Intro
Unlock the power of Excel VBA global variables and boost your coding efficiency. Discover 5 effective ways to use global variables in Excel VBA, including declaring, initializing, and applying them in modules, worksheets, and userforms. Improve code readability, reusability, and performance with expert tips on global variable best practices.
In the world of Excel VBA programming, variables are essential for storing and manipulating data. Among these variables, global variables play a crucial role in making your code more efficient and easier to maintain. However, using global variables effectively requires a deep understanding of their scope, declaration, and application. In this article, we will explore five ways to use Excel VBA global variables effectively, ensuring you get the most out of your programming endeavors.
What are Global Variables in Excel VBA?
Before diving into the ways to use global variables effectively, let's first understand what they are. In Excel VBA, a global variable is a variable that is declared outside a procedure or function, making it accessible to all modules within a VBA project. Global variables are typically declared at the top of a module, outside any procedure or function.
Why Use Global Variables in Excel VBA?
Using global variables in Excel VBA can significantly improve the efficiency and readability of your code. Here are a few reasons why:
- Simplified Code: Global variables allow you to avoid passing variables as arguments between procedures, making your code more straightforward and easier to understand.
- Improved Performance: By storing data in global variables, you can avoid repeated calculations and lookups, resulting in faster execution times.
- Easier Maintenance: Global variables provide a centralized location for storing and updating data, making it easier to modify and maintain your code.
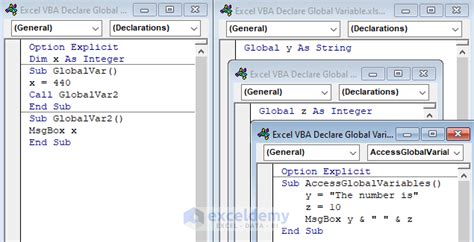
1. Declaring Global Variables
Declaring global variables is a straightforward process. You can declare them at the top of a module, outside any procedure or function, using the Public
or Global
keyword. Here's an example:
Public gSheetName As String
2. Using Global Variables Across Modules
One of the primary advantages of global variables is their ability to be accessed across multiple modules. To use a global variable in another module, you don't need to declare it again or pass it as an argument. Simply use the variable name, and VBA will recognize it. For example:
' Module1
Public gSheetName As String
' Module2
Sub MySub()
Debug.Print gSheetName
End Sub
3. Controlling Global Variables with Functions
Functions can be used to control and manipulate global variables. You can create functions to get or set the value of a global variable, providing a layer of abstraction and security. Here's an example:
Public gUserName As String
Function GetUserName() As String
GetUserName = gUserName
End Function
Sub SetUserName(name As String)
gUserName = name
End Sub
4. Handling Global Variables in Multi-User Environments
In multi-user environments, global variables can be problematic. Since global variables are shared across all instances of a VBA project, changes made by one user can affect others. To handle this, you can use a combination of global variables and user-specific variables or use alternative storage methods, such as databases or external files.
5. Avoiding Common Pitfalls
While global variables can be incredibly useful, they can also lead to issues if not used carefully. Here are a few common pitfalls to avoid:
- Overuse: Avoid using global variables excessively, as this can lead to confusing and hard-to-debug code.
- Namespace Collisions: Be cautious when using global variables with the same name in different modules or projects, as this can lead to namespace collisions.
- Scalability: As your project grows, global variables can become difficult to manage. Consider alternative storage methods or modularize your code to avoid this issue.
Excel VBA Global Variables Gallery

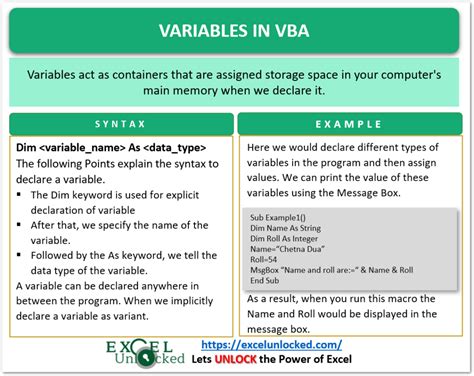
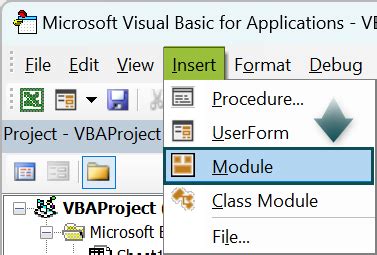
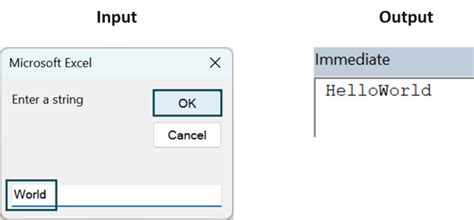
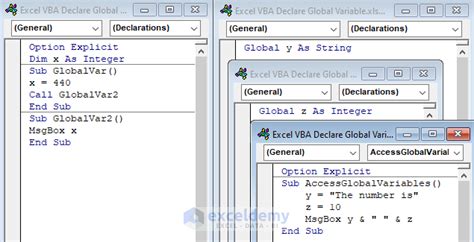
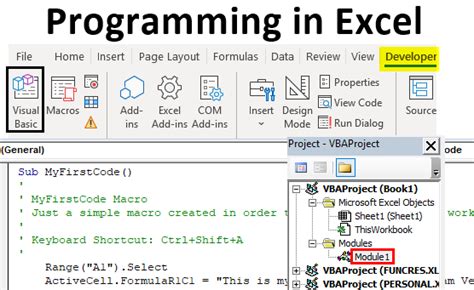
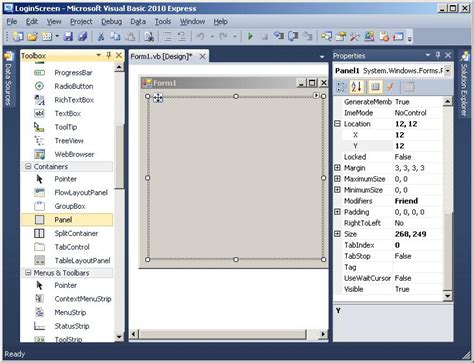
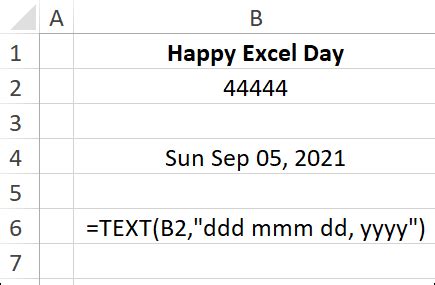
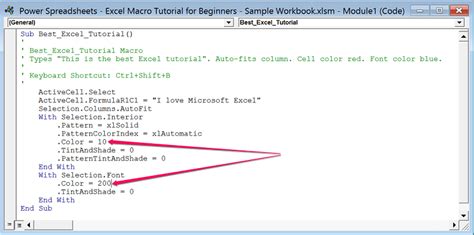
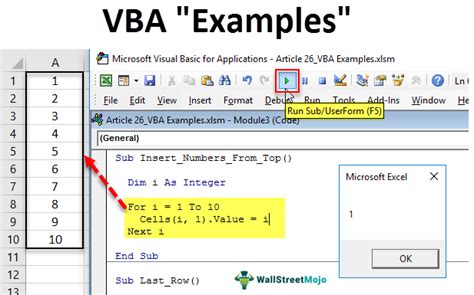
By following these best practices and avoiding common pitfalls, you can effectively utilize Excel VBA global variables to improve the efficiency, readability, and maintainability of your code. Remember to use global variables judiciously and consider alternative storage methods when necessary. Happy coding!