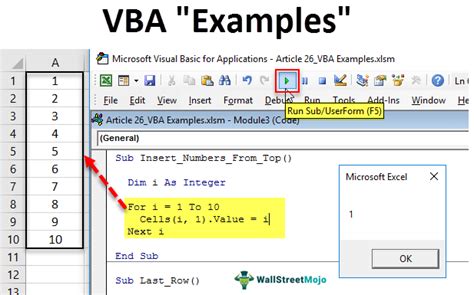
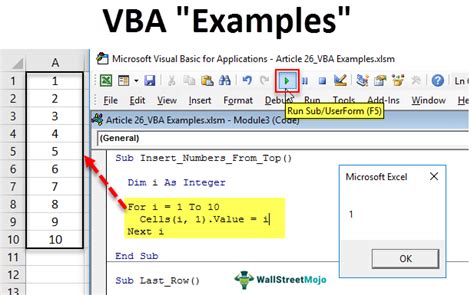
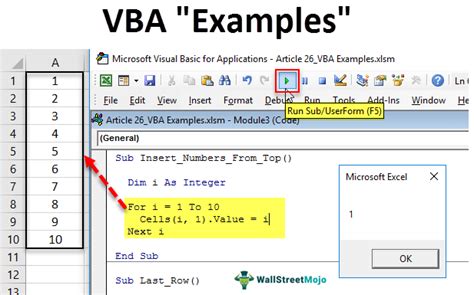
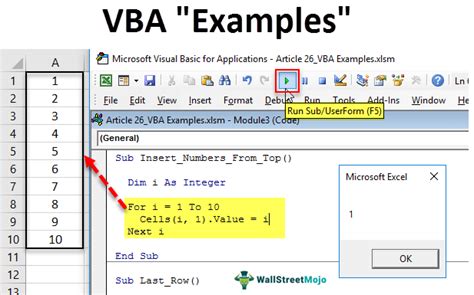
Conclusion
Creating a new Excel sheet with VBA is a powerful way to automate tasks and improve productivity. By following the steps outlined in this article, you can create custom macros that create new sheets with the desired layout and formatting. Remember to experiment with different VBA codes and examples to improve your skills and become more efficient in your work.
Final Thoughts
Creating a new Excel sheet with VBA is just the beginning. With the skills you've learned in this article, you can now explore more advanced VBA techniques, such as creating custom charts and tables, automating data analysis, and even integrating Excel with other Microsoft Office applications.
Don't be afraid to experiment and try new things. With practice and patience, you'll become a VBA expert in no time!
Frequently Asked Questions
Q: What is VBA and why should I use it? A: VBA, or Visual Basic for Applications, is a programming language that allows you to automate tasks in Excel. By using VBA, you can create custom macros that can perform a variety of tasks, such as formatting cells, inserting charts, and even creating new worksheets.
Q: How do I open the Visual Basic Editor in Excel? A: To open the Visual Basic Editor in Excel, press Alt + F11 or navigate to Developer > Visual Basic in the ribbon.
Q: Can I create multiple sheets with the same layout and formatting using VBA? A: Yes, you can modify the macro to create multiple sheets with the same layout and formatting.