Intro
Master formatting time in VBA with essential functions and examples. Learn how to work with Date and Time data types, format hours, minutes, and seconds, and create custom time formats using VBAs built-in functions, such as Format, TimeValue, and DateAdd. Improve your VBA skills with this in-depth guide.
Working with dates and times is a common task in VBA programming. Whether you're creating automated reports, scheduling tasks, or analyzing data, understanding how to format time in VBA is crucial. In this article, we'll explore the essential functions and examples for formatting time in VBA.
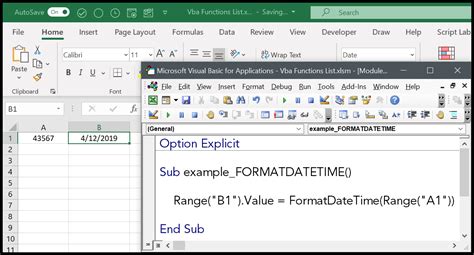
Understanding VBA Time Formats
Before we dive into formatting time, it's essential to understand how VBA stores and displays time. VBA uses the serial number system to represent dates and times. This system represents each date as a unique number, where January 1, 1900, is represented as 1. This system allows for easy calculations and comparisons between dates.
When it comes to time, VBA stores it as a fraction of a day. For example, 12:00 PM is represented as 0.5, while 6:00 AM is represented as 0.25.
VBA Time Formatting Functions
VBA provides several functions for formatting time. Here are some of the most essential ones:
Format Function
The Format function is one of the most versatile functions in VBA. It allows you to format time in various ways, using a combination of symbols and characters.
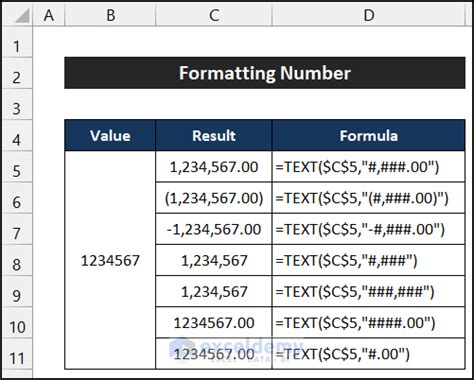
Here are some common time format symbols used in the Format function:
h
orhh
: Displays the hour in 12-hour format (1-12)H
orHH
: Displays the hour in 24-hour format (0-23)m
ormm
: Displays the minute (0-59)s
orss
: Displays the second (0-59)AM/PM
oram/pm
: Displays the time in 12-hour format with AM/PM indicator
Example:
Dim timeValue As Date
timeValue = #12:30:45 PM#
Debug.Print Format(timeValue, "h:mm:ss AM/PM") ' Outputs: 12:30:45 PM
TimeSerial Function
The TimeSerial function returns a time value based on the hour, minute, and second values you provide.
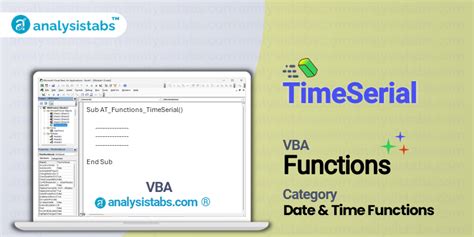
Example:
Dim timeValue As Date
timeValue = TimeSerial(12, 30, 45)
Debug.Print timeValue ' Outputs: 12:30:45 PM
TimeValue Function
The TimeValue function returns a time value based on a string representation of the time.
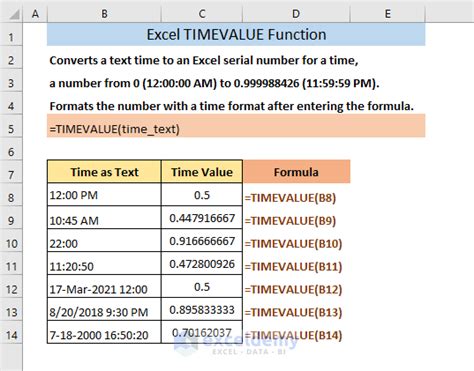
Example:
Dim timeValue As Date
timeValue = TimeValue("12:30:45 PM")
Debug.Print timeValue ' Outputs: 12:30:45 PM
Formatting Time in VBA Examples
Here are some examples of formatting time in VBA:
- Example 1: Displaying time in 12-hour format
Dim timeValue As Date
timeValue = #12:30:45 PM#
Debug.Print Format(timeValue, "h:mm:ss AM/PM") ' Outputs: 12:30:45 PM
- Example 2: Displaying time in 24-hour format
Dim timeValue As Date
timeValue = #12:30:45 PM#
Debug.Print Format(timeValue, "HH:mm:ss") ' Outputs: 12:30:45
- Example 3: Displaying time with milliseconds
Dim timeValue As Date
timeValue = #12:30:45.678 PM#
Debug.Print Format(timeValue, "h:mm:ss.000 AM/PM") ' Outputs: 12:30:45.678 PM
Gallery of Time Formatting Examples
Time Formatting Examples
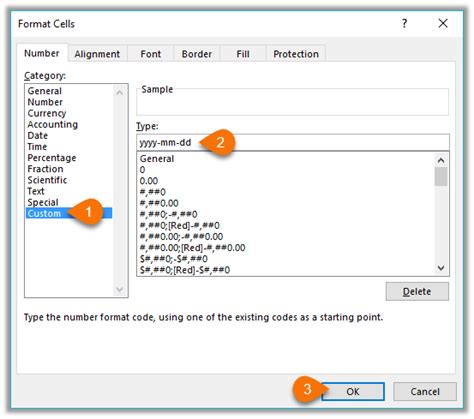
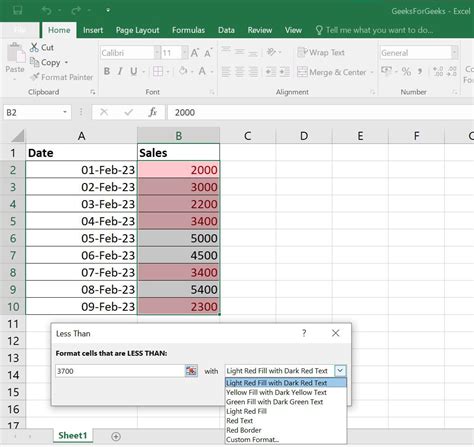
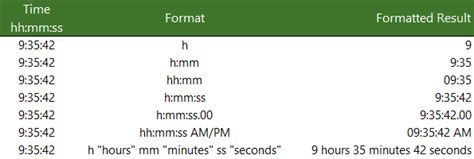
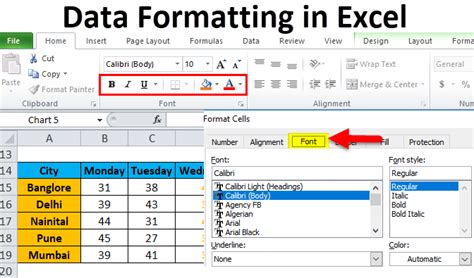
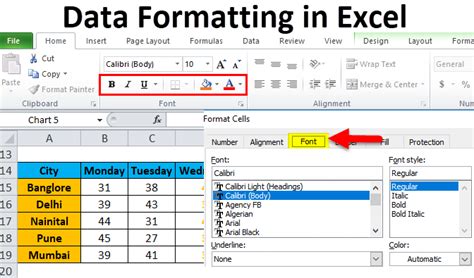
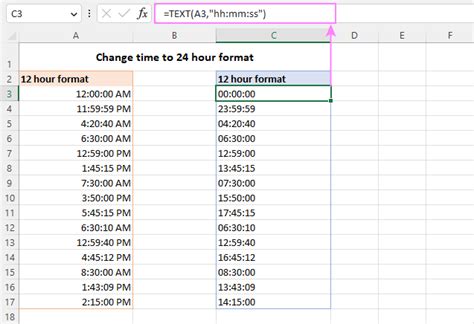
Conclusion
Formatting time in VBA is an essential skill for any programmer. By using the Format function, TimeSerial function, and TimeValue function, you can easily format time in various ways to suit your needs. Whether you're creating automated reports or analyzing data, understanding how to format time in VBA will save you time and effort in the long run. We hope this article has provided you with the essential functions and examples you need to get started with formatting time in VBA.