Creating a new workbook using VBA can be a useful skill for automating tasks and increasing productivity in Excel. VBA, or Visual Basic for Applications, is a programming language used to create and automate tasks in Microsoft Office applications, including Excel. Here's a comprehensive guide on how to create a new workbook using VBA.
Why Use VBA to Create a New Workbook?
Before we dive into the code, let's explore why you might want to use VBA to create a new workbook. Here are a few reasons:
- Automation: VBA allows you to automate repetitive tasks, such as creating a new workbook with a specific layout or formatting.
- Consistency: By using VBA to create a new workbook, you can ensure that all workbooks have a consistent layout and formatting.
- Speed: VBA can create a new workbook much faster than doing it manually.
Basic VBA Code to Create a New Workbook
Here's the basic VBA code to create a new workbook:
Sub CreateNewWorkbook()
Workbooks.Add
End Sub
This code creates a new workbook with a single worksheet. The Workbooks.Add
method creates a new workbook and adds it to the Workbooks
collection.
Creating a New Workbook with a Specific Name
If you want to create a new workbook with a specific name, you can use the following code:
Sub CreateNewWorkbookWithName()
Dim newWorkbook As Workbook
Set newWorkbook = Workbooks.Add
newWorkbook.SaveAs "MyNewWorkbook.xlsx"
End Sub
This code creates a new workbook and saves it with the name "MyNewWorkbook.xlsx". You can replace "MyNewWorkbook.xlsx" with any name you like.
Creating a New Workbook with Multiple Worksheets
If you want to create a new workbook with multiple worksheets, you can use the following code:
Sub CreateNewWorkbookWithMultipleWorksheets()
Dim newWorkbook As Workbook
Set newWorkbook = Workbooks.Add
newWorkbook.Worksheets.Add.Count = 5
End Sub
This code creates a new workbook with 5 worksheets. You can adjust the number of worksheets to suit your needs.
Adding Formatting to a New Workbook
If you want to add formatting to a new workbook, you can use the following code:
Sub CreateNewWorkbookWithFormatting()
Dim newWorkbook As Workbook
Set newWorkbook = Workbooks.Add
newWorkbook.Worksheets(1).Range("A1").Value = "Hello World!"
newWorkbook.Worksheets(1).Range("A1").Font.Bold = True
End Sub
This code creates a new workbook and adds formatting to the first worksheet, including a bold font and a value of "Hello World!" in cell A1.
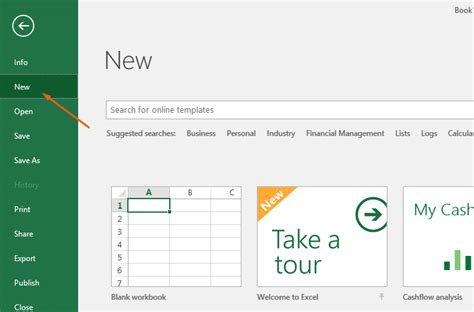
Practical Examples of Creating a New Workbook Using VBA
Here are some practical examples of creating a new workbook using VBA:
- Creating a new workbook for a specific project or client
- Creating a new workbook with a specific layout or formatting for a report or presentation
- Creating a new workbook with multiple worksheets for a complex analysis or model
Tips and Tricks for Creating a New Workbook Using VBA
Here are some tips and tricks for creating a new workbook using VBA:
- Use the
Workbooks.Add
method to create a new workbook. - Use the
SaveAs
method to save the new workbook with a specific name. - Use the
Worksheets.Add
method to add multiple worksheets to the new workbook. - Use formatting methods, such as
Font.Bold
orRange.Value
, to add formatting to the new workbook.
Gallery of VBA Code Examples
VBA Code Examples Gallery
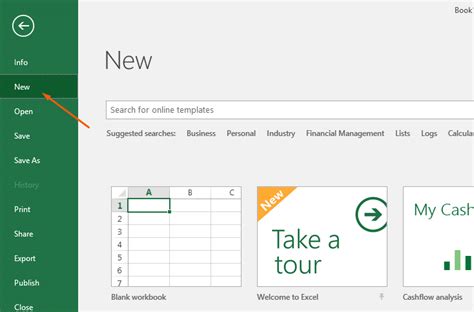
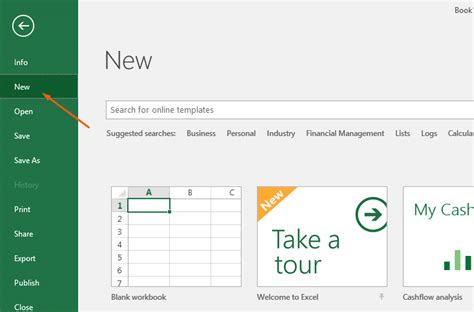
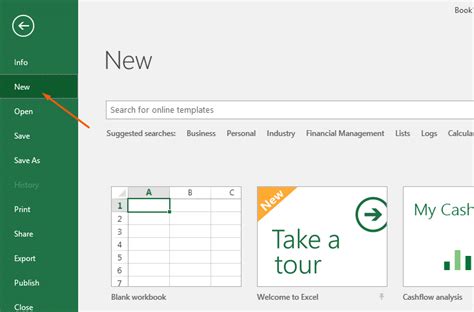
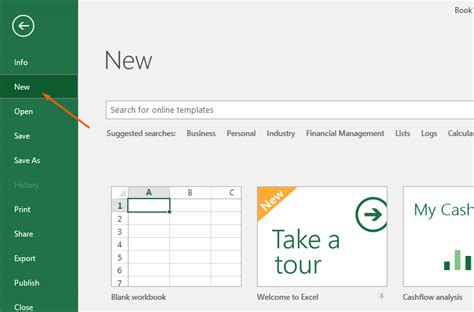
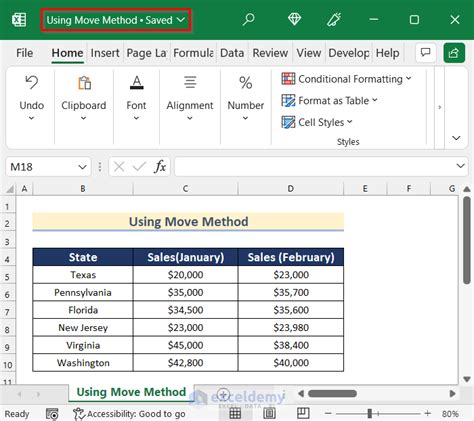
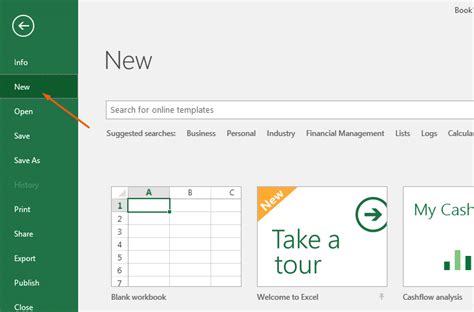
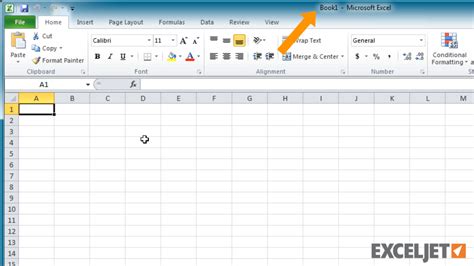
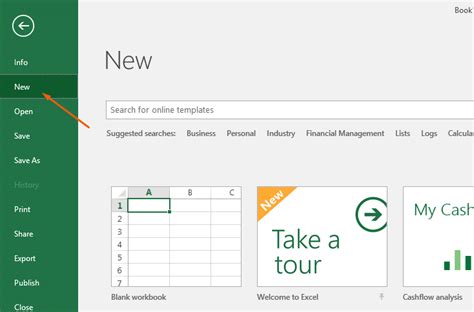
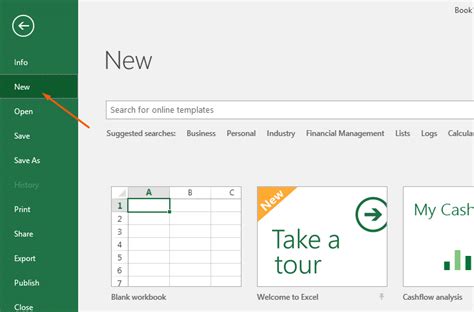
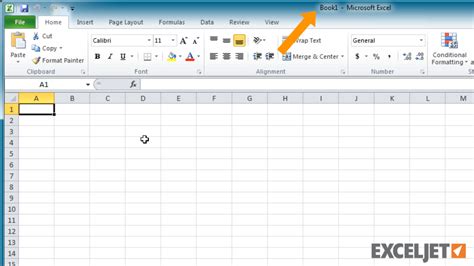
Frequently Asked Questions
Q: How do I create a new workbook using VBA?
A: Use the Workbooks.Add
method to create a new workbook.
Q: How do I save a new workbook with a specific name using VBA?
A: Use the SaveAs
method to save the new workbook with a specific name.
Q: How do I add multiple worksheets to a new workbook using VBA?
A: Use the Worksheets.Add
method to add multiple worksheets to the new workbook.
Q: How do I add formatting to a new workbook using VBA?
A: Use formatting methods, such as Font.Bold
or Range.Value
, to add formatting to the new workbook.
Conclusion
Creating a new workbook using VBA can be a useful skill for automating tasks and increasing productivity in Excel. By following the examples and tips in this article, you can learn how to create a new workbook with specific formatting and layout. Remember to use the Workbooks.Add
method to create a new workbook, and the SaveAs
method to save the new workbook with a specific name. Happy coding!