Intro
Learn how to automate file management with VBA code. Discover the easy way to kill a file if it exists using VBA, streamlining your workflow and saving time. Master file handling techniques, error checking, and script optimization with our expert guidance and examples. Boost productivity with efficient VBA coding.
Killing a file if it exists in VBA can be a straightforward process. Here's a comprehensive guide to help you understand the importance of managing files and how to easily delete them if they exist using VBA.
In various scenarios, especially in data analysis, automation, or reporting, you might need to manage files that are generated or utilized by your VBA applications. Sometimes, these files need to be deleted if they already exist to avoid version conflicts, to maintain a clean directory, or to prepare for new data imports. This is where knowing how to kill a file if it exists in VBA becomes crucial.
Why Managing Files in VBA is Important
Managing files effectively in VBA can significantly improve the efficiency and reliability of your applications. Here are a few reasons why:
- Data Integrity: Automatically deleting old files can help maintain data integrity by ensuring that only the latest versions of files are used.
- Version Control: Deleting existing files can help in version control, ensuring that your application always uses the most recent data or configurations.
- Clean Workspace: Keeping your directories clean can improve the performance of your applications and make maintenance easier.
How to Kill a File If It Exists in VBA
To delete a file if it exists in VBA, you can use the Kill
statement. However, it's good practice to first check if the file exists to avoid errors. Here's how you can do it:
Sub DeleteFileIfExists()
Dim filePath As String
filePath = "C:\path\to\your\file.txt" 'Change this to the path of your file
If Dir(filePath) <> "" Then
'File exists, so delete it
Kill filePath
MsgBox "File deleted successfully.", vbInformation
Else
MsgBox "File does not exist.", vbInformation
End If
End Sub
This code snippet checks if the specified file exists using the Dir
function. If the file exists (Dir(filePath) <> ""
), it uses the Kill
statement to delete the file. A message box then pops up to inform you whether the file was deleted or if it didn't exist in the first place.
Practical Examples and Variations
Here are a few variations and examples to help you understand how to adapt this code to different scenarios:
Deleting Multiple Files
If you need to delete multiple files at once, you can list all the file paths in an array and loop through them.
Sub DeleteMultipleFiles()
Dim filePaths() As String
filePaths = Array("C:\file1.txt", "C:\file2.txt", "C:\file3.txt")
Dim filePath As Variant
For Each filePath In filePaths
If Dir(filePath) <> "" Then
Kill filePath
Debug.Print filePath & " deleted."
Else
Debug.Print filePath & " does not exist."
End If
Next filePath
End Sub
Deleting Files in a Specific Directory
If you want to delete all files in a specific directory (but not subdirectories), you can use the Dir
function to loop through all files.
Sub DeleteFilesInDirectory()
Dim directoryPath As String
directoryPath = "C:\your\directory\"
Dim fileName As String
fileName = Dir(directoryPath & "*.*")
Do While fileName <> ""
Kill directoryPath & fileName
Debug.Print fileName & " deleted."
fileName = Dir
Loop
End Sub
Error Handling
It's a good practice to include error handling when deleting files, especially if the file is in use or if there are permissions issues.
Sub DeleteFileWithErrorHandling()
On Error Resume Next
Kill "C:\path\to\your\file.txt"
If Err.Number <> 0 Then
MsgBox "Error deleting file: " & Err.Description, vbCritical
Else
MsgBox "File deleted successfully.", vbInformation
End If
On Error GoTo 0
End Sub
Gallery of VBA File Management
VBA File Management Image Gallery
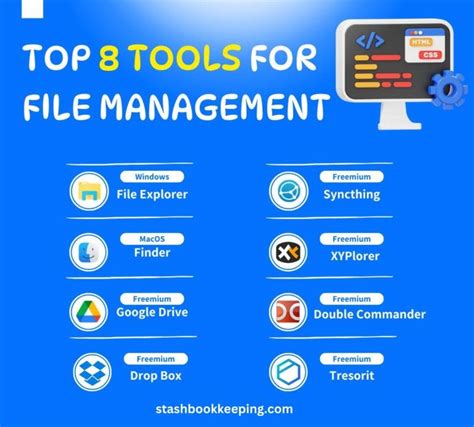
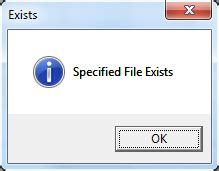
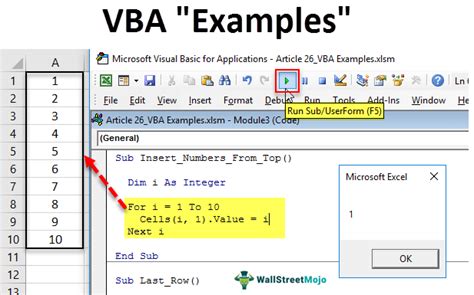
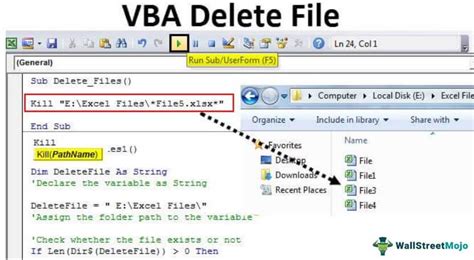
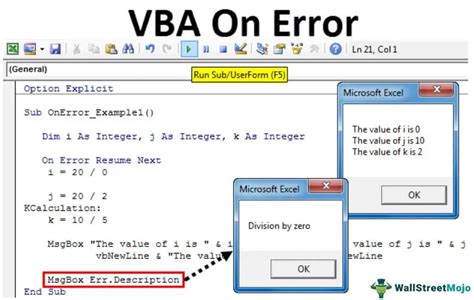
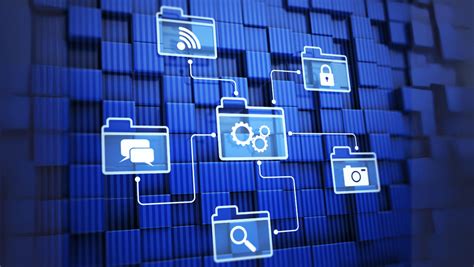
In conclusion, managing files in VBA, including deleting them if they exist, is a fundamental skill for any VBA developer. By understanding how to use the Kill
statement effectively and how to handle errors, you can create more robust and efficient VBA applications. Remember to always test your code in a safe environment to avoid unintended data loss.
Feel free to ask any questions or share your experiences with managing files in VBA in the comments section below!