Intro
Unlock the power of VBA with our expert guide on how to clear clipboard using VBA. Discover four efficient methods to delete clipboard contents, including Range, DataObject, and API calls. Learn how to automate clipboard management and boost productivity in Excel VBA. Master clipboard clearing techniques with our step-by-step tutorial.
The humble clipboard. It's a fundamental tool in our daily computing lives, allowing us to copy and paste text, images, and other data with ease. However, when working with VBA (Visual Basic for Applications), the clipboard can sometimes become a hindrance, especially when dealing with sensitive data or trying to automate tasks. In this article, we'll explore four ways to clear the clipboard using VBA, helping you maintain control and security over your data.
Why Clear the Clipboard?
Before we dive into the methods, let's quickly discuss why clearing the clipboard is important. When you copy data, it's stored in the clipboard, which can be accessed by other applications or even malicious code. This poses a significant risk, especially when working with sensitive information like passwords, credit card numbers, or confidential business data. By clearing the clipboard, you ensure that this sensitive data is removed, reducing the risk of unauthorized access.
Method 1: Using the MSForms.DataObject
The first method involves using the MSForms.DataObject, which is a part of the Microsoft Forms 2.0 Object Library. This object allows you to interact with the clipboard, including clearing its contents.
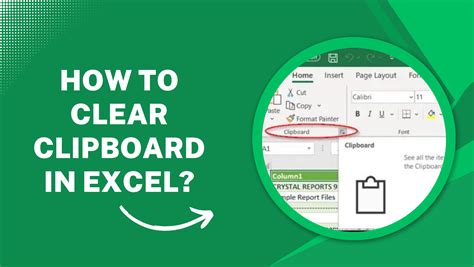
To use this method, you'll need to add a reference to the Microsoft Forms 2.0 Object Library in your VBA project. Here's the code:
Sub ClearClipboard_MSForms()
Dim dataObj As New MSForms.DataObject
dataObj.Clear
End Sub
Method 2: Using the Windows API
The second method involves using the Windows API (Application Programming Interface) to clear the clipboard. This method is more complex, but it provides a high degree of control over the clipboard.
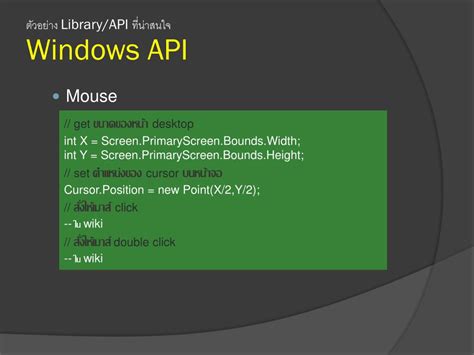
To use this method, you'll need to declare the necessary API functions and variables. Here's the code:
Declare Function OpenClipboard Lib "user32" (ByVal hwnd As Long) As Long
Declare Function EmptyClipboard Lib "user32" () As Long
Declare Function CloseClipboard Lib "user32" () As Long
Sub ClearClipboard_WindowsAPI()
Dim hwnd As Long
hwnd = 0
OpenClipboard hwnd
EmptyClipboard
CloseClipboard
End Sub
Method 3: Using Late Binding
The third method involves using late binding to clear the clipboard. This method is similar to the first method, but it doesn't require a reference to the Microsoft Forms 2.0 Object Library.
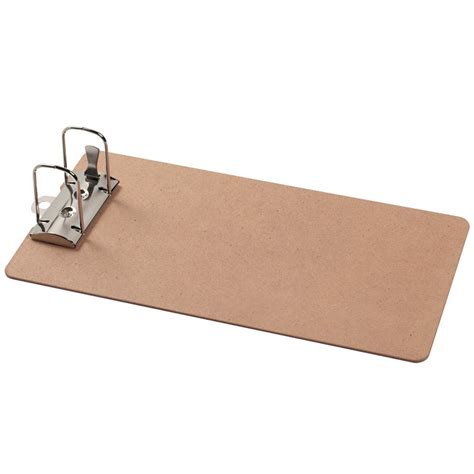
Here's the code:
Sub ClearClipboard_LateBinding()
Dim dataObj As Object
Set dataObj = CreateObject("new:{1C3B4210-F441-11CE-B9EA-00AA006B1A69}")
dataObj.Clear
Set dataObj = Nothing
End Sub
Method 4: Using the Shell
The fourth method involves using the Shell object to clear the clipboard. This method is the simplest of the four, but it may not be as effective as the other methods.
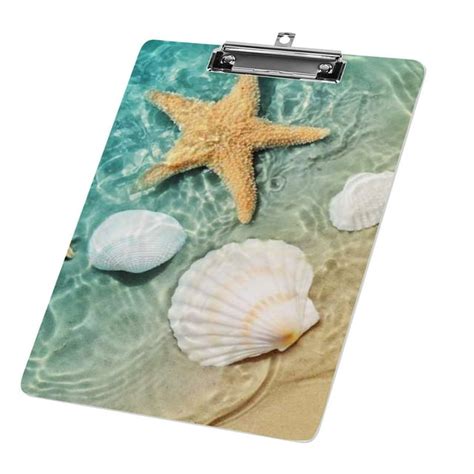
Here's the code:
Sub ClearClipboard_Shell()
Dim shell As Object
Set shell = CreateObject("WScript.Shell")
shell.Run "cmd /c echo off | clip", 0, False
Set shell = Nothing
End Sub
Gallery of Clipboard-Related Images
Clipboard Image Gallery
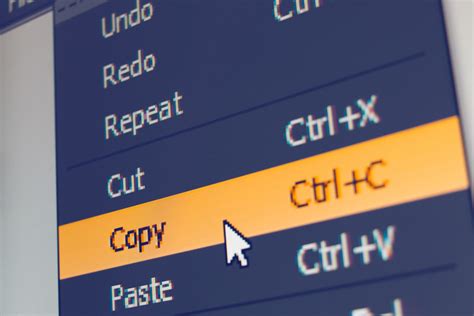
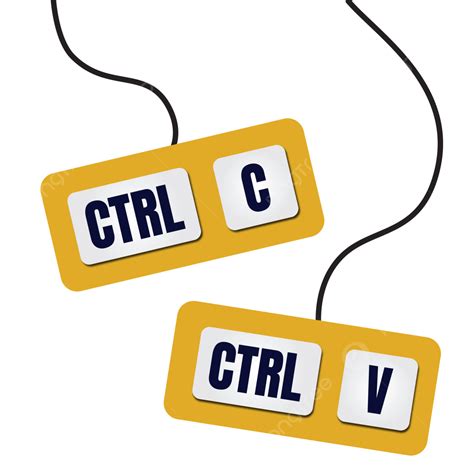

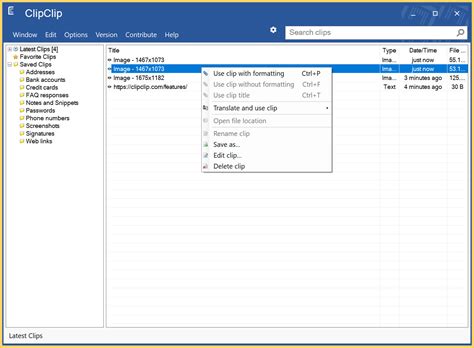
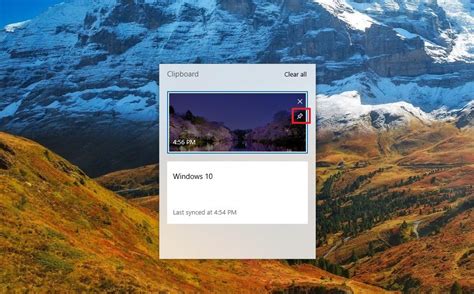
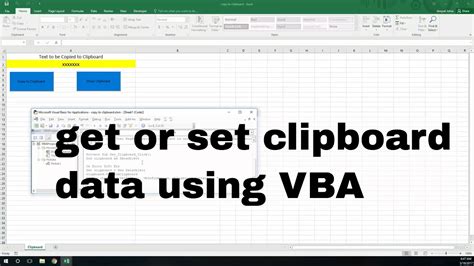
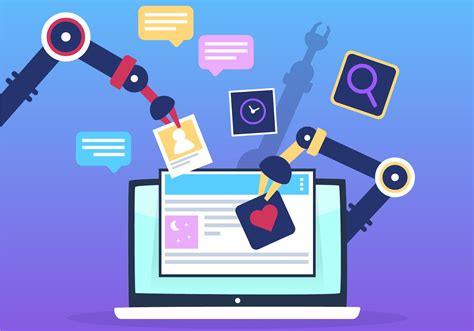
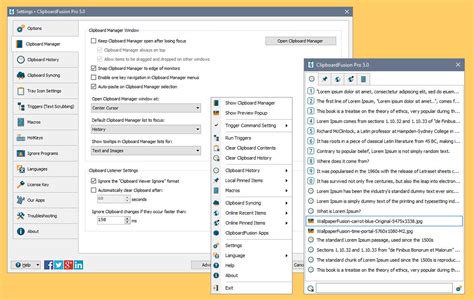
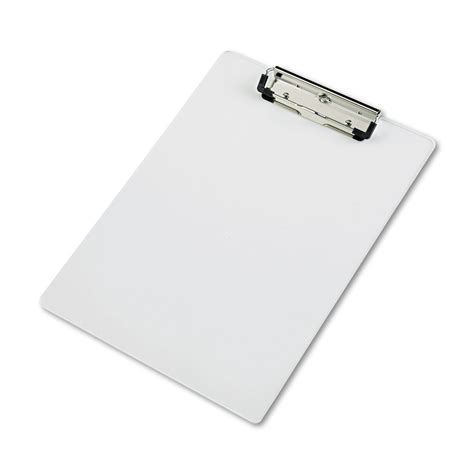
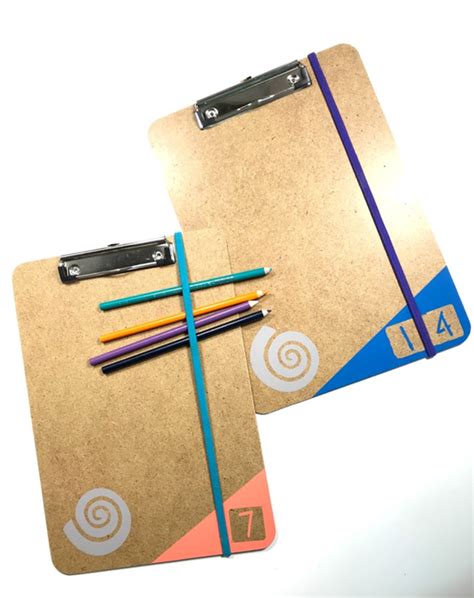
Final Thoughts
In conclusion, clearing the clipboard is an essential task when working with sensitive data or automating tasks in VBA. The four methods outlined in this article provide a range of options for clearing the clipboard, from simple to complex. By using these methods, you can ensure that your data is secure and your automated tasks run smoothly. Do you have any questions or comments about clearing the clipboard in VBA? Share your thoughts in the comments section below!