Intro
Master robust error handling in Excel VBA with try-catch blocks. Learn how to identify, trap, and resolve errors using VBAs built-in error handling mechanisms, including the Try-Catch block, On Error statement, and Err object. Improve your VBA coding skills and create more reliable, error-free applications.
Mastering Excel VBA Error Handling with Try-Catch Blocks is an essential skill for any Excel VBA developer. Error handling is a critical aspect of writing robust and reliable code, and Try-Catch blocks are a powerful tool for achieving this goal.
What is Error Handling in Excel VBA?
Error handling is the process of anticipating and managing errors that may occur during the execution of your VBA code. Errors can arise from a variety of sources, including invalid user input, missing or corrupted data, and unexpected changes to the Excel environment. Without proper error handling, your code may crash or produce unexpected results, leading to frustration and lost productivity.
Why Use Try-Catch Blocks for Error Handling?
Try-Catch blocks are a fundamental construct in VBA error handling. They allow you to wrap your code in a try block, which executes the code and catches any errors that occur. The catch block then handles the error, allowing you to provide a meaningful response to the user or take corrective action. Try-Catch blocks provide several benefits, including:
- Improved code reliability: By catching and handling errors, you can prevent your code from crashing or producing unexpected results.
- Enhanced user experience: Try-Catch blocks allow you to provide meaningful error messages and feedback to the user, improving their overall experience.
- Simplified debugging: With Try-Catch blocks, you can identify and diagnose errors more easily, reducing the time and effort required to debug your code.
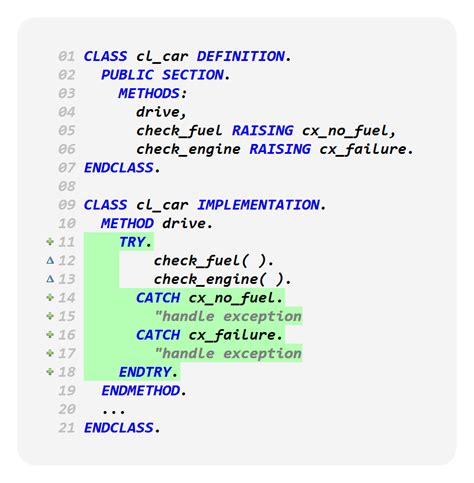
How to Use Try-Catch Blocks in Excel VBA
Using Try-Catch blocks in Excel VBA is straightforward. Here is the basic syntax:
Try
' Code to execute
Catch [Optional] [Exception]
' Error handling code
End Try
The try block contains the code you want to execute, and the catch block handles any errors that occur.
Example: Using Try-Catch Blocks to Handle Errors
Suppose you have a VBA procedure that divides two numbers, and you want to handle the error that occurs when the user attempts to divide by zero. Here is an example of how you can use Try-Catch blocks to achieve this:
Sub DivideNumbers()
Dim numerator As Double
Dim denominator As Double
Dim result As Double
numerator = 10
denominator = 0
Try
result = numerator / denominator
Catch e As Exception
MsgBox "Error: " & e.Description, vbExclamation, "Error"
End Try
End Sub
In this example, the try block attempts to divide the numerator by the denominator. If an error occurs (in this case, a division by zero error), the catch block catches the error and displays a meaningful error message to the user.
Best Practices for Using Try-Catch Blocks
Here are some best practices to keep in mind when using Try-Catch blocks in Excel VBA:
- Keep try blocks short and focused: Try to keep the code in your try block concise and focused on a specific task. This makes it easier to identify and diagnose errors.
- Use specific exception types: Instead of catching the general Exception type, try to catch specific exception types that are relevant to your code. This allows you to provide more targeted error handling.
- Provide meaningful error messages: When handling errors, provide meaningful error messages that help the user understand what went wrong and how to correct the issue.
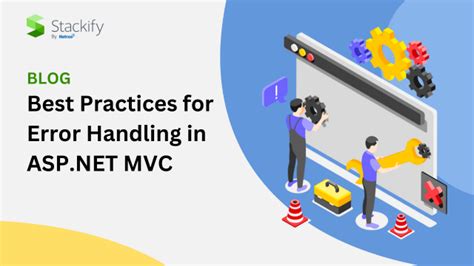
Common Errors in Excel VBA and How to Handle Them
Here are some common errors that can occur in Excel VBA, along with examples of how to handle them using Try-Catch blocks:
- Type mismatch errors: These errors occur when you attempt to assign a value of the wrong data type to a variable. For example:
Sub Example()
Dim x As Integer
Try
x = "Hello"
Catch e As Exception
MsgBox "Error: " & e.Description, vbExclamation, "Error"
End Try
End Sub
- Null pointer exceptions: These errors occur when you attempt to access a null object reference. For example:
Sub Example()
Dim ws As Worksheet
Try
ws.Range("A1").Value = 10
Catch e As Exception
MsgBox "Error: " & e.Description, vbExclamation, "Error"
End Try
End Sub
- Division by zero errors: These errors occur when you attempt to divide a number by zero. For example:
Sub Example()
Dim x As Double
Dim y As Double
Try
x = 10 / 0
Catch e As Exception
MsgBox "Error: " & e.Description, vbExclamation, "Error"
End Try
End Sub
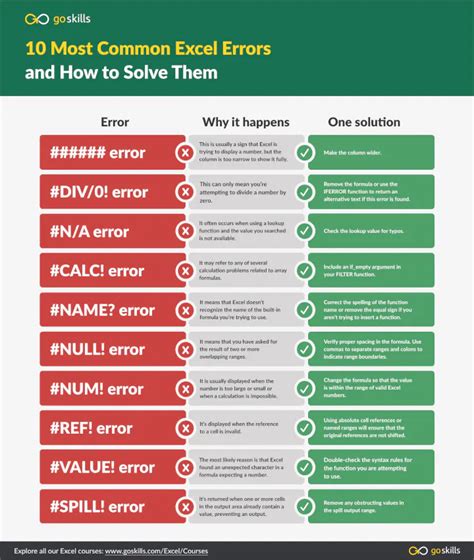
Conclusion
Mastering Excel VBA error handling with Try-Catch blocks is an essential skill for any Excel VBA developer. By using Try-Catch blocks, you can write more robust and reliable code, improve the user experience, and simplify debugging. Remember to keep try blocks short and focused, use specific exception types, and provide meaningful error messages. With practice and experience, you can become proficient in using Try-Catch blocks to handle errors in Excel VBA.
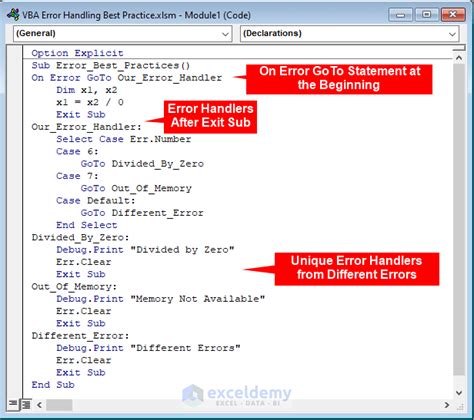
Share Your Thoughts
We hope this article has helped you understand the importance of error handling in Excel VBA and how to use Try-Catch blocks to handle errors. Share your thoughts and experiences with error handling in the comments below. Do you have any questions or topics you would like to discuss? Let us know!
Excel VBA Error Handling Image Gallery
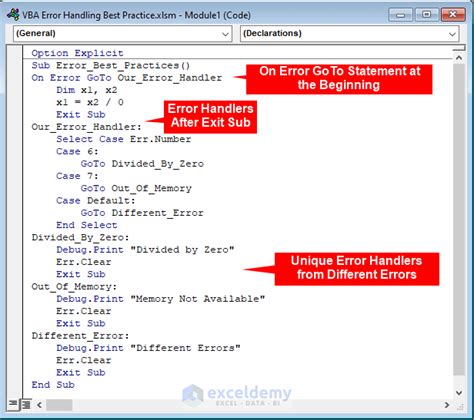
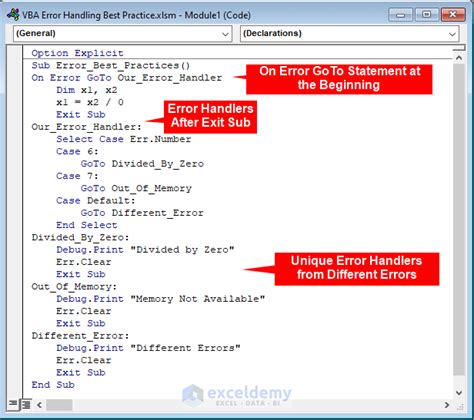
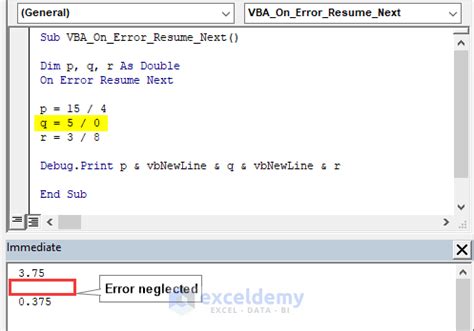
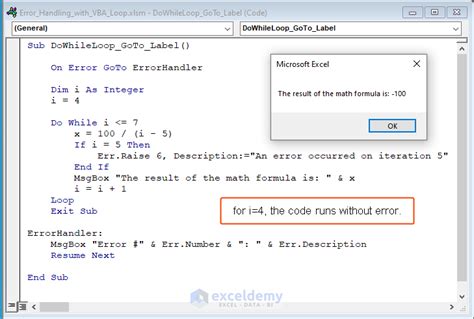
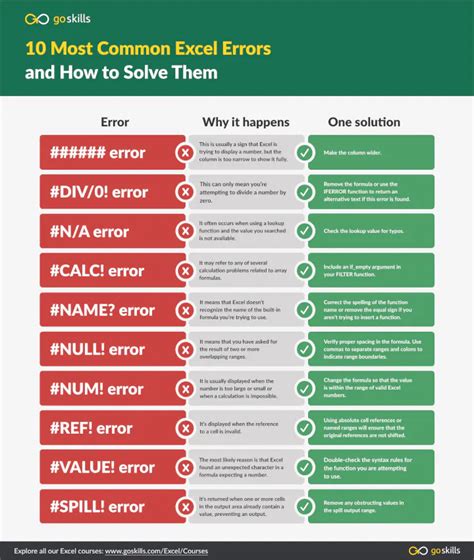
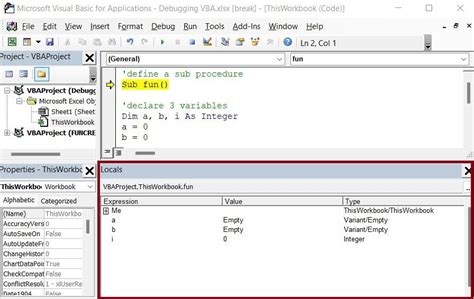
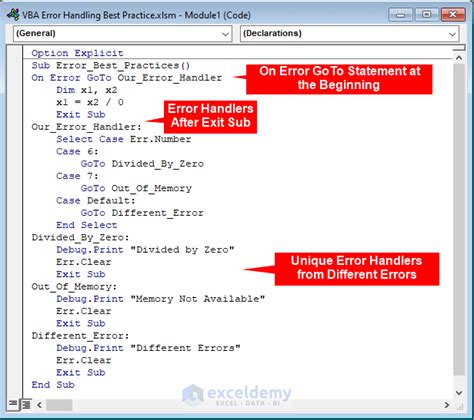
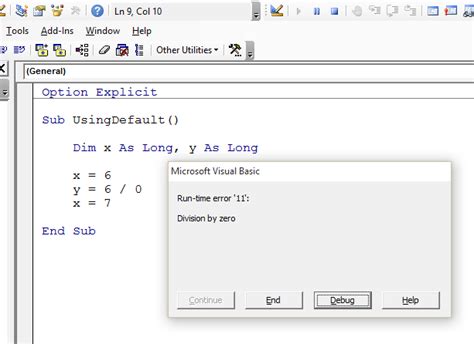
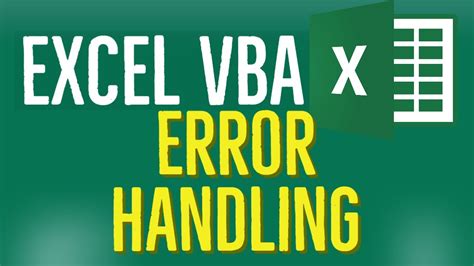
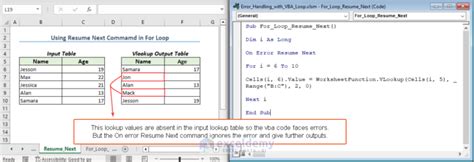
Frequently Asked Questions
Q: What is error handling in Excel VBA? A: Error handling is the process of anticipating and managing errors that may occur during the execution of your VBA code.
Q: What are Try-Catch blocks? A: Try-Catch blocks are a fundamental construct in VBA error handling. They allow you to wrap your code in a try block, which executes the code and catches any errors that occur.
Q: Why use Try-Catch blocks for error handling? A: Try-Catch blocks provide several benefits, including improved code reliability, enhanced user experience, and simplified debugging.
Q: How do I use Try-Catch blocks in Excel VBA? A: Using Try-Catch blocks in Excel VBA is straightforward. You can wrap your code in a try block, and catch any errors that occur in the catch block.
Q: What are some common errors in Excel VBA and how do I handle them? A: Some common errors in Excel VBA include type mismatch errors, null pointer exceptions, and division by zero errors. You can handle these errors using Try-Catch blocks and providing meaningful error messages to the user.