Intro
Mastering the art of filtering in Excel VBA has never been easier! Learn how to clear filters in Excel VBA with our step-by-step guide. Discover how to remove filters, reset data, and optimize your VBA code for seamless automation. Say goodbye to manual filter clearing and hello to streamlined workflows with our expert VBA tips and tricks.
Clearing filters in Excel VBA can be a bit tricky, but with the right approach, it can be made easy. Filters are a great way to narrow down data in a worksheet, but sometimes you need to clear them to view all the data again. In this article, we will explore the different ways to clear filters in Excel VBA, and provide examples and code snippets to help you understand the process.
Why Clear Filters in Excel VBA?
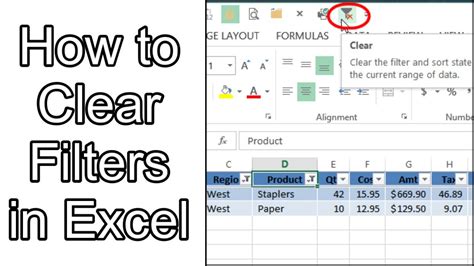
Before we dive into the code, let's talk about why you would want to clear filters in Excel VBA. There are several scenarios where clearing filters is necessary:
- When you want to view all the data in a worksheet after filtering.
- When you want to automate a process that requires clearing filters.
- When you want to create a macro that clears filters as part of a larger process.
Clearing Filters Using the AutoFilter Method
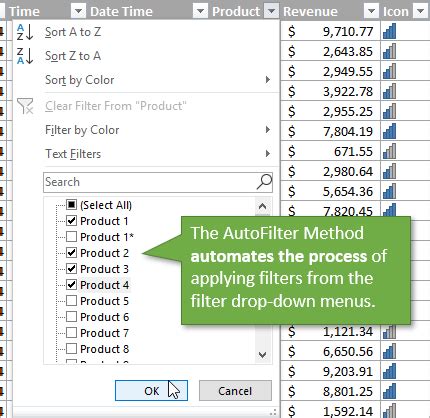
One way to clear filters in Excel VBA is by using the AutoFilter method. This method allows you to clear filters on a specific range of cells. Here is an example of how to use the AutoFilter method:
Sub ClearFiltersUsingAutoFilter()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
' Clear filters on the entire worksheet
ws.AutoFilterMode = False
' Clear filters on a specific range of cells
ws.Range("A1:E10").AutoFilter
End Sub
In this example, the AutoFilterMode
property is set to False
to clear filters on the entire worksheet. The AutoFilter
method is then used to clear filters on a specific range of cells (A1:E10
).
Clearing Filters Using the ShowAllData Method
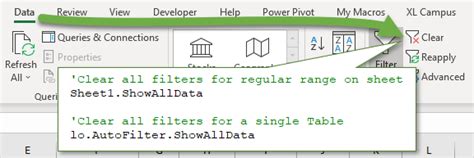
Another way to clear filters in Excel VBA is by using the ShowAllData
method. This method allows you to clear filters on the entire worksheet. Here is an example of how to use the ShowAllData
method:
Sub ClearFiltersUsingShowAllData()
Dim ws As Worksheet
Set ws = ThisWorkbook.Worksheets("Sheet1")
' Clear filters on the entire worksheet
ws.ShowAllData
End Sub
In this example, the ShowAllData
method is used to clear filters on the entire worksheet.
Clearing Filters Using a Loop
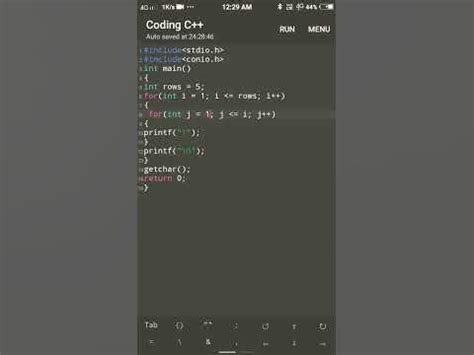
If you need to clear filters on multiple worksheets or ranges, you can use a loop to iterate through the worksheets or ranges and clear the filters. Here is an example of how to use a loop to clear filters:
Sub ClearFiltersUsingLoop()
Dim ws As Worksheet
Dim rng As Range
' Loop through all worksheets
For Each ws In ThisWorkbook.Worksheets
' Clear filters on the entire worksheet
ws.AutoFilterMode = False
Next ws
' Loop through a specific range of cells
For Each rng In ThisWorkbook.Worksheets("Sheet1").Range("A1:E10")
' Clear filters on the range
rng.AutoFilter
Next rng
End Sub
In this example, a loop is used to iterate through all the worksheets in the workbook and clear the filters on each worksheet. Another loop is used to iterate through a specific range of cells and clear the filters on that range.
Best Practices for Clearing Filters in Excel VBA
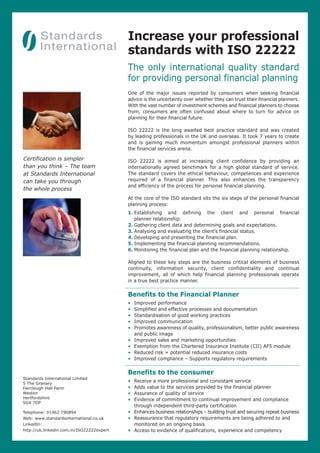
Here are some best practices to keep in mind when clearing filters in Excel VBA:
- Always use the
AutoFilterMode
property to clear filters on the entire worksheet. - Use the
ShowAllData
method to clear filters on the entire worksheet. - Use a loop to iterate through multiple worksheets or ranges and clear the filters.
- Make sure to test your code to ensure that the filters are cleared correctly.
Gallery of Clear Filter Examples:
Clear Filter Examples
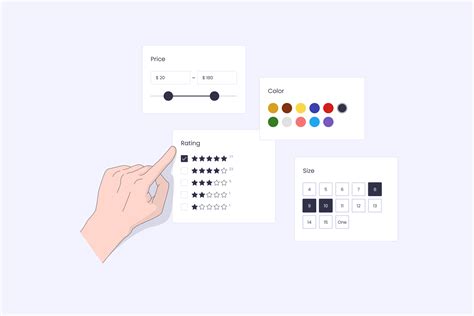
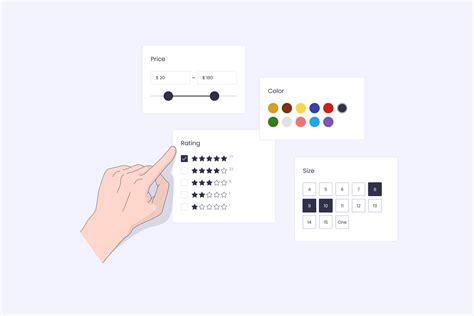
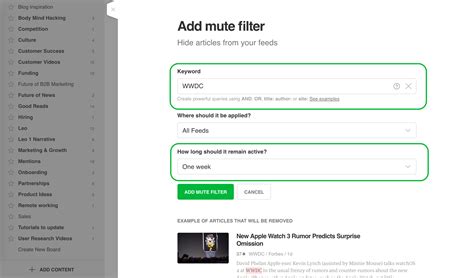
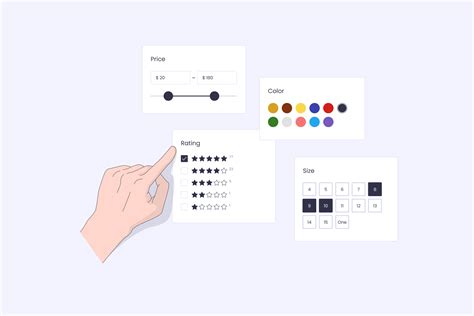
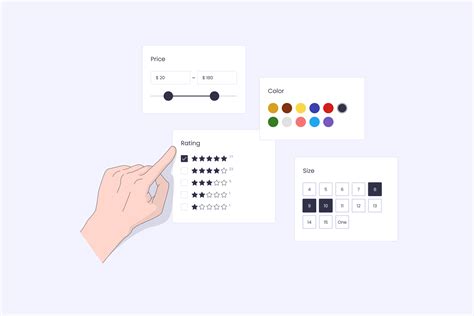
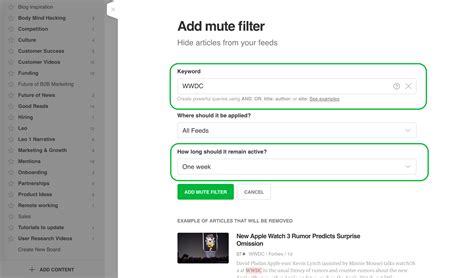
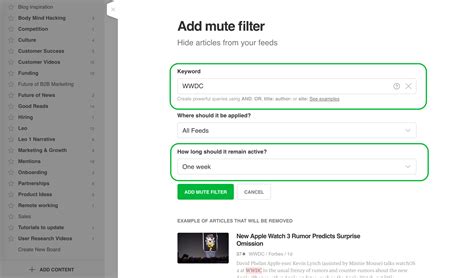
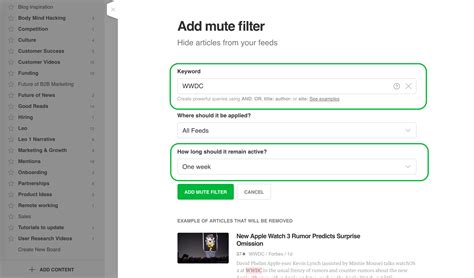
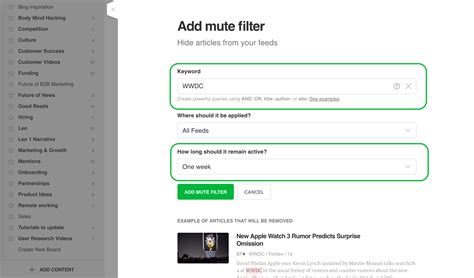
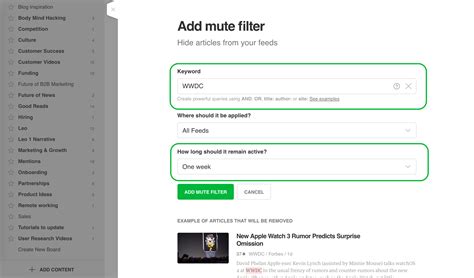