Adjusting column widths in Excel VBA can significantly enhance the readability and overall presentation of your worksheets. Whether you're working with data that requires precise column adjustments or aiming to automate formatting tasks, knowing how to manipulate column widths programmatically is a valuable skill. In this article, we'll delve into five ways to adjust VBA Excel column widths, providing you with the tools to efficiently manage your spreadsheet's layout.
Understanding the Importance of Column Widths
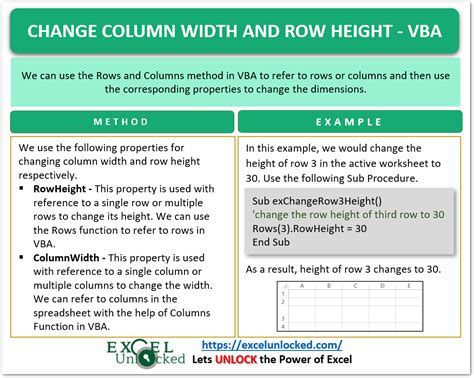
Column widths play a crucial role in Excel. Properly adjusted columns can make your data more readable, reduce the need for horizontal scrolling, and improve the overall aesthetics of your worksheet. While Excel provides manual methods for adjusting column widths, using VBA can offer more precision and efficiency, especially when dealing with large datasets or repetitive tasks.
Method 1: Adjusting Column Widths Using the Range Object
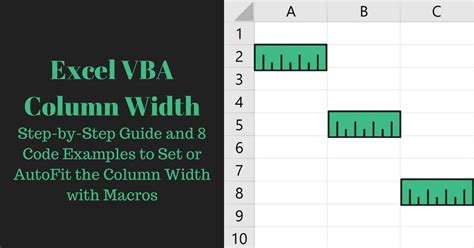
One of the most straightforward ways to adjust column widths in VBA is by using the Range
object. This method allows you to specify the exact width of a column in points.
Sub AdjustColumnWidthRangeObject()
' Adjust the width of column A to 15 points
Columns("A").ColumnWidth = 15
End Sub
This code snippet adjusts the width of column A to 15 points. You can replace "A"
with any column letter or number you wish to adjust.
Method 2: Auto-Fitting Column Widths
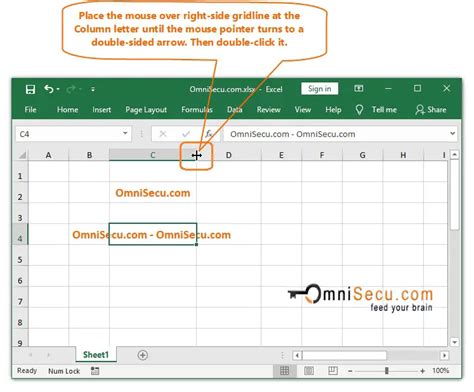
Auto-fitting is a convenient way to adjust column widths based on the content. This method ensures that columns are wide enough to display their contents without unnecessary whitespace.
Sub AutoFitColumnWidth()
' Auto-fit the width of column A
Columns("A").AutoFit
End Sub
This code auto-fits the width of column A, adjusting it to perfectly fit the content.
Method 3: Using the `Cells` Object for Column Width Adjustment
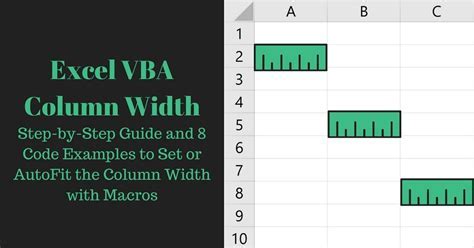
The Cells
object can also be used to adjust column widths. This method is particularly useful when you need to adjust the width of multiple columns at once.
Sub AdjustColumnWidthCellsObject()
' Adjust the width of columns A to C to 12 points
Range("A:C").ColumnWidth = 12
End Sub
This code adjusts the width of columns A to C to 12 points.
Method 4: Dynamically Adjusting Column Widths Based on Content
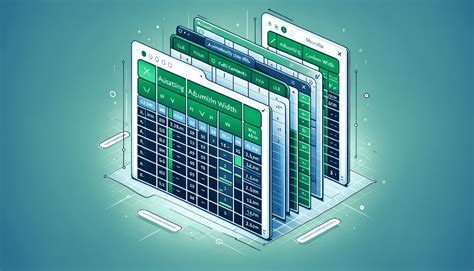
Sometimes, you might need to adjust column widths dynamically based on the content of specific cells. This can be achieved by reading the content of the cells and adjusting the column widths accordingly.
Sub DynamicColumnWidth()
Dim ws As Worksheet
Set ws = ActiveSheet
Dim lastRow As Long
lastRow = ws.Cells(ws.Rows.Count, "A").End(xlUp).Row
For i = 1 To lastRow
If Len(ws.Cells(i, 1).Value) > 10 Then
ws.Columns("A").ColumnWidth = 15
Else
ws.Columns("A").ColumnWidth = 10
End If
Next i
End Sub
This code checks the length of the content in column A and adjusts the column width to either 10 or 15 points based on the content length.
Method 5: Adjusting Column Widths Across Multiple Worksheets
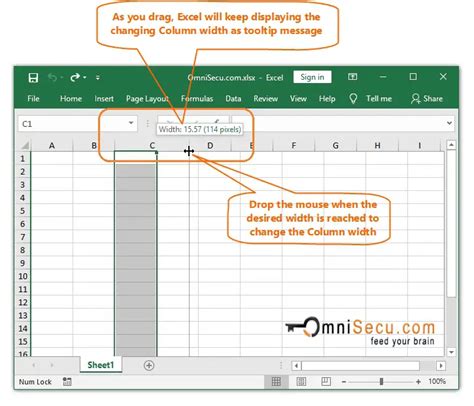
In scenarios where you need to apply uniform column widths across multiple worksheets in a workbook, VBA provides an efficient solution.
Sub UniformColumnWidthAcrossWorksheets()
Dim ws As Worksheet
For Each ws In ThisWorkbook.Worksheets
ws.Columns("A").ColumnWidth = 12
Next ws
End Sub
This code adjusts the width of column A to 12 points across all worksheets in the active workbook.
Gallery of Adjusting VBA Excel Column Widths
Column Width Adjustment in Excel VBA
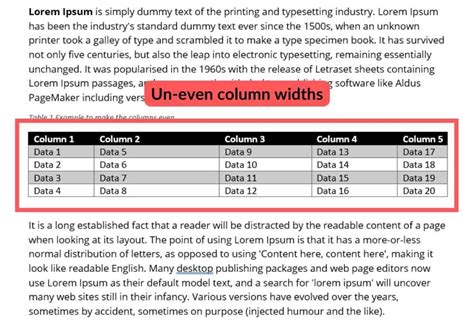
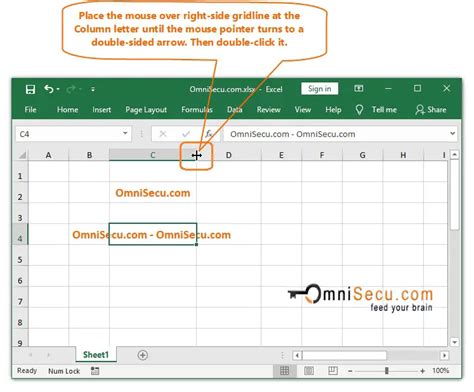
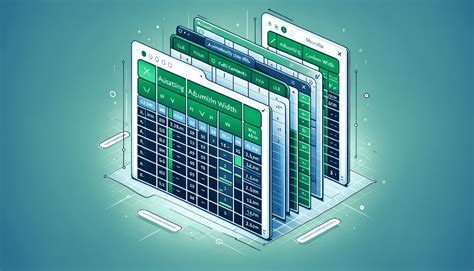
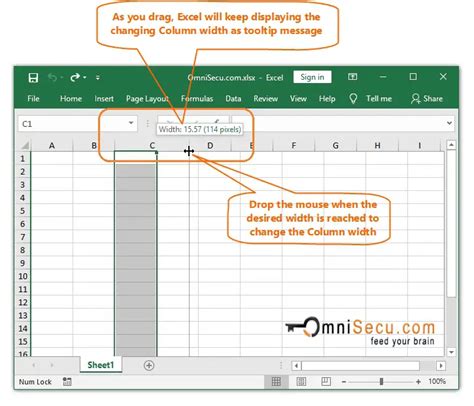
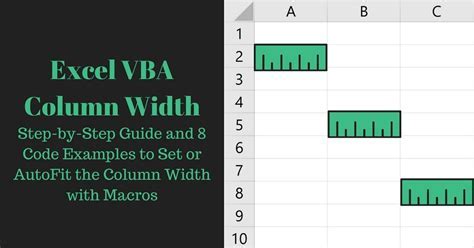
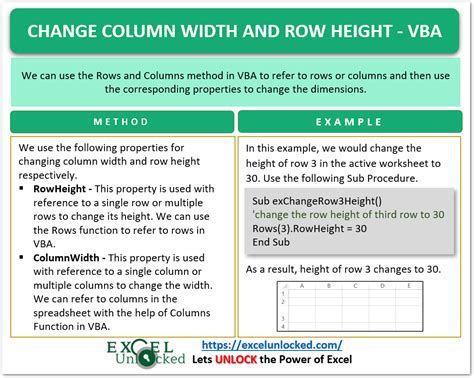
In conclusion, mastering the art of adjusting column widths in Excel VBA can significantly improve your productivity and the presentation of your worksheets. Whether you're auto-fitting, using the Range
or Cells
objects, adjusting dynamically, or applying uniform widths across multiple worksheets, VBA offers flexible and efficient methods to meet your needs. Experiment with these techniques to enhance your Excel VBA skills and improve your spreadsheet management.