When working with numbers in Excel VBA, rounding values is a common task. Rounding can be necessary for various reasons, such as simplifying calculations, reducing decimal places, or meeting specific formatting requirements. Excel VBA provides several methods to round up numbers, each with its own strengths and use cases. Here, we'll explore five ways to round up in Excel VBA, including their syntax, examples, and practical applications.
1. Using the RoundUp Function
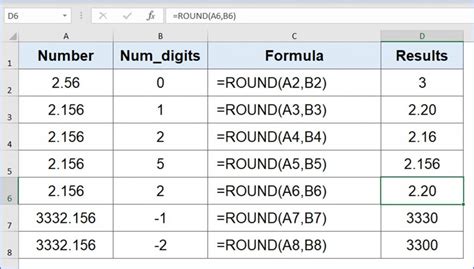
The RoundUp
function is a part of the WorksheetFunction
object in Excel VBA. It rounds a number up to the nearest multiple of a specified value.
Sub RoundUpExample()
Dim num As Double
num = 12.345
' Round up to the nearest integer
MsgBox WorksheetFunction.RoundUp(num, 0)
' Round up to the nearest tenth
MsgBox WorksheetFunction.RoundUp(num, 1)
End Sub
Syntax:
WorksheetFunction.RoundUp(Number, Num_digits)
Number
: The number to be rounded up.Num_digits
: The number of digits to round up to.
2. Using the Ceiling Function
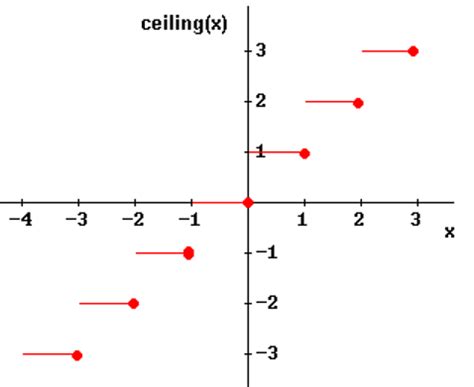
The Ceiling
function is another part of the WorksheetFunction
object in Excel VBA. It returns the smallest integer that is greater than or equal to a given number.
Sub CeilingExample()
Dim num As Double
num = 12.345
' Round up to the nearest integer
MsgBox WorksheetFunction.Ceiling(num)
' Round up to the nearest multiple of 5
MsgBox WorksheetFunction.Ceiling(num, 5)
End Sub
Syntax:
WorksheetFunction.Ceiling(Number, [Significance])
Number
: The number to be rounded up.[Significance]
: The multiple to which the number should be rounded up.
3. Using the Application.WorksheetFunction.RoundUp Method
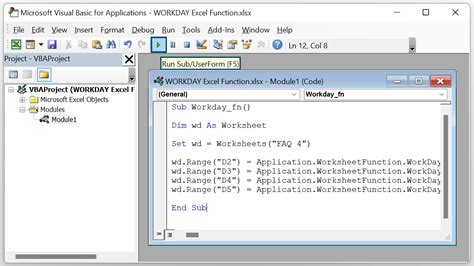
This method is similar to the WorksheetFunction.RoundUp
function but is accessed through the Application
object.
Sub ApplicationRoundUpExample()
Dim num As Double
num = 12.345
' Round up to the nearest integer
MsgBox Application.WorksheetFunction.RoundUp(num, 0)
' Round up to the nearest tenth
MsgBox Application.WorksheetFunction.RoundUp(num, 1)
End Sub
Syntax:
Application.WorksheetFunction.RoundUp(Number, Num_digits)
Number
: The number to be rounded up.Num_digits
: The number of digits to round up to.
4. Using VBA's Built-in Round Function
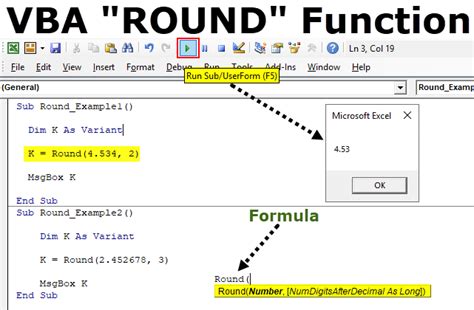
VBA has its own Round
function, which can be used to round numbers up or down.
Sub VARoundExample()
Dim num As Double
num = 12.345
' Round to the nearest integer
MsgBox Round(num)
' Round to the nearest tenth
MsgBox Round(num, 1)
End Sub
Syntax:
Round(Number, [Num_digits])
Number
: The number to be rounded.[Num_digits]
: The number of digits to round to.
Note that this function does not always round up; it rounds to the nearest value based on the specified number of digits.
5. Using a Custom VBA Function
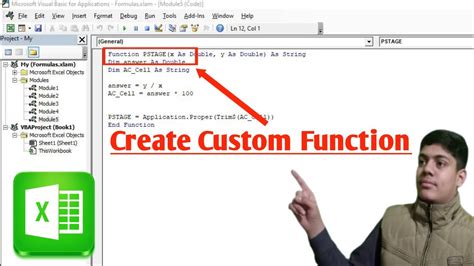
If you need more control over the rounding process or want to create a reusable function, you can create a custom VBA function.
Function RoundUpCustom(num As Double, Optional digits As Integer = 0) As Double
RoundUpCustom = WorksheetFunction.RoundUp(num, digits)
End Function
Syntax:
RoundUpCustom(Number, [Num_digits])
Number
: The number to be rounded up.[Num_digits]
: The number of digits to round up to.
This custom function uses the WorksheetFunction.RoundUp
function but allows for more flexibility and readability in your code.
Excel VBA RoundUp Image Gallery
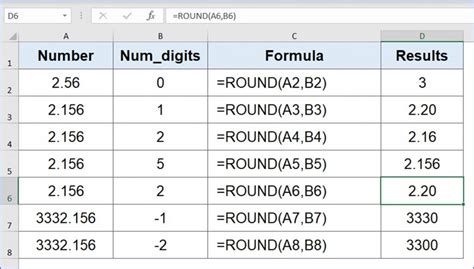
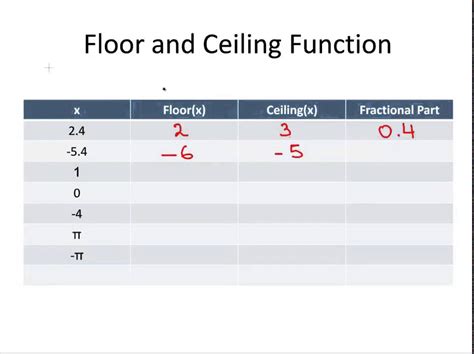
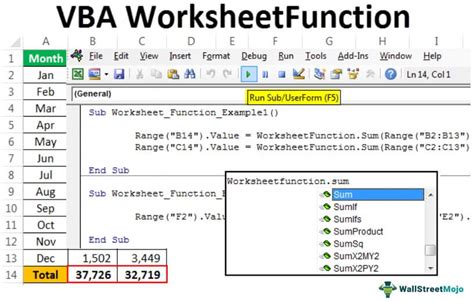
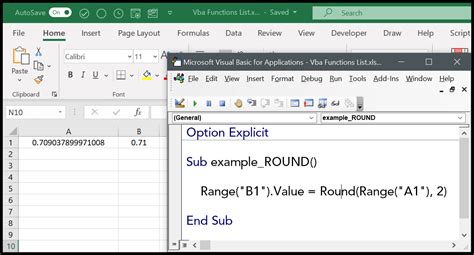
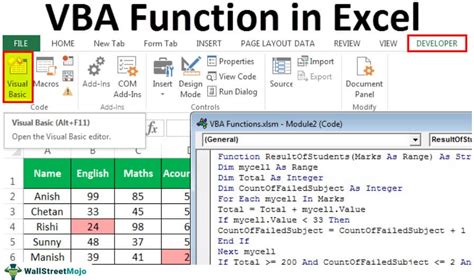
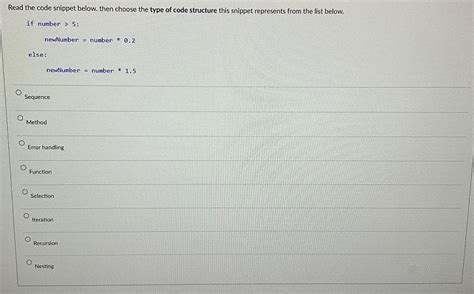
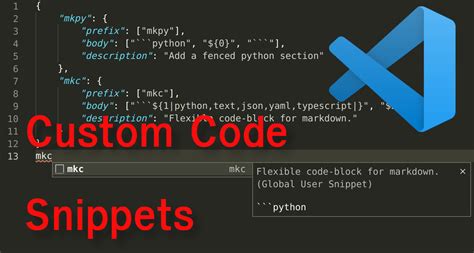
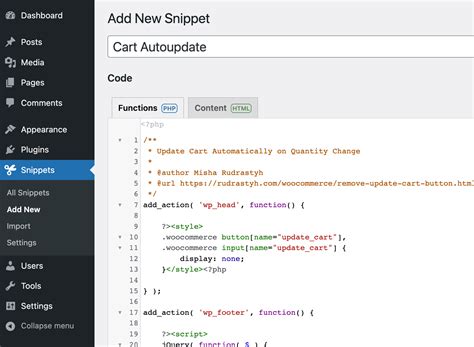
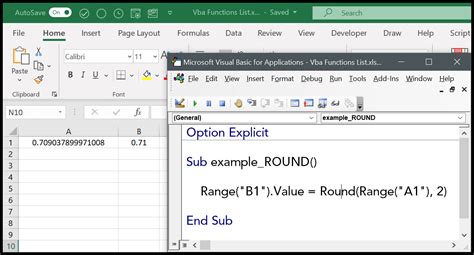
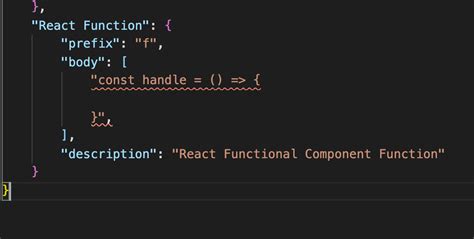
In conclusion, Excel VBA offers multiple ways to round up numbers, each with its own strengths and use cases. By choosing the most suitable method for your specific needs, you can simplify your code, improve readability, and achieve more accurate results.